Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / SpellCheck.cs / 1 / SpellCheck.cs
//---------------------------------------------------------------------------- // // File: SpellCheck.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Speller properties for TextBoxBase. // //--------------------------------------------------------------------------- namespace System.Windows.Controls { using System.Threading; using System.Windows.Documents; using System.Windows.Controls.Primitives; ////// Speller properties for TextBoxBase. /// public sealed class SpellCheck { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Ctor. internal SpellCheck(TextBoxBase owner) { _owner = owner; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Enables and disables spell checking within the associated TextBoxBase. /// ////// Defaults to false. /// public bool IsEnabled { get { return (bool)_owner.GetValue(IsEnabledProperty); } set { _owner.SetValue(IsEnabledProperty, value); } } ////// Enables and disables spell checking within a TextBoxBase. /// public static void SetIsEnabled(TextBoxBase textBoxBase, bool value) { if (textBoxBase == null) { throw new ArgumentNullException("textBoxBase"); } textBoxBase.SetValue(IsEnabledProperty, value); } ////// Enables and disables spell checking within the associated TextBoxBase. /// ////// Defaults to false. /// public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.RegisterAttached( "IsEnabled", typeof(bool), typeof(SpellCheck), new FrameworkPropertyMetadata(new PropertyChangedCallback(OnIsEnabledChanged))); ////// The spelling reform mode for the associated TextBoxBase. /// ////// In languages with reformed spelling rules (such as German or French), /// this property specifies whether to apply old (prereform) or new /// (postreform) spelling rules to examined text. /// public SpellingReform SpellingReform { get { return (SpellingReform)_owner.GetValue(SpellingReformProperty); } set { _owner.SetValue(SpellingReformProperty, value); } } ////// Sets the spelling reform mode for a TextBoxBase. /// public static void SetSpellingReform(TextBoxBase textBoxBase, SpellingReform value) { if (textBoxBase == null) { throw new ArgumentNullException("textBoxBase"); } textBoxBase.SetValue(SpellingReformProperty, value); } ////// The spelling reform mode for the associated TextBoxBase. /// ////// In languages with reformed spelling rules (such as German or French), /// this property specifies whether to apply old (prereform) or new /// (postreform) spelling rules to examined text. /// public static readonly DependencyProperty SpellingReformProperty = DependencyProperty.RegisterAttached( "SpellingReform", typeof(SpellingReform), typeof(SpellCheck), new FrameworkPropertyMetadata(Thread.CurrentThread.CurrentCulture.TwoLetterISOLanguageName == "de" ? SpellingReform.Postreform : SpellingReform.PreAndPostreform, new PropertyChangedCallback(OnSpellingReformChanged))); #endregion Public Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Callback for changes to the IsEnabled property. private static void OnIsEnabledChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TextBoxBase textBoxBase = d as TextBoxBase; if (textBoxBase != null) { TextEditor textEditor = TextEditor._GetTextEditor(textBoxBase); if (textEditor != null) { textEditor.SetSpellCheckEnabled((bool)e.NewValue); } } } // SpellingReformProperty change callback. private static void OnSpellingReformChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TextBoxBase textBoxBase = d as TextBoxBase; if (textBoxBase != null) { TextEditor textEditor = TextEditor._GetTextEditor(textBoxBase); if (textEditor != null) { textEditor.SetSpellingReform((SpellingReform)e.NewValue); } } } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // TextBoxBase mapped to this object. private readonly TextBoxBase _owner; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: SpellCheck.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Speller properties for TextBoxBase. // //--------------------------------------------------------------------------- namespace System.Windows.Controls { using System.Threading; using System.Windows.Documents; using System.Windows.Controls.Primitives; ////// Speller properties for TextBoxBase. /// public sealed class SpellCheck { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Ctor. internal SpellCheck(TextBoxBase owner) { _owner = owner; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Enables and disables spell checking within the associated TextBoxBase. /// ////// Defaults to false. /// public bool IsEnabled { get { return (bool)_owner.GetValue(IsEnabledProperty); } set { _owner.SetValue(IsEnabledProperty, value); } } ////// Enables and disables spell checking within a TextBoxBase. /// public static void SetIsEnabled(TextBoxBase textBoxBase, bool value) { if (textBoxBase == null) { throw new ArgumentNullException("textBoxBase"); } textBoxBase.SetValue(IsEnabledProperty, value); } ////// Enables and disables spell checking within the associated TextBoxBase. /// ////// Defaults to false. /// public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.RegisterAttached( "IsEnabled", typeof(bool), typeof(SpellCheck), new FrameworkPropertyMetadata(new PropertyChangedCallback(OnIsEnabledChanged))); ////// The spelling reform mode for the associated TextBoxBase. /// ////// In languages with reformed spelling rules (such as German or French), /// this property specifies whether to apply old (prereform) or new /// (postreform) spelling rules to examined text. /// public SpellingReform SpellingReform { get { return (SpellingReform)_owner.GetValue(SpellingReformProperty); } set { _owner.SetValue(SpellingReformProperty, value); } } ////// Sets the spelling reform mode for a TextBoxBase. /// public static void SetSpellingReform(TextBoxBase textBoxBase, SpellingReform value) { if (textBoxBase == null) { throw new ArgumentNullException("textBoxBase"); } textBoxBase.SetValue(SpellingReformProperty, value); } ////// The spelling reform mode for the associated TextBoxBase. /// ////// In languages with reformed spelling rules (such as German or French), /// this property specifies whether to apply old (prereform) or new /// (postreform) spelling rules to examined text. /// public static readonly DependencyProperty SpellingReformProperty = DependencyProperty.RegisterAttached( "SpellingReform", typeof(SpellingReform), typeof(SpellCheck), new FrameworkPropertyMetadata(Thread.CurrentThread.CurrentCulture.TwoLetterISOLanguageName == "de" ? SpellingReform.Postreform : SpellingReform.PreAndPostreform, new PropertyChangedCallback(OnSpellingReformChanged))); #endregion Public Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Callback for changes to the IsEnabled property. private static void OnIsEnabledChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TextBoxBase textBoxBase = d as TextBoxBase; if (textBoxBase != null) { TextEditor textEditor = TextEditor._GetTextEditor(textBoxBase); if (textEditor != null) { textEditor.SetSpellCheckEnabled((bool)e.NewValue); } } } // SpellingReformProperty change callback. private static void OnSpellingReformChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TextBoxBase textBoxBase = d as TextBoxBase; if (textBoxBase != null) { TextEditor textEditor = TextEditor._GetTextEditor(textBoxBase); if (textEditor != null) { textEditor.SetSpellingReform((SpellingReform)e.NewValue); } } } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // TextBoxBase mapped to this object. private readonly TextBoxBase _owner; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
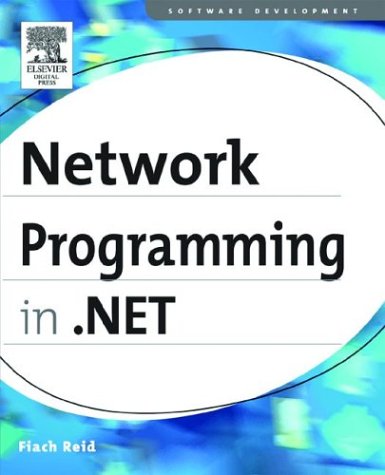
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesigntimeLicenseContext.cs
- MaterialCollection.cs
- DispatcherTimer.cs
- SvcMapFileLoader.cs
- ToolStripSettings.cs
- MediaTimeline.cs
- ToolStripSplitButton.cs
- DataIdProcessor.cs
- XmlNotation.cs
- TimeSpanValidator.cs
- BaseTemplatedMobileComponentEditor.cs
- NavigationCommands.cs
- Image.cs
- ConfigurationManager.cs
- FilteredReadOnlyMetadataCollection.cs
- ProvidePropertyAttribute.cs
- OleDbSchemaGuid.cs
- MTConfigUtil.cs
- WithStatement.cs
- httpstaticobjectscollection.cs
- SafeEventLogWriteHandle.cs
- WebResponse.cs
- NumericUpDown.cs
- _IPv6Address.cs
- DetailsViewDeletedEventArgs.cs
- EditorAttribute.cs
- ListControl.cs
- WorkflowDesignerColors.cs
- XmlSchemaCollection.cs
- SimpleNameService.cs
- SmtpFailedRecipientException.cs
- ContextMenuService.cs
- LabelLiteral.cs
- LocalizedNameDescriptionPair.cs
- MsmqInputChannel.cs
- KeyGestureValueSerializer.cs
- OutputScopeManager.cs
- SecurityTokenContainer.cs
- AccessViolationException.cs
- VisualBasic.cs
- PathHelper.cs
- CompilerErrorCollection.cs
- ScriptResourceInfo.cs
- EffectiveValueEntry.cs
- DmlSqlGenerator.cs
- SqlXml.cs
- InternalResources.cs
- FirstMatchCodeGroup.cs
- InputManager.cs
- PointLightBase.cs
- PropertyEmitter.cs
- ToolStripManager.cs
- XPathChildIterator.cs
- CompositeActivityTypeDescriptorProvider.cs
- ListViewHitTestInfo.cs
- Visual3D.cs
- UrlMappingCollection.cs
- DragDropManager.cs
- MarkupProperty.cs
- HostVisual.cs
- OperatingSystem.cs
- SafeThemeHandle.cs
- Function.cs
- X509ChainElement.cs
- MaskPropertyEditor.cs
- XmlUtil.cs
- mediapermission.cs
- GlyphCache.cs
- ChannelSinkStacks.cs
- precedingsibling.cs
- FontNamesConverter.cs
- MonthChangedEventArgs.cs
- SystemEvents.cs
- PrintController.cs
- Model3DGroup.cs
- Canvas.cs
- oledbmetadatacolumnnames.cs
- TreeNodeStyleCollection.cs
- UriGenerator.cs
- OleDbConnectionFactory.cs
- WorkflowMarkupSerializationProvider.cs
- ImageSource.cs
- TableHeaderCell.cs
- UpdatePanelTrigger.cs
- OdbcDataReader.cs
- CollectionContainer.cs
- SubMenuStyle.cs
- iisPickupDirectory.cs
- StorageBasedPackageProperties.cs
- HtmlInputSubmit.cs
- InstancePersistenceEvent.cs
- SQLMoney.cs
- InvalidCommandTreeException.cs
- WindowsTooltip.cs
- TdsParameterSetter.cs
- SchemaSetCompiler.cs
- RadioButtonPopupAdapter.cs
- SerializationSectionGroup.cs
- BlockUIContainer.cs
- CatalogPartCollection.cs