Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / ServiceModelExtensionElement.cs / 1 / ServiceModelExtensionElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System.Collections.Generic; using System.ServiceModel; using System.Configuration; using System.Diagnostics; using System.Globalization; using System.Security.Permissions; using System.ServiceModel.Diagnostics; using System.Xml; using System.Security; [ConfigurationPermission(SecurityAction.InheritanceDemand, Unrestricted = true)] public abstract class ServiceModelExtensionElement : ConfigurationElement, IConfigurationContextProviderInternal { ////// Critical - stores information used in a security decision /// [SecurityCritical] EvaluationContextHelper contextHelper; ContextInformation containingEvaluationContext = null; string configurationElementName = String.Empty; string extensionCollectionName = String.Empty; bool modified = false; protected ServiceModelExtensionElement() : base() { } ////// Critical - calls SecurityCritical method UnsafeLookupCollection which elevates in order to load config /// Safe - does not leak any config objects /// [SecurityCritical, SecurityTreatAsSafe] internal bool CanAdd(string extensionCollectionName, ContextInformation evaluationContext) { bool retVal = false; ExtensionElementCollection collection = ExtensionsSection.UnsafeLookupCollection(extensionCollectionName, evaluationContext); if (null != collection && collection.Count != 0) { foreach (ExtensionElement extensionElement in collection) { if (extensionElement.Type.Equals(this.GetType().AssemblyQualifiedName, StringComparison.Ordinal)) { retVal = true; break; } } if (!retVal && DiagnosticUtility.ShouldTraceWarning) { TraceUtility.TraceEvent(TraceEventType.Warning, TraceCode.ConfiguredExtensionTypeNotFound, this.CreateCanAddRecord(extensionCollectionName), this, null); } } else if (DiagnosticUtility.ShouldTraceWarning) { TraceCode traceCode = TraceCode.ExtensionCollectionDoesNotExist; if (null != collection && collection.Count == 0) { traceCode = TraceCode.ExtensionCollectionIsEmpty; } TraceUtility.TraceEvent(TraceEventType.Warning, traceCode, this.CreateCanAddRecord(extensionCollectionName), this, null); } return retVal; } public string ConfigurationElementName { get { if (String.IsNullOrEmpty(this.configurationElementName)) { this.configurationElementName = this.GetConfigurationElementName(); } return this.configurationElementName; } } internal ContextInformation ContainingEvaluationContext { get { return this.containingEvaluationContext; } set { this.containingEvaluationContext = value; } } public virtual void CopyFrom(ServiceModelExtensionElement from) { if (this.IsReadOnly()) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException(SR.GetString(SR.ConfigReadOnly))); } if (from == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("from"); } } DictionaryTraceRecord CreateCanAddRecord(string extensionCollectionName) { Dictionaryvalues = new Dictionary (2); values["ElementType"] = DiagnosticTrace.XmlEncode(this.GetType().AssemblyQualifiedName); values["CollectionName"] = ConfigurationStrings.ExtensionsSectionPath + "/" + extensionCollectionName; return new DictionaryTraceRecord(values); } internal void DeserializeInternal(XmlReader reader, bool serializeCollectionKey) { this.DeserializeElement(reader, serializeCollectionKey); } internal string ExtensionCollectionName { set { this.extensionCollectionName = value; } get { return this.extensionCollectionName; } } internal ContextInformation EvalContext { get { return this.EvaluationContext; } } internal object FromProperty(ConfigurationProperty property) { return this[property]; } /// /// Critical - calls SecurityCritical methods UnsafeLookupCollection and UnsafeLookupAssociatedCollection which elevate in order to load config /// Safe - does not leak any config objects /// [SecurityCritical, SecurityTreatAsSafe] string GetConfigurationElementName() { string configurationElementName = String.Empty; ExtensionElementCollection collection = null; Type extensionSectionType = this.GetType(); ContextInformation evaluationContext = ConfigurationHelpers.GetEvaluationContext(this); if (evaluationContext == null) { evaluationContext = this.ContainingEvaluationContext; } if (String.IsNullOrEmpty(this.extensionCollectionName)) { if (DiagnosticUtility.ShouldTraceWarning) { TraceUtility.TraceEvent(TraceEventType.Warning, TraceCode.ExtensionCollectionNameNotFound, this, (Exception)null); } collection = ExtensionsSection.UnsafeLookupAssociatedCollection(this.GetType(), evaluationContext, out this.extensionCollectionName); } else { collection = ExtensionsSection.UnsafeLookupCollection(this.extensionCollectionName, evaluationContext); } if (null == collection) { if (String.IsNullOrEmpty(this.extensionCollectionName)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException(SR.GetString(SR.ConfigNoExtensionCollectionAssociatedWithType, extensionSectionType.AssemblyQualifiedName), this.ElementInformation.Source, this.ElementInformation.LineNumber)); } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException(SR.GetString(SR.ConfigExtensionCollectionNotFound, this.extensionCollectionName), this.ElementInformation.Source, this.ElementInformation.LineNumber)); } } for (int i = 0; i < collection.Count; i++) { ExtensionElement collectionElement = collection[i]; // Optimize for assembly qualified names. if (collectionElement.Type.Equals(extensionSectionType.AssemblyQualifiedName, StringComparison.Ordinal)) { configurationElementName = collectionElement.Name; break; } // Check type directly for the case that the extension is registered with something less than // an full assembly qualified name. Type collectionElementType = Type.GetType(collectionElement.Type, false); if (null != collectionElementType && extensionSectionType.Equals(collectionElementType)) { configurationElementName = collectionElement.Name; break; } } if (String.IsNullOrEmpty(configurationElementName)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ConfigurationErrorsException(SR.GetString(SR.ConfigExtensionTypeNotRegisteredInCollection, extensionSectionType.AssemblyQualifiedName, this.extensionCollectionName), this.ElementInformation.Source, this.ElementInformation.LineNumber)); } return configurationElementName; } internal void InternalInitializeDefault() { this.InitializeDefault(); } protected override bool IsModified() { return this.modified | base.IsModified(); } internal bool IsModifiedInternal() { return this.IsModified(); } internal ConfigurationPropertyCollection PropertiesInternal { get { return this.Properties; } } internal void ResetModifiedInternal() { this.ResetModified(); } protected override bool SerializeElement(XmlWriter writer, bool serializeCollectionKey) { base.SerializeElement(writer, serializeCollectionKey); return true; } internal bool SerializeInternal(XmlWriter writer, bool serializeCollectionKey) { return this.SerializeElement(writer, serializeCollectionKey); } internal void SetReadOnlyInternal() { this.SetReadOnly(); } ////// Critical - accesses critical field contextHelper /// [SecurityCritical] protected override void Reset(ConfigurationElement parentElement) { this.contextHelper.OnReset(parentElement); base.Reset(parentElement); } ContextInformation IConfigurationContextProviderInternal.GetEvaluationContext() { return this.EvaluationContext; } ////// Critical -- accesses critical field contextHelper /// RequiresReview -- the return value will be used for a security decision -- see comment in interface definition /// [SecurityCritical] ContextInformation IConfigurationContextProviderInternal.GetOriginalEvaluationContext() { return this.contextHelper.GetOriginalContext(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
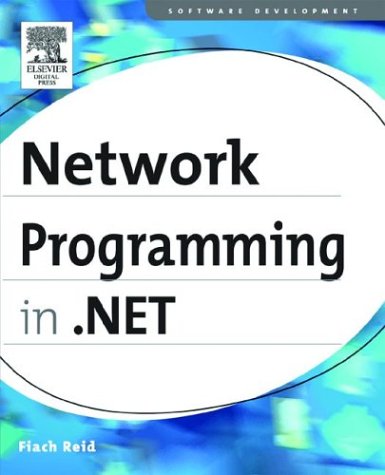
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializableTypeCodeDomSerializer.cs
- SevenBitStream.cs
- CharAnimationBase.cs
- GeneratedView.cs
- Schema.cs
- TextRangeEditTables.cs
- SoapServerProtocol.cs
- BaseCodePageEncoding.cs
- MailHeaderInfo.cs
- MimeParameters.cs
- RegistryDataKey.cs
- XmlSchemaSimpleContentExtension.cs
- TrimSurroundingWhitespaceAttribute.cs
- ContextMenuStrip.cs
- DBSchemaTable.cs
- CollectionChangedEventManager.cs
- XmlNullResolver.cs
- DataControlField.cs
- SelectionEditingBehavior.cs
- BinaryParser.cs
- LookupTables.cs
- HtmlTextArea.cs
- ProtocolsConfigurationHandler.cs
- TimeIntervalCollection.cs
- OdbcPermission.cs
- Condition.cs
- HttpListenerRequestUriBuilder.cs
- EventDescriptorCollection.cs
- SequenceDesigner.cs
- ArraySortHelper.cs
- QilChoice.cs
- BlurBitmapEffect.cs
- ConfigurationConverterBase.cs
- MsmqOutputMessage.cs
- Claim.cs
- RegexTypeEditor.cs
- XmlSerializerFactory.cs
- CustomErrorsSection.cs
- IndexedEnumerable.cs
- BidOverLoads.cs
- MgmtConfigurationRecord.cs
- CodeMethodReturnStatement.cs
- BindingSource.cs
- XmlQueryTypeFactory.cs
- IdentifierCreationService.cs
- SrgsElementList.cs
- UserControlAutomationPeer.cs
- ExpressionBuilder.cs
- XmlCDATASection.cs
- PerformanceCounters.cs
- EditCommandColumn.cs
- ProcessModelSection.cs
- ModelItem.cs
- CompilerInfo.cs
- TextProperties.cs
- HttpRequest.cs
- XamlTreeBuilder.cs
- IdentityReference.cs
- ValueQuery.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- OutputCacheProfileCollection.cs
- SerializationHelper.cs
- NextPreviousPagerField.cs
- BitmapEffect.cs
- VariableQuery.cs
- ZoneButton.cs
- SafeUserTokenHandle.cs
- ObjectView.cs
- ReflectTypeDescriptionProvider.cs
- GridViewSortEventArgs.cs
- PointKeyFrameCollection.cs
- HttpApplicationFactory.cs
- DataGridGeneralPage.cs
- WebConfigurationManager.cs
- RoleManagerSection.cs
- ListSourceHelper.cs
- NameTable.cs
- DateTimeFormat.cs
- storepermission.cs
- SimpleWorkerRequest.cs
- QueryCursorEventArgs.cs
- FixedTextBuilder.cs
- MouseBinding.cs
- TearOffProxy.cs
- InsufficientMemoryException.cs
- TargetFrameworkAttribute.cs
- TransformerTypeCollection.cs
- SamlSerializer.cs
- RegexCompilationInfo.cs
- XmlRootAttribute.cs
- SequenceDesignerAccessibleObject.cs
- _StreamFramer.cs
- MbpInfo.cs
- SortKey.cs
- TypeToken.cs
- XmlSerializationWriter.cs
- XmlAnyAttributeAttribute.cs
- ThrowHelper.cs
- AnnotationMap.cs
- ConstructorBuilder.cs