Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / HMACSHA512.cs / 1305376 / HMACSHA512.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // HMACSHA512.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public class HMACSHA512 : HMAC { private bool m_useLegacyBlockSize = Utils._ProduceLegacyHmacValues(); // // public constructors // public HMACSHA512 () : this (Utils.GenerateRandom(128)) {} [System.Security.SecuritySafeCritical] // auto-generated public HMACSHA512 (byte[] key) { m_hashName = "SHA512"; m_hash1 = new SHA512Managed(); m_hash2 = new SHA512Managed(); HashSizeValue = 512; BlockSizeValue = BlockSize; base.InitializeKey(key); } private int BlockSize { get { return m_useLegacyBlockSize ? 64 : 128; } } ////// In Whidbey we incorrectly used a block size of 64 bytes for HMAC-SHA-384 and HMAC-SHA-512, /// rather than using the correct value of 128 bytes. Setting this to true causes us to fall /// back to the Whidbey mode which produces incorrect HMAC values. /// /// This value should be set only once, before hashing has begun, since we need to reset the key /// buffer for the block size change to take effect. /// /// The default vaue is off, however this can be toggled for the application by setting the /// legacyHMACMode config switch. /// public bool ProduceLegacyHmacValues { get { return m_useLegacyBlockSize; } set { m_useLegacyBlockSize = value; BlockSizeValue = BlockSize; InitializeKey(KeyValue); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // HMACSHA512.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public class HMACSHA512 : HMAC { private bool m_useLegacyBlockSize = Utils._ProduceLegacyHmacValues(); // // public constructors // public HMACSHA512 () : this (Utils.GenerateRandom(128)) {} [System.Security.SecuritySafeCritical] // auto-generated public HMACSHA512 (byte[] key) { m_hashName = "SHA512"; m_hash1 = new SHA512Managed(); m_hash2 = new SHA512Managed(); HashSizeValue = 512; BlockSizeValue = BlockSize; base.InitializeKey(key); } private int BlockSize { get { return m_useLegacyBlockSize ? 64 : 128; } } ////// In Whidbey we incorrectly used a block size of 64 bytes for HMAC-SHA-384 and HMAC-SHA-512, /// rather than using the correct value of 128 bytes. Setting this to true causes us to fall /// back to the Whidbey mode which produces incorrect HMAC values. /// /// This value should be set only once, before hashing has begun, since we need to reset the key /// buffer for the block size change to take effect. /// /// The default vaue is off, however this can be toggled for the application by setting the /// legacyHMACMode config switch. /// public bool ProduceLegacyHmacValues { get { return m_useLegacyBlockSize; } set { m_useLegacyBlockSize = value; BlockSizeValue = BlockSize; InitializeKey(KeyValue); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
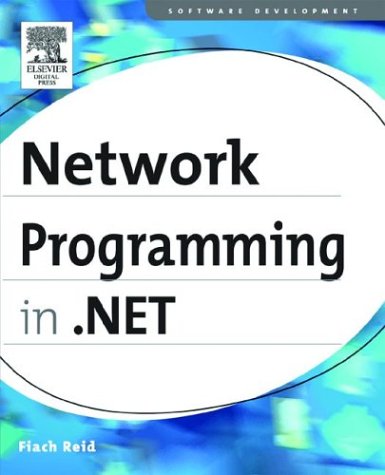
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AxHost.cs
- BigInt.cs
- InputChannel.cs
- PrintControllerWithStatusDialog.cs
- JsonMessageEncoderFactory.cs
- Variant.cs
- Geometry3D.cs
- DATA_BLOB.cs
- EmissiveMaterial.cs
- XmlEventCache.cs
- HostedTransportConfigurationBase.cs
- ObjectDisposedException.cs
- GPPOINTF.cs
- RotateTransform.cs
- EnumerableCollectionView.cs
- RadioButtonPopupAdapter.cs
- XmlSchema.cs
- XmlAttributeHolder.cs
- ToolStripComboBox.cs
- FileRecordSequence.cs
- CompilationRelaxations.cs
- MetadataItemEmitter.cs
- CurrentChangingEventManager.cs
- listviewsubitemcollectioneditor.cs
- TableCell.cs
- ReaderContextStackData.cs
- TabControlCancelEvent.cs
- XamlReader.cs
- DictionarySectionHandler.cs
- RoutedEvent.cs
- linebase.cs
- QilLiteral.cs
- FixedDocument.cs
- MenuItem.cs
- PrincipalPermission.cs
- DataGridViewComboBoxEditingControl.cs
- RowToFieldTransformer.cs
- Slider.cs
- TraceContext.cs
- TreeNodeStyle.cs
- ImageMap.cs
- _AcceptOverlappedAsyncResult.cs
- AssignDesigner.xaml.cs
- SizeChangedInfo.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- DataKeyCollection.cs
- SamlSecurityToken.cs
- SchemaImporter.cs
- TransformationRules.cs
- StoreItemCollection.cs
- UpdatePanel.cs
- ExcludeFromCodeCoverageAttribute.cs
- ConstraintStruct.cs
- StylusPlugInCollection.cs
- StringComparer.cs
- LoginView.cs
- CompositeActivityDesigner.cs
- JsonFormatGeneratorStatics.cs
- Model3DCollection.cs
- DispatcherProcessingDisabled.cs
- RowTypeElement.cs
- HtmlHistory.cs
- CopyOfAction.cs
- ContentValidator.cs
- AuthenticatingEventArgs.cs
- GifBitmapEncoder.cs
- Speller.cs
- EventPropertyMap.cs
- HandlerBase.cs
- WmfPlaceableFileHeader.cs
- MarkupWriter.cs
- DataGridViewBand.cs
- TransformValueSerializer.cs
- PolicyManager.cs
- TextEffectCollection.cs
- GlobalItem.cs
- ResourceExpression.cs
- AppliedDeviceFiltersDialog.cs
- Point3DAnimationBase.cs
- CookielessData.cs
- WebPartVerb.cs
- AutomationProperty.cs
- SafeFileMapViewHandle.cs
- AppDomainUnloadedException.cs
- PersistenceMetadataNamespace.cs
- BamlResourceDeserializer.cs
- InfoCardProofToken.cs
- SafeBitVector32.cs
- WSSecurityOneDotZeroSendSecurityHeader.cs
- DBAsyncResult.cs
- ItemContainerGenerator.cs
- ContentFileHelper.cs
- TypeExtension.cs
- SafeRightsManagementSessionHandle.cs
- KeySplineConverter.cs
- CodeCommentStatement.cs
- SecUtil.cs
- ComplexLine.cs
- ShimAsPublicXamlType.cs
- DataObjectCopyingEventArgs.cs