Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / Mail / DelegatedStream.cs / 1 / DelegatedStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Net.Sockets; using System.IO; internal class DelegatedStream : Stream { Stream stream; NetworkStream netStream; protected DelegatedStream() { } protected DelegatedStream(Stream stream) { if (stream == null) throw new ArgumentNullException("stream"); this.stream = stream; netStream = stream as NetworkStream; } protected Stream BaseStream { get { return this.stream; } } public override bool CanRead { get { return this.stream.CanRead; } } public override bool CanSeek { get { return this.stream.CanSeek; } } public override bool CanWrite { get { return this.stream.CanWrite; } } public override long Length { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Length; } } public override long Position { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Position; } set { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.Position = value; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginRead (buffer, offset, count, callback, state); } else{ result = this.stream.BeginRead (buffer, offset, count, callback, state); } return result; } public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginWrite(buffer, offset, count, callback, state); } else{ result = this.stream.BeginWrite (buffer, offset, count, callback, state); } return result; } //This calls close on the inner stream //however, the stream may not be actually closed, but simpy flushed public override void Close() { this.stream.Close(); } public override int EndRead(IAsyncResult asyncResult) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.EndRead (asyncResult); return read; } public override void EndWrite(IAsyncResult asyncResult) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.EndWrite (asyncResult); } public override void Flush() { this.stream.Flush(); } public override int Read(byte[] buffer, int offset, int count) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.Read(buffer, offset, count); return read; } public override long Seek(long offset, SeekOrigin origin) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); long position = this.stream.Seek(offset, origin); return position; } public override void SetLength(long value) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.SetLength(value); } public override void Write(byte[] buffer, int offset, int count) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.Write(buffer, offset, count); } } }
Link Menu
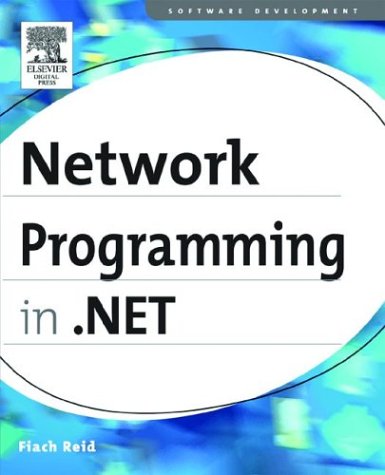
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConditionalWeakTable.cs
- InvocationExpression.cs
- TextInfo.cs
- SoapExtensionStream.cs
- ColumnWidthChangedEvent.cs
- QilSortKey.cs
- EventProviderClassic.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- TitleStyle.cs
- SamlAttributeStatement.cs
- Vector3DKeyFrameCollection.cs
- ClientScriptManager.cs
- Matrix3D.cs
- InputProcessorProfiles.cs
- SystemSounds.cs
- DbXmlEnabledProviderManifest.cs
- sortedlist.cs
- DrawingCollection.cs
- HwndSource.cs
- DataColumnPropertyDescriptor.cs
- altserialization.cs
- SqlCommandAsyncResult.cs
- RawStylusInput.cs
- Container.cs
- _PooledStream.cs
- StrokeSerializer.cs
- Stack.cs
- StructuredType.cs
- FolderBrowserDialog.cs
- safex509handles.cs
- HtmlTernaryTree.cs
- SessionPageStatePersister.cs
- CharacterBufferReference.cs
- AsyncCompletedEventArgs.cs
- SQLInt32.cs
- EntityAdapter.cs
- HttpProcessUtility.cs
- ProvidePropertyAttribute.cs
- CollectionViewSource.cs
- KeyManager.cs
- DocumentSignatureManager.cs
- FormatSettings.cs
- SqlDataSourceTableQuery.cs
- StateDesigner.cs
- METAHEADER.cs
- ListBindingConverter.cs
- LockedAssemblyCache.cs
- ContentType.cs
- StateInitializationDesigner.cs
- Point3DConverter.cs
- PropertyValueUIItem.cs
- GetMemberBinder.cs
- SessionStateModule.cs
- FieldNameLookup.cs
- ETagAttribute.cs
- X509CertificateInitiatorServiceCredential.cs
- ImageSource.cs
- BaseValidator.cs
- HttpBrowserCapabilitiesWrapper.cs
- ToolStripOverflowButton.cs
- MetadataItemCollectionFactory.cs
- FixedSOMFixedBlock.cs
- CodeMethodInvokeExpression.cs
- IdentityManager.cs
- CodePageEncoding.cs
- UpdatePanelTriggerCollection.cs
- ValidatorUtils.cs
- RegionIterator.cs
- XmlReflectionMember.cs
- InstanceLockTracking.cs
- StdValidatorsAndConverters.cs
- AdRotator.cs
- StructuredTypeEmitter.cs
- thaishape.cs
- __Error.cs
- BuildProviderUtils.cs
- _FtpDataStream.cs
- SchemaSetCompiler.cs
- InnerItemCollectionView.cs
- BamlRecordHelper.cs
- MenuItem.cs
- MeshGeometry3D.cs
- RelatedEnd.cs
- SiteMembershipCondition.cs
- EditorPartChrome.cs
- _ListenerAsyncResult.cs
- UriTemplateTable.cs
- DateTimeConstantAttribute.cs
- IISUnsafeMethods.cs
- SpecialNameAttribute.cs
- DocumentsTrace.cs
- GridViewRowEventArgs.cs
- WorkerRequest.cs
- Utils.cs
- Menu.cs
- KeyValueInternalCollection.cs
- Domain.cs
- StylusPointPropertyId.cs
- LayoutSettings.cs
- ThemeDirectoryCompiler.cs