Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Annotations / LocatorBase.cs / 1 / LocatorBase.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // ContentLocatorBase represents an object that identifies a piece of data. It // can be an ordered list of ContentLocatorParts (in which case its a // ContentLocator) or it can be a set of Locators (in which case its a // ContentLocatorGroup). // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 10/04/2002: rruiz: Added header comment to ObjectModel.cs // 07/03/2003: magedz: Renamed Link, LinkSequence to LocatorPart and Locator // respectively. // 05/31/2003: LGolding: Ported to WCP tree. // 07/15/2003: rruiz: Rewrote implementations to extend abstract classes // instead of implement interfaces; got rid of obsolete // classes; put each class in separate file. // 12/09/2003: ssimova: Added Id property // 12/03/2003: ssimova: Changed LocatorParts to Parts // 06/20/2004: rruiz: Stripped out most of the API and made this an abstract // class which supports notifying its owner of changes. // New concrete classes were introduced for sets and // sequences. //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Xml; using MS.Internal.Annotations; namespace System.Windows.Annotations { ////// ContentLocatorBase represents an object that identifies a piece of data. /// It can be an ordered list of ContentLocatorParts (in which case its a /// ContentLocator) or it can be a set of Locators (in which case its a ContentLocatorGroup). /// public abstract class ContentLocatorBase : INotifyPropertyChanged2, IOwnedObject { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Internal constructor. This makes the abstract class /// unsubclassable by third-parties, as desired. /// internal ContentLocatorBase() { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Create a deep clone of this ContentLocatorBase. /// ///a deep clone of this ContentLocatorBase; never returns null public abstract object Clone(); #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- //----------------------------------------------------- // // Internal Events // //------------------------------------------------------ #region Public Events ////// /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add{ _propertyChanged += value; } remove{ _propertyChanged -= value; } } #endregion Public Events //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Notify the owner this ContentLocatorBase has changed. /// This method should be protected so only subclasses /// could call it but that would expose it in the /// public API space so we keep it internal. /// internal void FireLocatorChanged(string name) { if (_propertyChanged != null) { _propertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs(name)); } } ////// bool IOwnedObject.Owned { get { return _owned; } set { _owned = value; } } ////// Internal Merge method used by the LocatorManager as it builds up /// Locators. We don't expose these methods publicly because they /// are of little use and are optimized for use by the LM (e.g., we /// know the arguments aren't owned by anyone and can be modified in /// place). /// /// the ContentLocatorBase to merge ///the resulting ContentLocatorBase (may be the same object the method /// was called on for perf reasons) internal abstract ContentLocatorBase Merge(ContentLocatorBase other); #endregion Internal Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields ////// private bool _owned; ////// /// private event PropertyChangedEventHandler _propertyChanged; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // ContentLocatorBase represents an object that identifies a piece of data. It // can be an ordered list of ContentLocatorParts (in which case its a // ContentLocator) or it can be a set of Locators (in which case its a // ContentLocatorGroup). // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 10/04/2002: rruiz: Added header comment to ObjectModel.cs // 07/03/2003: magedz: Renamed Link, LinkSequence to LocatorPart and Locator // respectively. // 05/31/2003: LGolding: Ported to WCP tree. // 07/15/2003: rruiz: Rewrote implementations to extend abstract classes // instead of implement interfaces; got rid of obsolete // classes; put each class in separate file. // 12/09/2003: ssimova: Added Id property // 12/03/2003: ssimova: Changed LocatorParts to Parts // 06/20/2004: rruiz: Stripped out most of the API and made this an abstract // class which supports notifying its owner of changes. // New concrete classes were introduced for sets and // sequences. //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Xml; using MS.Internal.Annotations; namespace System.Windows.Annotations { ////// ContentLocatorBase represents an object that identifies a piece of data. /// It can be an ordered list of ContentLocatorParts (in which case its a /// ContentLocator) or it can be a set of Locators (in which case its a ContentLocatorGroup). /// public abstract class ContentLocatorBase : INotifyPropertyChanged2, IOwnedObject { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Internal constructor. This makes the abstract class /// unsubclassable by third-parties, as desired. /// internal ContentLocatorBase() { } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Create a deep clone of this ContentLocatorBase. /// ///a deep clone of this ContentLocatorBase; never returns null public abstract object Clone(); #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- //----------------------------------------------------- // // Internal Events // //------------------------------------------------------ #region Public Events ////// /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add{ _propertyChanged += value; } remove{ _propertyChanged -= value; } } #endregion Public Events //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Notify the owner this ContentLocatorBase has changed. /// This method should be protected so only subclasses /// could call it but that would expose it in the /// public API space so we keep it internal. /// internal void FireLocatorChanged(string name) { if (_propertyChanged != null) { _propertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs(name)); } } ////// bool IOwnedObject.Owned { get { return _owned; } set { _owned = value; } } ////// Internal Merge method used by the LocatorManager as it builds up /// Locators. We don't expose these methods publicly because they /// are of little use and are optimized for use by the LM (e.g., we /// know the arguments aren't owned by anyone and can be modified in /// place). /// /// the ContentLocatorBase to merge ///the resulting ContentLocatorBase (may be the same object the method /// was called on for perf reasons) internal abstract ContentLocatorBase Merge(ContentLocatorBase other); #endregion Internal Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields ////// private bool _owned; ////// /// private event PropertyChangedEventHandler _propertyChanged; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
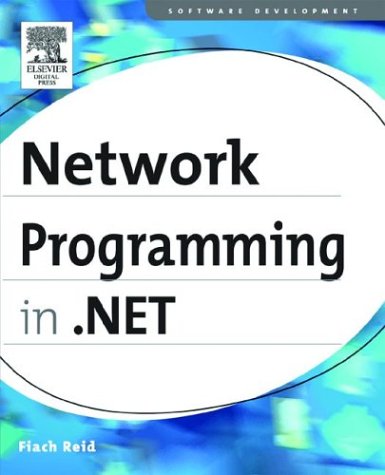
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TraceContextEventArgs.cs
- IPPacketInformation.cs
- DispatcherHookEventArgs.cs
- ApplyTemplatesAction.cs
- SaveWorkflowCommand.cs
- EntityTransaction.cs
- LinkUtilities.cs
- SoapServerProtocol.cs
- SourceLineInfo.cs
- SessionPageStatePersister.cs
- XmlDownloadManager.cs
- SafeLibraryHandle.cs
- ConvertersCollection.cs
- SafeNativeMethods.cs
- TypeDescriptionProviderAttribute.cs
- MenuItem.cs
- TdsParserHelperClasses.cs
- TextAdaptor.cs
- CallbackValidator.cs
- ProxyManager.cs
- _DigestClient.cs
- XmlSchemaException.cs
- MimeMultiPart.cs
- HttpFileCollection.cs
- TraceUtils.cs
- safemediahandle.cs
- DockAndAnchorLayout.cs
- DataViewManagerListItemTypeDescriptor.cs
- WindowsRichEdit.cs
- DispatcherFrame.cs
- DynamicObjectAccessor.cs
- LoginUtil.cs
- SetterBase.cs
- Trigger.cs
- WinFormsUtils.cs
- __Filters.cs
- Stylus.cs
- ExpressionPrefixAttribute.cs
- OperationFormatStyle.cs
- InputProcessorProfilesLoader.cs
- NamedPermissionSet.cs
- TdsValueSetter.cs
- StringComparer.cs
- SAPICategories.cs
- InputScopeAttribute.cs
- SqlAliaser.cs
- IFlowDocumentViewer.cs
- TreeViewHitTestInfo.cs
- CommonXSendMessage.cs
- SocketInformation.cs
- DataRowComparer.cs
- ColorBlend.cs
- NavigationWindow.cs
- StreamingContext.cs
- TextSelectionProcessor.cs
- DecimalFormatter.cs
- ConfigurationLocationCollection.cs
- MediaTimeline.cs
- DocumentViewerAutomationPeer.cs
- HtmlUtf8RawTextWriter.cs
- BitmapEffectGeneralTransform.cs
- ListCollectionView.cs
- RepeatButtonAutomationPeer.cs
- ServicePointManagerElement.cs
- CompositeFontFamily.cs
- Evidence.cs
- PrivacyNoticeElement.cs
- AsymmetricKeyExchangeDeformatter.cs
- ClrProviderManifest.cs
- Pair.cs
- MediaElementAutomationPeer.cs
- FunctionParameter.cs
- VectorValueSerializer.cs
- ChangeToolStripParentVerb.cs
- DateTime.cs
- CompleteWizardStep.cs
- PropertyItem.cs
- SystemGatewayIPAddressInformation.cs
- XmlSchemaAnnotation.cs
- SecurityRuntime.cs
- Query.cs
- MenuItem.cs
- SurrogateSelector.cs
- EnumerableRowCollectionExtensions.cs
- VSWCFServiceContractGenerator.cs
- ObjectDataSourceChooseTypePanel.cs
- IBuiltInEvidence.cs
- XmlEventCache.cs
- DataViewSettingCollection.cs
- DropShadowEffect.cs
- RectAnimation.cs
- StreamGeometry.cs
- DbConnectionPoolCounters.cs
- ReceiveCompletedEventArgs.cs
- ModuleBuilderData.cs
- SigningProgress.cs
- SQLDateTime.cs
- SqlReorderer.cs
- ToolStripDropDownButton.cs
- XmlSchemaException.cs