Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / ValidationError.cs / 1 / ValidationError.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: // An error in validation -- either created by an ValidationRule // or explicitly through MarkInvalid on BindingExpression or MultiBindingExpression. // // See specs at http://avalon/connecteddata/Specs/Validation.mht // // History: // 5/3/2004 mharper: created. // //--------------------------------------------------------------------------- using System; namespace System.Windows.Controls { ////// An error in validation -- either created by an ValidationRule /// or explicitly through MarkInvalid on BindingExpression or MultiBindingExpression. /// public class ValidationError { ////// ValidationError ctor /// /// rule that detected validation error /// BindingExpression for which validation failed /// validation rule specific details to the error /// exception that caused the validation failure; optional, can be null public ValidationError(ValidationRule ruleInError, object bindingInError, object errorContent, Exception exception) { if (ruleInError == null) throw new ArgumentNullException("ruleInError"); if (bindingInError == null) throw new ArgumentNullException("bindingInError"); _ruleInError = ruleInError; _bindingInError = bindingInError; _errorContent = errorContent; _exception = exception; } ////// ValidationError ctor /// rule that detected validation error /// BindingExpression for which validation failed /// public ValidationError(ValidationRule ruleInError, object bindingInError) : this(ruleInError, bindingInError, null, null) { } ////// If the validationError is as the result of an ValidationRule, /// then this is the reference to that ValidationRule. /// public ValidationRule RuleInError { get { return _ruleInError; } set { _ruleInError = value; } } ////// Some additional context for the ValidationError, such as /// a string describing the error. /// public object ErrorContent { get { return _errorContent; } set { _errorContent = value; } } ////// If the ValidationError is the result of some Exception, /// this will be a reference to that exception. /// public Exception Exception { get { return _exception; } set { _exception = value; } } ////// The BindingExpression or MultiBindingExpression that was marked invalid /// either explicitly, or while validating the ValidationRules collection. /// public object BindingInError { get { return _bindingInError; } } private ValidationRule _ruleInError; private object _errorContent; private Exception _exception; private object _bindingInError; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: // An error in validation -- either created by an ValidationRule // or explicitly through MarkInvalid on BindingExpression or MultiBindingExpression. // // See specs at http://avalon/connecteddata/Specs/Validation.mht // // History: // 5/3/2004 mharper: created. // //--------------------------------------------------------------------------- using System; namespace System.Windows.Controls { ////// An error in validation -- either created by an ValidationRule /// or explicitly through MarkInvalid on BindingExpression or MultiBindingExpression. /// public class ValidationError { ////// ValidationError ctor /// /// rule that detected validation error /// BindingExpression for which validation failed /// validation rule specific details to the error /// exception that caused the validation failure; optional, can be null public ValidationError(ValidationRule ruleInError, object bindingInError, object errorContent, Exception exception) { if (ruleInError == null) throw new ArgumentNullException("ruleInError"); if (bindingInError == null) throw new ArgumentNullException("bindingInError"); _ruleInError = ruleInError; _bindingInError = bindingInError; _errorContent = errorContent; _exception = exception; } ////// ValidationError ctor /// rule that detected validation error /// BindingExpression for which validation failed /// public ValidationError(ValidationRule ruleInError, object bindingInError) : this(ruleInError, bindingInError, null, null) { } ////// If the validationError is as the result of an ValidationRule, /// then this is the reference to that ValidationRule. /// public ValidationRule RuleInError { get { return _ruleInError; } set { _ruleInError = value; } } ////// Some additional context for the ValidationError, such as /// a string describing the error. /// public object ErrorContent { get { return _errorContent; } set { _errorContent = value; } } ////// If the ValidationError is the result of some Exception, /// this will be a reference to that exception. /// public Exception Exception { get { return _exception; } set { _exception = value; } } ////// The BindingExpression or MultiBindingExpression that was marked invalid /// either explicitly, or while validating the ValidationRules collection. /// public object BindingInError { get { return _bindingInError; } } private ValidationRule _ruleInError; private object _errorContent; private Exception _exception; private object _bindingInError; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
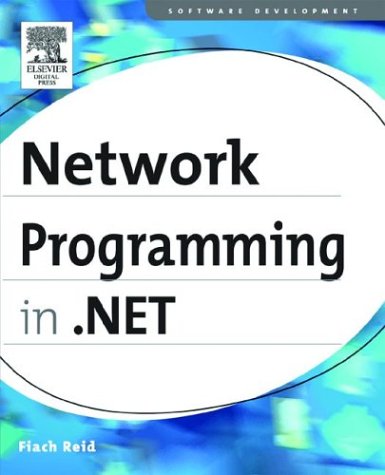
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlUtf8RawTextWriter.cs
- CqlLexer.cs
- PenLineCapValidation.cs
- Command.cs
- _LocalDataStoreMgr.cs
- TemplateBamlRecordReader.cs
- XmlSchemaRedefine.cs
- DataGridHeaderBorder.cs
- PingReply.cs
- TimeZone.cs
- FullTextBreakpoint.cs
- MemberCollection.cs
- WindowsListViewSubItem.cs
- WebBrowserDocumentCompletedEventHandler.cs
- DataSvcMapFileSerializer.cs
- DecimalStorage.cs
- BindingBase.cs
- StyleReferenceConverter.cs
- ConsoleCancelEventArgs.cs
- RayMeshGeometry3DHitTestResult.cs
- TextRangeAdaptor.cs
- CombinedGeometry.cs
- WebResourceAttribute.cs
- OraclePermission.cs
- PropertyFilter.cs
- NotifyIcon.cs
- ProviderSettings.cs
- BamlLocalizationDictionary.cs
- GlyphRunDrawing.cs
- HtmlToClrEventProxy.cs
- EventLogPermissionAttribute.cs
- HttpCapabilitiesEvaluator.cs
- NetworkStream.cs
- DocumentReferenceCollection.cs
- SqlOuterApplyReducer.cs
- RootAction.cs
- GridViewRowCollection.cs
- _SslStream.cs
- RelationshipEnd.cs
- IndexedEnumerable.cs
- EntityDataSourceChangingEventArgs.cs
- WebBaseEventKeyComparer.cs
- NotSupportedException.cs
- ComponentCache.cs
- HiddenField.cs
- InternalsVisibleToAttribute.cs
- FileVersion.cs
- SoapReflectionImporter.cs
- ValidationEventArgs.cs
- RegexMatchCollection.cs
- XmlEncodedRawTextWriter.cs
- ComboBoxItem.cs
- GridViewAutomationPeer.cs
- WindowsAuthenticationModule.cs
- ColorMap.cs
- SystemBrushes.cs
- BooleanStorage.cs
- DockEditor.cs
- DropDownHolder.cs
- NestPullup.cs
- StructuralComparisons.cs
- DbConnectionPoolGroupProviderInfo.cs
- UIElement3D.cs
- HtmlInputHidden.cs
- EntityDataSourceWizardForm.cs
- QuaternionIndependentAnimationStorage.cs
- BamlTreeUpdater.cs
- SqlDataSourceAdvancedOptionsForm.cs
- CounterCreationDataCollection.cs
- ThreadSafeList.cs
- PreviewPageInfo.cs
- Bidi.cs
- ActivityDesigner.cs
- ContractMapping.cs
- CallSiteHelpers.cs
- XamlParser.cs
- ScaleTransform.cs
- ControlDesigner.cs
- DataGridViewCellStyleConverter.cs
- WebPartDeleteVerb.cs
- ZipIOFileItemStream.cs
- MinimizableAttributeTypeConverter.cs
- NumberFormatInfo.cs
- TimerEventSubscription.cs
- Label.cs
- validation.cs
- LineServicesCallbacks.cs
- InvariantComparer.cs
- _ProxyChain.cs
- GPPOINT.cs
- ObjectSet.cs
- SimpleLine.cs
- HwndSourceKeyboardInputSite.cs
- ComponentDesigner.cs
- MarkupExtensionParser.cs
- ApplicationSecurityInfo.cs
- Effect.cs
- CollectionView.cs
- NotifyIcon.cs
- ArrayList.cs