Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / ExportFileRequest.cs / 1 / ExportFileRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.IO; using System.Runtime.InteropServices; using System.Collections.Generic; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Security.Principal; using System.Xml; using System.Security.Cryptography.Xml; using System.Security; using System.Security.AccessControl; using System.Security.Cryptography; using System.Text; using System.Xml.Schema; // // Export cards to a file protected by a passphrase // class ExportFileRequest : UIAgentRequest { string m_filename; string m_passphrase; string[ ] m_cardIds; public ExportFileRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } // // Summary // Read the arguments // protected override void OnMarshalInArgs() { BinaryReader reader = new InfoCardBinaryReader( InArgs, System.Text.Encoding.Unicode ); m_filename = Utility.DeserializeString( reader ); m_passphrase = Utility.DeserializeString( reader ); UInt32 count = reader.ReadUInt32(); m_cardIds = new string[ count ]; for( UInt32 i = 0; i < count; i++ ) { m_cardIds[ i ] = Utility.DeserializeString( reader ); } } // // Summary // Selectively export the cards to the file and protect is with a passphrase // protected override void OnProcess() { IDT.Assert( !String.IsNullOrEmpty( m_filename ), "No file name was specified" ); IDT.Assert( !String.IsNullOrEmpty( m_passphrase ), " No passphrase specified for the file" ); StoreConnection connection = StoreConnection.GetConnection(); try { // // Start exporting the card to an xml format // RoamingStoreFile storeFile = new RoamingStoreFile(); try { for( int i = 0; i < m_cardIds.Length; i++ ) { IDT.TraceDebug( "Exporting card {0}", m_cardIds[ i ] ); InfoCard card = new InfoCard( new Uri( m_cardIds[ i ] ) ); card.Get( connection ); card.GetMasterKey( connection ); storeFile.Cards.Add( card ); } ; using( FileStream exportfile = new FileStream( m_filename, FileMode.Create ) ) { XmlWriterSettings settings = new XmlWriterSettings(); settings.CloseOutput = false; using( XmlWriter writer = XmlWriter.Create( exportfile, settings ) ) { storeFile.WriteTo( m_passphrase, writer ); writer.Flush(); } exportfile.Flush(); #if DEBUG exportfile.Seek( 0, SeekOrigin.Begin ); using( XmlReader reader = InfoCardSchemas.CreateReader( exportfile ) ) { while( reader.Read() ); } #endif } } finally { foreach( InfoCard card in storeFile.Cards ) { card.ClearSensitiveData(); } } AuditLog.AuditStoreExport(); } catch( XmlSchemaValidationException e ) { throw IDT.ThrowHelperError( new ExportException( SR.GetString( SR.SchemaValidationFailed ), e ) ); } catch( XmlException e ) { throw IDT.ThrowHelperError( new ExportException( SR.GetString( SR.InvalidImportFile ), e ) ); } catch( UnauthorizedAccessException e ) { throw IDT.ThrowHelperError( new ExportException( SR.GetString( SR.ImportInaccesibleFile ), e ) ); } catch( IOException e ) { throw IDT.ThrowHelperError( new ExportException( SR.GetString( SR.InvalidImportFile ), e ) ); } catch( ArgumentException ae ) { // // FileInfo.Open can throw this exception for reserved file names // throw IDT.ThrowHelperError( new ExportException( SR.GetString( SR.ImportInaccesibleFile),ae ) ); } catch ( SerializationIncompleteException sie ) { // // This is an export, so we should expect to recover from all serialization failures // throw IDT.ThrowHelperError( new ExportException( SR.GetString( SR.FailedToSerializeObject, sie.ObjectType ), sie ) ); } finally { connection.Close(); } } protected override void OnMarshalOutArgs() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
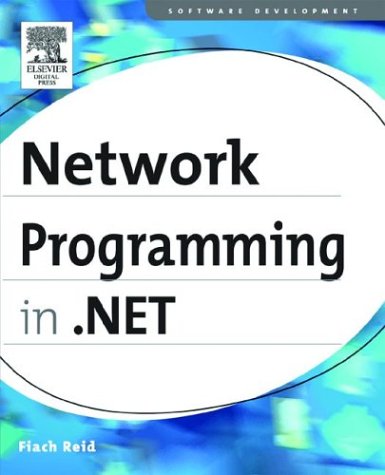
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CharAnimationBase.cs
- PeerInvitationResponse.cs
- wgx_sdk_version.cs
- Emitter.cs
- KeyPressEvent.cs
- Transform3DCollection.cs
- EntityReference.cs
- SecurityProtocolCorrelationState.cs
- SudsParser.cs
- __Filters.cs
- DBCSCodePageEncoding.cs
- UnmanagedMemoryStream.cs
- RIPEMD160Managed.cs
- FormsAuthenticationUserCollection.cs
- Baml2006ReaderFrame.cs
- CacheMemory.cs
- PassportAuthentication.cs
- WebDisplayNameAttribute.cs
- ObjectQueryState.cs
- EventManager.cs
- SamlDoNotCacheCondition.cs
- UrlMappingsSection.cs
- MetadataArtifactLoaderResource.cs
- InputLanguage.cs
- WebPartConnectVerb.cs
- SizeF.cs
- ReferencedType.cs
- CultureSpecificCharacterBufferRange.cs
- PrintPreviewDialog.cs
- EntityProviderServices.cs
- ValueUtilsSmi.cs
- XmlChildNodes.cs
- CompilationRelaxations.cs
- StorageMappingItemLoader.cs
- ClientCultureInfo.cs
- HitTestParameters3D.cs
- BitmapEffectState.cs
- RectAnimationBase.cs
- DataListItem.cs
- FixedPageStructure.cs
- CustomValidator.cs
- KoreanLunisolarCalendar.cs
- WrapPanel.cs
- CodeNamespace.cs
- BooleanSwitch.cs
- PixelFormats.cs
- SmiEventSink_Default.cs
- ScrollViewerAutomationPeer.cs
- CreateSequenceResponse.cs
- RequestResizeEvent.cs
- KeyConverter.cs
- PropertyPath.cs
- OleCmdHelper.cs
- EmbossBitmapEffect.cs
- CodeTypeDelegate.cs
- StrokeCollectionDefaultValueFactory.cs
- BrowserCapabilitiesCodeGenerator.cs
- OdbcError.cs
- SymLanguageVendor.cs
- DoubleAnimation.cs
- FakeModelItemImpl.cs
- ListBoxAutomationPeer.cs
- XmlMtomReader.cs
- HtmlSelect.cs
- EntityDataSourceEntitySetNameItem.cs
- Code.cs
- SplitContainer.cs
- RemotingConfiguration.cs
- DbFunctionCommandTree.cs
- SqlUdtInfo.cs
- CultureInfoConverter.cs
- ValueUtilsSmi.cs
- IISUnsafeMethods.cs
- FactoryMaker.cs
- XMLDiffLoader.cs
- Renderer.cs
- AnonymousIdentificationModule.cs
- XmlNotation.cs
- RandomNumberGenerator.cs
- FontDriver.cs
- GeneralTransform2DTo3D.cs
- CompiledXpathExpr.cs
- HuffModule.cs
- Msec.cs
- InheritanceRules.cs
- MenuCommand.cs
- WebPartAuthorizationEventArgs.cs
- XmlEncodedRawTextWriter.cs
- SafeWaitHandle.cs
- RegistrySecurity.cs
- AppDomain.cs
- ConfigurationElementCollection.cs
- FeatureAttribute.cs
- EntityProviderFactory.cs
- DATA_BLOB.cs
- MediaElement.cs
- IIS7WorkerRequest.cs
- SpeechSynthesizer.cs
- WebPartManagerDesigner.cs
- RenderTargetBitmap.cs