Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / DrawingCollection.cs / 1 / DrawingCollection.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains non-generated DrawingCollection // methods. // // History: // // 2005/03/18 : [....] - Created it. // //--------------------------------------------------------------------------- using System.Collections; using System.Collections.Generic; using System.Windows.Media.Animation; using System.Windows.Markup; namespace System.Windows.Media { ////// Collection of Drawing objects /// public sealed partial class DrawingCollection : Animatable, IList, IList{ /// /// Appends the entire input DrawingCollection, while only firing a single set of /// public events. If an exception is thrown from the public events, the /// Append operation is rolled back. /// internal void TransactionalAppend(DrawingCollection collectionToAppend) { // Use appendCount to avoid inconsistencies & runaway loops when // this == collectionToAppend, and to ensure collectionToAppend.Count // is only evaluated once. int appendCount = collectionToAppend.Count; // First, append the collection for(int i = 0; i < appendCount; i++) { AddWithoutFiringPublicEvents(collectionToAppend.Internal_GetItem(i)); } // Fire the public Changed event after all the elements have been added. // // If an exception is thrown, then the Append operation is rolled-back without // firing additional events. try { FireChanged(); } catch (Exception) { // Compute the number of elements that existed before the append int beforeAppendCount = Count - appendCount; // Remove the appended elements in reverse order without firing Changed events. for ( int i = Count - 1; // Start at the current last index i >= beforeAppendCount; // Until the previous last index i-- // Move to the preceding index ) { RemoveAtWithoutFiringPublicEvents(i); } // Avoid firing WritePostscript events (e.g., OnChanged) after rolling-back // the current operation. // // This ensures that only a single set of events is fired for both exceptional & // typical cases, and it's likely that firing events would cause another exception. throw; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
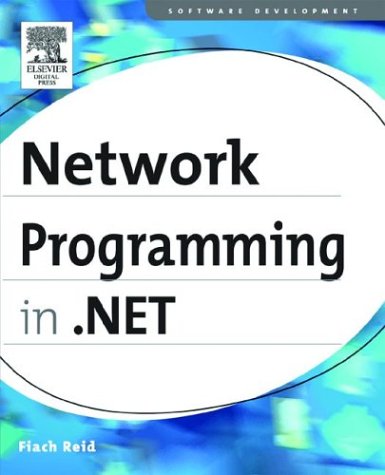
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReversePositionQuery.cs
- Base64Decoder.cs
- SoapElementAttribute.cs
- AutomationPattern.cs
- FormViewUpdateEventArgs.cs
- MsmqActivation.cs
- RelationshipConverter.cs
- JsonGlobals.cs
- DataObjectMethodAttribute.cs
- Validator.cs
- ServiceDescription.cs
- SmtpDigestAuthenticationModule.cs
- EncodingNLS.cs
- VectorValueSerializer.cs
- EntityDataSourceContainerNameItem.cs
- NativeMethods.cs
- HtmlHead.cs
- StringCollection.cs
- prompt.cs
- ClientScriptManagerWrapper.cs
- AppDomain.cs
- UriTemplateTable.cs
- Figure.cs
- DispatcherBuilder.cs
- ThreadWorkerController.cs
- PropertyGroupDescription.cs
- ProfileSection.cs
- SessionParameter.cs
- OdbcParameter.cs
- TemplateNodeContextMenu.cs
- Vars.cs
- Hex.cs
- DataServiceException.cs
- MethodImplAttribute.cs
- Transform.cs
- GiveFeedbackEvent.cs
- DefaultMemberAttribute.cs
- InputLanguage.cs
- BaseValidatorDesigner.cs
- ReaderWriterLockWrapper.cs
- SatelliteContractVersionAttribute.cs
- Transform.cs
- DetailsViewAutoFormat.cs
- GenericEnumerator.cs
- RightsManagementEncryptedStream.cs
- FtpCachePolicyElement.cs
- XmlAttributeProperties.cs
- IntegerFacetDescriptionElement.cs
- BitmapEffectInput.cs
- HandlerBase.cs
- PropertyContainer.cs
- ProgressBar.cs
- EntitySetBaseCollection.cs
- SQLRoleProvider.cs
- SharedStatics.cs
- xsdvalidator.cs
- TypeDescriptor.cs
- _SslStream.cs
- DebugView.cs
- DecoratedNameAttribute.cs
- UriSectionData.cs
- ValueQuery.cs
- PropertyInfoSet.cs
- CommandBindingCollection.cs
- SchemaMapping.cs
- IdleTimeoutMonitor.cs
- SecurityIdentifierConverter.cs
- ColorConverter.cs
- AppSettingsExpressionBuilder.cs
- TimeSpanMinutesConverter.cs
- SystemResources.cs
- BinaryObjectReader.cs
- TaskForm.cs
- TypeDescriptor.cs
- AdapterDictionary.cs
- TextParagraphCache.cs
- ImportDesigner.xaml.cs
- ActivityCollectionMarkupSerializer.cs
- AnonymousIdentificationSection.cs
- LiteralControl.cs
- SmtpCommands.cs
- DependencyObject.cs
- SimpleLine.cs
- AnimationStorage.cs
- ListViewTableRow.cs
- SystemTcpStatistics.cs
- ExtensionWindow.cs
- EncodedStreamFactory.cs
- CompiledELinqQueryState.cs
- Vector3DValueSerializer.cs
- ParameterBinding.cs
- ConnectionConsumerAttribute.cs
- WebBrowsableAttribute.cs
- XPathMultyIterator.cs
- PropertyValue.cs
- LongTypeConverter.cs
- SqlCacheDependencyDatabase.cs
- DefaultTraceListener.cs
- OleStrCAMarshaler.cs
- ConnectionManagementSection.cs