Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / FilteredAttributeCollection.cs / 2 / FilteredAttributeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; ////// Contains a filtered (by device filter) view of the attributes parsed from a tag /// internal sealed class FilteredAttributeDictionary : IDictionary { private string _filter; private IDictionary _data; private ParsedAttributeCollection _owner; internal FilteredAttributeDictionary(ParsedAttributeCollection owner, string filter) { _filter = filter; _owner = owner; _data = new ListDictionary(StringComparer.OrdinalIgnoreCase); } ////// The actual dictionary used for storing the data /// internal IDictionary Data { get { return _data; } } ////// The filter that this collection is filtering on /// public string Filter { get { return _filter; } } ////// Returns the value of a particular attribute for this filter /// public string this[string key] { get { return (string)_data[key]; } set { _owner.ReplaceFilteredAttribute(_filter, key, value); } } ////// Adds a new attribute for this filter /// public void Add(string key, string value) { _owner.AddFilteredAttribute(_filter, key, value); } ////// Clears all attributes for this filter /// public void Clear() { _owner.ClearFilter(_filter); } ////// Returns true if this filtered view contains the specified attribute /// public bool Contains(string key) { return _data.Contains(key); } ////// Removes the specified attribute for this filter /// public void Remove(string key) { _owner.RemoveFilteredAttribute(_filter, key); } #region IDictionary implementation ///bool IDictionary.IsFixedSize { get { return false; } } /// bool IDictionary.IsReadOnly { get { return false; } } /// object IDictionary.this[object key] { get { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return this[key.ToString()]; } set { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } this[key.ToString()] = value.ToString(); } } /// ICollection IDictionary.Keys { get { return _data.Keys; } } /// ICollection IDictionary.Values { get { return _data.Values; } } /// void IDictionary.Add(object key, object value) { if (key == null) { throw new ArgumentNullException("key"); } if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } if (value == null) { value = String.Empty; } Add(key.ToString(), value.ToString()); } /// bool IDictionary.Contains(object key) { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return Contains(key.ToString()); } /// void IDictionary.Clear() { Clear(); } /// IDictionaryEnumerator IDictionary.GetEnumerator() { return _data.GetEnumerator(); } /// void IDictionary.Remove(object key) { Remove(key.ToString()); } #endregion IDictionary implementation #region ICollection implementation /// int ICollection.Count { get { return _data.Count; } } /// bool ICollection.IsSynchronized { get { return ((ICollection)_data).IsSynchronized; } } /// object ICollection.SyncRoot { get { return _data.SyncRoot; } } /// void ICollection.CopyTo(Array array, int index) { _data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return _data.GetEnumerator(); } #endregion ICollection implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; ////// Contains a filtered (by device filter) view of the attributes parsed from a tag /// internal sealed class FilteredAttributeDictionary : IDictionary { private string _filter; private IDictionary _data; private ParsedAttributeCollection _owner; internal FilteredAttributeDictionary(ParsedAttributeCollection owner, string filter) { _filter = filter; _owner = owner; _data = new ListDictionary(StringComparer.OrdinalIgnoreCase); } ////// The actual dictionary used for storing the data /// internal IDictionary Data { get { return _data; } } ////// The filter that this collection is filtering on /// public string Filter { get { return _filter; } } ////// Returns the value of a particular attribute for this filter /// public string this[string key] { get { return (string)_data[key]; } set { _owner.ReplaceFilteredAttribute(_filter, key, value); } } ////// Adds a new attribute for this filter /// public void Add(string key, string value) { _owner.AddFilteredAttribute(_filter, key, value); } ////// Clears all attributes for this filter /// public void Clear() { _owner.ClearFilter(_filter); } ////// Returns true if this filtered view contains the specified attribute /// public bool Contains(string key) { return _data.Contains(key); } ////// Removes the specified attribute for this filter /// public void Remove(string key) { _owner.RemoveFilteredAttribute(_filter, key); } #region IDictionary implementation ///bool IDictionary.IsFixedSize { get { return false; } } /// bool IDictionary.IsReadOnly { get { return false; } } /// object IDictionary.this[object key] { get { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return this[key.ToString()]; } set { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } this[key.ToString()] = value.ToString(); } } /// ICollection IDictionary.Keys { get { return _data.Keys; } } /// ICollection IDictionary.Values { get { return _data.Values; } } /// void IDictionary.Add(object key, object value) { if (key == null) { throw new ArgumentNullException("key"); } if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } if (value == null) { value = String.Empty; } Add(key.ToString(), value.ToString()); } /// bool IDictionary.Contains(object key) { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return Contains(key.ToString()); } /// void IDictionary.Clear() { Clear(); } /// IDictionaryEnumerator IDictionary.GetEnumerator() { return _data.GetEnumerator(); } /// void IDictionary.Remove(object key) { Remove(key.ToString()); } #endregion IDictionary implementation #region ICollection implementation /// int ICollection.Count { get { return _data.Count; } } /// bool ICollection.IsSynchronized { get { return ((ICollection)_data).IsSynchronized; } } /// object ICollection.SyncRoot { get { return _data.SyncRoot; } } /// void ICollection.CopyTo(Array array, int index) { _data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return _data.GetEnumerator(); } #endregion ICollection implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
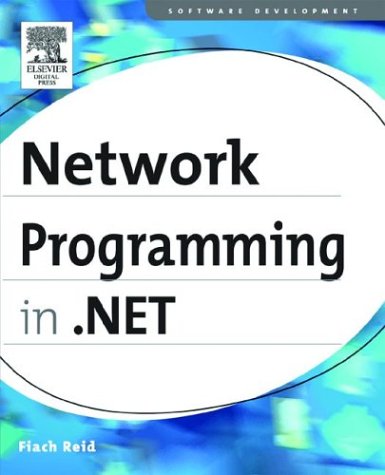
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinHexDecoder.cs
- PropertiesTab.cs
- ToolbarAUtomationPeer.cs
- DefaultMemberAttribute.cs
- BinaryUtilClasses.cs
- WebPartEditVerb.cs
- PropertyItemInternal.cs
- ClientSideQueueItem.cs
- DataViewManagerListItemTypeDescriptor.cs
- PenCursorManager.cs
- RegexStringValidatorAttribute.cs
- SecurityRuntime.cs
- Geometry.cs
- EventSinkHelperWriter.cs
- Pointer.cs
- ReplacementText.cs
- ControlType.cs
- NonParentingControl.cs
- UnsafeMethods.cs
- ImportOptions.cs
- SettingsPropertyWrongTypeException.cs
- StorageFunctionMapping.cs
- EntityDesignerUtils.cs
- SafeLocalMemHandle.cs
- SoapSchemaMember.cs
- SqlDataSourceCommandParser.cs
- PasswordTextContainer.cs
- StretchValidation.cs
- NestPullup.cs
- CuspData.cs
- PresentationSource.cs
- GridProviderWrapper.cs
- RegexInterpreter.cs
- PageCache.cs
- FunctionImportElement.cs
- DataGridViewTopRowAccessibleObject.cs
- FormViewUpdatedEventArgs.cs
- ButtonColumn.cs
- SoapUnknownHeader.cs
- Graphics.cs
- DataReceivedEventArgs.cs
- Parameter.cs
- AnnotationStore.cs
- DesignerSerializationOptionsAttribute.cs
- Size3DValueSerializer.cs
- ToolBar.cs
- VariableExpressionConverter.cs
- FormatterServicesNoSerializableCheck.cs
- OleDragDropHandler.cs
- FixedSOMPageElement.cs
- FontStyleConverter.cs
- SqlDataSourceSelectingEventArgs.cs
- XmlDictionaryString.cs
- ObservableDictionary.cs
- ParentQuery.cs
- AssemblyContextControlItem.cs
- DataControlImageButton.cs
- TransportContext.cs
- HtmlGenericControl.cs
- AppLevelCompilationSectionCache.cs
- ListControl.cs
- ComPersistableTypeElement.cs
- SpellCheck.cs
- WinEventTracker.cs
- GridViewDeletedEventArgs.cs
- webbrowsersite.cs
- SqlDependency.cs
- ProxyElement.cs
- WMIInterop.cs
- SqlError.cs
- MergePropertyDescriptor.cs
- DataGridViewCell.cs
- FrameDimension.cs
- C14NUtil.cs
- BooleanProjectedSlot.cs
- TreeViewImageKeyConverter.cs
- ToolStripDropDown.cs
- FileStream.cs
- CoreSwitches.cs
- ListViewContainer.cs
- XamlContextStack.cs
- XPathNodeHelper.cs
- IgnoreFlushAndCloseStream.cs
- RequestCache.cs
- webbrowsersite.cs
- QueryStatement.cs
- FontInfo.cs
- GraphicsState.cs
- MemoryResponseElement.cs
- Application.cs
- GroupByExpressionRewriter.cs
- FormsAuthenticationCredentials.cs
- _ServiceNameStore.cs
- CharAnimationUsingKeyFrames.cs
- SortedList.cs
- CodeDomConfigurationHandler.cs
- AnnotationResource.cs
- TabRenderer.cs
- WrappedIUnknown.cs
- TableRowCollection.cs