Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Shared / MS / Internal / GenericEnumerator.cs / 1 / GenericEnumerator.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: GenericEnumerator.cs //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Windows; using MS.Utility; #if PRESENTATION_CORE using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #else using SR=System.Windows.SR; using SRID=System.Windows.SRID; #endif namespace MS.Internal { ////// GenericEnumerator /// internal class GenericEnumerator : IEnumerator { #region Delegates internal delegate int GetGenerationIDDelegate(); #endregion #region Constructors private GenericEnumerator() { } internal GenericEnumerator(IList array, GetGenerationIDDelegate getGenerationID) { _array = array; _count = _array.Count; _position = -1; _getGenerationID = getGenerationID; _originalGenerationID = _getGenerationID(); } #endregion #region Private private void VerifyCurrent() { if ( (-1 == _position) || (_position >= _count)) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); } } #endregion #region IEnumerator ////// Returns the object at the current location of the key times list. /// Use the strongly typed version instead. /// object IEnumerator.Current { get { VerifyCurrent(); return _current; } } ////// Move to the next value in the key times list /// ///true if succeeded, false if at the end of the list public bool MoveNext() { if (_getGenerationID() != _originalGenerationID) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } _position++; if (_position >= _count) { _position = _count; return false; } else { Debug.Assert(_position >= 0); _current = _array[_position]; return true; } } ////// Move to the position before the first value in the list. /// public void Reset() { if (_getGenerationID() != _originalGenerationID) { throw new InvalidOperationException(SR.Get(SRID.Enumerator_CollectionChanged)); } else { _position = -1; } } #endregion #region Data private IList _array; private object _current; private int _count; private int _position; private int _originalGenerationID; private GetGenerationIDDelegate _getGenerationID; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
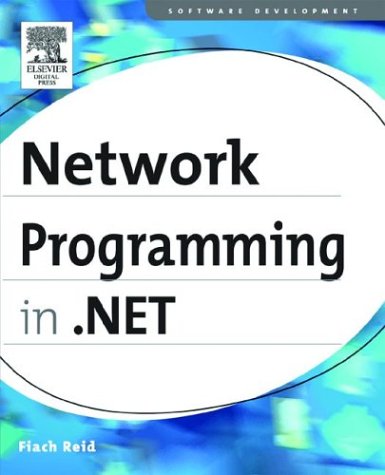
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IISUnsafeMethods.cs
- ParameterToken.cs
- SocketPermission.cs
- EnumMember.cs
- DataRecordObjectView.cs
- PreloadedPackages.cs
- TextControl.cs
- XPathNavigatorReader.cs
- DtcInterfaces.cs
- DependencyObjectPropertyDescriptor.cs
- DataGridViewColumnCollection.cs
- TemplateEditingFrame.cs
- DataKeyCollection.cs
- DefaultValueAttribute.cs
- EntityViewContainer.cs
- TextLineBreak.cs
- ThreadAttributes.cs
- DrawingContext.cs
- ViewValidator.cs
- EntityStoreSchemaFilterEntry.cs
- GestureRecognitionResult.cs
- NullableFloatSumAggregationOperator.cs
- InputBinder.cs
- ExplicitDiscriminatorMap.cs
- SqlGenericUtil.cs
- ByteAnimationBase.cs
- KerberosTicketHashIdentifierClause.cs
- CategoryNameCollection.cs
- RecordManager.cs
- PagerSettings.cs
- DependencyPropertyKey.cs
- ObjectSpanRewriter.cs
- DataServiceHost.cs
- PropagatorResult.cs
- TextSelectionHighlightLayer.cs
- metadatamappinghashervisitor.cs
- CacheManager.cs
- Line.cs
- RijndaelManaged.cs
- DbProviderConfigurationHandler.cs
- ReservationCollection.cs
- TreeNode.cs
- PerformanceCounterLib.cs
- FormsAuthenticationUser.cs
- LazyTextWriterCreator.cs
- MetadataArtifactLoaderResource.cs
- ResourceManager.cs
- MasterPageParser.cs
- ToolTipService.cs
- SubpageParaClient.cs
- DataGridViewSelectedRowCollection.cs
- Misc.cs
- WindowsMenu.cs
- SimpleTextLine.cs
- GroupBoxRenderer.cs
- ActiveXHost.cs
- IERequestCache.cs
- AssociatedControlConverter.cs
- TransformBlockRequest.cs
- SqlDataSourceCommandEventArgs.cs
- DependencyPropertyChangedEventArgs.cs
- IgnoreFileBuildProvider.cs
- NavigationCommands.cs
- FrugalList.cs
- CatalogZoneBase.cs
- SafeNativeMethodsOther.cs
- SQLByte.cs
- TraceSwitch.cs
- ReceiveSecurityHeader.cs
- RoutingChannelExtension.cs
- PageAsyncTaskManager.cs
- StorageModelBuildProvider.cs
- ProgressiveCrcCalculatingStream.cs
- XPathNavigatorKeyComparer.cs
- DefaultTextStore.cs
- RootDesignerSerializerAttribute.cs
- ContainerParagraph.cs
- _BasicClient.cs
- WindowsListViewSubItem.cs
- HtmlLink.cs
- AliasedSlot.cs
- XamlTreeBuilderBamlRecordWriter.cs
- MemoryFailPoint.cs
- XhtmlBasicValidationSummaryAdapter.cs
- RangeValidator.cs
- FloaterParagraph.cs
- CodeDomDesignerLoader.cs
- HttpHandlersSection.cs
- GeometryHitTestResult.cs
- TreeNodeStyleCollection.cs
- CodeTypeOfExpression.cs
- DbConnectionInternal.cs
- TiffBitmapDecoder.cs
- DSASignatureDeformatter.cs
- StorageComplexPropertyMapping.cs
- PropertyPathWorker.cs
- FollowerQueueCreator.cs
- NameGenerator.cs
- HttpResponseHeader.cs
- IfAction.cs