Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Text / InlineObject.cs / 1305600 / InlineObject.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: InlineObject.cs // // Description: Cached metrics of an inline objects. // // History: // 04/25/2003 : [....] - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; namespace MS.Internal.Text { ////// Inline object representation as TextRun. /// internal sealed class InlineObject: TextEmbeddedObject { ////// Constructor. /// /// Text position of the inline object in the text array. /// Number of text position in the text array occupied by the inline object. /// UIElement representing the inline object. /// Text run properties for the inline object. /// TextBlock element - the host of the inline object. internal InlineObject(int dcp, int cch, UIElement element, TextRunProperties textProps, System.Windows.Controls.TextBlock host) { _dcp = dcp; _cch = cch; _element = element; _textProps = textProps; _host = host; } ////// Get inline object's measurement metrics. /// /// Remaining paragraph width. ///Inline object metrics. public override TextEmbeddedObjectMetrics Format(double remainingParagraphWidth) { Size desiredSize = _host.MeasureChild(this); // Make sure that LS/PTS limitations are not exceeded for object's size. TextDpi.EnsureValidObjSize(ref desiredSize); double baseline = desiredSize.Height; double baselineOffsetValue = (double) Element.GetValue(TextBlock.BaselineOffsetProperty); if(!DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = baselineOffsetValue; } return new TextEmbeddedObjectMetrics(desiredSize.Width, desiredSize.Height, baseline); } ////// Get computed bounding box of the inline object. /// /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. ///Computed bounding box size of text object. public override Rect ComputeBoundingBox(bool rightToLeft, bool sideways) { if (_element.IsArrangeValid) { // Initially assume that bounding box is the same as layout box. Size size = _element.DesiredSize; double baseline = !sideways ? size.Height : size.Width; double baselineOffsetValue = (double)Element.GetValue(TextBlock.BaselineOffsetProperty); if (!sideways && !DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = (double)baselineOffsetValue; } return new Rect(0, -baseline, sideways ? size.Height : size.Width, sideways ? size.Width : size.Height); } else { return Rect.Empty; } } ////// Draw the inline object. /// /// Drawing context. /// Origin where the object is drawn. /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. public override void Draw(DrawingContext drawingContext, Point origin, bool rightToLeft, bool sideways) { // Inline object has its own visual and it is attached to a visual // tree during arrange process. // Do nothing here. } ////// Reference to character buffer. /// public override CharacterBufferReference CharacterBufferReference { get { return new CharacterBufferReference(String.Empty, 0); } } ////// Length of the inline object run. /// public override int Length { get { return _cch; } } ////// A set of properties shared by every characters in the run /// public override TextRunProperties Properties { get { return _textProps; } } ////// Line break condition before the inline object. /// public override LineBreakCondition BreakBefore { get { return LineBreakCondition.BreakDesired; } } ////// Line break condition after the inline object. /// public override LineBreakCondition BreakAfter { get { return LineBreakCondition.BreakDesired; } } ////// Flag indicates whether inline object has fixed size regardless of where /// it is placed within a line. /// public override bool HasFixedSize { get { // Size of inline object is not dependent on position in the line. return true; } } ////// Text position on the inline object in the text array. /// internal int Dcp { get { return _dcp; } } ////// UIElement representing the inline object. /// internal UIElement Element { get { return _element; } } ////// Text position on the inline object in the text array. /// private readonly int _dcp; ////// Number of text position in the text array occupied by the inline object. /// private readonly int _cch; ////// UIElement representing the inline object. /// private readonly UIElement _element; ////// Text run properties for the inline object. /// private readonly TextRunProperties _textProps; ////// Text element - the host of the inline object. /// private readonly System.Windows.Controls.TextBlock _host; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: InlineObject.cs // // Description: Cached metrics of an inline objects. // // History: // 04/25/2003 : [....] - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; namespace MS.Internal.Text { ////// Inline object representation as TextRun. /// internal sealed class InlineObject: TextEmbeddedObject { ////// Constructor. /// /// Text position of the inline object in the text array. /// Number of text position in the text array occupied by the inline object. /// UIElement representing the inline object. /// Text run properties for the inline object. /// TextBlock element - the host of the inline object. internal InlineObject(int dcp, int cch, UIElement element, TextRunProperties textProps, System.Windows.Controls.TextBlock host) { _dcp = dcp; _cch = cch; _element = element; _textProps = textProps; _host = host; } ////// Get inline object's measurement metrics. /// /// Remaining paragraph width. ///Inline object metrics. public override TextEmbeddedObjectMetrics Format(double remainingParagraphWidth) { Size desiredSize = _host.MeasureChild(this); // Make sure that LS/PTS limitations are not exceeded for object's size. TextDpi.EnsureValidObjSize(ref desiredSize); double baseline = desiredSize.Height; double baselineOffsetValue = (double) Element.GetValue(TextBlock.BaselineOffsetProperty); if(!DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = baselineOffsetValue; } return new TextEmbeddedObjectMetrics(desiredSize.Width, desiredSize.Height, baseline); } ////// Get computed bounding box of the inline object. /// /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. ///Computed bounding box size of text object. public override Rect ComputeBoundingBox(bool rightToLeft, bool sideways) { if (_element.IsArrangeValid) { // Initially assume that bounding box is the same as layout box. Size size = _element.DesiredSize; double baseline = !sideways ? size.Height : size.Width; double baselineOffsetValue = (double)Element.GetValue(TextBlock.BaselineOffsetProperty); if (!sideways && !DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = (double)baselineOffsetValue; } return new Rect(0, -baseline, sideways ? size.Height : size.Width, sideways ? size.Width : size.Height); } else { return Rect.Empty; } } ////// Draw the inline object. /// /// Drawing context. /// Origin where the object is drawn. /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. public override void Draw(DrawingContext drawingContext, Point origin, bool rightToLeft, bool sideways) { // Inline object has its own visual and it is attached to a visual // tree during arrange process. // Do nothing here. } ////// Reference to character buffer. /// public override CharacterBufferReference CharacterBufferReference { get { return new CharacterBufferReference(String.Empty, 0); } } ////// Length of the inline object run. /// public override int Length { get { return _cch; } } ////// A set of properties shared by every characters in the run /// public override TextRunProperties Properties { get { return _textProps; } } ////// Line break condition before the inline object. /// public override LineBreakCondition BreakBefore { get { return LineBreakCondition.BreakDesired; } } ////// Line break condition after the inline object. /// public override LineBreakCondition BreakAfter { get { return LineBreakCondition.BreakDesired; } } ////// Flag indicates whether inline object has fixed size regardless of where /// it is placed within a line. /// public override bool HasFixedSize { get { // Size of inline object is not dependent on position in the line. return true; } } ////// Text position on the inline object in the text array. /// internal int Dcp { get { return _dcp; } } ////// UIElement representing the inline object. /// internal UIElement Element { get { return _element; } } ////// Text position on the inline object in the text array. /// private readonly int _dcp; ////// Number of text position in the text array occupied by the inline object. /// private readonly int _cch; ////// UIElement representing the inline object. /// private readonly UIElement _element; ////// Text run properties for the inline object. /// private readonly TextRunProperties _textProps; ////// Text element - the host of the inline object. /// private readonly System.Windows.Controls.TextBlock _host; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
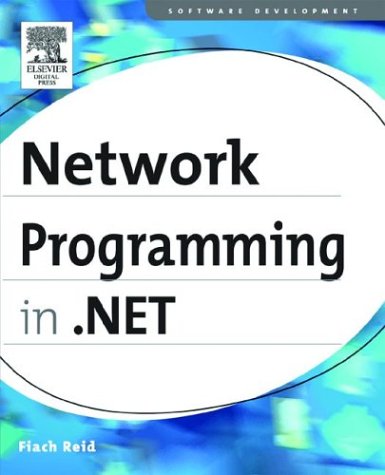
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HybridCollection.cs
- SqlTriggerAttribute.cs
- InternalBufferOverflowException.cs
- CustomValidator.cs
- MouseGestureConverter.cs
- TabPage.cs
- TraceUtility.cs
- SqlFileStream.cs
- DataGridViewElement.cs
- VectorKeyFrameCollection.cs
- Grid.cs
- BuiltInExpr.cs
- Evidence.cs
- TextSpan.cs
- BuilderPropertyEntry.cs
- PointKeyFrameCollection.cs
- TransferRequestHandler.cs
- ParsedRoute.cs
- BinaryCommonClasses.cs
- X509ScopedServiceCertificateElementCollection.cs
- Rules.cs
- TextSchema.cs
- StringOutput.cs
- CaseInsensitiveHashCodeProvider.cs
- XmlAttributeOverrides.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- Rect.cs
- FactoryGenerator.cs
- GZipDecoder.cs
- CommandLibraryHelper.cs
- DictionaryGlobals.cs
- HealthMonitoringSection.cs
- WindowsIdentity.cs
- StringDictionaryEditor.cs
- IssuedTokenClientBehaviorsElement.cs
- DropDownButton.cs
- EntityCommandCompilationException.cs
- cookie.cs
- _ConnectOverlappedAsyncResult.cs
- Vertex.cs
- EventHandlerList.cs
- GlyphTypeface.cs
- AccessibleObject.cs
- ValidatedControlConverter.cs
- WebPartExportVerb.cs
- PageVisual.cs
- PropertyStore.cs
- ComponentCollection.cs
- HijriCalendar.cs
- AllowedAudienceUriElementCollection.cs
- M3DUtil.cs
- PersonalizationAdministration.cs
- XmlHierarchyData.cs
- _NtlmClient.cs
- DataTableNewRowEvent.cs
- MsmqChannelFactory.cs
- Parsers.cs
- StrokeCollectionDefaultValueFactory.cs
- QilParameter.cs
- EnumDataContract.cs
- Vector3DCollection.cs
- _BasicClient.cs
- PackageFilter.cs
- VarInfo.cs
- ManagementOptions.cs
- JsonEnumDataContract.cs
- TimeSpanMinutesConverter.cs
- OrderByBuilder.cs
- WebResourceUtil.cs
- sortedlist.cs
- KnownIds.cs
- Geometry.cs
- ListViewGroupItemCollection.cs
- XmlDomTextWriter.cs
- CodeExpressionStatement.cs
- XmlWriterTraceListener.cs
- TcpHostedTransportConfiguration.cs
- MemberRelationshipService.cs
- BaseTemplateCodeDomTreeGenerator.cs
- DispatcherSynchronizationContext.cs
- WebPartEventArgs.cs
- AmbientProperties.cs
- WsdlHelpGeneratorElement.cs
- DesignerActionHeaderItem.cs
- WindowsAuthenticationModule.cs
- CompilationPass2Task.cs
- ImageConverter.cs
- DisplayNameAttribute.cs
- DPCustomTypeDescriptor.cs
- AccessibilityHelperForXpWin2k3.cs
- Convert.cs
- QilChoice.cs
- StringWriter.cs
- XamlRtfConverter.cs
- CryptoApi.cs
- GlyphElement.cs
- ColumnCollection.cs
- GridViewUpdatedEventArgs.cs
- DataTableReaderListener.cs
- DBParameter.cs