Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / AssemblyContextControlItem.cs / 1305376 / AssemblyContextControlItem.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Hosting { using System; using System.Collections.Generic; using System.Reflection; using System.Runtime; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation.Hosting; using System.IO; using System.Linq; //This class is required by the TypeBrowser - it allows browsing defined types either in VS scenario or in //rehosted scenario. The types are divided into two categories - types defined in local assembly (i.e. the one //contained in current project - for that assembly, types are loaded using GetTypes() method), and all other //referenced types - for them, type list is loaded using GetExportedTypes() method. // //if this object is not set in desinger's Items collection or both members are null, the type //browser will not display "Browse for types" option. [Fx.Tag.XamlVisible(false)] public sealed class AssemblyContextControlItem : ContextItem { public AssemblyName LocalAssemblyName { get; set; } [SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification = "This is by design")] public IListReferencedAssemblyNames { get; set; } public override Type ItemType { get { return typeof(AssemblyContextControlItem); } } public IEnumerable AllAssemblyNamesInContext { get { if ((LocalAssemblyName != null) && LocalAssemblyName.CodeBase != null && (File.Exists(new Uri(LocalAssemblyName.CodeBase).LocalPath))) { yield return LocalAssemblyName.FullName; } foreach (AssemblyName assemblyName in GetEnvironmentAssemblyNames()) { //avoid returning local name twice if (LocalAssemblyName == null || !assemblyName.FullName.Equals(LocalAssemblyName.FullName, StringComparison.Ordinal)) { yield return assemblyName.FullName; } } } } public IEnumerable GetEnvironmentAssemblyNames() { if (this.ReferencedAssemblyNames != null) { return this.ReferencedAssemblyNames; } else { List assemblyNames = new List (); foreach (Assembly assembly in AppDomain.CurrentDomain.GetAssemblies()) { if (!assembly.IsDynamic) { assemblyNames.Add(assembly.GetName()); } } return assemblyNames; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public IEnumerable GetEnvironmentAssemblies (IMultiTargetingSupportService multiTargetingService) { if (this.ReferencedAssemblyNames == null) { return AppDomain.CurrentDomain.GetAssemblies().Where (assembly => !assembly.IsDynamic); } else { List assemblies = new List (); foreach (AssemblyName assemblyName in this.ReferencedAssemblyNames) { Assembly assembly = GetAssembly(assemblyName, multiTargetingService); if (assembly != null) { assemblies.Add(assembly); } } return assemblies; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public static Assembly GetAssembly(AssemblyName assemblyName, IMultiTargetingSupportService multiTargetingService) { Assembly assembly = null; try { if (multiTargetingService != null) { assembly = multiTargetingService.GetReflectionAssembly(assemblyName); } else { assembly = Assembly.Load(assemblyName); } } catch (FileNotFoundException) { //this exception may occur if current project is not compiled yet } catch (FileLoadException) { //the assembly could not be loaded, ignore the error } catch (BadImageFormatException) { //bad assembly } return assembly; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Hosting { using System; using System.Collections.Generic; using System.Reflection; using System.Runtime; using System.Diagnostics.CodeAnalysis; using System.Activities.Presentation.Hosting; using System.IO; using System.Linq; //This class is required by the TypeBrowser - it allows browsing defined types either in VS scenario or in //rehosted scenario. The types are divided into two categories - types defined in local assembly (i.e. the one //contained in current project - for that assembly, types are loaded using GetTypes() method), and all other //referenced types - for them, type list is loaded using GetExportedTypes() method. // //if this object is not set in desinger's Items collection or both members are null, the type //browser will not display "Browse for types" option. [Fx.Tag.XamlVisible(false)] public sealed class AssemblyContextControlItem : ContextItem { public AssemblyName LocalAssemblyName { get; set; } [SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification = "This is by design")] public IList ReferencedAssemblyNames { get; set; } public override Type ItemType { get { return typeof(AssemblyContextControlItem); } } public IEnumerable AllAssemblyNamesInContext { get { if ((LocalAssemblyName != null) && LocalAssemblyName.CodeBase != null && (File.Exists(new Uri(LocalAssemblyName.CodeBase).LocalPath))) { yield return LocalAssemblyName.FullName; } foreach (AssemblyName assemblyName in GetEnvironmentAssemblyNames()) { //avoid returning local name twice if (LocalAssemblyName == null || !assemblyName.FullName.Equals(LocalAssemblyName.FullName, StringComparison.Ordinal)) { yield return assemblyName.FullName; } } } } public IEnumerable GetEnvironmentAssemblyNames() { if (this.ReferencedAssemblyNames != null) { return this.ReferencedAssemblyNames; } else { List assemblyNames = new List (); foreach (Assembly assembly in AppDomain.CurrentDomain.GetAssemblies()) { if (!assembly.IsDynamic) { assemblyNames.Add(assembly.GetName()); } } return assemblyNames; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public IEnumerable GetEnvironmentAssemblies (IMultiTargetingSupportService multiTargetingService) { if (this.ReferencedAssemblyNames == null) { return AppDomain.CurrentDomain.GetAssemblies().Where (assembly => !assembly.IsDynamic); } else { List assemblies = new List (); foreach (AssemblyName assemblyName in this.ReferencedAssemblyNames) { Assembly assembly = GetAssembly(assemblyName, multiTargetingService); if (assembly != null) { assemblies.Add(assembly); } } return assemblies; } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Multi-Targeting makes sense")] public static Assembly GetAssembly(AssemblyName assemblyName, IMultiTargetingSupportService multiTargetingService) { Assembly assembly = null; try { if (multiTargetingService != null) { assembly = multiTargetingService.GetReflectionAssembly(assemblyName); } else { assembly = Assembly.Load(assemblyName); } } catch (FileNotFoundException) { //this exception may occur if current project is not compiled yet } catch (FileLoadException) { //the assembly could not be loaded, ignore the error } catch (BadImageFormatException) { //bad assembly } return assembly; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
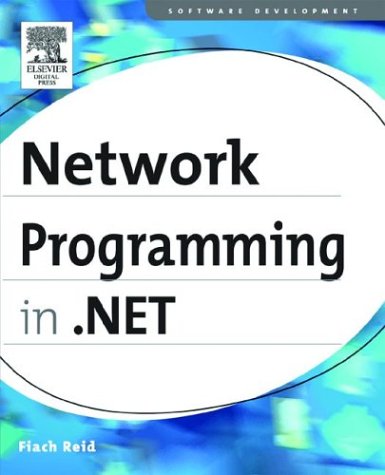
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IDataContractSurrogate.cs
- XmlUrlResolver.cs
- ExtensibleClassFactory.cs
- ConditionalAttribute.cs
- Rotation3D.cs
- SimpleBitVector32.cs
- FontUnitConverter.cs
- IChannel.cs
- HtmlShimManager.cs
- XmlSchemaObject.cs
- DataServicePagingProviderWrapper.cs
- DocumentSchemaValidator.cs
- UniqueConstraint.cs
- ToolStripCollectionEditor.cs
- NameValuePair.cs
- DefaultTextStoreTextComposition.cs
- QilXmlReader.cs
- EventWaitHandle.cs
- ServiceBuildProvider.cs
- DataReaderContainer.cs
- AbsoluteQuery.cs
- FrameworkElementAutomationPeer.cs
- AnnotationHelper.cs
- DelegatedStream.cs
- ProcessHost.cs
- MultiAsyncResult.cs
- Convert.cs
- SqlIdentifier.cs
- HttpListenerRequest.cs
- OwnerDrawPropertyBag.cs
- ChannelManager.cs
- X509SecurityTokenProvider.cs
- ISFTagAndGuidCache.cs
- FixedSOMElement.cs
- PathParser.cs
- UidManager.cs
- PackUriHelper.cs
- ProtocolsSection.cs
- BridgeDataRecord.cs
- NativeRecognizer.cs
- LassoHelper.cs
- IPGlobalProperties.cs
- MemberHolder.cs
- NamespaceInfo.cs
- Schema.cs
- SizeConverter.cs
- ScriptBehaviorDescriptor.cs
- BindingWorker.cs
- DataSourceControlBuilder.cs
- listviewsubitemcollectioneditor.cs
- IntellisenseTextBox.designer.cs
- FontUnitConverter.cs
- OdbcDataAdapter.cs
- _UriSyntax.cs
- TcpProcessProtocolHandler.cs
- HtmlElementCollection.cs
- TextBoxAutomationPeer.cs
- ErrorFormatterPage.cs
- TypeDelegator.cs
- Trustee.cs
- HighlightVisual.cs
- ExpressionParser.cs
- BlockExpression.cs
- DesignerUtility.cs
- ScriptingRoleServiceSection.cs
- ObjectNotFoundException.cs
- ClientSideProviderDescription.cs
- SecurityKeyType.cs
- _HeaderInfo.cs
- HasCopySemanticsAttribute.cs
- ColumnPropertiesGroup.cs
- DataKeyArray.cs
- JoinElimination.cs
- SafeArrayRankMismatchException.cs
- DropShadowBitmapEffect.cs
- UrlAuthFailedErrorFormatter.cs
- glyphs.cs
- XmlSigningNodeWriter.cs
- CodeCastExpression.cs
- SqlDataSourceStatusEventArgs.cs
- DrawingContext.cs
- BindingNavigator.cs
- DtrList.cs
- SecureUICommand.cs
- SafeMemoryMappedViewHandle.cs
- DataGridViewButtonColumn.cs
- StdValidatorsAndConverters.cs
- SelectionItemPattern.cs
- UnauthorizedWebPart.cs
- AssemblyBuilderData.cs
- UnaryNode.cs
- VectorConverter.cs
- SpAudioStreamWrapper.cs
- ColorConverter.cs
- EntitySqlException.cs
- CommandBinding.cs
- UnauthorizedAccessException.cs
- ConfigXmlWhitespace.cs
- CursorConverter.cs
- WorkflowIdleElement.cs