Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / MaskPropertyEditor.cs / 1 / MaskPropertyEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System; using System.Design; using System.Drawing.Design; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms; using System.Diagnostics; ////// Design time editing class for the Mask property of the MaskedTextBox control. /// internal class MaskPropertyEditor : UITypeEditor { ////// Constructor. /// public MaskPropertyEditor() { } ////// Gets the mask property value fromt the MaskDesignerDialog. /// The IUIService is used to show the mask designer dialog within VS so it doesn't get blocked if focus /// is moved to anoter app. /// internal static string EditMask(ITypeDiscoveryService discoverySvc, IUIService uiSvc, MaskedTextBox instance, IHelpService helpService) { Debug.Assert( instance != null, "Null masked text box." ); string mask = null; MaskDesignerDialog dlg = new MaskDesignerDialog(instance, helpService); try { dlg.DiscoverMaskDescriptors( discoverySvc ); // fine if service is null. // Show dialog from VS. // Debug.Assert( uiSvc != null, "Expected IUIService, defaulting to an intrusive way to show the dialog..." ); DialogResult dlgResult = uiSvc != null ? uiSvc.ShowDialog( dlg ) : dlg.ShowDialog(); if ( dlgResult == DialogResult.OK) { mask = dlg.Mask; // ValidatingType is not browsable so we don't need to set the property through the designer. if (dlg.ValidatingType != instance.ValidatingType) { instance.ValidatingType = dlg.ValidatingType; } } } finally { dlg.Dispose(); } // Will return null if dlgResult != OK. return mask; } ////// Edits the Mask property of the MaskedTextBox control from the PropertyGrid. /// // This should be okay since System.Design only runs in FullTrust. // SECREVIEW: Isn't that true [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public override object EditValue(System.ComponentModel.ITypeDescriptorContext context, IServiceProvider provider, object value) { if (context != null && provider != null) { ITypeDiscoveryService discoverySvc = (ITypeDiscoveryService) provider.GetService(typeof(ITypeDiscoveryService)); // fine if service is not found. IUIService uiSvc = (IUIService) provider.GetService(typeof(IUIService)); IHelpService helpService = (IHelpService)provider.GetService(typeof(IHelpService)); string mask = MaskPropertyEditor.EditMask(discoverySvc, uiSvc, context.Instance as MaskedTextBox, helpService); if( mask != null ) { return mask; } } return value; } ////// Painting a representation of the Mask value is not supported. /// // This should be okay since System.Design only runs in FullTrust. // SECREVIEW: Isn't that true [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public override bool GetPaintValueSupported(System.ComponentModel.ITypeDescriptorContext context) { return false; } ////// Gets the edit style of the type editor. /// // This should be okay since System.Design only runs in FullTrust. // SECREVIEW: Isn't that true [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public override UITypeEditorEditStyle GetEditStyle(System.ComponentModel.ITypeDescriptorContext context) { return UITypeEditorEditStyle.Modal; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
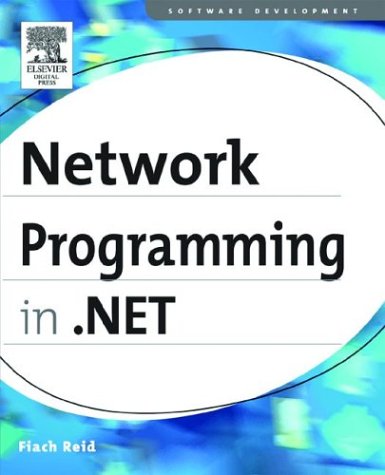
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CustomValidator.cs
- XPathParser.cs
- MultipleViewPattern.cs
- COSERVERINFO.cs
- VisualBrush.cs
- SerializationAttributes.cs
- WebBrowsableAttribute.cs
- QueryAccessibilityHelpEvent.cs
- DbParameterHelper.cs
- Ray3DHitTestResult.cs
- DataKey.cs
- X509ChainElement.cs
- AsynchronousChannelMergeEnumerator.cs
- PathGradientBrush.cs
- InternalTypeHelper.cs
- VariableQuery.cs
- LinkButton.cs
- Classification.cs
- ToolBar.cs
- EventHandlerList.cs
- FollowerQueueCreator.cs
- VisualState.cs
- TCPClient.cs
- ConfigurationStrings.cs
- X509RawDataKeyIdentifierClause.cs
- ContainerParagraph.cs
- SmiMetaDataProperty.cs
- TemplateControl.cs
- altserialization.cs
- Renderer.cs
- FullTextLine.cs
- TemplateXamlTreeBuilder.cs
- ItemAutomationPeer.cs
- DocumentOrderQuery.cs
- VirtualizedCellInfoCollection.cs
- ProfileGroupSettingsCollection.cs
- MessageParameterAttribute.cs
- InvokeBase.cs
- XPathNodeList.cs
- indexingfiltermarshaler.cs
- StylusPlugin.cs
- httpserverutility.cs
- QueryResult.cs
- TCEAdapterGenerator.cs
- ItemCheckedEvent.cs
- _Connection.cs
- MembershipUser.cs
- RuntimeEnvironment.cs
- HealthMonitoringSectionHelper.cs
- Collection.cs
- NullableBoolConverter.cs
- SvcMapFile.cs
- SmiEventSink_Default.cs
- ExpanderAutomationPeer.cs
- ResourceLoader.cs
- Parameter.cs
- AdapterUtil.cs
- COM2ExtendedTypeConverter.cs
- ResourceDefaultValueAttribute.cs
- ForwardPositionQuery.cs
- XmlSchemaSimpleTypeRestriction.cs
- MulticastNotSupportedException.cs
- ObjectCloneHelper.cs
- Evidence.cs
- UnmanagedMarshal.cs
- Image.cs
- DataGridViewEditingControlShowingEventArgs.cs
- TakeQueryOptionExpression.cs
- ComEventsHelper.cs
- CroppedBitmap.cs
- UriSectionReader.cs
- TableSectionStyle.cs
- RegexWriter.cs
- TypeElement.cs
- TimeEnumHelper.cs
- EventLevel.cs
- columnmapfactory.cs
- FilteredDataSetHelper.cs
- DetailsViewUpdatedEventArgs.cs
- Odbc32.cs
- XomlSerializationHelpers.cs
- OrderPreservingSpoolingTask.cs
- HttpListener.cs
- HostingEnvironmentWrapper.cs
- AnchorEditor.cs
- ApplySecurityAndSendAsyncResult.cs
- Missing.cs
- ComboBoxRenderer.cs
- EventDrivenDesigner.cs
- WindowsPrincipal.cs
- DataGridViewDataErrorEventArgs.cs
- Clock.cs
- ContainerVisual.cs
- ISFClipboardData.cs
- Activity.cs
- WindowsComboBox.cs
- DataRowChangeEvent.cs
- SchemaObjectWriter.cs
- ResourceType.cs
- SQLRoleProvider.cs