Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Reflection / Emit / Opcode.cs / 1305376 / Opcode.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] namespace System.Reflection.Emit { using System; using System.Security.Permissions; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public struct OpCode { internal String m_stringname; internal StackBehaviour m_pop; internal StackBehaviour m_push; internal OperandType m_operand; internal OpCodeType m_type; internal int m_size; internal byte m_s1; internal byte m_s2; internal FlowControl m_ctrl; // Specifies whether the current instructions causes the control flow to // change unconditionally. internal bool m_endsUncondJmpBlk; // Specifies the stack change that the current instruction causes not // taking into account the operand dependant stack changes. internal int m_stackChange; internal OpCode(String stringname, StackBehaviour pop, StackBehaviour push, OperandType operand, OpCodeType type, int size, byte s1, byte s2, FlowControl ctrl, bool endsjmpblk, int stack) { m_stringname = stringname; m_pop = pop; m_push = push; m_operand = operand; m_type = type; m_size = size; m_s1 = s1; m_s2 = s2; m_ctrl = ctrl; m_endsUncondJmpBlk = endsjmpblk; m_stackChange = stack; } internal bool EndsUncondJmpBlk() { return m_endsUncondJmpBlk; } internal int StackChange() { return m_stackChange; } public OperandType OperandType { get { return (m_operand); } } public FlowControl FlowControl { get { return (m_ctrl); } } public OpCodeType OpCodeType { get { return (m_type); } } public StackBehaviour StackBehaviourPop { get { return (m_pop); } } public StackBehaviour StackBehaviourPush { get { return (m_push); } } public int Size { get { return (m_size); } } public short Value { get { if (m_size == 2) return (short) (m_s1 << 8 | m_s2); return (short) m_s2; } } public String Name { get { return m_stringname; } } [Pure] public override bool Equals(Object obj) { if (obj is OpCode) return Equals((OpCode)obj); else return false; } [Pure] public bool Equals(OpCode obj) { return obj.m_s1 == m_s1 && obj.m_s2 == m_s2; } [Pure] public static bool operator ==(OpCode a, OpCode b) { return a.Equals(b); } [Pure] public static bool operator !=(OpCode a, OpCode b) { return !(a == b); } public override int GetHashCode() { return this.m_stringname.GetHashCode(); } public override String ToString() { return m_stringname; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
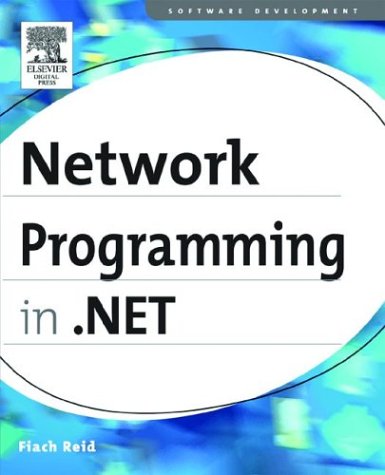
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowPatternIdentifiers.cs
- WinInet.cs
- RequiredFieldValidator.cs
- SessionParameter.cs
- SqlBuilder.cs
- CodeDomSerializationProvider.cs
- Variable.cs
- DebuggerAttributes.cs
- GeneralTransformGroup.cs
- EdmComplexPropertyAttribute.cs
- WebPartVerb.cs
- CultureInfo.cs
- ExtendedPropertyCollection.cs
- dataprotectionpermission.cs
- HashHelper.cs
- PropertyIDSet.cs
- DesignerSerializationOptionsAttribute.cs
- Command.cs
- Line.cs
- Parameter.cs
- SchemaImporterExtensionElement.cs
- MembershipSection.cs
- TextTreeFixupNode.cs
- PropertyChangingEventArgs.cs
- SpeakProgressEventArgs.cs
- FocusManager.cs
- PassportPrincipal.cs
- MetaModel.cs
- InkCanvasInnerCanvas.cs
- ProjectionQueryOptionExpression.cs
- DataListGeneralPage.cs
- DataSourceExpression.cs
- NavigationWindow.cs
- ScriptingJsonSerializationSection.cs
- InitializationEventAttribute.cs
- MergeFilterQuery.cs
- ConditionalExpression.cs
- ComponentConverter.cs
- HelpKeywordAttribute.cs
- AesManaged.cs
- GeneralTransform.cs
- EdgeModeValidation.cs
- SqlGenerator.cs
- ScopedMessagePartSpecification.cs
- TypeForwardedToAttribute.cs
- RefExpr.cs
- FontSourceCollection.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- ManagementClass.cs
- XmlSchemaParticle.cs
- Validator.cs
- ListBoxItem.cs
- SrgsSemanticInterpretationTag.cs
- Helper.cs
- X509SecurityToken.cs
- _Connection.cs
- CorruptStoreException.cs
- CacheOutputQuery.cs
- WebSysDisplayNameAttribute.cs
- AccessViolationException.cs
- PerfProviderCollection.cs
- TransactionTraceIdentifier.cs
- OdbcUtils.cs
- TextContainerChangeEventArgs.cs
- BoolExpressionVisitors.cs
- FixedHyperLink.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- EastAsianLunisolarCalendar.cs
- DataGridDetailsPresenterAutomationPeer.cs
- CatalogPart.cs
- PrinterResolution.cs
- ApplyImportsAction.cs
- ErrorHandler.cs
- WorkflowLayouts.cs
- Aggregates.cs
- ComplexType.cs
- SynchronizedInputAdaptor.cs
- IncrementalCompileAnalyzer.cs
- ThreadInterruptedException.cs
- Model3D.cs
- SamlAttribute.cs
- Rotation3DKeyFrameCollection.cs
- PrimitiveDataContract.cs
- DataBindingExpressionBuilder.cs
- CategoryAttribute.cs
- GridViewUpdatedEventArgs.cs
- UTF8Encoding.cs
- IsolatedStorageException.cs
- SetIterators.cs
- RectConverter.cs
- VisualStyleInformation.cs
- AutoResetEvent.cs
- ConnectionPoint.cs
- WinInet.cs
- ElementsClipboardData.cs
- RectangleGeometry.cs
- DataSetViewSchema.cs
- OleDbFactory.cs
- AgileSafeNativeMemoryHandle.cs
- QueryOperator.cs