Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / BidOverLoads.cs / 2 / BidOverLoads.cs
//------------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //----------------------------------------------------------------------------------- using System; using System.Text; using System.Security; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SecurityTreatAsSafe] internal static partial class EntityBid { private const string dllName = "System.Data.dll"; internal static bool AdvancedOn { get { return Bid.AdvancedOn; } } internal static bool TraceOn { get { return Bid.TraceOn; } } #region PutStr internal static void PutStr(string str) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.PutStr(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, str); } internal static void PutStrChunked(System.String str) { // It sure would be nice if this were something that didn't have to be chunked... if (Bid.TraceOn && Bid.IsInitialized) { RawPutStrChuncked(str); } } private static void RawPutStrChuncked(System.String str) { for (int i = 0; i < str.Length; i += 1000) { NativeMethods.PutStr(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, str.Substring(i, Math.Min(str.Length - i, 1000))); } } #endregion #region PlanCompiler internal static bool PlanCompilerOn { get { return Bid.IsOn(System.Data.Query.PlanCompiler.PlanCompiler.PlanCompilerTracePoints) && (Bid.IsInitialized); } } internal static void PlanCompilerPutStr(System.String str) { if (PlanCompilerOn) { RawPutStrChuncked(str); } } internal static void PlanCompilerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (PlanCompilerOn) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } #endregion #region ScopeAuto internal struct ScopeAuto : IDisposable { internal ScopeAuto(string fmtPrintfW, System.Int32 arg) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out _hscp, fmtPrintfW, arg); } else { _hscp = Bid.NoData; } } internal ScopeAuto(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out _hscp, fmtPrintfW, a1, a2); } else { _hscp = Bid.NoData; } } public void Dispose() { if (Bid.ScopeOn && Bid.IsInitialized && _hscp != Bid.NoData) { NativeMethods.ScopeLeave(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, ref _hscp); } // NOTE: In contrast with standalone ScopeLeave, // there is no need to assign "Bid.NoData" to _hscp. } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] private IntPtr _hscp; } #endregion #region ScopeEnter with 1 parameters internal static void ScopeEnter(out IntPtr hScp, System.String strConst) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out hScp, strConst); } else { hScp = Bid.NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1); } else { hScp = Bid.NoData; } } #endregion #region ScopeEnter with 2 parameters internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if (Bid.ScopeOn && Bid.IsInitialized) NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, a2); else hScp = Bid.NoData; } #endregion #region ScopeLeave internal static void ScopeLeave(ref IntPtr hScp) { Bid.ScopeLeave(ref hScp); } #endregion #region Trace with 1 parameter internal static void Trace(string strConst) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, strConst); } internal static void Trace(string fmtPrintfW, System.Boolean a1) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void Trace(string fmtPrintfW, System.Int32 a1) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void Trace(string fmtPrintfW, System.String a1) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } #endregion #region Trace with 2 parameters internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void Trace(string fmtPrintfW, System.String a1, System.String a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } #endregion #region Trace with 3 parameters internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2, a3); } #endregion // // Interop calls to pluggable hooks [SuppressUnmanagedCodeSecurity] applied // [SuppressUnmanagedCodeSecurity] private static partial class NativeMethods { #region PutStr [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.StdCall, EntryPoint = "DllBidPutStrW")] extern internal static void PutStr(IntPtr hID, UIntPtr src, UIntPtr info, string str); #endregion #region ScopeEnter with 1 parameter [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string strConst); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1); #endregion #region ScopeEnter with 2 parameters [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2); #endregion #region ScopeLeave [DllImport(dllName, EntryPoint = "DllBidScopeLeave")] extern internal static void ScopeLeave(IntPtr hID, UIntPtr src, UIntPtr info, ref IntPtr hScp); #endregion #region Trace with 1 parameter [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string strConst); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1); #endregion #region Trace with 2 parameters [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int64 a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.String a2); #endregion #region Trace with 3 parameters [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3); #endregion #region Trace with 4 parameters [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //----------------------------------------------------------------------------------- using System; using System.Text; using System.Security; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SecurityTreatAsSafe] internal static partial class EntityBid { private const string dllName = "System.Data.dll"; internal static bool AdvancedOn { get { return Bid.AdvancedOn; } } internal static bool TraceOn { get { return Bid.TraceOn; } } #region PutStr internal static void PutStr(string str) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.PutStr(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, str); } internal static void PutStrChunked(System.String str) { // It sure would be nice if this were something that didn't have to be chunked... if (Bid.TraceOn && Bid.IsInitialized) { RawPutStrChuncked(str); } } private static void RawPutStrChuncked(System.String str) { for (int i = 0; i < str.Length; i += 1000) { NativeMethods.PutStr(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, str.Substring(i, Math.Min(str.Length - i, 1000))); } } #endregion #region PlanCompiler internal static bool PlanCompilerOn { get { return Bid.IsOn(System.Data.Query.PlanCompiler.PlanCompiler.PlanCompilerTracePoints) && (Bid.IsInitialized); } } internal static void PlanCompilerPutStr(System.String str) { if (PlanCompilerOn) { RawPutStrChuncked(str); } } internal static void PlanCompilerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (PlanCompilerOn) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } #endregion #region ScopeAuto internal struct ScopeAuto : IDisposable { internal ScopeAuto(string fmtPrintfW, System.Int32 arg) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out _hscp, fmtPrintfW, arg); } else { _hscp = Bid.NoData; } } internal ScopeAuto(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out _hscp, fmtPrintfW, a1, a2); } else { _hscp = Bid.NoData; } } public void Dispose() { if (Bid.ScopeOn && Bid.IsInitialized && _hscp != Bid.NoData) { NativeMethods.ScopeLeave(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, ref _hscp); } // NOTE: In contrast with standalone ScopeLeave, // there is no need to assign "Bid.NoData" to _hscp. } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] private IntPtr _hscp; } #endregion #region ScopeEnter with 1 parameters internal static void ScopeEnter(out IntPtr hScp, System.String strConst) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out hScp, strConst); } else { hScp = Bid.NoData; } } internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1) { if (Bid.ScopeOn && Bid.IsInitialized) { NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1); } else { hScp = Bid.NoData; } } #endregion #region ScopeEnter with 2 parameters internal static void ScopeEnter(out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if (Bid.ScopeOn && Bid.IsInitialized) NativeMethods.ScopeEnter(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, out hScp, fmtPrintfW, a1, a2); else hScp = Bid.NoData; } #endregion #region ScopeLeave internal static void ScopeLeave(ref IntPtr hScp) { Bid.ScopeLeave(ref hScp); } #endregion #region Trace with 1 parameter internal static void Trace(string strConst) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, strConst); } internal static void Trace(string fmtPrintfW, System.Boolean a1) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void Trace(string fmtPrintfW, System.Int32 a1) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } internal static void Trace(string fmtPrintfW, System.String a1) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } #endregion #region Trace with 2 parameters internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Boolean a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } internal static void Trace(string fmtPrintfW, System.String a1, System.String a2) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } #endregion #region Trace with 3 parameters internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if (Bid.TraceOn && Bid.IsInitialized) NativeMethods.Trace(Bid.ID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2, a3); } #endregion // // Interop calls to pluggable hooks [SuppressUnmanagedCodeSecurity] applied // [SuppressUnmanagedCodeSecurity] private static partial class NativeMethods { #region PutStr [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.StdCall, EntryPoint = "DllBidPutStrW")] extern internal static void PutStr(IntPtr hID, UIntPtr src, UIntPtr info, string str); #endregion #region ScopeEnter with 1 parameter [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string strConst); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1); #endregion #region ScopeEnter with 2 parameters [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidScopeEnterCW")] extern internal static void ScopeEnter(IntPtr hID, UIntPtr src, UIntPtr info, out IntPtr hScp, string fmtPrintfW, System.Int32 a1, System.Int32 a2); #endregion #region ScopeLeave [DllImport(dllName, EntryPoint = "DllBidScopeLeave")] extern internal static void ScopeLeave(IntPtr hID, UIntPtr src, UIntPtr info, ref IntPtr hScp); #endregion #region Trace with 1 parameter [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string strConst); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Boolean a1); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1); #endregion #region Trace with 2 parameters [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Boolean a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int64 a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.String a2); #endregion #region Trace with 3 parameters [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3); [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3); #endregion #region Trace with 4 parameters [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
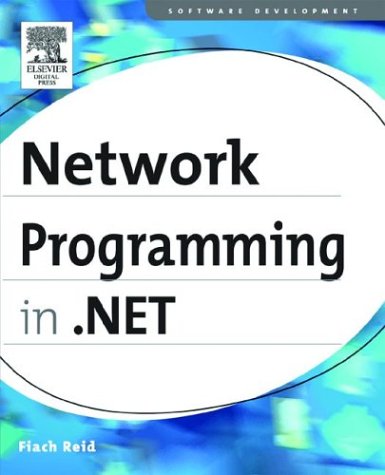
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Ipv6Element.cs
- SharedDp.cs
- ExchangeUtilities.cs
- MdbDataFileEditor.cs
- SiteMapSection.cs
- TextFormatterImp.cs
- DataGridRowsPresenter.cs
- SelectionWordBreaker.cs
- DataServiceHost.cs
- HttpSessionStateWrapper.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- SerialErrors.cs
- MailAddressParser.cs
- RangeContentEnumerator.cs
- RequestContext.cs
- ResXResourceSet.cs
- FormattedText.cs
- XamlStackWriter.cs
- WebEventTraceProvider.cs
- Grant.cs
- ControlCollection.cs
- TypeInitializationException.cs
- ServerType.cs
- FormsAuthenticationTicket.cs
- TimeEnumHelper.cs
- XamlTreeBuilder.cs
- OpenTypeCommon.cs
- NavigationPropertySingletonExpression.cs
- DrawingContextWalker.cs
- Effect.cs
- XmlTextWriter.cs
- EntityType.cs
- ReadOnlyDataSource.cs
- MsmqProcessProtocolHandler.cs
- InvalidDataContractException.cs
- ProviderIncompatibleException.cs
- EventDescriptorCollection.cs
- BindingsCollection.cs
- MetricEntry.cs
- TypedTableBaseExtensions.cs
- ExpressionSelection.cs
- LightweightCodeGenerator.cs
- Tuple.cs
- X509Extension.cs
- BitmapCodecInfoInternal.cs
- SyndicationLink.cs
- AnonymousIdentificationModule.cs
- DataObjectEventArgs.cs
- GridViewDeleteEventArgs.cs
- Condition.cs
- Overlapped.cs
- ConfigurationConverterBase.cs
- AnimationStorage.cs
- HttpResponseBase.cs
- RawStylusActions.cs
- DefaultSection.cs
- UmAlQuraCalendar.cs
- WindowsNonControl.cs
- FileCodeGroup.cs
- ContentPosition.cs
- AsyncResult.cs
- DataGridViewRowPrePaintEventArgs.cs
- ForwardPositionQuery.cs
- BamlLocalizableResource.cs
- TextSchema.cs
- EncryptedType.cs
- UnsafeNativeMethods.cs
- SkipQueryOptionExpression.cs
- InkCanvasFeedbackAdorner.cs
- NumberAction.cs
- Rule.cs
- BinaryUtilClasses.cs
- SafeLibraryHandle.cs
- Assembly.cs
- ExecutionContext.cs
- Size.cs
- XmlResolver.cs
- PolygonHotSpot.cs
- XmlSchemaAll.cs
- DecimalAnimationUsingKeyFrames.cs
- WebSysDisplayNameAttribute.cs
- WebPartZone.cs
- GAC.cs
- TagMapCollection.cs
- Win32SafeHandles.cs
- DiffuseMaterial.cs
- TextEditorMouse.cs
- EntityContainerEmitter.cs
- RegionData.cs
- SimpleBitVector32.cs
- _DisconnectOverlappedAsyncResult.cs
- RegisteredExpandoAttribute.cs
- DataObjectPastingEventArgs.cs
- AttributeEmitter.cs
- TransformerConfigurationWizardBase.cs
- ScaleTransform3D.cs
- DBSqlParserTable.cs
- SerializableTypeCodeDomSerializer.cs
- ProjectionNode.cs
- SqlNotificationRequest.cs