Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / VariableAction.cs / 1305376 / VariableAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Diagnostics; using System.Xml.XPath; namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; internal enum VariableType { GlobalVariable, GlobalParameter, LocalVariable, LocalParameter, WithParameter, } internal class VariableAction : ContainerAction, IXsltContextVariable { public static object BeingComputedMark = new object(); private const int ValueCalculated = 2; protected XmlQualifiedName name; protected string nameStr; protected string baseUri; protected int selectKey = Compiler.InvalidQueryKey; protected int stylesheetid; protected VariableType varType; private int varKey; internal int Stylesheetid { get { return this.stylesheetid; } } internal XmlQualifiedName Name { get { return this.name; } } internal string NameStr { get { return this.nameStr; } } internal VariableType VarType { get { return this.varType; } } internal int VarKey { get { return this.varKey; } } internal bool IsGlobal { get { return this.varType == VariableType.GlobalVariable || this.varType == VariableType.GlobalParameter; } } internal VariableAction(VariableType type) { this.varType = type; } internal override void Compile(Compiler compiler) { this.stylesheetid = compiler.Stylesheetid; this.baseUri = compiler.Input.BaseURI; CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.name, "name"); if (compiler.Recurse()) { CompileTemplate(compiler); compiler.ToParent(); if (this.selectKey != Compiler.InvalidQueryKey && this.containedActions != null) { throw XsltException.Create(Res.Xslt_VariableCntSel2, this.nameStr); } } if (this.containedActions != null) { baseUri = baseUri + '#' + compiler.GetUnicRtfId(); } else { baseUri = null; } this.varKey = compiler.InsertVariable(this); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Ref.Equal(name, compiler.Atoms.Name)) { Debug.Assert(this.name == null && this.nameStr == null); this.nameStr = value; this.name = compiler.CreateXPathQName(this.nameStr); } else if (Ref.Equal(name, compiler.Atoms.Select)) { this.selectKey = compiler.AddQuery(value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null && frame.State != ValueCalculated); object value = null; switch(frame.State) { case Initialized: if (IsGlobal) { if (frame.GetVariable(this.varKey) != null) { // This var was calculated already frame.Finished(); break; } // Mark that the variable is being computed to check for circular references frame.SetVariable(this.varKey, BeingComputedMark); } // If this is a parameter, check whether the caller has passed the value if (this.varType == VariableType.GlobalParameter) { value = processor.GetGlobalParameter(this.name); } else if (this.varType == VariableType.LocalParameter) { value = processor.GetParameter(this.name); } if (value != null) { goto case ValueCalculated; } // If value was not passed, check the 'select' attribute if (this.selectKey != Compiler.InvalidQueryKey) { value = processor.RunQuery(frame, this.selectKey); goto case ValueCalculated; } // If there is no 'select' attribute and the content is empty, use the empty string if (this.containedActions == null) { value = string.Empty; goto case ValueCalculated; } // RTF case NavigatorOutput output = new NavigatorOutput(this.baseUri); processor.PushOutput(output); processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; case ProcessingChildren: RecordOutput recOutput = processor.PopOutput(); Debug.Assert(recOutput is NavigatorOutput); value = ((NavigatorOutput)recOutput).Navigator; goto case ValueCalculated; case ValueCalculated: Debug.Assert(value != null); frame.SetVariable(this.varKey, value); frame.Finished(); break; default: Debug.Fail("Invalid execution state inside VariableAction.Execute"); break; } } // ---------------------- IXsltContextVariable -------------------- XPathResultType IXsltContextVariable.VariableType { get { return XPathResultType.Any; } } object IXsltContextVariable.Evaluate(XsltContext xsltContext) { return ((XsltCompileContext)xsltContext).EvaluateVariable(this); } bool IXsltContextVariable.IsLocal { get { return this.varType == VariableType.LocalVariable || this.varType == VariableType.LocalParameter; } } bool IXsltContextVariable.IsParam { get { return this.varType == VariableType.LocalParameter || this.varType == VariableType.GlobalParameter; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Diagnostics; using System.Xml.XPath; namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; internal enum VariableType { GlobalVariable, GlobalParameter, LocalVariable, LocalParameter, WithParameter, } internal class VariableAction : ContainerAction, IXsltContextVariable { public static object BeingComputedMark = new object(); private const int ValueCalculated = 2; protected XmlQualifiedName name; protected string nameStr; protected string baseUri; protected int selectKey = Compiler.InvalidQueryKey; protected int stylesheetid; protected VariableType varType; private int varKey; internal int Stylesheetid { get { return this.stylesheetid; } } internal XmlQualifiedName Name { get { return this.name; } } internal string NameStr { get { return this.nameStr; } } internal VariableType VarType { get { return this.varType; } } internal int VarKey { get { return this.varKey; } } internal bool IsGlobal { get { return this.varType == VariableType.GlobalVariable || this.varType == VariableType.GlobalParameter; } } internal VariableAction(VariableType type) { this.varType = type; } internal override void Compile(Compiler compiler) { this.stylesheetid = compiler.Stylesheetid; this.baseUri = compiler.Input.BaseURI; CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.name, "name"); if (compiler.Recurse()) { CompileTemplate(compiler); compiler.ToParent(); if (this.selectKey != Compiler.InvalidQueryKey && this.containedActions != null) { throw XsltException.Create(Res.Xslt_VariableCntSel2, this.nameStr); } } if (this.containedActions != null) { baseUri = baseUri + '#' + compiler.GetUnicRtfId(); } else { baseUri = null; } this.varKey = compiler.InsertVariable(this); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Ref.Equal(name, compiler.Atoms.Name)) { Debug.Assert(this.name == null && this.nameStr == null); this.nameStr = value; this.name = compiler.CreateXPathQName(this.nameStr); } else if (Ref.Equal(name, compiler.Atoms.Select)) { this.selectKey = compiler.AddQuery(value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null && frame.State != ValueCalculated); object value = null; switch(frame.State) { case Initialized: if (IsGlobal) { if (frame.GetVariable(this.varKey) != null) { // This var was calculated already frame.Finished(); break; } // Mark that the variable is being computed to check for circular references frame.SetVariable(this.varKey, BeingComputedMark); } // If this is a parameter, check whether the caller has passed the value if (this.varType == VariableType.GlobalParameter) { value = processor.GetGlobalParameter(this.name); } else if (this.varType == VariableType.LocalParameter) { value = processor.GetParameter(this.name); } if (value != null) { goto case ValueCalculated; } // If value was not passed, check the 'select' attribute if (this.selectKey != Compiler.InvalidQueryKey) { value = processor.RunQuery(frame, this.selectKey); goto case ValueCalculated; } // If there is no 'select' attribute and the content is empty, use the empty string if (this.containedActions == null) { value = string.Empty; goto case ValueCalculated; } // RTF case NavigatorOutput output = new NavigatorOutput(this.baseUri); processor.PushOutput(output); processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; case ProcessingChildren: RecordOutput recOutput = processor.PopOutput(); Debug.Assert(recOutput is NavigatorOutput); value = ((NavigatorOutput)recOutput).Navigator; goto case ValueCalculated; case ValueCalculated: Debug.Assert(value != null); frame.SetVariable(this.varKey, value); frame.Finished(); break; default: Debug.Fail("Invalid execution state inside VariableAction.Execute"); break; } } // ---------------------- IXsltContextVariable -------------------- XPathResultType IXsltContextVariable.VariableType { get { return XPathResultType.Any; } } object IXsltContextVariable.Evaluate(XsltContext xsltContext) { return ((XsltCompileContext)xsltContext).EvaluateVariable(this); } bool IXsltContextVariable.IsLocal { get { return this.varType == VariableType.LocalVariable || this.varType == VariableType.LocalParameter; } } bool IXsltContextVariable.IsParam { get { return this.varType == VariableType.LocalParameter || this.varType == VariableType.GlobalParameter; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
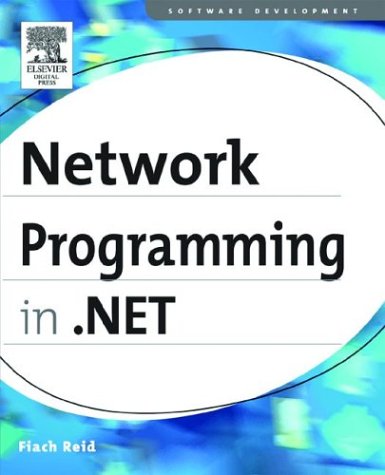
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _AcceptOverlappedAsyncResult.cs
- ReliableOutputSessionChannel.cs
- XmlnsCache.cs
- DependencyProperty.cs
- XmlSchemaAttributeGroup.cs
- HostedHttpTransportManager.cs
- SqlServices.cs
- RegexNode.cs
- DecimalFormatter.cs
- XmlSerializerNamespaces.cs
- DecimalStorage.cs
- FontStyleConverter.cs
- AddingNewEventArgs.cs
- Crypto.cs
- Button.cs
- WmiEventSink.cs
- DesignerDataSchemaClass.cs
- IndependentlyAnimatedPropertyMetadata.cs
- ListViewInsertedEventArgs.cs
- Scene3D.cs
- HtmlInputFile.cs
- DataBindingHandlerAttribute.cs
- CopyCodeAction.cs
- DefaultValueAttribute.cs
- CompressionTracing.cs
- SignedPkcs7.cs
- XmlEventCache.cs
- XmlSecureResolver.cs
- DoubleLinkList.cs
- PriorityItem.cs
- ProcessHostConfigUtils.cs
- XmlDataCollection.cs
- MultiSelectRootGridEntry.cs
- StorageModelBuildProvider.cs
- _ListenerResponseStream.cs
- WebDescriptionAttribute.cs
- TokenBasedSet.cs
- PackageProperties.cs
- EntityContainerEntitySet.cs
- CustomExpressionEventArgs.cs
- Enlistment.cs
- Sorting.cs
- EFColumnProvider.cs
- PackageDigitalSignature.cs
- DSASignatureFormatter.cs
- Variable.cs
- ConditionalAttribute.cs
- Panel.cs
- ScriptingSectionGroup.cs
- EntityTypeEmitter.cs
- ConversionValidationRule.cs
- TreeNodeConverter.cs
- CompilerError.cs
- DesignerAutoFormatCollection.cs
- MissingSatelliteAssemblyException.cs
- PrintDialog.cs
- EntityKeyElement.cs
- templategroup.cs
- InvalidDataException.cs
- DatatypeImplementation.cs
- ColorConverter.cs
- IProvider.cs
- EventLogPermission.cs
- XmlDataDocument.cs
- DbConnectionPoolGroupProviderInfo.cs
- QueryHandler.cs
- TemplatePartAttribute.cs
- ObjectConverter.cs
- ObjectStateEntry.cs
- HotSpot.cs
- DataGridBoundColumn.cs
- sortedlist.cs
- X509Extension.cs
- BamlLocalizabilityResolver.cs
- PathStreamGeometryContext.cs
- SetterBaseCollection.cs
- AnnotationComponentChooser.cs
- _TimerThread.cs
- ExpandoClass.cs
- FontSource.cs
- FormatException.cs
- XPathMultyIterator.cs
- RegexCompilationInfo.cs
- EventLogPermission.cs
- ExpandCollapseProviderWrapper.cs
- Delay.cs
- ReadOnlyAttribute.cs
- XLinq.cs
- TextRangeBase.cs
- DecimalAnimation.cs
- LinkDescriptor.cs
- WebPartVerbsEventArgs.cs
- DummyDataSource.cs
- OutputCacheProfileCollection.cs
- FormsAuthenticationConfiguration.cs
- XmlIncludeAttribute.cs
- Annotation.cs
- ActivityCodeDomSerializationManager.cs
- CryptoConfig.cs
- EntityModelBuildProvider.cs