Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Data / System / Data / Common / DBCommand.cs / 1 / DBCommand.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.ComponentModel; using System.Data; #if WINFSInternalOnly internal #else public #endif abstract class DbCommand : Component, IDbCommand { // V1.2.3300 protected DbCommand() : base() { } [ DefaultValue(""), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandText), ] abstract public string CommandText { get; set; } [ ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandTimeout), ] abstract public int CommandTimeout { get; set; } [ DefaultValue(System.Data.CommandType.Text), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandType), ] abstract public CommandType CommandType { get; set; } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Connection), ] public DbConnection Connection { get { return DbConnection; } set { DbConnection = value; } } IDbConnection IDbCommand.Connection { get { return DbConnection; } set { DbConnection = (DbConnection)value; } } abstract protected DbConnection DbConnection { // V1.2.3300 get; set; } abstract protected DbParameterCollection DbParameterCollection { // V1.2.3300 get; } abstract protected DbTransaction DbTransaction { // V1.2.3300 get; set; } // @devnote: By default, the cmd object is visible on the design surface (i.e. VS7 Server Tray) // to limit the number of components that clutter the design surface, // when the DataAdapter design wizard generates the insert/update/delete commands it will // set the DesignTimeVisible property to false so that cmds won't appear as individual objects [ DefaultValue(true), DesignOnly(true), Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never), ] public abstract bool DesignTimeVisible { get; set; } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Parameters), ] public DbParameterCollection Parameters { get { return DbParameterCollection; } } IDataParameterCollection IDbCommand.Parameters { get { return (DbParameterCollection)DbParameterCollection; } } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.DbCommand_Transaction), ] public DbTransaction Transaction { get { return DbTransaction; } set { DbTransaction = value; } } IDbTransaction IDbCommand.Transaction { get { return DbTransaction; } set { DbTransaction = (DbTransaction)value; } } [ DefaultValue(System.Data.UpdateRowSource.Both), ResCategoryAttribute(Res.DataCategory_Update), ResDescriptionAttribute(Res.DbCommand_UpdatedRowSource), ] abstract public UpdateRowSource UpdatedRowSource { get; set; } abstract public void Cancel(); public DbParameter CreateParameter(){ // V1.2.3300 return CreateDbParameter(); } IDbDataParameter IDbCommand.CreateParameter() { // V1.2.3300 return CreateDbParameter(); } abstract protected DbParameter CreateDbParameter(); abstract protected DbDataReader ExecuteDbDataReader(CommandBehavior behavior); abstract public int ExecuteNonQuery(); public DbDataReader ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } IDataReader IDbCommand.ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } public DbDataReader ExecuteReader(CommandBehavior behavior){ return (DbDataReader)ExecuteDbDataReader(behavior); } IDataReader IDbCommand.ExecuteReader(CommandBehavior behavior) { return (DbDataReader)ExecuteDbDataReader(behavior); } abstract public object ExecuteScalar(); abstract public void Prepare(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.ComponentModel; using System.Data; #if WINFSInternalOnly internal #else public #endif abstract class DbCommand : Component, IDbCommand { // V1.2.3300 protected DbCommand() : base() { } [ DefaultValue(""), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandText), ] abstract public string CommandText { get; set; } [ ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandTimeout), ] abstract public int CommandTimeout { get; set; } [ DefaultValue(System.Data.CommandType.Text), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_CommandType), ] abstract public CommandType CommandType { get; set; } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Connection), ] public DbConnection Connection { get { return DbConnection; } set { DbConnection = value; } } IDbConnection IDbCommand.Connection { get { return DbConnection; } set { DbConnection = (DbConnection)value; } } abstract protected DbConnection DbConnection { // V1.2.3300 get; set; } abstract protected DbParameterCollection DbParameterCollection { // V1.2.3300 get; } abstract protected DbTransaction DbTransaction { // V1.2.3300 get; set; } // @devnote: By default, the cmd object is visible on the design surface (i.e. VS7 Server Tray) // to limit the number of components that clutter the design surface, // when the DataAdapter design wizard generates the insert/update/delete commands it will // set the DesignTimeVisible property to false so that cmds won't appear as individual objects [ DefaultValue(true), DesignOnly(true), Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never), ] public abstract bool DesignTimeVisible { get; set; } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbCommand_Parameters), ] public DbParameterCollection Parameters { get { return DbParameterCollection; } } IDataParameterCollection IDbCommand.Parameters { get { return (DbParameterCollection)DbParameterCollection; } } [ Browsable(false), DefaultValue(null), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.DbCommand_Transaction), ] public DbTransaction Transaction { get { return DbTransaction; } set { DbTransaction = value; } } IDbTransaction IDbCommand.Transaction { get { return DbTransaction; } set { DbTransaction = (DbTransaction)value; } } [ DefaultValue(System.Data.UpdateRowSource.Both), ResCategoryAttribute(Res.DataCategory_Update), ResDescriptionAttribute(Res.DbCommand_UpdatedRowSource), ] abstract public UpdateRowSource UpdatedRowSource { get; set; } abstract public void Cancel(); public DbParameter CreateParameter(){ // V1.2.3300 return CreateDbParameter(); } IDbDataParameter IDbCommand.CreateParameter() { // V1.2.3300 return CreateDbParameter(); } abstract protected DbParameter CreateDbParameter(); abstract protected DbDataReader ExecuteDbDataReader(CommandBehavior behavior); abstract public int ExecuteNonQuery(); public DbDataReader ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } IDataReader IDbCommand.ExecuteReader() { return (DbDataReader)ExecuteDbDataReader(CommandBehavior.Default); } public DbDataReader ExecuteReader(CommandBehavior behavior){ return (DbDataReader)ExecuteDbDataReader(behavior); } IDataReader IDbCommand.ExecuteReader(CommandBehavior behavior) { return (DbDataReader)ExecuteDbDataReader(behavior); } abstract public object ExecuteScalar(); abstract public void Prepare(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
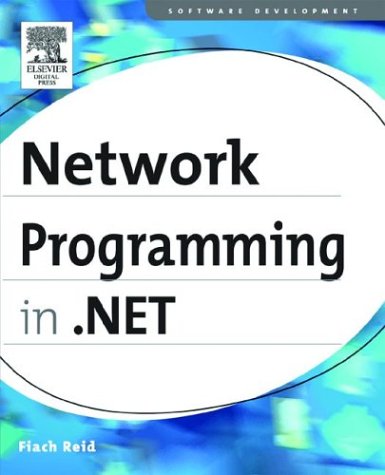
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClassData.cs
- SupportsEventValidationAttribute.cs
- WebPartMenu.cs
- Mouse.cs
- GeneralTransform3DCollection.cs
- ListGeneralPage.cs
- SslStream.cs
- BinaryUtilClasses.cs
- FilterEventArgs.cs
- RemoteHelper.cs
- XmlLoader.cs
- AccessibleObject.cs
- SafeLibraryHandle.cs
- RedistVersionInfo.cs
- XmlNodeComparer.cs
- MsmqInputSessionChannel.cs
- SQLCharsStorage.cs
- Inline.cs
- TrackingAnnotationCollection.cs
- XmlSerializer.cs
- Int32KeyFrameCollection.cs
- BaseParser.cs
- BinaryFormatter.cs
- SamlEvidence.cs
- BaseWebProxyFinder.cs
- CanonicalFontFamilyReference.cs
- Vector3DCollectionValueSerializer.cs
- LinqDataSourceInsertEventArgs.cs
- StructuralType.cs
- OleDbCommand.cs
- AssemblyBuilder.cs
- RuntimeConfig.cs
- CallContext.cs
- DrawListViewSubItemEventArgs.cs
- MatrixStack.cs
- FontUnit.cs
- CodeMemberMethod.cs
- BinaryFormatterWriter.cs
- DataGridViewUtilities.cs
- SoapParser.cs
- DbMetaDataCollectionNames.cs
- LOSFormatter.cs
- WebPartTransformerCollection.cs
- HttpModuleActionCollection.cs
- XmlValidatingReader.cs
- RectangleGeometry.cs
- ToolStripOverflowButton.cs
- Object.cs
- KnownIds.cs
- ADRoleFactoryConfiguration.cs
- PageContentCollection.cs
- XmlImplementation.cs
- ActivityInfo.cs
- Converter.cs
- TagPrefixAttribute.cs
- FactoryId.cs
- FreezableDefaultValueFactory.cs
- HyperLinkField.cs
- HeaderCollection.cs
- ListViewPagedDataSource.cs
- ItemList.cs
- StringStorage.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- _ProxyChain.cs
- streamingZipPartStream.cs
- Hashtable.cs
- CompModSwitches.cs
- SaveFileDialog.cs
- DesignSurfaceCollection.cs
- DataGridItemAttachedStorage.cs
- FacetChecker.cs
- xamlnodes.cs
- SubpageParagraph.cs
- PathFigure.cs
- SchemaCollectionPreprocessor.cs
- PrintEvent.cs
- HtmlInputText.cs
- HttpSocketManager.cs
- NegotiationTokenAuthenticatorState.cs
- Schema.cs
- DefaultExpressionVisitor.cs
- tabpagecollectioneditor.cs
- NamespaceDecl.cs
- HtmlGenericControl.cs
- ProfileService.cs
- SQLSingle.cs
- Debugger.cs
- FixedPageProcessor.cs
- Decimal.cs
- HttpResponseHeader.cs
- Brush.cs
- HttpModuleCollection.cs
- MulticastNotSupportedException.cs
- COAUTHIDENTITY.cs
- CodeCatchClauseCollection.cs
- MissingFieldException.cs
- DelegatingConfigHost.cs
- MetabaseSettingsIis7.cs
- HandleExceptionArgs.cs
- LiteralTextContainerControlBuilder.cs