Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Shared / MS / Internal / HashHelper.cs / 1 / HashHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Static class to help work around hashing-related bugs. // //--------------------------------------------------------------------------- using System; using MS.Internal; // BaseHashHelper #if WINDOWS_BASE namespace MS.Internal.Hashing.WindowsBase #elif PRESENTATION_CORE namespace MS.Internal.Hashing.PresentationCore #elif PRESENTATIONFRAMEWORK using System.ComponentModel; // ICustomTypeDescriptor namespace MS.Internal.Hashing.PresentationFramework #else #error Attempt to define HashHelper in an unknown assembly. namespace MS.Internal.YourAssemblyName #endif { internal static class HashHelper { // The class cctor registers this assembly's exceptional types with // the base helper. static HashHelper() { Initialize(); // this makes FxCop happy - otherwise Initialize is "unused code" Type[] types = new Type[] { #if WINDOWS_BASE #elif PRESENTATION_CORE typeof(System.Windows.Media.CharacterMetrics), // bug 1612093 typeof(System.Windows.Ink.ExtendedProperty), // bug 1612101 typeof(System.Windows.Media.FamilyTypeface), // bug 1612103 typeof(System.Windows.Media.NumberSubstitution), // bug 1612105 #elif PRESENTATIONFRAMEWORK typeof(System.Windows.Markup.Localizer.BamlLocalizableResource), // bug 1612118 typeof(System.Windows.ComponentResourceKey), // bug 1612119 #endif }; BaseHashHelper.RegisterTypes(typeof(HashHelper).Assembly, types); // initialize lower-level assemblies #if PRESENTATIONFRAMEWORK MS.Internal.Hashing.PresentationCore.HashHelper.Initialize(); #endif } // certain objects don't have reliable hashcodes, and cannot be used // within a Hashtable, Dictionary, etc. internal static bool HasReliableHashCode(object item) { return BaseHashHelper.HasReliableHashCode(item); } // this method doesn't do anything, but calling it makes sure the static // cctor gets called internal static void Initialize() { } #if PRESENTATIONFRAMEWORK // Helper to identify DataRowView internal static bool IsDataRowView(object item, Type type) { // the earlier tests filter out most false results before doing // the final test. If we did the final test first, it would load // System.Data unnecessarily. return (item is ICustomTypeDescriptor && item is IDataErrorInfo && type.Namespace == "System.Data" && IsDataRowViewType(type)); } // separate method to avoid loading System.Data until needed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static bool IsDataRowViewType(Type type) { return (type == typeof(System.Data.DataRowView)); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Static class to help work around hashing-related bugs. // //--------------------------------------------------------------------------- using System; using MS.Internal; // BaseHashHelper #if WINDOWS_BASE namespace MS.Internal.Hashing.WindowsBase #elif PRESENTATION_CORE namespace MS.Internal.Hashing.PresentationCore #elif PRESENTATIONFRAMEWORK using System.ComponentModel; // ICustomTypeDescriptor namespace MS.Internal.Hashing.PresentationFramework #else #error Attempt to define HashHelper in an unknown assembly. namespace MS.Internal.YourAssemblyName #endif { internal static class HashHelper { // The class cctor registers this assembly's exceptional types with // the base helper. static HashHelper() { Initialize(); // this makes FxCop happy - otherwise Initialize is "unused code" Type[] types = new Type[] { #if WINDOWS_BASE #elif PRESENTATION_CORE typeof(System.Windows.Media.CharacterMetrics), // bug 1612093 typeof(System.Windows.Ink.ExtendedProperty), // bug 1612101 typeof(System.Windows.Media.FamilyTypeface), // bug 1612103 typeof(System.Windows.Media.NumberSubstitution), // bug 1612105 #elif PRESENTATIONFRAMEWORK typeof(System.Windows.Markup.Localizer.BamlLocalizableResource), // bug 1612118 typeof(System.Windows.ComponentResourceKey), // bug 1612119 #endif }; BaseHashHelper.RegisterTypes(typeof(HashHelper).Assembly, types); // initialize lower-level assemblies #if PRESENTATIONFRAMEWORK MS.Internal.Hashing.PresentationCore.HashHelper.Initialize(); #endif } // certain objects don't have reliable hashcodes, and cannot be used // within a Hashtable, Dictionary, etc. internal static bool HasReliableHashCode(object item) { return BaseHashHelper.HasReliableHashCode(item); } // this method doesn't do anything, but calling it makes sure the static // cctor gets called internal static void Initialize() { } #if PRESENTATIONFRAMEWORK // Helper to identify DataRowView internal static bool IsDataRowView(object item, Type type) { // the earlier tests filter out most false results before doing // the final test. If we did the final test first, it would load // System.Data unnecessarily. return (item is ICustomTypeDescriptor && item is IDataErrorInfo && type.Namespace == "System.Data" && IsDataRowViewType(type)); } // separate method to avoid loading System.Data until needed [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static bool IsDataRowViewType(Type type) { return (type == typeof(System.Data.DataRowView)); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
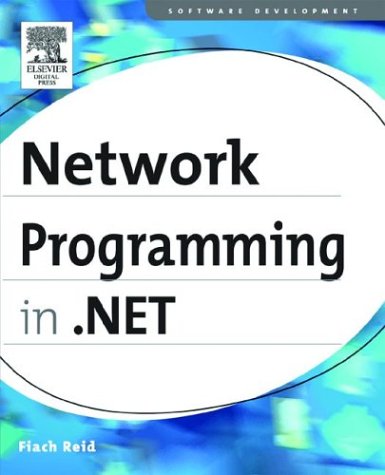
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HScrollProperties.cs
- LogPolicy.cs
- GraphicsState.cs
- TypeExtensions.cs
- _ConnectStream.cs
- ThrowHelper.cs
- PrintDocument.cs
- ServiceThrottlingElement.cs
- GridItemProviderWrapper.cs
- CounterSampleCalculator.cs
- Pkcs9Attribute.cs
- WindowsFormsHostAutomationPeer.cs
- RegexCapture.cs
- SqlCommandBuilder.cs
- ExpressionReplacer.cs
- TimelineCollection.cs
- SettingsPropertyNotFoundException.cs
- EventEntry.cs
- BaseTemplatedMobileComponentEditor.cs
- SqlBulkCopyColumnMapping.cs
- DataMember.cs
- QilExpression.cs
- DefinitionBase.cs
- DBCommand.cs
- PointUtil.cs
- ContractBase.cs
- WebDisplayNameAttribute.cs
- WSDualHttpSecurityMode.cs
- RSAPKCS1SignatureDeformatter.cs
- KeyNotFoundException.cs
- LinkAreaEditor.cs
- LayoutInformation.cs
- EntityDataSourceQueryBuilder.cs
- XmlCompatibilityReader.cs
- TypeNameHelper.cs
- DynamicResourceExtension.cs
- RSAPKCS1SignatureDeformatter.cs
- IisTraceListener.cs
- ConfigurationValidatorBase.cs
- DecoderBestFitFallback.cs
- Point3DAnimationBase.cs
- DrawListViewSubItemEventArgs.cs
- GuidelineCollection.cs
- FrameworkTemplate.cs
- IgnoreFlushAndCloseStream.cs
- TaskFileService.cs
- MessageBuilder.cs
- MyContact.cs
- RoleService.cs
- ObjectParameterCollection.cs
- documentsequencetextpointer.cs
- ElementUtil.cs
- HttpWebResponse.cs
- FontInfo.cs
- PagesChangedEventArgs.cs
- XsdDuration.cs
- Keywords.cs
- BaseTemplateParser.cs
- ConfigurationLockCollection.cs
- CategoryGridEntry.cs
- RangeValuePattern.cs
- ClaimComparer.cs
- DataListItemCollection.cs
- SqlNotificationEventArgs.cs
- RemoteCryptoSignHashRequest.cs
- MetadataUtilsSmi.cs
- BasicKeyConstraint.cs
- SBCSCodePageEncoding.cs
- FixedPageAutomationPeer.cs
- HostedImpersonationContext.cs
- WebRequestModulesSection.cs
- Visitors.cs
- wmiprovider.cs
- ExpandCollapseProviderWrapper.cs
- EditingCoordinator.cs
- PropertyDescriptor.cs
- OracleBinary.cs
- CompiledXpathExpr.cs
- CheckBox.cs
- XmlDataSource.cs
- SoapAttributes.cs
- TriggerActionCollection.cs
- isolationinterop.cs
- PropertyBuilder.cs
- peernodestatemanager.cs
- EditorZoneBase.cs
- Pkcs7Signer.cs
- WbmpConverter.cs
- TextReturnReader.cs
- SqlInternalConnectionSmi.cs
- ExecutionEngineException.cs
- GeneratedCodeAttribute.cs
- UrlMappingsModule.cs
- DefaultTextStoreTextComposition.cs
- RegisteredExpandoAttribute.cs
- RoleManagerModule.cs
- XmlMembersMapping.cs
- BufferedGraphicsManager.cs
- ListViewAutomationPeer.cs
- UnaryExpression.cs