Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / SourceLineInfo.cs / 1305376 / SourceLineInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Diagnostics; namespace System.Xml.Xsl { [DebuggerDisplay("({Line},{Pos})")] internal struct Location { private ulong value; public int Line { get { return (int)(this.value >> 32); } } public int Pos { get { return (int)(this.value ); } } public Location(int line, int pos) { this.value = (((ulong)line) << 32) | (uint)pos; } public Location(Location that) { this.value = that.value; } public bool LessOrEqual(Location that) { return this.value <= that.value; } } [DebuggerDisplay("{Uri} [{StartLine},{StartPos} -- {EndLine},{EndPos}]")] internal class SourceLineInfo : ISourceLineInfo { protected string uriString; protected Location start; protected Location end; public SourceLineInfo(string uriString, int startLine, int startPos, int endLine, int endPos) : this(uriString, new Location(startLine, startPos), new Location(endLine, endPos)) {} public SourceLineInfo(string uriString, Location start, Location end) { this.uriString = uriString; this.start = start; this.end = end; Validate(this); } public string Uri { get { return this.uriString ; } } public int StartLine { get { return this.start.Line; } } public int StartPos { get { return this.start.Pos ; } } public int EndLine { get { return this.end.Line ; } } public int EndPos { get { return this.end.Pos ; } } public Location End { get { return this.end ; } } public Location Start { get { return this.start ; } } ////// Magic number 0xfeefee is used in PDB to denote a section of IL that does not map to any user code. /// When VS debugger steps into IL marked with 0xfeefee, it will continue the step until it reaches /// some user code. /// protected const int NoSourceMagicNumber = 0xfeefee; public static SourceLineInfo NoSource = new SourceLineInfo(string.Empty, NoSourceMagicNumber, 0, NoSourceMagicNumber, 0); public bool IsNoSource { get { return this.StartLine == NoSourceMagicNumber; } } [Conditional("DEBUG")] public static void Validate(ISourceLineInfo lineInfo) { if (lineInfo.Start.Line == 0 || lineInfo.Start.Line == NoSourceMagicNumber) { Debug.Assert(lineInfo.Start.Line == lineInfo.End.Line); Debug.Assert(lineInfo.Start.Pos == 0 && lineInfo.End.Pos == 0); } else { Debug.Assert(0 < lineInfo.Start.Line && 0 < lineInfo.Start.Pos, "0 < start"); Debug.Assert(0 < lineInfo.End.Line && 0 < lineInfo.End.Pos , "0 < end"); Debug.Assert(lineInfo.Start.LessOrEqual(lineInfo.End), "start <= end"); } } // Returns file path for local and network URIs. Used for PDB generating and error reporting. public static string GetFileName(string uriString) { Debug.Assert(uriString != null); Uri uri; if (uriString.Length != 0 && System.Uri.TryCreate(uriString, UriKind.Absolute, out uri) && uri.IsFile ) { return uri.LocalPath; } return uriString; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
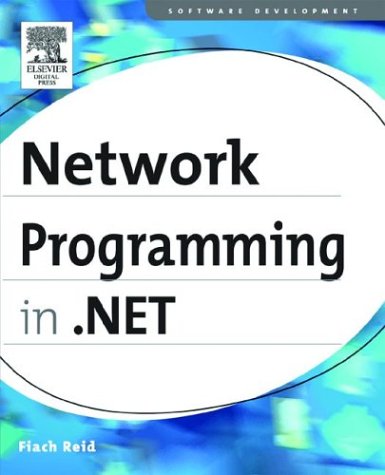
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BooleanAnimationUsingKeyFrames.cs
- VersionPair.cs
- DataServiceHostWrapper.cs
- MenuItemBinding.cs
- IPAddressCollection.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- TableProviderWrapper.cs
- FileSystemWatcher.cs
- FloatAverageAggregationOperator.cs
- ResourceKey.cs
- FormClosingEvent.cs
- Function.cs
- ChannelTerminatedException.cs
- ManipulationDevice.cs
- ByteStreamGeometryContext.cs
- SecurityUniqueId.cs
- SizeLimitedCache.cs
- DiagnosticTrace.cs
- ToolStripOverflowButton.cs
- SqlBinder.cs
- RuntimeIdentifierPropertyAttribute.cs
- WebBrowserContainer.cs
- DataGridViewColumnConverter.cs
- DataGridViewColumnCollection.cs
- AutomationPropertyInfo.cs
- NetTcpSection.cs
- ArrangedElementCollection.cs
- TcpAppDomainProtocolHandler.cs
- DataBoundControl.cs
- EditorBrowsableAttribute.cs
- MergePropertyDescriptor.cs
- HttpTransportElement.cs
- HyperlinkAutomationPeer.cs
- Highlights.cs
- TokenFactoryFactory.cs
- FilterUserControlBase.cs
- assemblycache.cs
- MailAddress.cs
- AuthenticatedStream.cs
- Line.cs
- PageCatalogPart.cs
- AbstractDataSvcMapFileLoader.cs
- SmtpFailedRecipientException.cs
- ObjectStateManagerMetadata.cs
- ListViewContainer.cs
- Panel.cs
- CodeTypeReferenceCollection.cs
- TextSelectionHelper.cs
- ScriptManager.cs
- ParseElementCollection.cs
- WinEventWrap.cs
- TreeViewImageGenerator.cs
- AddInBase.cs
- StructuralComparisons.cs
- Triplet.cs
- BamlRecordReader.cs
- DataGridViewRowStateChangedEventArgs.cs
- ListDictionaryInternal.cs
- PersonalizationProviderCollection.cs
- FactoryRecord.cs
- FilterEventArgs.cs
- Camera.cs
- infer.cs
- LayoutExceptionEventArgs.cs
- EventSourceCreationData.cs
- ConnectionStringsSection.cs
- FlowLayoutPanel.cs
- DeobfuscatingStream.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- Parameter.cs
- DefaultSection.cs
- ProcessModuleCollection.cs
- EmptyQuery.cs
- TargetControlTypeCache.cs
- PriorityChain.cs
- CodeCompileUnit.cs
- ListViewItemEventArgs.cs
- FactoryGenerator.cs
- FormViewUpdatedEventArgs.cs
- Substitution.cs
- ToolStripHighContrastRenderer.cs
- SqlMultiplexer.cs
- DataFormats.cs
- CodeParameterDeclarationExpressionCollection.cs
- StreamProxy.cs
- VersionUtil.cs
- CodeThrowExceptionStatement.cs
- SchemaCollectionCompiler.cs
- VScrollBar.cs
- ReferentialConstraint.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- TextFormatterImp.cs
- CompiledQueryCacheKey.cs
- EventLogPermissionEntry.cs
- Privilege.cs
- MatrixValueSerializer.cs
- UpdateException.cs
- UnknownMessageReceivedEventArgs.cs
- TextLineResult.cs
- SchemaDeclBase.cs