Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / Baml2006 / Baml2006ReaderFrame.cs / 1305600 / Baml2006ReaderFrame.cs
using System; using System.Collections.Generic; using System.Xaml; using System.Diagnostics; using MS.Internal.Xaml.Context; namespace System.Windows.Baml2006 { internal class Baml2006ReaderFrame : XamlFrame { protected Dictionary_namespaces; public Baml2006ReaderFrame() { DelayedConnectionId = -1; } public Baml2006ReaderFrame(Baml2006ReaderFrame source) { XamlType = source.XamlType; Member = source.Member; if (source._namespaces != null) { _namespaces = new Dictionary (source._namespaces); } } public override XamlFrame Clone() { return new Baml2006ReaderFrame(this); } public XamlType XamlType { get; set; } public XamlMember Member { get; set; } public KeyRecord Key { get; set; } public int DelayedConnectionId { get; set; } public XamlMember ContentProperty { get; set; } public bool FreezeFreezables { get; set; } public void AddNamespace(string prefix, string xamlNs) { if (null == _namespaces) { _namespaces = new Dictionary (); } _namespaces.Add(prefix, xamlNs); } public void SetNamespaces(Dictionary namespaces) { _namespaces = namespaces; } public bool TryGetNamespaceByPrefix(string prefix, out string xamlNs) { if (_namespaces != null && _namespaces.TryGetValue(prefix, out xamlNs)) { return true; } xamlNs = null; return false; } public bool TryGetPrefixByNamespace(string xamlNs, out string prefix) { if (_namespaces != null) { foreach (KeyValuePair pair in _namespaces) { if (pair.Value == xamlNs) { prefix = pair.Key; return true; } } } prefix = null; return false; } public override void Reset() { XamlType = null; Member = null; if (_namespaces != null) { _namespaces.Clear(); } Flags = Baml2006ReaderFrameFlags.None; LineNumber = 0; LineOffset = 0; IsDeferredContent = false; Key = null; DelayedConnectionId = -1; ContentProperty = null; } public Baml2006ReaderFrameFlags Flags { get; set; } public int LineNumber { get; set; } public int LineOffset { get; set; } public bool IsDeferredContent { get; set; } } internal enum Baml2006ReaderFrameFlags:byte { None, IsImplict, HasImplicitProperty } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
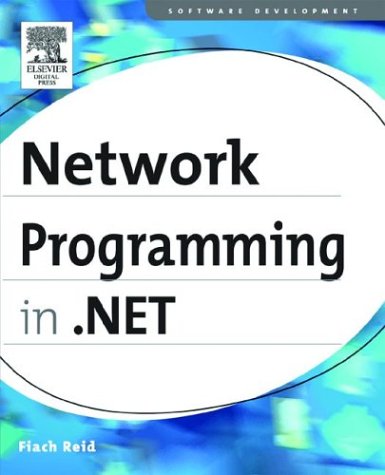
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditBehavior.cs
- WSTrust.cs
- SqlUtils.cs
- TranslateTransform3D.cs
- WebEventTraceProvider.cs
- Avt.cs
- ExceptionHelpers.cs
- ColumnReorderedEventArgs.cs
- UnmanagedMarshal.cs
- FaultReason.cs
- AffineTransform3D.cs
- LoadGrammarCompletedEventArgs.cs
- __ComObject.cs
- OptimizedTemplateContentHelper.cs
- ModelProperty.cs
- TransactedBatchContext.cs
- VisualBrush.cs
- PointAnimationUsingKeyFrames.cs
- IndexOutOfRangeException.cs
- DbConnectionPool.cs
- RowCache.cs
- ReaderWriterLock.cs
- RoleExceptions.cs
- UpDownEvent.cs
- CodeDirectionExpression.cs
- ListControl.cs
- ColorContextHelper.cs
- GridViewRowEventArgs.cs
- PropertyGridEditorPart.cs
- EtwTrace.cs
- ConfigurationStrings.cs
- ProxyWebPartConnectionCollection.cs
- Paragraph.cs
- WorkflowApplicationCompletedException.cs
- Char.cs
- SupportsEventValidationAttribute.cs
- QueryableDataSourceEditData.cs
- AnimationLayer.cs
- SplitterEvent.cs
- ImageAnimator.cs
- ThreadInterruptedException.cs
- DataSpaceManager.cs
- DateTimeConstantAttribute.cs
- PrimitiveCodeDomSerializer.cs
- ProcessThread.cs
- PathGeometry.cs
- PointCollectionConverter.cs
- Block.cs
- ChangeTracker.cs
- ExceptionCollection.cs
- FontFamilyConverter.cs
- ISAPIWorkerRequest.cs
- ThemeableAttribute.cs
- PowerModeChangedEventArgs.cs
- _BaseOverlappedAsyncResult.cs
- PropertyPushdownHelper.cs
- ImageIndexConverter.cs
- JsonXmlDataContract.cs
- BlurBitmapEffect.cs
- HttpRawResponse.cs
- FieldBuilder.cs
- BamlResourceDeserializer.cs
- TextChange.cs
- DataReaderContainer.cs
- CommandManager.cs
- UserUseLicenseDictionaryLoader.cs
- PopupEventArgs.cs
- XmlValueConverter.cs
- KeyedCollection.cs
- WindowShowOrOpenTracker.cs
- CodeAssignStatement.cs
- DragCompletedEventArgs.cs
- ISSmlParser.cs
- SiteMap.cs
- MetadataReference.cs
- DataContractFormatAttribute.cs
- BufferAllocator.cs
- controlskin.cs
- DropShadowEffect.cs
- DataTableNameHandler.cs
- PartialClassGenerationTask.cs
- CopyAttributesAction.cs
- SR.cs
- SubMenuStyleCollection.cs
- XmlSchemaSimpleContentRestriction.cs
- MessagePropertyVariants.cs
- NavigateEvent.cs
- webproxy.cs
- SqlDataSourceConfigureFilterForm.cs
- NativeRightsManagementAPIsStructures.cs
- ProtocolInformationReader.cs
- ListViewCommandEventArgs.cs
- SuppressMergeCheckAttribute.cs
- HttpCacheVaryByContentEncodings.cs
- ToolStrip.cs
- DependsOnAttribute.cs
- LinkLabelLinkClickedEvent.cs
- XslException.cs
- PasswordDeriveBytes.cs
- Pen.cs