Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / DocumentStructures / SemanticBasicElement.cs / 1 / SemanticBasicElement.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // History: // 05/12/2005 : mingliu - created. // // //--------------------------------------------------------------------------- using MS.Internal.Documents; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Reflection; using System.Security.Permissions; using System.Windows.Controls.Primitives; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Shapes; using System.Windows.Markup; [assembly: XmlnsDefinition( "http://schemas.microsoft.com/xps/2005/06/documentstructure", "System.Windows.Documents.DocumentStructures")] namespace System.Windows.Documents.DocumentStructures { ////// /// public class SemanticBasicElement : BlockElement { ////// /// internal SemanticBasicElement() { _elementList = new List(); } internal List BlockElementList { get { return _elementList; } } /// /// /// internal List_elementList; } /// /// /// public class SectionStructure : SemanticBasicElement, IAddChildInternal { ////// /// public SectionStructure() { _elementType = FixedElement.ElementType.Section; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is FigureStructure || value is ListStructure || value is TableStructure ) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(FigureStructure), typeof(ListStructure), typeof(TableStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ParagraphStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ParagraphStructure() { _elementType = FixedElement.ElementType.Paragraph; } void IAddChild.AddChild(object value) { if (value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType1, value.GetType(), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { } } //We are keeping these classes around for V.Next #if DEBUG ////// /// internal class Inline : SemanticBasicElement { ////// /// public Inline() { _elementType = FixedElement.ElementType.Inline; } } ////// /// internal class Span : Inline, IAddChild { ////// /// public Span() { _elementType = FixedElement.ElementType.Span; } void IAddChild.AddChild(object value) { if (value is Inline || value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(ParagraphStructure), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { //throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, typeof(string)), "text"); } } ////// /// internal class Run : Inline, IAddChild { ////// /// public Run() { _elementType = FixedElement.ElementType.Run; } void IAddChild.AddChild(object value) { throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType()), "value"); } void IAddChild.AddText(string text) { } } #endif ////// /// public class FigureStructure : SemanticBasicElement, IAddChildInternal { ////// /// public FigureStructure() { _elementType = FixedElement.ElementType.Figure; } void IAddChild.AddChild(object value) { if (value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ListStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ListStructure() { _elementType = FixedElement.ElementType.List; } void IAddChild.AddChild(object value) { if (value is ListItemStructure) { _elementList.Add((ListItemStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(ListItemStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ListItemStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ListItemStructure() { _elementType = FixedElement.ElementType.ListItem; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is TableStructure || value is ListStructure || value is FigureStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(TableStructure), typeof(ListStructure), typeof(FigureStructure)), "value"); } void IAddChild.AddText(string text) { } ////// /// public String Marker { get { return _markerName; } set { _markerName = value; } } private String _markerName; } ////// /// public class TableStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableStructure() { _elementType = FixedElement.ElementType.Table; } void IAddChild.AddChild(object value) { if (value is TableRowGroupStructure) { _elementList.Add((TableRowGroupStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRowGroupStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableRowGroupStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableRowGroupStructure() { _elementType = FixedElement.ElementType.TableRowGroup; } void IAddChild.AddChild(object value) { if (value is TableRowStructure) { _elementList.Add((TableRowStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRowStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableRowStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableRowStructure() { _elementType = FixedElement.ElementType.TableRow; } void IAddChild.AddChild(object value) { if (value is TableCellStructure) { _elementList.Add((TableCellStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableCellStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableCellStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableCellStructure() { _elementType = FixedElement.ElementType.TableCell; _rowSpan = 1; _columnSpan = 1; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is TableStructure || value is ListStructure || value is FigureStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(TableStructure), typeof(ListStructure), typeof(FigureStructure)), "value"); } void IAddChild.AddText(string text) { } ////// /// public int RowSpan { get { return _rowSpan; } set {_rowSpan = value; } } ////// /// public int ColumnSpan { get { return _columnSpan; } set {_columnSpan = value; } } private int _rowSpan; private int _columnSpan; } //We are keeping these classes for V.Next #if DEBUG ////// Header will not participate in the selection fow. /// internal class Header : SemanticBasicElement, IAddChild { ////// /// public Header() { _elementType = FixedElement.ElementType.Header; } void IAddChild.AddChild(object value) { if (value is NamedElement || value is ParagraphStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(NamedElement), typeof(ParagraphStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// Footer will not participate in the selection fow. /// internal class Footer : SemanticBasicElement, IAddChild { ////// /// public Footer() { _elementType = FixedElement.ElementType.Footer; } void IAddChild.AddChild(object value) { if (value is NamedElement || value is ParagraphStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(NamedElement), typeof(ParagraphStructure)), "value"); } void IAddChild.AddText(string text) { } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // History: // 05/12/2005 : mingliu - created. // // //--------------------------------------------------------------------------- using MS.Internal.Documents; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Reflection; using System.Security.Permissions; using System.Windows.Controls.Primitives; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Shapes; using System.Windows.Markup; [assembly: XmlnsDefinition( "http://schemas.microsoft.com/xps/2005/06/documentstructure", "System.Windows.Documents.DocumentStructures")] namespace System.Windows.Documents.DocumentStructures { ////// /// public class SemanticBasicElement : BlockElement { ////// /// internal SemanticBasicElement() { _elementList = new List(); } internal List BlockElementList { get { return _elementList; } } /// /// /// internal List_elementList; } /// /// /// public class SectionStructure : SemanticBasicElement, IAddChildInternal { ////// /// public SectionStructure() { _elementType = FixedElement.ElementType.Section; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is FigureStructure || value is ListStructure || value is TableStructure ) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(FigureStructure), typeof(ListStructure), typeof(TableStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ParagraphStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ParagraphStructure() { _elementType = FixedElement.ElementType.Paragraph; } void IAddChild.AddChild(object value) { if (value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType1, value.GetType(), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { } } //We are keeping these classes around for V.Next #if DEBUG ////// /// internal class Inline : SemanticBasicElement { ////// /// public Inline() { _elementType = FixedElement.ElementType.Inline; } } ////// /// internal class Span : Inline, IAddChild { ////// /// public Span() { _elementType = FixedElement.ElementType.Span; } void IAddChild.AddChild(object value) { if (value is Inline || value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(ParagraphStructure), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { //throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, typeof(string)), "text"); } } ////// /// internal class Run : Inline, IAddChild { ////// /// public Run() { _elementType = FixedElement.ElementType.Run; } void IAddChild.AddChild(object value) { throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType()), "value"); } void IAddChild.AddText(string text) { } } #endif ////// /// public class FigureStructure : SemanticBasicElement, IAddChildInternal { ////// /// public FigureStructure() { _elementType = FixedElement.ElementType.Figure; } void IAddChild.AddChild(object value) { if (value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ListStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ListStructure() { _elementType = FixedElement.ElementType.List; } void IAddChild.AddChild(object value) { if (value is ListItemStructure) { _elementList.Add((ListItemStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(ListItemStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ListItemStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ListItemStructure() { _elementType = FixedElement.ElementType.ListItem; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is TableStructure || value is ListStructure || value is FigureStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(TableStructure), typeof(ListStructure), typeof(FigureStructure)), "value"); } void IAddChild.AddText(string text) { } ////// /// public String Marker { get { return _markerName; } set { _markerName = value; } } private String _markerName; } ////// /// public class TableStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableStructure() { _elementType = FixedElement.ElementType.Table; } void IAddChild.AddChild(object value) { if (value is TableRowGroupStructure) { _elementList.Add((TableRowGroupStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRowGroupStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableRowGroupStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableRowGroupStructure() { _elementType = FixedElement.ElementType.TableRowGroup; } void IAddChild.AddChild(object value) { if (value is TableRowStructure) { _elementList.Add((TableRowStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRowStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableRowStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableRowStructure() { _elementType = FixedElement.ElementType.TableRow; } void IAddChild.AddChild(object value) { if (value is TableCellStructure) { _elementList.Add((TableCellStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableCellStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableCellStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableCellStructure() { _elementType = FixedElement.ElementType.TableCell; _rowSpan = 1; _columnSpan = 1; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is TableStructure || value is ListStructure || value is FigureStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(TableStructure), typeof(ListStructure), typeof(FigureStructure)), "value"); } void IAddChild.AddText(string text) { } ////// /// public int RowSpan { get { return _rowSpan; } set {_rowSpan = value; } } ////// /// public int ColumnSpan { get { return _columnSpan; } set {_columnSpan = value; } } private int _rowSpan; private int _columnSpan; } //We are keeping these classes for V.Next #if DEBUG ////// Header will not participate in the selection fow. /// internal class Header : SemanticBasicElement, IAddChild { ////// /// public Header() { _elementType = FixedElement.ElementType.Header; } void IAddChild.AddChild(object value) { if (value is NamedElement || value is ParagraphStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(NamedElement), typeof(ParagraphStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// Footer will not participate in the selection fow. /// internal class Footer : SemanticBasicElement, IAddChild { ////// /// public Footer() { _elementType = FixedElement.ElementType.Footer; } void IAddChild.AddChild(object value) { if (value is NamedElement || value is ParagraphStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(NamedElement), typeof(ParagraphStructure)), "value"); } void IAddChild.AddText(string text) { } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
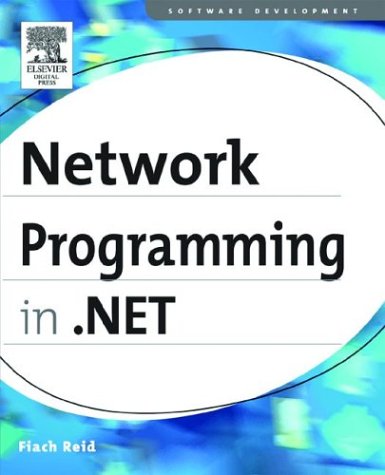
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Matrix3DConverter.cs
- ScalarType.cs
- MultiSelectRootGridEntry.cs
- RestClientProxyHandler.cs
- MemberProjectedSlot.cs
- HMACSHA512.cs
- WebControl.cs
- BaseTreeIterator.cs
- FlowPanelDesigner.cs
- UrlAuthorizationModule.cs
- IsolatedStorageFilePermission.cs
- PeerServiceMessageContracts.cs
- EventHandlerList.cs
- DataGridViewCheckBoxColumn.cs
- StatusBarPanel.cs
- Lock.cs
- XmlText.cs
- Thickness.cs
- MetadataFile.cs
- WorkflowRuntimeServiceElement.cs
- CheckBoxRenderer.cs
- Rotation3D.cs
- RegisteredExpandoAttribute.cs
- WebDescriptionAttribute.cs
- WebScriptEndpoint.cs
- PromptBuilder.cs
- ValueTypeFixupInfo.cs
- Helpers.cs
- FloaterParagraph.cs
- Visual.cs
- Accessible.cs
- AttachInfo.cs
- SaveFileDialog.cs
- Set.cs
- FileDialogPermission.cs
- IndexerHelper.cs
- Vertex.cs
- NamedElement.cs
- DataColumn.cs
- GroupByQueryOperator.cs
- LoadRetryConstantStrategy.cs
- SoapReflectionImporter.cs
- XmlSignatureManifest.cs
- Typography.cs
- LinkedList.cs
- SourceExpressionException.cs
- MethodCallConverter.cs
- XmlWriterSettings.cs
- ToolStripSplitButton.cs
- MaskedTextBoxDesigner.cs
- SerializationEventsCache.cs
- StatusBar.cs
- BehaviorEditorPart.cs
- VirtualPath.cs
- ProfileProvider.cs
- EntitySetDataBindingList.cs
- NavigationWindow.cs
- ToolStripScrollButton.cs
- DateTimeFormatInfo.cs
- WizardSideBarListControlItem.cs
- EditBehavior.cs
- OleDbInfoMessageEvent.cs
- AnnotationHelper.cs
- UnknownWrapper.cs
- DefaultTextStoreTextComposition.cs
- DocumentXmlWriter.cs
- Html32TextWriter.cs
- Matrix.cs
- ACL.cs
- HeaderUtility.cs
- DesignSurfaceCollection.cs
- ConfigXmlText.cs
- StatementContext.cs
- TransactionScope.cs
- hresults.cs
- DiffuseMaterial.cs
- ExpressionCopier.cs
- ToolStripLabel.cs
- EventLogPermission.cs
- SiteMapHierarchicalDataSourceView.cs
- SelectionProviderWrapper.cs
- Rotation3D.cs
- SecurityDescriptor.cs
- MetadataWorkspace.cs
- SubMenuStyleCollectionEditor.cs
- TableLayoutSettingsTypeConverter.cs
- MenuItemStyleCollection.cs
- ProviderConnectionPointCollection.cs
- InvocationExpression.cs
- Track.cs
- PackWebRequestFactory.cs
- DetailsViewCommandEventArgs.cs
- RuntimeConfig.cs
- TreeViewImageIndexConverter.cs
- ButtonFlatAdapter.cs
- ArrayElementGridEntry.cs
- BookmarkUndoUnit.cs
- SpellCheck.cs
- NetworkInformationPermission.cs
- MDIClient.cs