Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / DefaultValueAttribute.cs / 1 / DefaultValueAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1019:DefineAccessorsForAttributeArguments")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1813:AvoidUnsealedAttributes")] [AttributeUsage(AttributeTargets.All)] public class DefaultValueAttribute : Attribute { ///Specifies the default value for a property. ////// This is the default value. /// private object value; ////// public DefaultValueAttribute(Type type, string value) { // The try/catch here is because attributes should never throw exceptions. We would fail to // load an otherwise normal class. try { this.value = TypeDescriptor.GetConverter(type).ConvertFromInvariantString(value); } catch { Debug.Fail("Default value attribute of type " + type.FullName + " threw converting from the string '" + value + "'."); } } ///Initializes a new instance of the ///class, converting the /// specified value to the /// specified type, and using the U.S. English culture as the /// translation /// context. /// public DefaultValueAttribute(char value) { this.value = value; } ///Initializes a new instance of the ///class using a Unicode /// character. /// public DefaultValueAttribute(byte value) { this.value = value; } ///Initializes a new instance of the ///class using an 8-bit unsigned /// integer. /// public DefaultValueAttribute(short value) { this.value = value; } ///Initializes a new instance of the ///class using a 16-bit signed /// integer. /// public DefaultValueAttribute(int value) { this.value = value; } ///Initializes a new instance of the ///class using a 32-bit signed /// integer. /// public DefaultValueAttribute(long value) { this.value = value; } ///Initializes a new instance of the ///class using a 64-bit signed /// integer. /// public DefaultValueAttribute(float value) { this.value = value; } ///Initializes a new instance of the ///class using a /// single-precision floating point /// number. /// public DefaultValueAttribute(double value) { this.value = value; } ///Initializes a new instance of the ///class using a /// double-precision floating point /// number. /// public DefaultValueAttribute(bool value) { this.value = value; } ///Initializes a new instance of the ///class using a /// value. /// public DefaultValueAttribute(string value) { this.value = value; } ///Initializes a new instance of the ///class using a . /// public DefaultValueAttribute(object value) { this.value = value; } ///Initializes a new instance of the ////// class. /// public virtual object Value { get { return value; } } public override bool Equals(object obj) { if (obj == this) { return true; } DefaultValueAttribute other = obj as DefaultValueAttribute; if (other != null) { if (Value != null) { return Value.Equals(other.Value); } else { return (other.Value == null); } } return false; } public override int GetHashCode() { return base.GetHashCode(); } protected void SetValue(object value) { this.value = value; } } }/// Gets the default value of the property this /// attribute is /// bound to. /// ///
Link Menu
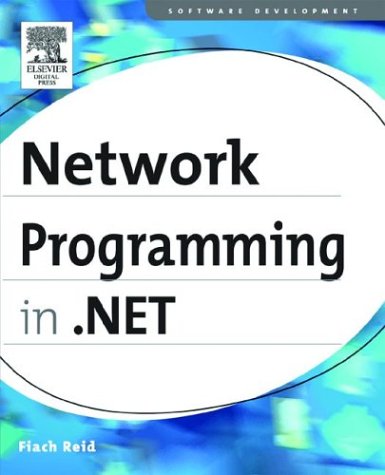
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColumnResizeAdorner.cs
- SHA256Managed.cs
- AssociationSetMetadata.cs
- UserThread.cs
- InvalidEnumArgumentException.cs
- ByteAnimationBase.cs
- TextInfo.cs
- WindowPatternIdentifiers.cs
- ToolStripDropDownClosingEventArgs.cs
- JsonServiceDocumentSerializer.cs
- XmlText.cs
- RealProxy.cs
- VisualTarget.cs
- ContentPresenter.cs
- GlyphingCache.cs
- DbConnectionPoolGroup.cs
- LogLogRecordEnumerator.cs
- StaticTextPointer.cs
- FormsAuthenticationModule.cs
- ToolTip.cs
- PEFileEvidenceFactory.cs
- SettingsPropertyValue.cs
- XmlEntityReference.cs
- CompModSwitches.cs
- ExceptionRoutedEventArgs.cs
- TypeInfo.cs
- ByteStack.cs
- NamespaceInfo.cs
- CommandArguments.cs
- ViewService.cs
- ChannelServices.cs
- Section.cs
- SectionRecord.cs
- OverflowException.cs
- ExpressionBuilder.cs
- ISCIIEncoding.cs
- ReverseQueryOperator.cs
- RTLAwareMessageBox.cs
- CustomPopupPlacement.cs
- OdbcUtils.cs
- NameObjectCollectionBase.cs
- SqlPersonalizationProvider.cs
- CookieProtection.cs
- ProfileGroupSettings.cs
- HttpContext.cs
- ComponentManagerBroker.cs
- FormsAuthenticationTicket.cs
- AssemblyAttributes.cs
- Light.cs
- Binding.cs
- DesignerSelectionListAdapter.cs
- ReceiveMessageContent.cs
- SyncMethodInvoker.cs
- ClientApiGenerator.cs
- LoginAutoFormat.cs
- LayoutEngine.cs
- NetWebProxyFinder.cs
- RowUpdatedEventArgs.cs
- EngineSiteSapi.cs
- AllMembershipCondition.cs
- StateRuntime.cs
- SystemIPInterfaceProperties.cs
- Process.cs
- MemberProjectionIndex.cs
- AudioDeviceOut.cs
- securestring.cs
- QueryContinueDragEventArgs.cs
- designeractionlistschangedeventargs.cs
- KnownTypeAttribute.cs
- OdbcConnectionHandle.cs
- NonParentingControl.cs
- FormViewDeletedEventArgs.cs
- Size3D.cs
- RequestQueue.cs
- NameValueFileSectionHandler.cs
- CapacityStreamGeometryContext.cs
- CapabilitiesAssignment.cs
- TextBoxAutoCompleteSourceConverter.cs
- SystemFonts.cs
- SubclassTypeValidatorAttribute.cs
- TableLayoutStyle.cs
- CheckedPointers.cs
- ISAPIApplicationHost.cs
- GlyphRunDrawing.cs
- wgx_render.cs
- SemanticBasicElement.cs
- InputLanguageManager.cs
- NetCodeGroup.cs
- SelectionRange.cs
- Frame.cs
- HttpServerVarsCollection.cs
- VisualBrush.cs
- DelegateHelpers.Generated.cs
- Table.cs
- WebZone.cs
- StoreItemCollection.Loader.cs
- PresentationSource.cs
- MarshalByRefObject.cs
- CodeTypeConstructor.cs
- LayoutExceptionEventArgs.cs