Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Regex / System / Text / RegularExpressions / RegexMatchCollection.cs / 1 / RegexMatchCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // The MatchCollection lists the successful matches that // result when searching a string for a regular expression. namespace System.Text.RegularExpressions { using System.Collections; /* * This collection returns a sequence of successful match results, either * from GetMatchCollection() or GetExecuteCollection(). It stops when the * first failure is encountered (it does not return the failed match). */ ////// [ Serializable() ] public class MatchCollection : ICollection { internal Regex _regex; internal ArrayList _matches; internal bool _done; internal String _input; internal int _beginning; internal int _length; internal int _startat; internal int _prevlen; private static int infinite = 0x7FFFFFFF; /* * Nonpublic constructor */ internal MatchCollection(Regex regex, String input, int beginning, int length, int startat) { if (startat < 0 || startat > input.Length) throw new ArgumentOutOfRangeException("startat", SR.GetString(SR.BeginIndexNotNegative)); _regex = regex; _input = input; _beginning = beginning; _length = length; _startat = startat; _prevlen = -1; _matches = new ArrayList(); _done = false; } internal Match GetMatch(int i) { if (i < 0) return null; if (_matches.Count > i) return(Match)_matches[i]; if (_done) return null; Match match; do { match = _regex.Run(false, _prevlen, _input, _beginning, _length, _startat); if (!match.Success) { _done = true; return null; } _matches.Add(match); _prevlen = match._length; _startat = match._textpos; } while (_matches.Count <= i); return match; } ////// Represents the set of names appearing as capturing group /// names in a regular expression. /// ////// public int Count { get { if (_done) return _matches.Count; GetMatch(infinite); return _matches.Count; } } ////// Returns the number of captures. /// ////// public Object SyncRoot { get { return this; } } ///[To be supplied.] ////// public bool IsSynchronized { get { return false; } } ///[To be supplied.] ////// public bool IsReadOnly { get { return true; } } ///[To be supplied.] ////// public virtual Match this[int i] { get { Match match; match = GetMatch(i); if (match == null) throw new ArgumentOutOfRangeException("i"); return match; } } ////// Returns the ith Match in the collection. /// ////// public void CopyTo(Array array, int arrayIndex) { // property access to force computation of whole array int count = Count; _matches.CopyTo(array, arrayIndex); } ////// Copies all the elements of the collection to the given array /// starting at the given index. /// ////// public IEnumerator GetEnumerator() { return new MatchEnumerator(this); } } /* * This non-public enumerator lists all the group matches. * Should it be public? */ [ Serializable() ] internal class MatchEnumerator : IEnumerator { internal MatchCollection _matchcoll; internal Match _match = null; internal int _curindex; internal bool _done; /* * Nonpublic constructor */ internal MatchEnumerator(MatchCollection matchcoll) { _matchcoll = matchcoll; } /* * Advance to the next match */ public bool MoveNext() { if (_done) return false; _match = _matchcoll.GetMatch(_curindex++); if (_match == null) { _done = true; return false; } return true; } /* * The current match */ public Object Current { get { if (_match == null) throw new InvalidOperationException(SR.GetString(SR.EnumNotStarted)); return _match; } } /* * Position before the first item */ public void Reset() { _curindex = 0; _done = false; _match = null; } } }/// Provides an enumerator in the same order as Item[i]. /// ///
Link Menu
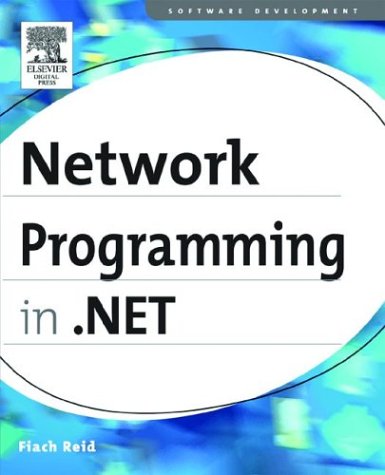
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadAttributes.cs
- TabletDeviceInfo.cs
- ScrollProviderWrapper.cs
- HandleRef.cs
- XamlContextStack.cs
- SqlClientPermission.cs
- TablePattern.cs
- Control.cs
- SelectedDatesCollection.cs
- RoutedEventValueSerializer.cs
- PropertySet.cs
- TextFormatter.cs
- FolderBrowserDialogDesigner.cs
- GridSplitter.cs
- InArgument.cs
- unitconverter.cs
- DataListItemEventArgs.cs
- TraceSwitch.cs
- DNS.cs
- SafeCryptoHandles.cs
- AssociationTypeEmitter.cs
- DPTypeDescriptorContext.cs
- ConnectionManagementElement.cs
- HostedTcpTransportManager.cs
- MemberMaps.cs
- ResourcePart.cs
- RoleService.cs
- GridViewPageEventArgs.cs
- WithParamAction.cs
- WindowPattern.cs
- CodeSubDirectoriesCollection.cs
- HierarchicalDataSourceIDConverter.cs
- StrongName.cs
- UInt16Storage.cs
- SQLByteStorage.cs
- CultureInfo.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- DrawingAttributes.cs
- ListControlBoundActionList.cs
- DataGridCellInfo.cs
- PointHitTestResult.cs
- QuotedPairReader.cs
- ErrorWebPart.cs
- NetworkInterface.cs
- IImplicitResourceProvider.cs
- EFDataModelProvider.cs
- CollectionView.cs
- JsonReader.cs
- Utilities.cs
- ListViewInsertionMark.cs
- XmlName.cs
- ComplexLine.cs
- StrokeCollectionConverter.cs
- WhitespaceRuleReader.cs
- Message.cs
- CrossContextChannel.cs
- FolderNameEditor.cs
- TargetConverter.cs
- ConnectionPointConverter.cs
- SqlColumnizer.cs
- QueryContinueDragEvent.cs
- ObjectListField.cs
- HMACSHA384.cs
- UnicodeEncoding.cs
- ACE.cs
- RuntimeIdentifierPropertyAttribute.cs
- TranslateTransform3D.cs
- SqlDependencyUtils.cs
- WebPermission.cs
- ContainerUtilities.cs
- CodeDelegateCreateExpression.cs
- CompiledAction.cs
- PenThreadWorker.cs
- FrameworkTextComposition.cs
- SignatureDescription.cs
- NotificationContext.cs
- BrushConverter.cs
- StaticSiteMapProvider.cs
- CssTextWriter.cs
- Base64Decoder.cs
- TemplatedAdorner.cs
- ValidateNames.cs
- RectangleGeometry.cs
- ChannelManagerBase.cs
- GenericIdentity.cs
- FilterQuery.cs
- SqlComparer.cs
- RemoveStoryboard.cs
- AttributeAction.cs
- ECDiffieHellmanCng.cs
- RegisteredHiddenField.cs
- GenericParameterDataContract.cs
- CategoryAttribute.cs
- XmlSchemaProviderAttribute.cs
- SerializationBinder.cs
- DrawListViewSubItemEventArgs.cs
- GridToolTip.cs
- ConstraintConverter.cs
- ToolStripPanel.cs
- _DisconnectOverlappedAsyncResult.cs