Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationTypes / MS / Internal / Automation / UiaCoreTypesApi.cs / 1 / UiaCoreTypesApi.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Imports from unmanaged UiaCore DLL // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Security; using System.Runtime.InteropServices; using Microsoft.Internal; namespace MS.Internal.Automation { internal static class UiaCoreTypesApi { //----------------------------------------------------- // // Other API types // //----------------------------------------------------- #region Other internal enum AutomationIdType { Property, Pattern, Event, ControlType, TextAttribute } internal const int UIA_E_ELEMENTNOTENABLED = unchecked((int)0x80040200); internal const int UIA_E_ELEMENTNOTAVAILABLE = unchecked((int)0x80040201); internal const int UIA_E_NOCLICKABLEPOINT = unchecked((int)0x80040202); internal const int UIA_E_PROXYASSEMBLYNOTLOADED = unchecked((int)0x80040203); #endregion Other //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // // Support methods... // ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method simply converts a Guid representing an automation type to an int, making it safe to use. /// [SecurityCritical,SecurityTreatAsSafe] internal static int UiaLookupId(AutomationIdType type, ref Guid guid) { return RawUiaLookupId( type, ref guid ); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method only returns a fixed known object representing an Unsupported value, making it safe to use. /// [SecurityCritical,SecurityTreatAsSafe] internal static object UiaGetReservedNotSupportedValue() { object notSupportedValue; CheckError(RawUiaGetReservedNotSupportedValue(out notSupportedValue)); return notSupportedValue; } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method only returns a fixed known object representing a MixedAttribute value, making it safe to use. /// [SecurityCritical,SecurityTreatAsSafe] internal static object UiaGetReservedMixedAttributeValue() { object mixedAttributeValue; CheckError(RawUiaGetReservedMixedAttributeValue(out mixedAttributeValue)); return mixedAttributeValue; } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Check hresult for error... private static void CheckError(int hr) { if (hr >= 0) { return; } Marshal.ThrowExceptionForHR(hr); } [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaLookupId", CharSet = CharSet.Unicode)] private static extern int RawUiaLookupId(AutomationIdType type, ref Guid guid); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaGetReservedNotSupportedValue", CharSet = CharSet.Unicode)] private static extern int RawUiaGetReservedNotSupportedValue([MarshalAs(UnmanagedType.IUnknown)] out object notSupportedValue); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaGetReservedMixedAttributeValue", CharSet = CharSet.Unicode)] private static extern int RawUiaGetReservedMixedAttributeValue([MarshalAs(UnmanagedType.IUnknown)] out object mixedAttributeValue); #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Imports from unmanaged UiaCore DLL // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Security; using System.Runtime.InteropServices; using Microsoft.Internal; namespace MS.Internal.Automation { internal static class UiaCoreTypesApi { //----------------------------------------------------- // // Other API types // //----------------------------------------------------- #region Other internal enum AutomationIdType { Property, Pattern, Event, ControlType, TextAttribute } internal const int UIA_E_ELEMENTNOTENABLED = unchecked((int)0x80040200); internal const int UIA_E_ELEMENTNOTAVAILABLE = unchecked((int)0x80040201); internal const int UIA_E_NOCLICKABLEPOINT = unchecked((int)0x80040202); internal const int UIA_E_PROXYASSEMBLYNOTLOADED = unchecked((int)0x80040203); #endregion Other //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // // Support methods... // ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method simply converts a Guid representing an automation type to an int, making it safe to use. /// [SecurityCritical,SecurityTreatAsSafe] internal static int UiaLookupId(AutomationIdType type, ref Guid guid) { return RawUiaLookupId( type, ref guid ); } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method only returns a fixed known object representing an Unsupported value, making it safe to use. /// [SecurityCritical,SecurityTreatAsSafe] internal static object UiaGetReservedNotSupportedValue() { object notSupportedValue; CheckError(RawUiaGetReservedNotSupportedValue(out notSupportedValue)); return notSupportedValue; } ////// Critical: This code calls into the unmanaged UIAutomationCore.dll /// TreatAsSafe: This method only returns a fixed known object representing a MixedAttribute value, making it safe to use. /// [SecurityCritical,SecurityTreatAsSafe] internal static object UiaGetReservedMixedAttributeValue() { object mixedAttributeValue; CheckError(RawUiaGetReservedMixedAttributeValue(out mixedAttributeValue)); return mixedAttributeValue; } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Check hresult for error... private static void CheckError(int hr) { if (hr >= 0) { return; } Marshal.ThrowExceptionForHR(hr); } [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaLookupId", CharSet = CharSet.Unicode)] private static extern int RawUiaLookupId(AutomationIdType type, ref Guid guid); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaGetReservedNotSupportedValue", CharSet = CharSet.Unicode)] private static extern int RawUiaGetReservedNotSupportedValue([MarshalAs(UnmanagedType.IUnknown)] out object notSupportedValue); [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(DllImport.UIAutomationCore, EntryPoint = "UiaGetReservedMixedAttributeValue", CharSet = CharSet.Unicode)] private static extern int RawUiaGetReservedMixedAttributeValue([MarshalAs(UnmanagedType.IUnknown)] out object mixedAttributeValue); #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
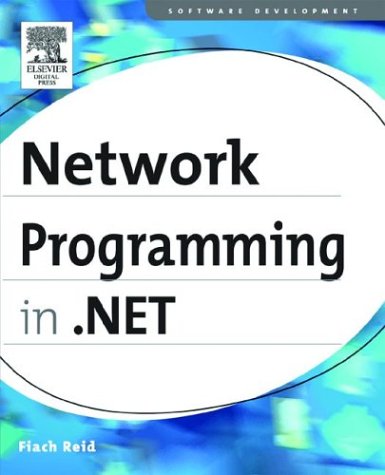
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Maps.cs
- DataViewManager.cs
- TemplatePropertyEntry.cs
- RadioButtonAutomationPeer.cs
- ListBox.cs
- ExecutionEngineException.cs
- SystemParameters.cs
- SqlDelegatedTransaction.cs
- MissingManifestResourceException.cs
- DelegatingTypeDescriptionProvider.cs
- BooleanToVisibilityConverter.cs
- TextDocumentView.cs
- MonitoringDescriptionAttribute.cs
- ArrangedElementCollection.cs
- BuildProvider.cs
- xml.cs
- ToolboxBitmapAttribute.cs
- Baml2006KnownTypes.cs
- SafeUserTokenHandle.cs
- RenderCapability.cs
- SizeChangedEventArgs.cs
- OrthographicCamera.cs
- EntityClientCacheKey.cs
- NetworkStream.cs
- DataObjectAttribute.cs
- SmtpFailedRecipientsException.cs
- NavigationProgressEventArgs.cs
- OciEnlistContext.cs
- MailMessageEventArgs.cs
- KnownColorTable.cs
- FilteredAttributeCollection.cs
- FastEncoder.cs
- MailWriter.cs
- WebBrowser.cs
- NullRuntimeConfig.cs
- ResetableIterator.cs
- ContentElement.cs
- PackageRelationship.cs
- UiaCoreTypesApi.cs
- DiscoveryInnerClientManaged11.cs
- _CookieModule.cs
- ColorInterpolationModeValidation.cs
- EntityConnectionStringBuilder.cs
- XmlEntity.cs
- DataFieldEditor.cs
- XmlSchemaInfo.cs
- ConfigurationPropertyAttribute.cs
- Matrix.cs
- GuidTagList.cs
- Int64AnimationBase.cs
- QilStrConcat.cs
- ZipIOLocalFileBlock.cs
- FileDialogPermission.cs
- HostVisual.cs
- HtmlForm.cs
- CommonServiceBehaviorElement.cs
- FaultPropagationRecord.cs
- StreamGeometry.cs
- AppSecurityManager.cs
- ExitEventArgs.cs
- XmlSchemaSimpleType.cs
- ButtonStandardAdapter.cs
- RegularExpressionValidator.cs
- ReturnEventArgs.cs
- TargetInvocationException.cs
- TextShapeableCharacters.cs
- SpellerStatusTable.cs
- XhtmlBasicCommandAdapter.cs
- DataControlReference.cs
- EffectiveValueEntry.cs
- PropertyBuilder.cs
- MdImport.cs
- WebPartsSection.cs
- CompilationPass2Task.cs
- BufferedReadStream.cs
- ZoneLinkButton.cs
- BitmapSource.cs
- XmlLinkedNode.cs
- DataContractAttribute.cs
- FlowLayoutSettings.cs
- XmlSchemaInferenceException.cs
- RequestTimeoutManager.cs
- InternalTransaction.cs
- SqlFacetAttribute.cs
- ApplicationException.cs
- AsymmetricAlgorithm.cs
- HttpSessionStateBase.cs
- CodeObject.cs
- HttpRawResponse.cs
- DataGridTableCollection.cs
- GuidConverter.cs
- Misc.cs
- FastEncoder.cs
- SystemResourceKey.cs
- SQLConvert.cs
- CompilationSection.cs
- OverlappedAsyncResult.cs
- precedingquery.cs
- wgx_exports.cs
- CompilerParameters.cs