Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / Vertex.cs / 1 / Vertex.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
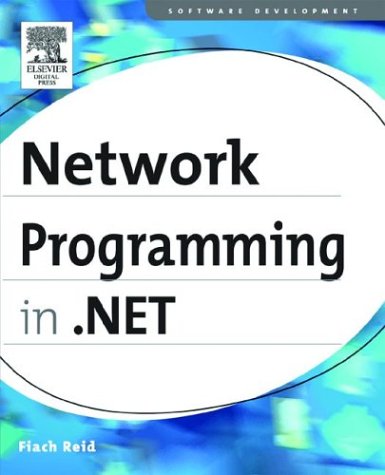
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FilterableAttribute.cs
- IpcPort.cs
- RegexStringValidator.cs
- AppDomainAttributes.cs
- AsmxEndpointPickerExtension.cs
- ClientProxyGenerator.cs
- ValueChangedEventManager.cs
- NamespaceTable.cs
- DockPattern.cs
- EntityStoreSchemaFilterEntry.cs
- FtpWebResponse.cs
- SqlHelper.cs
- Condition.cs
- HeaderUtility.cs
- WebPartConnectionsConfigureVerb.cs
- ErrorHandler.cs
- TypeElement.cs
- TableColumn.cs
- UnhandledExceptionEventArgs.cs
- SizeF.cs
- OutOfMemoryException.cs
- NumericPagerField.cs
- ListMarkerSourceInfo.cs
- DSASignatureFormatter.cs
- FocusChangedEventArgs.cs
- Transform.cs
- PersonalizationStateQuery.cs
- IndependentAnimationStorage.cs
- MemberAssignment.cs
- BooleanExpr.cs
- ButtonBase.cs
- ZoomPercentageConverter.cs
- GeneralTransform3DGroup.cs
- RuntimeResourceSet.cs
- NominalTypeEliminator.cs
- BaseTemplateBuildProvider.cs
- DocumentProperties.cs
- LinqDataSourceView.cs
- XmlWriter.cs
- CreateParams.cs
- Publisher.cs
- ScriptHandlerFactory.cs
- ValidationError.cs
- SQLResource.cs
- UdpSocketReceiveManager.cs
- PathSegmentCollection.cs
- LayoutExceptionEventArgs.cs
- TreeViewImageIndexConverter.cs
- CalendarModeChangedEventArgs.cs
- XmlSchema.cs
- MailBnfHelper.cs
- TrackingServices.cs
- DataGridViewColumnEventArgs.cs
- WindowInteropHelper.cs
- ResolvedKeyFrameEntry.cs
- ReflectionTypeLoadException.cs
- FieldDescriptor.cs
- SslStreamSecurityUpgradeProvider.cs
- ListenerSessionConnectionReader.cs
- CodeGeneratorAttribute.cs
- SerializationStore.cs
- LinearKeyFrames.cs
- ConstraintStruct.cs
- ClaimTypes.cs
- NetSectionGroup.cs
- DataGridViewTextBoxEditingControl.cs
- CfgParser.cs
- WebPartManager.cs
- ImageListUtils.cs
- NamedPipeChannelListener.cs
- EventListener.cs
- SettingsPropertyValueCollection.cs
- CompilerError.cs
- DelimitedListTraceListener.cs
- SourceInterpreter.cs
- Adorner.cs
- CursorConverter.cs
- DbgUtil.cs
- RegistrySecurity.cs
- DayRenderEvent.cs
- DesignerAutoFormatStyle.cs
- ExpressionBuilderContext.cs
- ProfileBuildProvider.cs
- OuterGlowBitmapEffect.cs
- EncodingTable.cs
- UserNameSecurityTokenParameters.cs
- ListViewGroupItemCollection.cs
- Deserializer.cs
- Parser.cs
- DBBindings.cs
- autovalidator.cs
- ToolbarAUtomationPeer.cs
- Color.cs
- RelativeSource.cs
- KeyNameIdentifierClause.cs
- CacheHelper.cs
- LinqDataSourceStatusEventArgs.cs
- MenuItem.cs
- WebControlsSection.cs
- MembershipPasswordException.cs