Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / ApplySecurityAndSendAsyncResult.cs / 1 / ApplySecurityAndSendAsyncResult.cs
//---------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security { using System.Runtime.InteropServices; using System.ServiceModel.Channels; abstract class ApplySecurityAndSendAsyncResult: AsyncResult where MessageSenderType : class { readonly MessageSenderType channel; readonly SecurityProtocol binding; volatile bool secureOutgoingMessageDone; static AsyncCallback sharedCallback = DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(SharedCallback)); SecurityProtocolCorrelationState newCorrelationState; TimeoutHelper timeoutHelper; public ApplySecurityAndSendAsyncResult(SecurityProtocol binding, MessageSenderType channel, TimeSpan timeout, AsyncCallback callback, object state) : base(callback, state) { this.binding = binding; this.channel = channel; this.timeoutHelper = new TimeoutHelper(timeout); } protected SecurityProtocolCorrelationState CorrelationState { get { return newCorrelationState; } } protected SecurityProtocol SecurityProtocol { get { return this.binding; } } protected void Begin(Message message, SecurityProtocolCorrelationState correlationState) { IAsyncResult result = this.binding.BeginSecureOutgoingMessage(message, timeoutHelper.RemainingTime(), correlationState, sharedCallback, this); if (result.CompletedSynchronously) { this.binding.EndSecureOutgoingMessage(result, out message, out newCorrelationState); bool completedSynchronously = this.OnSecureOutgoingMessageComplete(message); if (completedSynchronously) { Complete(true); } } } protected static void OnEnd(ApplySecurityAndSendAsyncResult self) { AsyncResult.End >(self); } bool OnSecureOutgoingMessageComplete(Message message) { if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("message")); } this.secureOutgoingMessageDone = true; IAsyncResult result = BeginSendCore(this.channel, message, timeoutHelper.RemainingTime(), sharedCallback, this); if (!result.CompletedSynchronously) { return false; } EndSendCore(this.channel, result); return this.OnSendComplete(); } protected abstract IAsyncResult BeginSendCore(MessageSenderType channel, Message message, TimeSpan timeout, AsyncCallback callback, object state); protected abstract void EndSendCore(MessageSenderType channel, IAsyncResult result); bool OnSendComplete() { OnSendCompleteCore(timeoutHelper.RemainingTime()); return true; } protected abstract void OnSendCompleteCore(TimeSpan timeout); static void SharedCallback(IAsyncResult result) { if (result == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("result")); } if (result.CompletedSynchronously) { return; } ApplySecurityAndSendAsyncResult self = result.AsyncState as ApplySecurityAndSendAsyncResult ; if (self == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.InvalidAsyncResult), "result")); } bool completeSelf = false; Exception completionException = null; try { if (!self.secureOutgoingMessageDone) { Message message; self.binding.EndSecureOutgoingMessage(result, out message, out self.newCorrelationState); completeSelf = self.OnSecureOutgoingMessageComplete(message); } else { self.EndSendCore(self.channel, result); completeSelf = self.OnSendComplete(); } } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { if (Diagnostics.ExceptionUtility.IsFatal(e)) throw; completeSelf = true; completionException = e; } if (completeSelf) { self.Complete(false, completionException); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
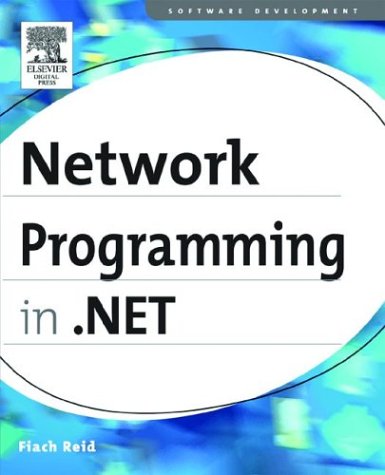
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlInputCheckBox.cs
- FixedTextView.cs
- SizeChangedInfo.cs
- NameValueConfigurationCollection.cs
- PrimitiveXmlSerializers.cs
- SplitterPanel.cs
- LexicalChunk.cs
- Shape.cs
- ProtectedConfiguration.cs
- StorageEntityContainerMapping.cs
- WebExceptionStatus.cs
- UIInitializationException.cs
- X509Certificate.cs
- FormViewUpdateEventArgs.cs
- Int16.cs
- AsyncOperationLifetimeManager.cs
- SymDocumentType.cs
- SharedPerformanceCounter.cs
- XmlSchemaAnyAttribute.cs
- MsmqIntegrationSecurity.cs
- DataGridViewColumnTypePicker.cs
- PriorityBindingExpression.cs
- ListView.cs
- HttpListenerResponse.cs
- ReachDocumentPageSerializerAsync.cs
- CheckBoxAutomationPeer.cs
- XmlAttribute.cs
- ChtmlMobileTextWriter.cs
- KnownBoxes.cs
- XmlReflectionImporter.cs
- BaseCollection.cs
- SoapRpcMethodAttribute.cs
- ResXResourceSet.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- SortFieldComparer.cs
- RotationValidation.cs
- FileUpload.cs
- Camera.cs
- TTSEngineProxy.cs
- CookieProtection.cs
- ServicePointManagerElement.cs
- DataMember.cs
- StickyNoteAnnotations.cs
- FastEncoderStatics.cs
- ValidationError.cs
- CalendarButton.cs
- ValueTypeFixupInfo.cs
- SoapAttributes.cs
- TypeToStringValueConverter.cs
- PnrpPermission.cs
- HtmlButton.cs
- TableLayoutPanelCellPosition.cs
- MailDefinition.cs
- PageParserFilter.cs
- SqlDataSourceConfigureSortForm.cs
- DeferrableContent.cs
- RefreshEventArgs.cs
- Math.cs
- ConfigXmlWhitespace.cs
- WebServiceClientProxyGenerator.cs
- xml.cs
- WebException.cs
- DataControlImageButton.cs
- CompilerTypeWithParams.cs
- StateFinalizationDesigner.cs
- BamlRecordReader.cs
- TextBoxBase.cs
- ClientRuntimeConfig.cs
- TreeNodeEventArgs.cs
- RecordBuilder.cs
- DynamicScriptObject.cs
- InstanceBehavior.cs
- x509store.cs
- NativeMethods.cs
- SecurityRuntime.cs
- SQLBinary.cs
- DateTimeSerializationSection.cs
- LockCookie.cs
- RightsDocument.cs
- PowerStatus.cs
- TopClause.cs
- PersonalizableAttribute.cs
- LongTypeConverter.cs
- ChannelProtectionRequirements.cs
- FilterableAttribute.cs
- ReaderContextStackData.cs
- HierarchicalDataTemplate.cs
- BamlBinaryReader.cs
- AutomationPropertyInfo.cs
- BaseWebProxyFinder.cs
- Label.cs
- TypeUtils.cs
- DataRelationCollection.cs
- DataGridViewRowStateChangedEventArgs.cs
- TemplateControl.cs
- CommandDevice.cs
- ArcSegment.cs
- EncoderExceptionFallback.cs
- ListViewSortEventArgs.cs
- ConfigXmlCDataSection.cs