Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Recognition / SrgsGrammar / SrgsElement.cs / 1 / SrgsElement.cs
//---------------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Xml; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Speech.Internal.SrgsParser; namespace System.Speech.Recognition.SrgsGrammar { ////// Base class for all SRGS object to build XML fragment corresponding to the object. /// [Serializable] [DebuggerDisplay ("SrgsElement Children:[{_items.Count}]")] [DebuggerTypeProxy (typeof (SrgsElementDebugDisplay))] public abstract class SrgsElement : MarshalByRefObject, IElement { ////// TODOC /// protected SrgsElement () { } //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal methods // Write the XML fragment describing the object. internal abstract void WriteSrgs (XmlWriter writer); // Debugger display string. internal abstract string DebuggerDisplayString (); // Validate the SRGS element. ////// Validate each element and recurse through all the children srgs /// elements if any. /// Any derived class implementing this mehod must call the base class /// in order for the children to be processed. /// internal virtual void Validate (SrgsGrammar grammar) { foreach (SrgsElement element in Children) { // Child validation element.Validate (grammar); } } void IElement.PostParse (IElement parent) { } #endregion //******************************************************************** // // Protected Properties // //******************************************************************* #region Protected Properties //TODOC Add Documentation virtual internal SrgsElement [] Children { get { return new SrgsElement [0]; } } #endregion //******************************************************************** // // Private Types // //******************************************************************** #region Private Types // Used by the debbugger display attribute internal class SrgsElementDebugDisplay { public SrgsElementDebugDisplay (SrgsElement element) { _elements = element.Children; } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SrgsElement [] AKeys { get { return _elements; } } private SrgsElement [] _elements; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // // Description: // // History: // 5/1/2004 jeanfp Created from the Kurosawa Code //--------------------------------------------------------------------------- using System; using System.Xml; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Speech.Internal.SrgsParser; namespace System.Speech.Recognition.SrgsGrammar { ////// Base class for all SRGS object to build XML fragment corresponding to the object. /// [Serializable] [DebuggerDisplay ("SrgsElement Children:[{_items.Count}]")] [DebuggerTypeProxy (typeof (SrgsElementDebugDisplay))] public abstract class SrgsElement : MarshalByRefObject, IElement { ////// TODOC /// protected SrgsElement () { } //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal methods // Write the XML fragment describing the object. internal abstract void WriteSrgs (XmlWriter writer); // Debugger display string. internal abstract string DebuggerDisplayString (); // Validate the SRGS element. ////// Validate each element and recurse through all the children srgs /// elements if any. /// Any derived class implementing this mehod must call the base class /// in order for the children to be processed. /// internal virtual void Validate (SrgsGrammar grammar) { foreach (SrgsElement element in Children) { // Child validation element.Validate (grammar); } } void IElement.PostParse (IElement parent) { } #endregion //******************************************************************** // // Protected Properties // //******************************************************************* #region Protected Properties //TODOC Add Documentation virtual internal SrgsElement [] Children { get { return new SrgsElement [0]; } } #endregion //******************************************************************** // // Private Types // //******************************************************************** #region Private Types // Used by the debbugger display attribute internal class SrgsElementDebugDisplay { public SrgsElementDebugDisplay (SrgsElement element) { _elements = element.Children; } [DebuggerBrowsable (DebuggerBrowsableState.RootHidden)] public SrgsElement [] AKeys { get { return _elements; } } private SrgsElement [] _elements; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
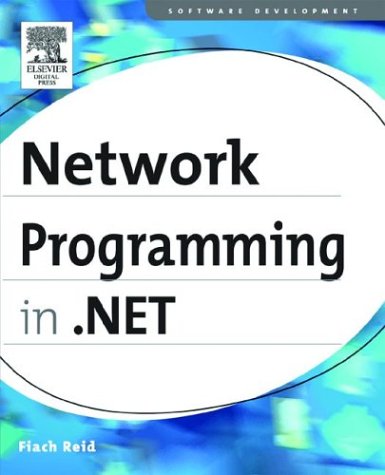
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextBoxLine.cs
- HtmlShim.cs
- TypedTableBase.cs
- XmlNamespaceMapping.cs
- DesignerHost.cs
- ProcessStartInfo.cs
- PartialArray.cs
- DeploymentSection.cs
- ChildTable.cs
- WebPartDescriptionCollection.cs
- VBIdentifierName.cs
- SendSecurityHeader.cs
- ListView.cs
- SqlProviderServices.cs
- DataSetUtil.cs
- SqlErrorCollection.cs
- AssemblySettingAttributes.cs
- ResourceDescriptionAttribute.cs
- Camera.cs
- DebugView.cs
- BreadCrumbTextConverter.cs
- InputDevice.cs
- SQLDouble.cs
- SchemaSetCompiler.cs
- ToolStripLocationCancelEventArgs.cs
- ObjectDataSourceStatusEventArgs.cs
- ScriptReference.cs
- Policy.cs
- MetadataUtilsSmi.cs
- BooleanStorage.cs
- Int32AnimationUsingKeyFrames.cs
- ProfileGroupSettingsCollection.cs
- ListViewItemEventArgs.cs
- InstanceCreationEditor.cs
- SimpleWebHandlerParser.cs
- Int32CAMarshaler.cs
- CodeParameterDeclarationExpression.cs
- DataList.cs
- DataGridViewRowsAddedEventArgs.cs
- TransformCryptoHandle.cs
- EventWaitHandleSecurity.cs
- DeviceSpecificChoiceCollection.cs
- AsymmetricAlgorithm.cs
- ComponentCommands.cs
- NativeMethods.cs
- OdbcEnvironment.cs
- StandardToolWindows.cs
- RowsCopiedEventArgs.cs
- Base64Stream.cs
- WebConfigurationHost.cs
- ObjectParameterCollection.cs
- DataControlFieldHeaderCell.cs
- StringDictionaryEditor.cs
- Internal.cs
- BeginGetFileNameFromUserRequest.cs
- EntityTypeEmitter.cs
- MessageDroppedTraceRecord.cs
- HttpRawResponse.cs
- PeerObject.cs
- SqlBulkCopyColumnMappingCollection.cs
- XmlDictionaryReaderQuotas.cs
- Marshal.cs
- Triplet.cs
- DynamicMethod.cs
- PriorityBindingExpression.cs
- JavaScriptString.cs
- AssociationTypeEmitter.cs
- DocumentSequenceHighlightLayer.cs
- MetadataElement.cs
- FlowDocumentPage.cs
- ColumnTypeConverter.cs
- SystemIPv4InterfaceProperties.cs
- SqlDataSourceSummaryPanel.cs
- FillErrorEventArgs.cs
- GroupItem.cs
- LoadItemsEventArgs.cs
- PersonalizationDictionary.cs
- ToolStripContentPanelRenderEventArgs.cs
- HwndSubclass.cs
- SimpleHandlerFactory.cs
- SiteMapDataSourceView.cs
- Vector3D.cs
- DataTableCollection.cs
- PieceNameHelper.cs
- StartUpEventArgs.cs
- TemplateField.cs
- TextServicesCompartmentEventSink.cs
- sqlpipe.cs
- MetafileHeaderWmf.cs
- EmbeddedMailObjectsCollection.cs
- PowerModeChangedEventArgs.cs
- RuntimeWrappedException.cs
- DefaultMemberAttribute.cs
- TokenBasedSetEnumerator.cs
- ConnectionConsumerAttribute.cs
- Validator.cs
- ValidationErrorCollection.cs
- VerticalConnector.xaml.cs
- UniformGrid.cs
- ToolZoneDesigner.cs