Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebControls / CustomValidator.cs / 1 / CustomValidator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System.Web; using System.Security.Permissions; using System.Web.Util; ////// [ DefaultEvent("ServerValidate"), ToolboxData("<{0}:CustomValidator runat=\"server\" ErrorMessage=\"CustomValidator\">{0}:CustomValidator>") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CustomValidator : BaseValidator { private static readonly object EventServerValidate= new object(); ///Allows custom code to perform /// validation on the client and/or server. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.CustomValidator_ClientValidationFunction) ] public string ClientValidationFunction { get { object o = ViewState["ClientValidationFunction"]; return((o == null) ? String.Empty : (string)o); } set { ViewState["ClientValidationFunction"] = value; } } [ WebCategory("Behavior"), Themeable(false), DefaultValue(false), WebSysDescription(SR.CustomValidator_ValidateEmptyText), ] public bool ValidateEmptyText { get { object o = ViewState["ValidateEmptyText"]; return((o == null) ? false : (bool)o); } set { ViewState["ValidateEmptyText"] = value; } } ///Gets and sets the custom client Javascript function used /// for validation. ////// [ WebSysDescription(SR.CustomValidator_ServerValidate) ] public event ServerValidateEventHandler ServerValidate { add { Events.AddHandler(EventServerValidate, value); } remove { Events.RemoveHandler(EventServerValidate, value); } } ///Represents the method that will handle the /// ///event of a /// . /// /// protected override void AddAttributesToRender(HtmlTextWriter writer) { base.AddAttributesToRender(writer); if (RenderUplevel) { string id = ClientID; HtmlTextWriter expandoAttributeWriter = (EnableLegacyRendering) ? writer : null; AddExpandoAttribute(expandoAttributeWriter, id, "evaluationfunction", "CustomValidatorEvaluateIsValid", false); if (ClientValidationFunction.Length > 0) { AddExpandoAttribute(expandoAttributeWriter, id, "clientvalidationfunction", ClientValidationFunction); if (ValidateEmptyText) { AddExpandoAttribute(expandoAttributeWriter, id, "validateemptytext", "true", false); } } } } ///Adds the properties of the ///control to the /// output stream for rendering on the client. /// /// protected override bool ControlPropertiesValid() { // Need to override the BaseValidator implementation, because for CustomValidator, it is fine // for the ControlToValidate to be blank. string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { // Check that the property points to a valid control. Will throw and exception if not found CheckControlValidationProperty(controlToValidate, "ControlToValidate"); } return true; } ///Checks the properties of the control for valid values. ////// /// EvaluateIsValid method /// protected override bool EvaluateIsValid() { // If no control is specified, we always fire the event. If they have specified a control, we // only fire the event if the input is non-blank. string controlValue = String.Empty; string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { controlValue = GetControlValidationValue(controlToValidate); Debug.Assert(controlValue != null, "Should have been caught be property check"); // If the text is empty, we return true. Whitespace is ignored for coordination wiht // RequiredFieldValidator. if ((controlValue == null || controlValue.Trim().Length == 0) && !ValidateEmptyText) { return true; } } return OnServerValidate(controlValue); } ////// protected virtual bool OnServerValidate(string value) { ServerValidateEventHandler handler = (ServerValidateEventHandler)Events[EventServerValidate]; ServerValidateEventArgs args = new ServerValidateEventArgs(value, true); if (handler != null) { handler(this, args); return args.IsValid; } else { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Raises the /// ///event for the . // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System.Web; using System.Security.Permissions; using System.Web.Util; ////// [ DefaultEvent("ServerValidate"), ToolboxData("<{0}:CustomValidator runat=\"server\" ErrorMessage=\"CustomValidator\">{0}:CustomValidator>") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CustomValidator : BaseValidator { private static readonly object EventServerValidate= new object(); ///Allows custom code to perform /// validation on the client and/or server. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.CustomValidator_ClientValidationFunction) ] public string ClientValidationFunction { get { object o = ViewState["ClientValidationFunction"]; return((o == null) ? String.Empty : (string)o); } set { ViewState["ClientValidationFunction"] = value; } } [ WebCategory("Behavior"), Themeable(false), DefaultValue(false), WebSysDescription(SR.CustomValidator_ValidateEmptyText), ] public bool ValidateEmptyText { get { object o = ViewState["ValidateEmptyText"]; return((o == null) ? false : (bool)o); } set { ViewState["ValidateEmptyText"] = value; } } ///Gets and sets the custom client Javascript function used /// for validation. ////// [ WebSysDescription(SR.CustomValidator_ServerValidate) ] public event ServerValidateEventHandler ServerValidate { add { Events.AddHandler(EventServerValidate, value); } remove { Events.RemoveHandler(EventServerValidate, value); } } ///Represents the method that will handle the /// ///event of a /// . /// /// protected override void AddAttributesToRender(HtmlTextWriter writer) { base.AddAttributesToRender(writer); if (RenderUplevel) { string id = ClientID; HtmlTextWriter expandoAttributeWriter = (EnableLegacyRendering) ? writer : null; AddExpandoAttribute(expandoAttributeWriter, id, "evaluationfunction", "CustomValidatorEvaluateIsValid", false); if (ClientValidationFunction.Length > 0) { AddExpandoAttribute(expandoAttributeWriter, id, "clientvalidationfunction", ClientValidationFunction); if (ValidateEmptyText) { AddExpandoAttribute(expandoAttributeWriter, id, "validateemptytext", "true", false); } } } } ///Adds the properties of the ///control to the /// output stream for rendering on the client. /// /// protected override bool ControlPropertiesValid() { // Need to override the BaseValidator implementation, because for CustomValidator, it is fine // for the ControlToValidate to be blank. string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { // Check that the property points to a valid control. Will throw and exception if not found CheckControlValidationProperty(controlToValidate, "ControlToValidate"); } return true; } ///Checks the properties of the control for valid values. ////// /// EvaluateIsValid method /// protected override bool EvaluateIsValid() { // If no control is specified, we always fire the event. If they have specified a control, we // only fire the event if the input is non-blank. string controlValue = String.Empty; string controlToValidate = ControlToValidate; if (controlToValidate.Length > 0) { controlValue = GetControlValidationValue(controlToValidate); Debug.Assert(controlValue != null, "Should have been caught be property check"); // If the text is empty, we return true. Whitespace is ignored for coordination wiht // RequiredFieldValidator. if ((controlValue == null || controlValue.Trim().Length == 0) && !ValidateEmptyText) { return true; } } return OnServerValidate(controlValue); } ////// protected virtual bool OnServerValidate(string value) { ServerValidateEventHandler handler = (ServerValidateEventHandler)Events[EventServerValidate]; ServerValidateEventArgs args = new ServerValidateEventArgs(value, true); if (handler != null) { handler(this, args); return args.IsValid; } else { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Raises the /// ///event for the .
Link Menu
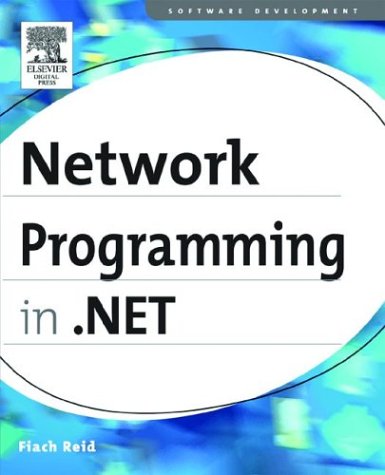
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecurityTokenSerializer.cs
- IPGlobalProperties.cs
- dsa.cs
- XamlTreeBuilder.cs
- HandlerFactoryCache.cs
- CharConverter.cs
- DataReceivedEventArgs.cs
- ServiceAuthorizationBehavior.cs
- PriorityBindingExpression.cs
- DataControlButton.cs
- CounterSample.cs
- WebPartAuthorizationEventArgs.cs
- XmlSubtreeReader.cs
- httpapplicationstate.cs
- AvTraceFormat.cs
- processwaithandle.cs
- DynamicResourceExtension.cs
- ListenDesigner.cs
- MessageEncoder.cs
- EventItfInfo.cs
- DynamicILGenerator.cs
- DesignTimeSiteMapProvider.cs
- ConfigurationStrings.cs
- SqlCacheDependencyDatabaseCollection.cs
- OleDbErrorCollection.cs
- HttpBrowserCapabilitiesWrapper.cs
- ReceiveMessageContent.cs
- XamlClipboardData.cs
- AggregationMinMaxHelpers.cs
- InternalPolicyElement.cs
- BooleanToVisibilityConverter.cs
- SimpleBitVector32.cs
- BoundPropertyEntry.cs
- FreeFormDesigner.cs
- GridViewRowEventArgs.cs
- errorpatternmatcher.cs
- ParameterBuilder.cs
- Transform3DGroup.cs
- MemberDescriptor.cs
- AvtEvent.cs
- CallbackTimeoutsBehavior.cs
- ImportCatalogPart.cs
- ResXDataNode.cs
- DiscreteKeyFrames.cs
- FormParameter.cs
- RelatedImageListAttribute.cs
- DBNull.cs
- MobileUITypeEditor.cs
- OracleBinary.cs
- NativeMethods.cs
- TrustLevel.cs
- SoapFault.cs
- NetworkStream.cs
- HttpPostedFileBase.cs
- tooltip.cs
- TypeDescriptor.cs
- FixedSchema.cs
- OutOfProcStateClientManager.cs
- DataGridViewCellPaintingEventArgs.cs
- CqlQuery.cs
- ContentElementCollection.cs
- DataGridAddNewRow.cs
- Attributes.cs
- SessionState.cs
- Comparer.cs
- PerformanceCounterManager.cs
- TransformFinalBlockRequest.cs
- EventManager.cs
- InspectionWorker.cs
- SecurityContext.cs
- DiscardableAttribute.cs
- CreateSequenceResponse.cs
- LeafCellTreeNode.cs
- ReflectEventDescriptor.cs
- LocatorBase.cs
- TextSearch.cs
- TextEffect.cs
- XmlSchemaExternal.cs
- GridItemPattern.cs
- MergeFilterQuery.cs
- Aggregates.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- BaseCollection.cs
- FullTrustAssembliesSection.cs
- Buffer.cs
- TextElementCollectionHelper.cs
- Error.cs
- Subtree.cs
- ToolStripTextBox.cs
- TextContainerChangedEventArgs.cs
- LocalizeDesigner.cs
- ConnectionPoolManager.cs
- GB18030Encoding.cs
- sqlnorm.cs
- SecurityManager.cs
- _AutoWebProxyScriptWrapper.cs
- GenericAuthenticationEventArgs.cs
- Popup.cs
- DebugInfoExpression.cs
- ValidateNames.cs