Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / BinHexDecoder.cs / 1 / BinHexDecoder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Xml { internal class BinHexDecoder : IncrementalReadDecoder { // // Fields // byte[] buffer; int startIndex; int curIndex; int endIndex; bool hasHalfByteCached; byte cachedHalfByte; // // IncrementalReadDecoder interface // internal override int DecodedCount { get { return curIndex - startIndex; } } internal override bool IsFull { get { return curIndex == endIndex; } } internal override unsafe int Decode( char[] chars, int startPos, int len ) { Debug.Assert( chars != null ); Debug.Assert( len >= 0 ); Debug.Assert( startPos >= 0 ); Debug.Assert( chars.Length - startPos >= len ); if ( len == 0 ) { return 0; } int bytesDecoded, charsDecoded; fixed ( char* pChars = &chars[startPos] ) { fixed ( byte* pBytes = &buffer[curIndex] ) { Decode( pChars, pChars + len, pBytes, pBytes + ( endIndex - curIndex ), ref this.hasHalfByteCached, ref this.cachedHalfByte, out charsDecoded, out bytesDecoded ); } } curIndex += bytesDecoded; return charsDecoded; } internal override unsafe int Decode( string str, int startPos, int len ) { Debug.Assert( str != null ); Debug.Assert( len >= 0 ); Debug.Assert( startPos >= 0 ); Debug.Assert( str.Length - startPos >= len ); if ( len == 0 ) { return 0; } int bytesDecoded, charsDecoded; fixed ( char* pChars = str ) { fixed ( byte* pBytes = &buffer[curIndex] ) { Decode( pChars + startPos, pChars + startPos + len, pBytes, pBytes + ( endIndex - curIndex ), ref this.hasHalfByteCached, ref this.cachedHalfByte, out charsDecoded, out bytesDecoded ); } } curIndex += bytesDecoded; return charsDecoded; } internal override void Reset() { this.hasHalfByteCached = false; this.cachedHalfByte = 0; } internal override void SetNextOutputBuffer( Array buffer, int index, int count ) { Debug.Assert( buffer != null ); Debug.Assert( count >= 0 ); Debug.Assert( index >= 0 ); Debug.Assert( buffer.Length - index >= count ); Debug.Assert( ( buffer as byte[] ) != null ); this.buffer = (byte[])buffer; this.startIndex = index; this.curIndex = index; this.endIndex = index + count; } // // Static methods // public static unsafe byte[] Decode( char[] chars, bool allowOddChars ) { if ( chars == null ) { throw new ArgumentException( "chars" ); } int len = chars.Length; if ( len == 0 ) { return new byte[0]; } byte[] bytes = new byte[ ( len + 1 ) / 2 ]; int bytesDecoded, charsDecoded; bool hasHalfByteCached = false; byte cachedHalfByte = 0; fixed ( char* pChars = &chars[0] ) { fixed ( byte* pBytes = &bytes[0] ) { Decode( pChars, pChars + len, pBytes, pBytes + bytes.Length, ref hasHalfByteCached, ref cachedHalfByte, out charsDecoded, out bytesDecoded ); } } if ( hasHalfByteCached && !allowOddChars ) { throw new XmlException( Res.Xml_InvalidBinHexValueOddCount, new string( chars ) ); } if ( bytesDecoded < bytes.Length ) { byte[] tmp = new byte[ bytesDecoded ]; Buffer.BlockCopy( bytes, 0, tmp, 0, bytesDecoded ); bytes = tmp; } return bytes; } // // Private methods // private static unsafe void Decode( char* pChars, char *pCharsEndPos, byte* pBytes, byte* pBytesEndPos, ref bool hasHalfByteCached, ref byte cachedHalfByte, out int charsDecoded, out int bytesDecoded ) { #if DEBUG Debug.Assert( pCharsEndPos - pChars >= 0 ); Debug.Assert( pBytesEndPos - pBytes >= 0 ); #endif char* pChar = pChars; byte* pByte = pBytes; XmlCharType xmlCharType = XmlCharType.Instance; while ( pChar < pCharsEndPos && pByte < pBytesEndPos ) { byte halfByte; char ch = *pChar++; if ( ch >= 'a' && ch <= 'f' ) { halfByte = (byte)(ch - 'a' + 10); } else if ( ch >= 'A' && ch <= 'F' ) { halfByte = (byte)(ch - 'A' + 10); } else if ( ch >= '0' && ch <= '9' ) { halfByte = (byte)(ch - '0'); } else if ( ( xmlCharType.charProperties[ch] & XmlCharType.fWhitespace ) != 0 ) { // else if ( xmlCharType.IsWhiteSpace( ch ) ) { continue; } else { throw new XmlException(Res.Xml_InvalidBinHexValue, new string( pChars, 0, (int)( pCharsEndPos - pChars ) ) ); } if ( hasHalfByteCached ) { *pByte++ = (byte)( ( cachedHalfByte << 4 ) + halfByte ); hasHalfByteCached = false; } else { cachedHalfByte = halfByte; hasHalfByteCached = true; } } bytesDecoded = (int)(pByte - pBytes); charsDecoded = (int)(pChar - pChars); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Xml { internal class BinHexDecoder : IncrementalReadDecoder { // // Fields // byte[] buffer; int startIndex; int curIndex; int endIndex; bool hasHalfByteCached; byte cachedHalfByte; // // IncrementalReadDecoder interface // internal override int DecodedCount { get { return curIndex - startIndex; } } internal override bool IsFull { get { return curIndex == endIndex; } } internal override unsafe int Decode( char[] chars, int startPos, int len ) { Debug.Assert( chars != null ); Debug.Assert( len >= 0 ); Debug.Assert( startPos >= 0 ); Debug.Assert( chars.Length - startPos >= len ); if ( len == 0 ) { return 0; } int bytesDecoded, charsDecoded; fixed ( char* pChars = &chars[startPos] ) { fixed ( byte* pBytes = &buffer[curIndex] ) { Decode( pChars, pChars + len, pBytes, pBytes + ( endIndex - curIndex ), ref this.hasHalfByteCached, ref this.cachedHalfByte, out charsDecoded, out bytesDecoded ); } } curIndex += bytesDecoded; return charsDecoded; } internal override unsafe int Decode( string str, int startPos, int len ) { Debug.Assert( str != null ); Debug.Assert( len >= 0 ); Debug.Assert( startPos >= 0 ); Debug.Assert( str.Length - startPos >= len ); if ( len == 0 ) { return 0; } int bytesDecoded, charsDecoded; fixed ( char* pChars = str ) { fixed ( byte* pBytes = &buffer[curIndex] ) { Decode( pChars + startPos, pChars + startPos + len, pBytes, pBytes + ( endIndex - curIndex ), ref this.hasHalfByteCached, ref this.cachedHalfByte, out charsDecoded, out bytesDecoded ); } } curIndex += bytesDecoded; return charsDecoded; } internal override void Reset() { this.hasHalfByteCached = false; this.cachedHalfByte = 0; } internal override void SetNextOutputBuffer( Array buffer, int index, int count ) { Debug.Assert( buffer != null ); Debug.Assert( count >= 0 ); Debug.Assert( index >= 0 ); Debug.Assert( buffer.Length - index >= count ); Debug.Assert( ( buffer as byte[] ) != null ); this.buffer = (byte[])buffer; this.startIndex = index; this.curIndex = index; this.endIndex = index + count; } // // Static methods // public static unsafe byte[] Decode( char[] chars, bool allowOddChars ) { if ( chars == null ) { throw new ArgumentException( "chars" ); } int len = chars.Length; if ( len == 0 ) { return new byte[0]; } byte[] bytes = new byte[ ( len + 1 ) / 2 ]; int bytesDecoded, charsDecoded; bool hasHalfByteCached = false; byte cachedHalfByte = 0; fixed ( char* pChars = &chars[0] ) { fixed ( byte* pBytes = &bytes[0] ) { Decode( pChars, pChars + len, pBytes, pBytes + bytes.Length, ref hasHalfByteCached, ref cachedHalfByte, out charsDecoded, out bytesDecoded ); } } if ( hasHalfByteCached && !allowOddChars ) { throw new XmlException( Res.Xml_InvalidBinHexValueOddCount, new string( chars ) ); } if ( bytesDecoded < bytes.Length ) { byte[] tmp = new byte[ bytesDecoded ]; Buffer.BlockCopy( bytes, 0, tmp, 0, bytesDecoded ); bytes = tmp; } return bytes; } // // Private methods // private static unsafe void Decode( char* pChars, char *pCharsEndPos, byte* pBytes, byte* pBytesEndPos, ref bool hasHalfByteCached, ref byte cachedHalfByte, out int charsDecoded, out int bytesDecoded ) { #if DEBUG Debug.Assert( pCharsEndPos - pChars >= 0 ); Debug.Assert( pBytesEndPos - pBytes >= 0 ); #endif char* pChar = pChars; byte* pByte = pBytes; XmlCharType xmlCharType = XmlCharType.Instance; while ( pChar < pCharsEndPos && pByte < pBytesEndPos ) { byte halfByte; char ch = *pChar++; if ( ch >= 'a' && ch <= 'f' ) { halfByte = (byte)(ch - 'a' + 10); } else if ( ch >= 'A' && ch <= 'F' ) { halfByte = (byte)(ch - 'A' + 10); } else if ( ch >= '0' && ch <= '9' ) { halfByte = (byte)(ch - '0'); } else if ( ( xmlCharType.charProperties[ch] & XmlCharType.fWhitespace ) != 0 ) { // else if ( xmlCharType.IsWhiteSpace( ch ) ) { continue; } else { throw new XmlException(Res.Xml_InvalidBinHexValue, new string( pChars, 0, (int)( pCharsEndPos - pChars ) ) ); } if ( hasHalfByteCached ) { *pByte++ = (byte)( ( cachedHalfByte << 4 ) + halfByte ); hasHalfByteCached = false; } else { cachedHalfByte = halfByte; hasHalfByteCached = true; } } bytesDecoded = (int)(pByte - pBytes); charsDecoded = (int)(pChar - pChars); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
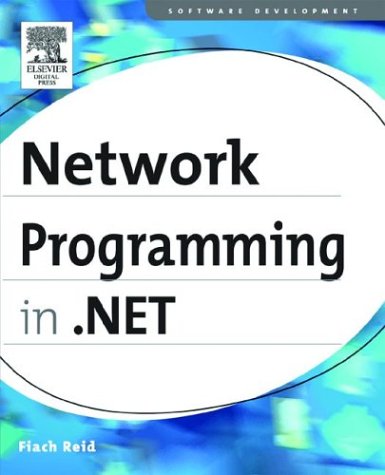
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectCloneHelper.cs
- HtmlMeta.cs
- PhysicalFontFamily.cs
- Focus.cs
- TargetConverter.cs
- SqlNotificationEventArgs.cs
- ResourceDescriptionAttribute.cs
- WebPartDescriptionCollection.cs
- CharUnicodeInfo.cs
- XmlUTF8TextReader.cs
- PackageRelationship.cs
- XmlSchemaSimpleContentExtension.cs
- SelectionPatternIdentifiers.cs
- ServiceSecurityAuditElement.cs
- SchemaTableColumn.cs
- MenuCommand.cs
- TimeEnumHelper.cs
- SHA1.cs
- ToolStripLabel.cs
- MetadataArtifactLoaderComposite.cs
- OptimalBreakSession.cs
- ArgumentsParser.cs
- SchemaCollectionCompiler.cs
- ManagementQuery.cs
- SaveWorkflowCommand.cs
- DeleteMemberBinder.cs
- ScriptingJsonSerializationSection.cs
- CriticalFinalizerObject.cs
- ChangeNode.cs
- IisTraceWebEventProvider.cs
- HighContrastHelper.cs
- RubberbandSelector.cs
- ChineseLunisolarCalendar.cs
- MessageQueueEnumerator.cs
- DataGridViewCellStyleChangedEventArgs.cs
- TextMetrics.cs
- DataPagerField.cs
- PeerNameResolver.cs
- VariableAction.cs
- UIElementCollection.cs
- WebAdminConfigurationHelper.cs
- WebPartConnectionsEventArgs.cs
- XPathNodeIterator.cs
- PreviewPrintController.cs
- PersonalizationDictionary.cs
- ColorPalette.cs
- ContextDataSourceContextData.cs
- SHA1CryptoServiceProvider.cs
- Interop.cs
- EntityViewGenerator.cs
- WCFBuildProvider.cs
- ModelTreeManager.cs
- DelegatingChannelListener.cs
- DeploymentExceptionMapper.cs
- XmlImplementation.cs
- DatatypeImplementation.cs
- Sentence.cs
- MiniMapControl.xaml.cs
- DesigntimeLicenseContext.cs
- EventLogRecord.cs
- HandlerMappingMemo.cs
- OverrideMode.cs
- String.cs
- DocumentOutline.cs
- BuildDependencySet.cs
- Utils.cs
- JoinCqlBlock.cs
- ArrayTypeMismatchException.cs
- ClosableStream.cs
- ApplicationContext.cs
- AssociationSet.cs
- OleDbWrapper.cs
- TableColumn.cs
- BookmarkManager.cs
- Journaling.cs
- FrameworkContentElementAutomationPeer.cs
- WinOEToolBoxItem.cs
- BooleanToVisibilityConverter.cs
- FlagsAttribute.cs
- OrderPreservingPipeliningMergeHelper.cs
- FileInfo.cs
- GuidelineSet.cs
- JsonDeserializer.cs
- DataRelation.cs
- ChildrenQuery.cs
- DefaultHttpHandler.cs
- Viewport3DVisual.cs
- DoubleUtil.cs
- BuildProvider.cs
- CodeStatementCollection.cs
- ApplicationHost.cs
- XmlSchemaSequence.cs
- MenuAutomationPeer.cs
- ComEventsMethod.cs
- IgnoreSectionHandler.cs
- IndependentlyAnimatedPropertyMetadata.cs
- CfgSemanticTag.cs
- ProviderConnectionPointCollection.cs
- HostProtectionPermission.cs
- TransformedBitmap.cs