Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / Serialization / SoapAttributes.cs / 1 / SoapAttributes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Serialization { using System; using System.Reflection; using System.Collections; using System.ComponentModel; internal enum SoapAttributeFlags { Enum = 0x1, Type = 0x2, Element = 0x4, Attribute = 0x8, } ////// /// public class SoapAttributes { bool soapIgnore; SoapTypeAttribute soapType; SoapElementAttribute soapElement; SoapAttributeAttribute soapAttribute; SoapEnumAttribute soapEnum; object soapDefaultValue = null; ///[To be supplied.] ////// /// public SoapAttributes() { } ///[To be supplied.] ////// /// public SoapAttributes(ICustomAttributeProvider provider) { object[] attrs = provider.GetCustomAttributes(false); for (int i = 0; i < attrs.Length; i++) { if (attrs[i] is SoapIgnoreAttribute || attrs[i] is ObsoleteAttribute) { this.soapIgnore = true; break; } else if (attrs[i] is SoapElementAttribute) { this.soapElement = (SoapElementAttribute)attrs[i]; } else if (attrs[i] is SoapAttributeAttribute) { this.soapAttribute = (SoapAttributeAttribute)attrs[i]; } else if (attrs[i] is SoapTypeAttribute) { this.soapType = (SoapTypeAttribute)attrs[i]; } else if (attrs[i] is SoapEnumAttribute) { this.soapEnum = (SoapEnumAttribute)attrs[i]; } else if (attrs[i] is DefaultValueAttribute) { this.soapDefaultValue = ((DefaultValueAttribute)attrs[i]).Value; } } if (soapIgnore) { this.soapElement = null; this.soapAttribute = null; this.soapType = null; this.soapEnum = null; this.soapDefaultValue = null; } } internal SoapAttributeFlags SoapFlags { get { SoapAttributeFlags flags = 0; if (soapElement != null) flags |= SoapAttributeFlags.Element; if (soapAttribute != null) flags |= SoapAttributeFlags.Attribute; if (soapEnum != null) flags |= SoapAttributeFlags.Enum; if (soapType != null) flags |= SoapAttributeFlags.Type; return flags; } } ///[To be supplied.] ////// /// public SoapTypeAttribute SoapType { get { return soapType; } set { soapType = value; } } ///[To be supplied.] ////// /// public SoapEnumAttribute SoapEnum { get { return soapEnum; } set { soapEnum = value; } } ///[To be supplied.] ////// /// public bool SoapIgnore { get { return soapIgnore; } set { soapIgnore = value; } } ///[To be supplied.] ////// /// public SoapElementAttribute SoapElement { get { return soapElement; } set { soapElement = value; } } ///[To be supplied.] ////// /// public SoapAttributeAttribute SoapAttribute { get { return soapAttribute; } set { soapAttribute = value; } } ///[To be supplied.] ////// /// public object SoapDefaultValue { get { return soapDefaultValue; } set { soapDefaultValue = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
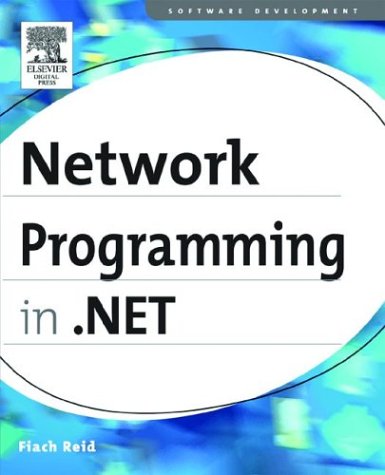
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AvTraceDetails.cs
- infer.cs
- ReferencedAssembly.cs
- MultipleViewPattern.cs
- RtfToken.cs
- SuppressMergeCheckAttribute.cs
- DiscoveryClientChannelBase.cs
- XmlBoundElement.cs
- FileDialogCustomPlace.cs
- TextViewBase.cs
- BitmapImage.cs
- DropShadowEffect.cs
- RelationshipEndMember.cs
- ToolStripContainer.cs
- DiagnosticTrace.cs
- DateTimeSerializationSection.cs
- SwitchElementsCollection.cs
- HandlerBase.cs
- HttpRuntime.cs
- TextCompositionEventArgs.cs
- DependencyObject.cs
- Int32Storage.cs
- ContainerSelectorActiveEvent.cs
- WebPartDisplayMode.cs
- ListViewSortEventArgs.cs
- Vector3DConverter.cs
- HttpDictionary.cs
- GeneralTransform3DGroup.cs
- FormsAuthenticationEventArgs.cs
- QuerySelectOp.cs
- SystemTcpStatistics.cs
- BulletedList.cs
- DataSourceCacheDurationConverter.cs
- ElementFactory.cs
- EnumerableRowCollection.cs
- DataPagerFieldCommandEventArgs.cs
- SystemGatewayIPAddressInformation.cs
- TypeSemantics.cs
- WebPartCloseVerb.cs
- filewebrequest.cs
- XmlWhitespace.cs
- XmlSchemaAttributeGroupRef.cs
- Profiler.cs
- SchemaElementDecl.cs
- EtwTrace.cs
- Timer.cs
- SafeProcessHandle.cs
- MediaContextNotificationWindow.cs
- HtmlEmptyTagControlBuilder.cs
- SurrogateEncoder.cs
- AmbientLight.cs
- SqlFileStream.cs
- IpcPort.cs
- PolicyException.cs
- RotationValidation.cs
- ToolTip.cs
- ServiceModelExtensionElement.cs
- MexHttpsBindingElement.cs
- XmlSerializationWriter.cs
- BaseUriWithWildcard.cs
- FlowDocumentFormatter.cs
- QilBinary.cs
- PluralizationServiceUtil.cs
- CodeExpressionStatement.cs
- CodeNamespaceImportCollection.cs
- AuthenticationException.cs
- AbstractExpressions.cs
- JsonEncodingStreamWrapper.cs
- COM2ExtendedBrowsingHandler.cs
- CharStorage.cs
- HandlerFactoryWrapper.cs
- TextRangeSerialization.cs
- SuppressMessageAttribute.cs
- BitmapInitialize.cs
- BamlReader.cs
- LayoutEngine.cs
- DataConnectionHelper.cs
- MulticastDelegate.cs
- TextLineBreak.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- SqlConnection.cs
- HelpEvent.cs
- SpnEndpointIdentity.cs
- XmlObjectSerializerReadContextComplexJson.cs
- MediaElementAutomationPeer.cs
- GreenMethods.cs
- GraphicsContainer.cs
- control.ime.cs
- TextEmbeddedObject.cs
- FormsAuthenticationUserCollection.cs
- DataGridAddNewRow.cs
- Mapping.cs
- DetailsViewInsertEventArgs.cs
- RuleInfoComparer.cs
- CollectionAdapters.cs
- RIPEMD160Managed.cs
- BooleanProjectedSlot.cs
- Package.cs
- ShaderEffect.cs
- LineInfo.cs