Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / EntityKeyElement.cs / 2 / EntityKeyElement.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an Key element in an EntityType element. /// internal sealed class EntityKeyElement : SchemaElement { private List_keyProperties; /// /// Constructs an EntityContainerAssociationSetEnd /// /// Reference to the schema element. public EntityKeyElement( SchemaEntityType parentElement ) : base( parentElement ) { } public IListKeyProperties { get { if (_keyProperties == null) { _keyProperties = new List (); } return _keyProperties; } } protected override bool HandleAttribute(XmlReader reader) { return false; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.PropertyRef)) { HandlePropertyRefElement(reader); return true; } return false; } /// /// /// /// private void HandlePropertyRefElement(XmlReader reader) { PropertyRefElement property = new PropertyRefElement((SchemaEntityType)ParentElement); property.Parse(reader); this.KeyProperties.Add(property); } ////// Used during the resolve phase to resolve the type name to the object that represents that type /// internal override void ResolveTopLevelNames() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); foreach (PropertyRefElement property in _keyProperties) { if (!property.ResolveNames((SchemaEntityType)this.ParentElement)) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNoProperty(this.ParentElement.FQName, property.Name)); } } } ////// Validate all the key properties /// internal override void Validate() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); DictionarypropertyLookUp = new Dictionary (StringComparer.Ordinal); foreach (PropertyRefElement keyProperty in _keyProperties) { StructuredProperty property = keyProperty.Property; Debug.Assert(property != null, "This should never be null, since if we were not able to resolve, we should have never reached to this point"); if (propertyLookUp.ContainsKey(property.Name)) { AddError(ErrorCode.DuplicatePropertySpecifiedInEntityKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.DuplicatePropertyNameSpecifiedInEntityKey(this.ParentElement.FQName, property.Name)); continue; } propertyLookUp.Add(property.Name, keyProperty); if (property.Nullable) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNullablePart(property.Name, this.ParentElement.Name)); } // currently we only support key properties of scalar type if ((!(property.Type is ScalarType)) || (property.CollectionKind != CollectionKind.None)) { AddError(ErrorCode.EntityKeyMustBeScalar, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EntityKeyMustBeScalar(property.Name, this.ParentElement.Name)); continue; } Debug.Assert(property.TypeUsage != null, "For scalar type, typeusage must be initialized"); // Bug 484200: Binary type key properties are currently not supported. PrimitiveTypeKind kind = ((PrimitiveType)property.TypeUsage.EdmType).PrimitiveTypeKind; if (!Helper.IsValidKeyType(kind)) { if (Schema.DataModel == SchemaDataModelOption.EntityDataModel) { AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupported(property.Name, this.ParentElement.FQName, kind)); } else { Debug.Assert(SchemaDataModelOption.ProviderDataModel == Schema.DataModel, "Invalid DataModel encountered"); AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupportedInSSDL(property.Name, this.ParentElement.FQName, property.TypeUsage.EdmType.Name, property.TypeUsage.EdmType.BaseType.FullName, kind)); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an Key element in an EntityType element. /// internal sealed class EntityKeyElement : SchemaElement { private List_keyProperties; /// /// Constructs an EntityContainerAssociationSetEnd /// /// Reference to the schema element. public EntityKeyElement( SchemaEntityType parentElement ) : base( parentElement ) { } public IListKeyProperties { get { if (_keyProperties == null) { _keyProperties = new List (); } return _keyProperties; } } protected override bool HandleAttribute(XmlReader reader) { return false; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.PropertyRef)) { HandlePropertyRefElement(reader); return true; } return false; } /// /// /// /// private void HandlePropertyRefElement(XmlReader reader) { PropertyRefElement property = new PropertyRefElement((SchemaEntityType)ParentElement); property.Parse(reader); this.KeyProperties.Add(property); } ////// Used during the resolve phase to resolve the type name to the object that represents that type /// internal override void ResolveTopLevelNames() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); foreach (PropertyRefElement property in _keyProperties) { if (!property.ResolveNames((SchemaEntityType)this.ParentElement)) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNoProperty(this.ParentElement.FQName, property.Name)); } } } ////// Validate all the key properties /// internal override void Validate() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); DictionarypropertyLookUp = new Dictionary (StringComparer.Ordinal); foreach (PropertyRefElement keyProperty in _keyProperties) { StructuredProperty property = keyProperty.Property; Debug.Assert(property != null, "This should never be null, since if we were not able to resolve, we should have never reached to this point"); if (propertyLookUp.ContainsKey(property.Name)) { AddError(ErrorCode.DuplicatePropertySpecifiedInEntityKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.DuplicatePropertyNameSpecifiedInEntityKey(this.ParentElement.FQName, property.Name)); continue; } propertyLookUp.Add(property.Name, keyProperty); if (property.Nullable) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNullablePart(property.Name, this.ParentElement.Name)); } // currently we only support key properties of scalar type if ((!(property.Type is ScalarType)) || (property.CollectionKind != CollectionKind.None)) { AddError(ErrorCode.EntityKeyMustBeScalar, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EntityKeyMustBeScalar(property.Name, this.ParentElement.Name)); continue; } Debug.Assert(property.TypeUsage != null, "For scalar type, typeusage must be initialized"); // Bug 484200: Binary type key properties are currently not supported. PrimitiveTypeKind kind = ((PrimitiveType)property.TypeUsage.EdmType).PrimitiveTypeKind; if (!Helper.IsValidKeyType(kind)) { if (Schema.DataModel == SchemaDataModelOption.EntityDataModel) { AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupported(property.Name, this.ParentElement.FQName, kind)); } else { Debug.Assert(SchemaDataModelOption.ProviderDataModel == Schema.DataModel, "Invalid DataModel encountered"); AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupportedInSSDL(property.Name, this.ParentElement.FQName, property.TypeUsage.EdmType.Name, property.TypeUsage.EdmType.BaseType.FullName, kind)); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
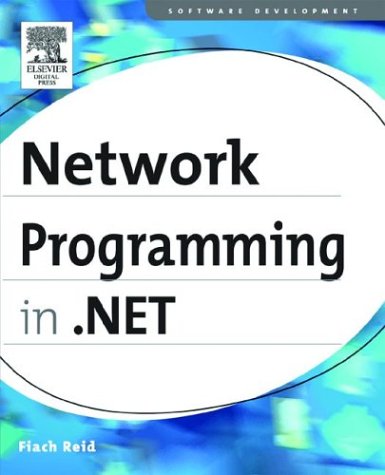
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutputCacheProfile.cs
- CommunicationObjectFaultedException.cs
- ComponentConverter.cs
- DataGridViewSelectedRowCollection.cs
- ComNativeDescriptor.cs
- SemaphoreSlim.cs
- BuilderPropertyEntry.cs
- ToolStripPanelRenderEventArgs.cs
- SoapInteropTypes.cs
- PointKeyFrameCollection.cs
- DirectoryGroupQuery.cs
- GenerateTemporaryAssemblyTask.cs
- DtdParser.cs
- IntSecurity.cs
- DataGridView.cs
- XmlEnumAttribute.cs
- PackUriHelper.cs
- HideDisabledControlAdapter.cs
- ReadContentAsBinaryHelper.cs
- ConsumerConnectionPoint.cs
- DiscoveryDocumentSearchPattern.cs
- odbcmetadatafactory.cs
- RuleInfoComparer.cs
- XmlWrappingReader.cs
- GenericEnumerator.cs
- SafeNativeMethods.cs
- ContextMarshalException.cs
- EventProperty.cs
- WmlPhoneCallAdapter.cs
- BrowserCapabilitiesFactoryBase.cs
- PropertiesTab.cs
- CurrentChangingEventArgs.cs
- DeviceSpecificDialogCachedState.cs
- ColorTranslator.cs
- Statements.cs
- DataKey.cs
- SynchronizedDispatch.cs
- SafeWaitHandle.cs
- KnownTypes.cs
- ConnectionConsumerAttribute.cs
- OperationInvokerTrace.cs
- CodeParameterDeclarationExpressionCollection.cs
- ObjectAnimationUsingKeyFrames.cs
- PersonalizationProvider.cs
- DateTimeOffset.cs
- SourceFileInfo.cs
- SerializationObjectManager.cs
- TextSelectionProcessor.cs
- CompressedStack.cs
- TextProperties.cs
- ImageAnimator.cs
- CheckoutException.cs
- QilParameter.cs
- Hex.cs
- FileDataSourceCache.cs
- RectAnimationUsingKeyFrames.cs
- ReadOnlyAttribute.cs
- FunctionQuery.cs
- RegexParser.cs
- TransformConverter.cs
- SecurityTokenTypes.cs
- CodeStatementCollection.cs
- GridViewRow.cs
- StorageRoot.cs
- SrgsElement.cs
- HttpClientChannel.cs
- UiaCoreApi.cs
- EntityViewGenerationConstants.cs
- CryptoStream.cs
- PathTooLongException.cs
- Label.cs
- ButtonBaseAdapter.cs
- SHA256.cs
- UnknownWrapper.cs
- XPathSelfQuery.cs
- StylusPointProperties.cs
- DiagnosticsConfigurationHandler.cs
- HttpCookieCollection.cs
- ObjectListShowCommandsEventArgs.cs
- WsatServiceAddress.cs
- TypeContext.cs
- Vector3D.cs
- SecurityChannelFactory.cs
- InlinedLocationReference.cs
- GrammarBuilder.cs
- LineServicesCallbacks.cs
- HuffmanTree.cs
- TypefaceCollection.cs
- OrderedHashRepartitionStream.cs
- ComplexBindingPropertiesAttribute.cs
- SecurityTokenRequirement.cs
- DataBoundLiteralControl.cs
- ValidatorUtils.cs
- keycontainerpermission.cs
- CookieHandler.cs
- DefaultTraceListener.cs
- CapabilitiesPattern.cs
- InstallerTypeAttribute.cs
- ReadOnlyDataSourceView.cs
- ProcessHostFactoryHelper.cs