Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / ProcessHostConfigUtils.cs / 2 / ProcessHostConfigUtils.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Xml; using System.Security; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; using System.Runtime.ConstrainedExecution; // // Uses IIS 7 native config // internal static class ProcessHostConfigUtils { internal const uint DEFAULT_SITE_ID_UINT = 1; internal const string DEFAULT_SITE_ID_STRING = "1"; private static string s_defaultSiteName; private static int s_InitedExternalConfig; private static NativeConfigWrapper _configWrapper; // static class ctor static ProcessHostConfigUtils() { HttpRuntime.ForceStaticInit(); } internal static void InitStandaloneConfig() { if (!HostingEnvironment.IsUnderIISProcess) { if (!ServerConfig.UseMetabase) { int inited= Interlocked.Exchange(ref s_InitedExternalConfig, 1); // only do this once if (0 == inited) { _configWrapper = new NativeConfigWrapper(); } } } } internal static string MapPathActual(string siteName, VirtualPath path) { string physicalPath = null; IntPtr pBstr = IntPtr.Zero; int cBstr = 0; try { int result = UnsafeIISMethods.MgdMapPathDirect(siteName, path.VirtualPathString, out pBstr, out cBstr); if (result < 0) { throw new InvalidOperationException(SR.GetString(SR.Cannot_map_path, path.VirtualPathString)); } physicalPath = (pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : null; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } return physicalPath; } internal static string GetSiteNameFromId(uint siteId) { if ( siteId == DEFAULT_SITE_ID_UINT && s_defaultSiteName != null) { return s_defaultSiteName; } IntPtr pBstr = IntPtr.Zero; int cBstr = 0; string siteName = null; try { int result = UnsafeIISMethods.MgdGetSiteNameFromId(siteId, out pBstr, out cBstr); siteName = (result == 0 && pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : String.Empty; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } if ( siteId == DEFAULT_SITE_ID_UINT) { s_defaultSiteName = siteName; } return siteName; } private class NativeConfigWrapper : CriticalFinalizerObject { internal NativeConfigWrapper() { int result = UnsafeIISMethods.MgdInitNativeConfig(); if (result < 0) { s_InitedExternalConfig = 0; throw new InvalidOperationException(SR.GetString(SR.Cant_Init_Native_Config, result.ToString("X8", CultureInfo.InvariantCulture))); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] ~NativeConfigWrapper() { UnsafeIISMethods.MgdTerminateNativeConfig(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Xml; using System.Security; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; using System.Runtime.ConstrainedExecution; // // Uses IIS 7 native config // internal static class ProcessHostConfigUtils { internal const uint DEFAULT_SITE_ID_UINT = 1; internal const string DEFAULT_SITE_ID_STRING = "1"; private static string s_defaultSiteName; private static int s_InitedExternalConfig; private static NativeConfigWrapper _configWrapper; // static class ctor static ProcessHostConfigUtils() { HttpRuntime.ForceStaticInit(); } internal static void InitStandaloneConfig() { if (!HostingEnvironment.IsUnderIISProcess) { if (!ServerConfig.UseMetabase) { int inited= Interlocked.Exchange(ref s_InitedExternalConfig, 1); // only do this once if (0 == inited) { _configWrapper = new NativeConfigWrapper(); } } } } internal static string MapPathActual(string siteName, VirtualPath path) { string physicalPath = null; IntPtr pBstr = IntPtr.Zero; int cBstr = 0; try { int result = UnsafeIISMethods.MgdMapPathDirect(siteName, path.VirtualPathString, out pBstr, out cBstr); if (result < 0) { throw new InvalidOperationException(SR.GetString(SR.Cannot_map_path, path.VirtualPathString)); } physicalPath = (pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : null; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } return physicalPath; } internal static string GetSiteNameFromId(uint siteId) { if ( siteId == DEFAULT_SITE_ID_UINT && s_defaultSiteName != null) { return s_defaultSiteName; } IntPtr pBstr = IntPtr.Zero; int cBstr = 0; string siteName = null; try { int result = UnsafeIISMethods.MgdGetSiteNameFromId(siteId, out pBstr, out cBstr); siteName = (result == 0 && pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : String.Empty; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } if ( siteId == DEFAULT_SITE_ID_UINT) { s_defaultSiteName = siteName; } return siteName; } private class NativeConfigWrapper : CriticalFinalizerObject { internal NativeConfigWrapper() { int result = UnsafeIISMethods.MgdInitNativeConfig(); if (result < 0) { s_InitedExternalConfig = 0; throw new InvalidOperationException(SR.GetString(SR.Cant_Init_Native_Config, result.ToString("X8", CultureInfo.InvariantCulture))); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] ~NativeConfigWrapper() { UnsafeIISMethods.MgdTerminateNativeConfig(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
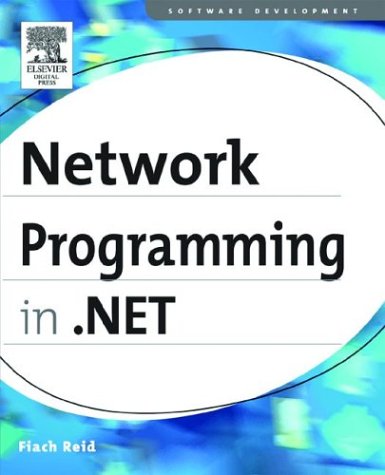
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsltContext.cs
- VisualStyleTypesAndProperties.cs
- SafeEventLogWriteHandle.cs
- GlobalEventManager.cs
- ThemeableAttribute.cs
- GeometryConverter.cs
- PhysicalOps.cs
- brushes.cs
- HtmlTableCellCollection.cs
- DbDataRecord.cs
- SmiMetaData.cs
- ContentType.cs
- OleDbParameter.cs
- GridViewColumnHeader.cs
- ChannelDispatcherCollection.cs
- WebPartActionVerb.cs
- WhileDesigner.cs
- securitycriticaldata.cs
- EmptyTextWriter.cs
- XmlLinkedNode.cs
- NavigateEvent.cs
- GlyphRun.cs
- SiteMap.cs
- initElementDictionary.cs
- ToolStripPanel.cs
- FocusWithinProperty.cs
- SecurityElement.cs
- FixedSchema.cs
- UniqueEventHelper.cs
- basecomparevalidator.cs
- SingleAnimationBase.cs
- QueryConverter.cs
- ObjectListShowCommandsEventArgs.cs
- FlowDocumentPageViewerAutomationPeer.cs
- ButtonAutomationPeer.cs
- WebConfigurationManager.cs
- XmlLangPropertyAttribute.cs
- ProjectionPruner.cs
- ScriptManagerProxy.cs
- ReturnEventArgs.cs
- DrawingGroup.cs
- ReadOnlyAttribute.cs
- InvokeMemberBinder.cs
- XhtmlBasicSelectionListAdapter.cs
- RuntimeConfigLKG.cs
- DecimalAnimation.cs
- DrawingCollection.cs
- CircleEase.cs
- ZipIOLocalFileDataDescriptor.cs
- HttpListenerRequestUriBuilder.cs
- BamlTreeUpdater.cs
- JavaScriptString.cs
- ComplexTypeEmitter.cs
- DataServiceHost.cs
- HttpResponseHeader.cs
- Debug.cs
- VirtualPath.cs
- dataprotectionpermission.cs
- XamlStream.cs
- ServicesUtilities.cs
- _WinHttpWebProxyDataBuilder.cs
- MonthCalendarDesigner.cs
- RemoteWebConfigurationHost.cs
- DefaultProxySection.cs
- RegexCompilationInfo.cs
- ViewStateException.cs
- ConditionCollection.cs
- DurationConverter.cs
- ScrollViewerAutomationPeer.cs
- versioninfo.cs
- ToolStripSettings.cs
- CategoryAttribute.cs
- MiniLockedBorderGlyph.cs
- MediaContext.cs
- SystemIcons.cs
- SkewTransform.cs
- ContentPropertyAttribute.cs
- WebConfigurationManager.cs
- HostingPreferredMapPath.cs
- SplayTreeNode.cs
- coordinatorscratchpad.cs
- ComponentSerializationService.cs
- EnumValAlphaComparer.cs
- TypeInfo.cs
- AssertSection.cs
- ProfilePropertySettings.cs
- ImageAnimator.cs
- Utility.cs
- ClassImporter.cs
- Boolean.cs
- CheckoutException.cs
- NameValuePair.cs
- Int32AnimationUsingKeyFrames.cs
- FillRuleValidation.cs
- GeometryDrawing.cs
- TableRowCollection.cs
- XPathItem.cs
- DocumentViewerBaseAutomationPeer.cs
- StyleBamlRecordReader.cs
- Helpers.cs