Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextLineBreak.cs / 1 / TextLineBreak.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextLineBreak.cs // // Contents: Text properties and state at the point where text is broken // by the line breaking process, which may need to be carried over // when formatting the next line. // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 12-5-2004 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Security; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Media; using MS.Internal; using MS.Internal.TextFormatting; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { ////// Text properties and state at the point where text is broken /// by the line breaking process. /// public sealed class TextLineBreak : IDisposable { private TextModifierScope _currentScope; private SecurityCriticalDataForSet_breakRecord; #region Constructors /// /// Internallly construct the line break /// internal TextLineBreak( TextModifierScope currentScope, SecurityCriticalDataForSetbreakRecord ) { _currentScope = currentScope; _breakRecord = breakRecord; if (breakRecord.Value == IntPtr.Zero) { // this object does not hold unmanaged resource, // remove it from the finalizer queue. GC.SuppressFinalize(this); } } #endregion /// /// Finalize the line break /// ~TextLineBreak() { DisposeInternal(true); } ////// Dispose the line break /// public void Dispose() { DisposeInternal(false); GC.SuppressFinalize(this); } ////// Clone a new instance of TextLineBreak /// ////// Critical - as this calls unmanaged API LoCloneBreakRecord. /// PublicOK - as it takes no parameter and retain no additional unmanaged resource. /// [SecurityCritical] public TextLineBreak Clone() { IntPtr pbreakrec = IntPtr.Zero; if (_breakRecord.Value != IntPtr.Zero) { LsErr lserr = UnsafeNativeMethods.LoCloneBreakRecord(_breakRecord.Value, out pbreakrec); if (lserr != LsErr.None) { TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.CloneBreakRecordFailure, lserr), lserr); } } return new TextLineBreak(_currentScope, new SecurityCriticalDataForSet(pbreakrec)); } /// /// Destroy LS unmanaged break records object inside the line break /// managed object. The parameter flag indicates whether the call is /// from finalizer thread or the main UI thread. /// ////// Critical - as this calls the setter of _breakRecord.Value which is type SecurityCriticalDataForSet. /// _breakRecord is the value received from call to LoCreateBreaks and being passed back in /// when building the next break. No code should have access to set it otherwise. /// Safe - as this does not take any parameter that it passes directly to the critical function. /// [SecurityCritical, SecurityTreatAsSafe] private void DisposeInternal(bool finalizing) { if (_breakRecord.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposeBreakRecord(_breakRecord.Value, finalizing); _breakRecord.Value = IntPtr.Zero; GC.KeepAlive(this); } } ////// Current text modifier scope, which can be null. /// internal TextModifierScope TextModifierScope { get { return _currentScope; } } ////// Unmanaged pointer to LS break records structure /// internal SecurityCriticalDataForSetBreakRecord { get { return _breakRecord; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextLineBreak.cs // // Contents: Text properties and state at the point where text is broken // by the line breaking process, which may need to be carried over // when formatting the next line. // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 12-5-2004 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Security; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Media; using MS.Internal; using MS.Internal.TextFormatting; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { /// /// Text properties and state at the point where text is broken /// by the line breaking process. /// public sealed class TextLineBreak : IDisposable { private TextModifierScope _currentScope; private SecurityCriticalDataForSet_breakRecord; #region Constructors /// /// Internallly construct the line break /// internal TextLineBreak( TextModifierScope currentScope, SecurityCriticalDataForSetbreakRecord ) { _currentScope = currentScope; _breakRecord = breakRecord; if (breakRecord.Value == IntPtr.Zero) { // this object does not hold unmanaged resource, // remove it from the finalizer queue. GC.SuppressFinalize(this); } } #endregion /// /// Finalize the line break /// ~TextLineBreak() { DisposeInternal(true); } ////// Dispose the line break /// public void Dispose() { DisposeInternal(false); GC.SuppressFinalize(this); } ////// Clone a new instance of TextLineBreak /// ////// Critical - as this calls unmanaged API LoCloneBreakRecord. /// PublicOK - as it takes no parameter and retain no additional unmanaged resource. /// [SecurityCritical] public TextLineBreak Clone() { IntPtr pbreakrec = IntPtr.Zero; if (_breakRecord.Value != IntPtr.Zero) { LsErr lserr = UnsafeNativeMethods.LoCloneBreakRecord(_breakRecord.Value, out pbreakrec); if (lserr != LsErr.None) { TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.CloneBreakRecordFailure, lserr), lserr); } } return new TextLineBreak(_currentScope, new SecurityCriticalDataForSet(pbreakrec)); } /// /// Destroy LS unmanaged break records object inside the line break /// managed object. The parameter flag indicates whether the call is /// from finalizer thread or the main UI thread. /// ////// Critical - as this calls the setter of _breakRecord.Value which is type SecurityCriticalDataForSet. /// _breakRecord is the value received from call to LoCreateBreaks and being passed back in /// when building the next break. No code should have access to set it otherwise. /// Safe - as this does not take any parameter that it passes directly to the critical function. /// [SecurityCritical, SecurityTreatAsSafe] private void DisposeInternal(bool finalizing) { if (_breakRecord.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposeBreakRecord(_breakRecord.Value, finalizing); _breakRecord.Value = IntPtr.Zero; GC.KeepAlive(this); } } ////// Current text modifier scope, which can be null. /// internal TextModifierScope TextModifierScope { get { return _currentScope; } } ////// Unmanaged pointer to LS break records structure /// internal SecurityCriticalDataForSetBreakRecord { get { return _breakRecord; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
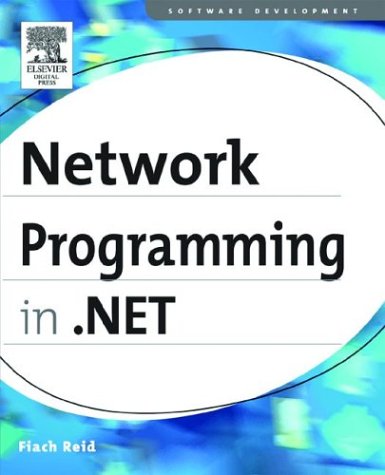
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CurrencyWrapper.cs
- HyperLinkDataBindingHandler.cs
- WindowsIPAddress.cs
- Encoder.cs
- AttributeCollection.cs
- HtmlFormParameterWriter.cs
- TextServicesCompartment.cs
- XmlSchemaChoice.cs
- XmlSchemaAttributeGroupRef.cs
- EventLogEntryCollection.cs
- PeerName.cs
- ServiceTimeoutsBehavior.cs
- String.cs
- PropertyManager.cs
- TagPrefixInfo.cs
- FixedSOMGroup.cs
- NameValueCollection.cs
- ToolboxItemAttribute.cs
- StructuralCache.cs
- TextAutomationPeer.cs
- WebConfigurationManager.cs
- FormViewPagerRow.cs
- EmptyEnumerator.cs
- SplitterPanelDesigner.cs
- LinkButton.cs
- GeometryValueSerializer.cs
- SqlUserDefinedAggregateAttribute.cs
- RelatedPropertyManager.cs
- SizeLimitedCache.cs
- ListBoxItem.cs
- AttachedAnnotation.cs
- SqlClientMetaDataCollectionNames.cs
- MemberRelationshipService.cs
- RepeaterCommandEventArgs.cs
- Adorner.cs
- Tuple.cs
- NameValueCollection.cs
- EntityDataSourceDesigner.cs
- TextAnchor.cs
- Rijndael.cs
- SchemaCollectionCompiler.cs
- BinHexEncoding.cs
- IncrementalHitTester.cs
- ApplicationContext.cs
- DocumentPaginator.cs
- WebPartCloseVerb.cs
- DoubleAnimationBase.cs
- LongCountAggregationOperator.cs
- LinqDataSourceContextData.cs
- TextTreeDeleteContentUndoUnit.cs
- DataStorage.cs
- StrongNameMembershipCondition.cs
- TemplateDefinition.cs
- WindowsGraphicsCacheManager.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- UnSafeCharBuffer.cs
- Calendar.cs
- SerialPort.cs
- StylusCaptureWithinProperty.cs
- AccessDataSourceDesigner.cs
- SafeEventLogWriteHandle.cs
- MessageBox.cs
- storepermission.cs
- ToolboxItem.cs
- EtwTrace.cs
- ListViewInsertionMark.cs
- AccessDataSourceView.cs
- TextContainerChangeEventArgs.cs
- PropertyMetadata.cs
- CompoundFileStorageReference.cs
- KeyEvent.cs
- HashAlgorithm.cs
- DbConnectionClosed.cs
- Popup.cs
- AmbientEnvironment.cs
- BooleanConverter.cs
- ConfigurationPropertyCollection.cs
- XmlSchemaExporter.cs
- ListControl.cs
- Unit.cs
- ImageAnimator.cs
- CalendarBlackoutDatesCollection.cs
- Substitution.cs
- DocumentViewerBase.cs
- PointConverter.cs
- HttpPostedFile.cs
- Page.cs
- ConstructorBuilder.cs
- DocumentViewerBaseAutomationPeer.cs
- SoapReflectionImporter.cs
- RowUpdatingEventArgs.cs
- XmlSchemaSubstitutionGroup.cs
- TableLayoutSettings.cs
- CodeTypeMember.cs
- RenderCapability.cs
- __TransparentProxy.cs
- HyperLinkStyle.cs
- CodeTypeReference.cs
- WmlLabelAdapter.cs
- Pts.cs