Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / ManagementClass.cs / 1305376 / ManagementClass.cs
using System; using System.Collections.Specialized; using System.Runtime.InteropServices; using System.Runtime.Serialization; using WbemClient_v1; using System.CodeDom; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// ///Represents a CIM management class from WMI. CIM (Common Information Model) classes /// represent management information including hardware, software, processes, etc. /// For more information about the CIM classes available in Windows search for ‘win32 classes’. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// [Serializable] public class ManagementClass : ManagementObject { private MethodDataCollection methods; protected override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData ( info, context ) ; } ///using System; /// using System.Management; /// /// // This example demonstrates getting information about a class using the ManagementClass object /// class Sample_ManagementClass /// { /// public static int Main(string[] args) { /// ManagementClass diskClass = new ManagementClass("Win32_LogicalDisk"); /// diskClass.Get(); /// Console.WriteLine("Logical Disk class has " + diskClass.Properties.Count + " properties"); /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This example demonstrates getting information about a class using the ManagementClass object /// Class Sample_ManagementClass /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("Win32_LogicalDisk") /// diskClass.Get() /// Console.WriteLine(("Logical Disk class has " & _ /// diskClass.Properties.Count.ToString() & _ /// " properties")) /// Return 0 /// End Function /// End Class ///
////// Internal factory for classes, used when deriving a class /// or cloning a class. For these purposes we always mark /// the class as "bound". /// /// The underlying WMI object /// Seed class from which we will get initialization info internal static ManagementClass GetManagementClass( IWbemClassObjectFreeThreaded wbemObject, ManagementClass mgObj) { ManagementClass newClass = new ManagementClass(); newClass.wbemObject = wbemObject; if (null != mgObj) { newClass.scope = ManagementScope._Clone(mgObj.scope); ManagementPath objPath = mgObj.Path; if (null != objPath) newClass.path = ManagementPath._Clone(objPath); // Ensure we have our class name in the path object className = null; int dummy = 0; int status = wbemObject.Get_("__CLASS", 0, ref className, ref dummy, ref dummy); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } if (className != System.DBNull.Value) newClass.path.internalClassName = (string)className; ObjectGetOptions options = mgObj.Options; if (null != options) newClass.options = ObjectGetOptions._Clone(options); // Finally we ensure that this object is marked as bound. // We do this as a last step since setting certain properties // (Options, Path and Scope) would mark it as unbound // // *** // * Changed isBound flag to wbemObject==null check. // * newClass.IsBound = true; // *** } return newClass; } internal static ManagementClass GetManagementClass( IWbemClassObjectFreeThreaded wbemObject, ManagementScope scope) { ManagementClass newClass = new ManagementClass(); newClass.path = new ManagementPath(ManagementPath.GetManagementPath(wbemObject)); if (null != scope) newClass.scope = ManagementScope._Clone(scope); newClass.wbemObject = wbemObject; return newClass; } //default constructor ////// Initializes a new instance /// of the ///class. /// /// ///Initializes a new instance of the ///class. This is the /// default constructor. /// public ManagementClass() : this ((ManagementScope)null, (ManagementPath)null, null) {} //parameterized constructors ///ManagementClass c = new ManagementClass(); ///
///Dim c As New ManagementClass() ///
////// /// AInitializes a new instance of the ///class initialized to the /// given path. specifying which WMI class to bind to. /// /// ///The ///parameter must specify a WMI class /// path. /// public ManagementClass(ManagementPath path) : this(null, path, null) {} ///ManagementClass c = new ManagementClass( /// new ManagementPath("Win32_LogicalDisk")); ///
///Dim c As New ManagementClass( _ /// New ManagementPath("Win32_LogicalDisk")) ///
////// /// The path to the WMI class. ///Initializes a new instance of the ///class initialized to the given path. /// public ManagementClass(string path) : this(null, new ManagementPath(path), null) {} ///ManagementClass c = new /// ManagementClass("Win32_LogicalDisk"); ///
///Dim c As New ManagementClass("Win32_LogicalDisk") ///
////// /// AInitializes a new instance of the ///class initialized to the /// given WMI class path using the specified options. representing the WMI class path. /// An representing the options to use when retrieving this class. /// /// public ManagementClass(ManagementPath path, ObjectGetOptions options) : this(null, path, options) {} ///ManagementPath p = new ManagementPath("Win32_Process"); /// //Options specify that amended qualifiers are to be retrieved along with the class /// ObjectGetOptions o = new ObjectGetOptions(null, true); /// ManagementClass c = new ManagementClass(p,o); ///
///Dim p As New ManagementPath("Win32_Process") /// ' Options specify that amended qualifiers are to be retrieved along with the class /// Dim o As New ObjectGetOptions(Null, True) /// Dim c As New ManagementClass(p,o) ///
////// /// The path to the WMI class. /// AnInitializes a new instance of the ///class initialized to the given WMI class path /// using the specified options. representing the options to use when retrieving the WMI class. /// /// public ManagementClass(string path, ObjectGetOptions options) : this(null, new ManagementPath(path), options) {} /////Options specify that amended qualifiers should be retrieved along with the class /// ObjectGetOptions o = new ObjectGetOptions(null, true); /// ManagementClass c = new ManagementClass("Win32_ComputerSystem",o); ///
///' Options specify that amended qualifiers should be retrieved along with the class /// Dim o As New ObjectGetOptions(Null, True) /// Dim c As New ManagementClass("Win32_ComputerSystem",o) ///
////// /// AInitializes a new instance of the ///class for the specified /// WMI class in the specified scope and with the specified options. that specifies the scope (server and namespace) where the WMI class resides. /// A that represents the path to the WMI class in the specified scope. /// An that specifies the options to use when retrieving the WMI class. /// /// ///The path can be specified as a full /// path (including server and namespace). However, if a scope is specified, it will /// override the first portion of the full path. ////// public ManagementClass(ManagementScope scope, ManagementPath path, ObjectGetOptions options) : base (scope, path, options) {} ///ManagementScope s = new ManagementScope("\\\\MyBox\\root\\cimv2"); /// ManagementPath p = new ManagementPath("Win32_Environment"); /// ObjectGetOptions o = new ObjectGetOptions(null, true); /// ManagementClass c = new ManagementClass(s, p, o); ///
///Dim s As New ManagementScope("\\MyBox\root\cimv2") /// Dim p As New ManagementPath("Win32_Environment") /// Dim o As New ObjectGetOptions(Null, True) /// Dim c As New ManagementClass(s, p, o) ///
////// /// The scope in which the WMI class resides. /// The path to the WMI class within the specified scope. /// AnInitializes a new instance of the ///class for the specified WMI class, in the /// specified scope, and with the specified options. that specifies the options to use when retrieving the WMI class. /// /// ///The path can be specified as a full /// path (including server and namespace). However, if a scope is specified, it will /// override the first portion of the full path. ////// public ManagementClass(string scope, string path, ObjectGetOptions options) : base (new ManagementScope(scope), new ManagementPath(path), options) {} protected ManagementClass(SerializationInfo info, StreamingContext context) : base(info, context) {} ///ManagementClass c = new ManagementClass("\\\\MyBox\\root\\cimv2", /// "Win32_Environment", /// new ObjectGetOptions(null, true)); ///
///Dim c As New ManagementClass("\\MyBox\root\cimv2", _ /// "Win32_Environment", _ /// new ObjectGetOptions(Null, True)) ///
////// ///Gets or sets the path of the WMI class to /// which the ////// object is bound. /// ///The path of the object's class. ////// ///When the property is set to a new value, /// the ////// object will be /// disconnected from any previously-bound WMI class. Reconnect to the new WMI class path. /// public override ManagementPath Path { get { return base.Path; } set { // This must be a class path or empty (don't allow instance paths) if ((null == value) || value.IsClass || value.IsEmpty) base.Path = value; else throw new ArgumentOutOfRangeException("value"); } } ///ManagementClass c = new ManagementClass(); /// c.Path = "Win32_Environment"; ///
///Dim c As New ManagementClass() /// c.Path = "Win32_Environment" ///
////// ///Gets or sets an array containing all WMI classes in the /// inheritance hierarchy from this class to the top. ////// A string collection containing the /// names of all WMI classes in the inheritance hierarchy of this class. /// ////// ///This property is read-only. ////// public StringCollection Derivation { get { StringCollection result = new StringCollection(); // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; int dummy1 = 0, dummy2 = 0; object val = null; int status = wbemObject.Get_("__DERIVATION", 0, ref val, ref dummy1, ref dummy2); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } if (null != val) result.AddRange((String [])val); return result; } } ///ManagementClass c = new ManagementClass("Win32_LogicalDisk"); /// foreach (string s in c.Derivation) /// Console.WriteLine("Further derived from : ", s); ///
///Dim c As New ManagementClass("Win32_LogicalDisk") /// Dim s As String /// For Each s In c.Derivation /// Console.WriteLine("Further derived from : " & s) /// Next s ///
////// ///Gets or sets a collection of ///objects that /// represent the methods defined in the WMI class. /// ///A ///representing the methods defined in the WMI class. /// public MethodDataCollection Methods { get { Initialize ( true ) ; if (methods == null) methods = new MethodDataCollection(this); return methods; } } // //Methods // //****************************************************** //GetInstances //***************************************************** ///ManagementClass c = new ManagementClass("Win32_Process"); /// foreach (Method m in c.Methods) /// Console.WriteLine("This class contains this method : ", m.Name); ///
///Dim c As New ManagementClass("Win32_Process") /// Dim m As Method /// For Each m in c.Methods /// Console.WriteLine("This class contains this method : " & m.Name) ///
////// Returns the collection of /// all instances of the class. /// ////// ///Returns the collection of all instances of the class. ////// ///A collection of the ///objects /// representing the instances of the class. /// public ManagementObjectCollection GetInstances() { return GetInstances((EnumerationOptions)null); } ///ManagementClass c = new ManagementClass("Win32_Process"); /// foreach (ManagementObject o in c.GetInstances()) /// Console.WriteLine("Next instance of Win32_Process : ", o.Path); ///
///Dim c As New ManagementClass("Win32_Process") /// Dim o As ManagementObject /// For Each o In c.GetInstances() /// Console.WriteLine("Next instance of Win32_Process : " & o.Path) /// Next o ///
////// /// The additional operation options. ///Returns the collection of all instances of the class using the specified options. ////// ///A collection of the ///objects /// representing the instances of the class, according to the specified options. /// public ManagementObjectCollection GetInstances(EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ); IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).CreateInstanceEnum_(ClassName, o.Flags, o.GetContext(), ref enumWbem ); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return new ManagementObjectCollection(Scope, o, enumWbem); } ///EnumerationOptions opt = new EnumerationOptions(); /// //Will enumerate instances of the given class and any subclasses. /// o.enumerateDeep = true; /// ManagementClass c = new ManagementClass("CIM_Service"); /// foreach (ManagementObject o in c.GetInstances(opt)) /// Console.WriteLine(o["Name"]); ///
///Dim opt As New EnumerationOptions() /// 'Will enumerate instances of the given class and any subclasses. /// o.enumerateDeep = True /// Dim c As New ManagementClass("CIM_Service") /// Dim o As ManagementObject /// For Each o In c.GetInstances(opt) /// Console.WriteLine(o["Name"]) /// Next o ///
////// /// The object to handle the asynchronous operation's progress. ///Returns the collection of all instances of the class, asynchronously. ////// public void GetInstances(ManagementOperationObserver watcher) { GetInstances(watcher, (EnumerationOptions)null); } ///ManagementClass c = new ManagementClass("Win32_Share"); /// MyHandler h = new MyHandler(); /// ManagementOperationObserver ob = new ManagementOperationObserver(); /// ob.ObjectReady += new ObjectReadyEventHandler (h.NewObject); /// ob.Completed += new CompletedEventHandler (h.Done); /// /// c.GetInstances(ob); /// /// while (!h.Completed) /// System.Threading.Thread.Sleep (1000); /// /// //Here you can use the object /// Console.WriteLine(o["SomeProperty"]); /// /// public class MyHandler /// { /// private bool completed = false; /// /// public void NewObject(object sender, ObjectReadyEventArgs e) { /// Console.WriteLine("New result arrived !", ((ManagementObject)(e.NewObject))["Name"]); /// } /// /// public void Done(object sender, CompletedEventArgs e) { /// Console.WriteLine("async Get completed !"); /// completed = true; /// } /// /// public bool Completed { /// get { /// return completed; /// } /// } /// } ///
///Dim c As New ManagementClass("Win32_Share") /// Dim h As New MyHandler() /// Dim ob As New ManagementOperationObserver() /// ob.ObjectReady += New ObjectReadyEventHandler(h.NewObject) /// ob.Completed += New CompletedEventHandler(h.Done) /// /// c.GetInstances(ob) /// /// While Not h.Completed /// System.Threading.Thread.Sleep(1000) /// End While /// /// 'Here you can use the object /// Console.WriteLine(o("SomeProperty")) /// /// Public Class MyHandler /// Private completed As Boolean = false /// /// Public Sub Done(sender As Object, e As EventArrivedEventArgs) /// Console.WriteLine("async Get completed !") /// completed = True /// End Sub /// /// Public ReadOnly Property Completed() As Boolean /// Get /// Return completed /// End Get /// End Property /// End Class ///
////// /// The object to handle the asynchronous operation's progress. /// The specified additional options for getting the instances. public void GetInstances(ManagementOperationObserver watcher, EnumerationOptions options) { if (null == watcher) throw new ArgumentNullException("watcher"); if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ) ; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink(Scope, o.Context); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).CreateInstanceEnumAsync_( ClassName, o.Flags, o.GetContext(), sink.Stub ); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } //***************************************************** //GetSubclasses //***************************************************** ///Returns the collection of all instances of the class, asynchronously, using /// the specified options. ////// Returns the collection of /// all derived classes for the class. /// ////// ///Returns the collection of all subclasses for the class. ////// public ManagementObjectCollection GetSubclasses() { return GetSubclasses((EnumerationOptions)null); } ///A collection of the ///objects that /// represent the subclasses of the WMI class. /// /// The specified additional options for retrieving subclasses of the class. ///Retrieves the subclasses of the class using the specified /// options. ////// ///A collection of the ///objects /// representing the subclasses of the WMI class, according to the specified /// options. /// public ManagementObjectCollection GetSubclasses(EnumerationOptions options) { if (null == Path) throw new InvalidOperationException(); Initialize ( false ) ; IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler( Scope.GetIWbemServices() ).CreateClassEnum_(ClassName, o.Flags, o.GetContext(), ref enumWbem); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return new ManagementObjectCollection(Scope, o, enumWbem); } ///EnumerationOptions opt = new EnumerationOptions(); /// /// //Causes return of deep subclasses as opposed to only immediate ones. /// opt.enumerateDeep = true; /// /// ManagementObjectCollection c = (new /// ManagementClass("Win32_Share")).GetSubclasses(opt); ///
///Dim opt As New EnumerationOptions() /// /// 'Causes return of deep subclasses as opposed to only immediate ones. /// opt.enumerateDeep = true /// /// Dim cls As New ManagementClass("Win32_Share") /// Dim c As ManagementObjectCollection /// /// c = cls.GetSubClasses(opt) ///
////// /// The object to handle the asynchronous operation's progress. public void GetSubclasses(ManagementOperationObserver watcher) { GetSubclasses(watcher, (EnumerationOptions)null); } ///Returns the collection of all classes derived from this class, asynchronously. ////// /// The object to handle the asynchronous operation's progress. /// The specified additional options to use in the derived class retrieval. public void GetSubclasses(ManagementOperationObserver watcher, EnumerationOptions options) { if (null == watcher) throw new ArgumentNullException("watcher"); if (null == Path) throw new InvalidOperationException(); Initialize ( false ) ; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink(Scope, o.Context); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).CreateClassEnumAsync_(ClassName, o.Flags, o.GetContext(), sink.Stub); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } //****************************************************** //Derive //***************************************************** ///Retrieves all classes derived from this class, asynchronously, using the specified /// options. ////// /// The name of the new class to be derived. ///Derives a new class from this class. ////// ///A new ////// that represents a new WMI class derived from the original class. /// ///Note that the newly returned class has not been committed /// until the ///() method is explicitly called. /// public ManagementClass Derive(string newClassName) { ManagementClass newClass = null; if (null == newClassName) throw new ArgumentNullException("newClassName"); else { // Check the path is valid ManagementPath path = new ManagementPath(); try { path.ClassName = newClassName; } catch { throw new ArgumentOutOfRangeException("newClassName"); } if (!path.IsClass) throw new ArgumentOutOfRangeException("newClassName"); } if (PutButNotGot) { //Path = new ManagementPath(putPath); Get(); PutButNotGot = false; } // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; IWbemClassObjectFreeThreaded newWbemClass = null; int status = this.wbemObject.SpawnDerivedClass_(0, out newWbemClass); if (status >= 0) { object val = newClassName; status = newWbemClass.Put_("__CLASS", 0, ref val, 0); if (status >= 0) newClass = ManagementClass.GetManagementClass(newWbemClass, this); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return newClass; } //****************************************************** //CreateInstance //****************************************************** ///ManagementClass existingClass = new ManagementClass("CIM_Service"); /// ManagementClass newClass = existingClass.Derive("My_Service"); /// newClass.Put(); //to commit the new class to the WMI repository. ///
///Dim existingClass As New ManagementClass("CIM_Service") /// Dim newClass As ManagementClass /// /// newClass = existingClass.Derive("My_Service") /// newClass.Put() 'to commit the new class to the WMI repository. ///
////// ///Creates a new instance of the WMI class. ////// ///A ///that represents a new /// instance of the WMI class. /// ///Note that the new instance is not committed until the /// ///() method is called. Before committing it, the key properties must /// be specified. /// public ManagementObject CreateInstance() { ManagementObject newInstance = null; if (PutButNotGot) { //Path = new ManagementPath(putPath); Get(); PutButNotGot = false; } // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; IWbemClassObjectFreeThreaded newWbemInstance = null; int status = this.wbemObject.SpawnInstance_(0, out newWbemInstance); if (status >= 0) newInstance = ManagementObject.GetManagementObject(newWbemInstance, Scope); else { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return newInstance; } ///ManagementClass envClass = new ManagementClass("Win32_Environment"); /// ManagementObject newInstance = /// existingClass.CreateInstance("My_Service"); /// newInstance["Name"] = "Cori"; /// newInstance.Put(); //to commit the new instance. ///
///Dim envClass As New ManagementClass("Win32_Environment") /// Dim newInstance As ManagementObject /// /// newInstance = existingClass.CreateInstance("My_Service") /// newInstance("Name") = "Cori" /// newInstance.Put() 'to commit the new instance. ///
////// ///Returns a copy of the object. ////// ///The cloned /// object. ////// public override Object Clone() { // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; IWbemClassObjectFreeThreaded theClone = null; int status = wbemObject.Clone_(out theClone); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return ManagementClass.GetManagementClass(theClone, this); } //***************************************************** //GetRelatedClasses //****************************************************** ///Note that this does not create a copy of the /// WMI class; only an additional representation is created. ////// Retrieves classes related /// to the WMI class. /// ////// ///Retrieves classes related to the WMI class. ////// ///A collection of the ///or /// objects that represents WMI classes or instances related to /// the WMI class. /// ///The method queries the WMI schema for all /// possible associations that the WMI class may have with other classes, or in rare /// cases, to instances. ////// public ManagementObjectCollection GetRelatedClasses() { return GetRelatedClasses((string)null); } ///ManagementClass c = new ManagementClass("Win32_LogicalDisk"); /// /// foreach (ManagementClass r in c.GetRelatedClasses()) /// Console.WriteLine("Instances of {0} may have /// relationships to this class", r["__CLASS"]); ///
///Dim c As New ManagementClass("Win32_LogicalDisk") /// Dim r As ManagementClass /// /// For Each r In c.GetRelatedClasses() /// Console.WriteLine("Instances of {0} may have relationships _ /// to this class", r("__CLASS")) /// Next r ///
////// ///Retrieves classes related to the WMI class. ///The class from which resulting classes have to be derived. ////// A collection of classes related to /// this class. /// public ManagementObjectCollection GetRelatedClasses( string relatedClass) { return GetRelatedClasses(relatedClass, null, null, null, null, null, null); } ////// ///Retrieves classes related to the WMI class based on the specified /// options. ///The class from which resulting classes have to be derived. /// The relationship type which resulting classes must have with the source class. /// This qualifier must be present on the relationship. /// This qualifier must be present on the resulting classes. /// The resulting classes must have this role in the relationship. /// The source class must have this role in the relationship. /// The options for retrieving the resulting classes. ////// public ManagementObjectCollection GetRelatedClasses( string relatedClass, string relationshipClass, string relationshipQualifier, string relatedQualifier, string relatedRole, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ) ; IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null != options) ? (EnumerationOptions)options.Clone() : new EnumerationOptions(); //Ensure EnumerateDeep flag bit is turned off as it's invalid for queries o.EnumerateDeep = true; RelatedObjectQuery q = new RelatedObjectQuery(true, Path.Path, relatedClass, relationshipClass, relatedQualifier, relationshipQualifier, relatedRole, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).ExecQuery_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), ref enumWbem); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } //Create collection object return new ManagementObjectCollection(Scope, o, enumWbem); } ///A collection of classes related to /// this class. ////// /// The object to handle the asynchronous operation's progress. public void GetRelatedClasses( ManagementOperationObserver watcher) { GetRelatedClasses(watcher, (string)null); } ///Retrieves classes /// related to the WMI class, asynchronously. ////// /// The object to handle the asynchronous operation's progress. /// The name of the related class. public void GetRelatedClasses( ManagementOperationObserver watcher, string relatedClass) { GetRelatedClasses(watcher, relatedClass, null, null, null, null, null, null); } ///Retrieves classes related to the WMI class, asynchronously, given the related /// class name. ////// /// Handler for progress and results of the asynchronous operation. ///Retrieves classes related to the /// WMI class, asynchronously, using the specified options. ///The class from which resulting classes have to be derived. /// The relationship type which resulting classes must have with the source class. /// This qualifier must be present on the relationship. /// This qualifier must be present on the resulting classes. /// The resulting classes must have this role in the relationship. /// The source class must have this role in the relationship. /// The options for retrieving the resulting classes. public void GetRelatedClasses( ManagementOperationObserver watcher, string relatedClass, string relationshipClass, string relationshipQualifier, string relatedQualifier, string relatedRole, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( true ) ; if (null == watcher) throw new ArgumentNullException("watcher"); else { EnumerationOptions o = (null != options) ? (EnumerationOptions)options.Clone() : new EnumerationOptions(); //Ensure EnumerateDeep flag bit is turned off as it's invalid for queries o.EnumerateDeep = true; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink( Scope, o.Context); RelatedObjectQuery q = new RelatedObjectQuery(true, Path.Path, relatedClass, relationshipClass, relatedQualifier, relationshipQualifier, relatedRole, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).ExecQueryAsync_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), sink.Stub); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } } //***************************************************** //GetRelationshipClasses //***************************************************** ////// Retrieves relationship /// classes that relate the class to others. /// ////// ///Retrieves relationship classes that relate the class to others. ////// public ManagementObjectCollection GetRelationshipClasses() { return GetRelationshipClasses((string)null); } ///A collection of association classes /// that relate the class to any other class. ////// /// The endpoint class for all relationship classes returned. ///Retrieves relationship classes that relate the class to others, where the /// endpoint class is the specified class. ////// public ManagementObjectCollection GetRelationshipClasses( string relationshipClass) { return GetRelationshipClasses(relationshipClass, null, null, null); } ///A collection of association classes /// that relate the class to the specified class. ////// ///Retrieves relationship classes that relate this class to others, according to /// specified options. ///All resulting relationship classes must derive from this class. /// Resulting relationship classes must have this qualifier. /// The source class must have this role in the resulting relationship classes. /// Specifies options for retrieving the results. ////// public ManagementObjectCollection GetRelationshipClasses( string relationshipClass, string relationshipQualifier, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ) ; IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null != options) ? options : new EnumerationOptions(); //Ensure EnumerateDeep flag is turned off as it's invalid for queries o.EnumerateDeep = true; RelationshipQuery q = new RelationshipQuery(true, Path.Path, relationshipClass, relationshipQualifier, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; //Execute WMI query try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).ExecQuery_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), ref enumWbem); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } //Create collection object return new ManagementObjectCollection(Scope, o, enumWbem); } ///A collection of association classes /// that relate this class to others, according to the specified options. ////// /// The object to handle the asynchronous operation's progress. public void GetRelationshipClasses( ManagementOperationObserver watcher) { GetRelationshipClasses(watcher, (string)null); } ///Retrieves relationship classes that relate the class to others, /// asynchronously. ////// /// The object to handle the asynchronous operation's progress. /// The WMI class to which all returned relationships should point. public void GetRelationshipClasses( ManagementOperationObserver watcher, string relationshipClass) { GetRelationshipClasses(watcher, relationshipClass, null, null, null); } ///Retrieves relationship classes that relate the class to the specified WMI class, /// asynchronously. ////// /// The handler for progress and results of the asynchronous operation. ///Retrieves relationship classes that relate the class according to the specified /// options, asynchronously. ///The class from which all resulting relationship classes must derive. /// The qualifier which the resulting relationship classes must have. /// The role which the source class must have in the resulting relationship classes. /// The options for retrieving the results. ////// public void GetRelationshipClasses( ManagementOperationObserver watcher, string relationshipClass, string relationshipQualifier, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); if (null == watcher) throw new ArgumentNullException("watcher"); else { Initialize ( true ) ; EnumerationOptions o = (null != options) ? (EnumerationOptions)options.Clone() : new EnumerationOptions(); //Ensure EnumerateDeep flag is turned off as it's invalid for queries o.EnumerateDeep = true; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink(Scope, o.Context); RelationshipQuery q = new RelationshipQuery(true, Path.Path, relationshipClass, relationshipQualifier, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices()).ExecQueryAsync_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), sink.Stub); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } } ///A collection of association classes /// relating this class to others, according to the given options. ////// ///Generates a strongly-typed class for a given WMI class. ////// ///Generates a strongly-typed class for a given WMI class. ///if the class for managing system properties must be included; otherwise, . /// if the generated class will manage system properties; otherwise, . /// /// ///A ///instance /// representing the declaration for the strongly-typed class. /// public CodeTypeDeclaration GetStronglyTypedClassCode(bool includeSystemClassInClassDef, bool systemPropertyClass) { // Ensure that the object is valid Get(); ManagementClassGenerator classGen = new ManagementClassGenerator(this); return classGen.GenerateCode(includeSystemClassInClassDef,systemPropertyClass); } ///using System; /// using System.Management; /// using System.CodeDom; /// using System.IO; /// using System.CodeDom.Compiler; /// using Microsoft.CSharp; /// /// void GenerateCSharpCode() /// { /// string strFilePath = "C:\\temp\\LogicalDisk.cs"; /// CodeTypeDeclaration ClsDom; /// /// ManagementClass cls1 = new ManagementClass(null,"Win32_LogicalDisk",null); /// ClsDom = cls1.GetStronglyTypedClassCode(false,false); /// /// ICodeGenerator cg = (new CSharpCodeProvider()).CreateGenerator (); /// CodeNamespace cn = new CodeNamespace("TestNamespace"); /// /// // Add any imports to the code /// cn.Imports.Add (new CodeNamespaceImport("System")); /// cn.Imports.Add (new CodeNamespaceImport("System.ComponentModel")); /// cn.Imports.Add (new CodeNamespaceImport("System.Management")); /// cn.Imports.Add(new CodeNamespaceImport("System.Collections")); /// /// // Add class to the namespace /// cn.Types.Add (ClsDom); /// /// //Now create the filestream (output file) /// TextWriter tw = new StreamWriter(new /// FileStream (strFilePath,FileMode.Create)); /// /// // And write it to the file /// cg.GenerateCodeFromNamespace (cn, tw, new CodeGeneratorOptions()); /// /// tw.Close(); /// } ///
////// /// The language of the code to be generated. /// The path of the file where the code is to be written. /// The .NET namespace into which the class should be generated. If this is empty, the namespace will be generated from the WMI namespace. ///Generates a strongly-typed class for a given WMI class. This function generates code for Visual Basic, /// C#, or JScript, depending on the input parameters. ////// ////// , if the method succeeded; /// otherwise, . /// public bool GetStronglyTypedClassCode(CodeLanguage lang, String filePath,String classNamespace) { // Ensure that the object is valid Get(); ManagementClassGenerator classGen = new ManagementClassGenerator(this); return classGen.GenerateCode(lang , filePath,classNamespace); } }//ManagementClass } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Specialized; using System.Runtime.InteropServices; using System.Runtime.Serialization; using WbemClient_v1; using System.CodeDom; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ///using System; /// using System.Management; /// /// ManagementClass cls = new ManagementClass(null,"Win32_LogicalDisk",null,""); /// cls.GetStronglyTypedClassCode(CodeLanguage.CSharp,"C:\temp\Logicaldisk.cs",String.Empty); ///
////// ///Represents a CIM management class from WMI. CIM (Common Information Model) classes /// represent management information including hardware, software, processes, etc. /// For more information about the CIM classes available in Windows search for ‘win32 classes’. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// [Serializable] public class ManagementClass : ManagementObject { private MethodDataCollection methods; protected override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData ( info, context ) ; } ///using System; /// using System.Management; /// /// // This example demonstrates getting information about a class using the ManagementClass object /// class Sample_ManagementClass /// { /// public static int Main(string[] args) { /// ManagementClass diskClass = new ManagementClass("Win32_LogicalDisk"); /// diskClass.Get(); /// Console.WriteLine("Logical Disk class has " + diskClass.Properties.Count + " properties"); /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This example demonstrates getting information about a class using the ManagementClass object /// Class Sample_ManagementClass /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("Win32_LogicalDisk") /// diskClass.Get() /// Console.WriteLine(("Logical Disk class has " & _ /// diskClass.Properties.Count.ToString() & _ /// " properties")) /// Return 0 /// End Function /// End Class ///
////// Internal factory for classes, used when deriving a class /// or cloning a class. For these purposes we always mark /// the class as "bound". /// /// The underlying WMI object /// Seed class from which we will get initialization info internal static ManagementClass GetManagementClass( IWbemClassObjectFreeThreaded wbemObject, ManagementClass mgObj) { ManagementClass newClass = new ManagementClass(); newClass.wbemObject = wbemObject; if (null != mgObj) { newClass.scope = ManagementScope._Clone(mgObj.scope); ManagementPath objPath = mgObj.Path; if (null != objPath) newClass.path = ManagementPath._Clone(objPath); // Ensure we have our class name in the path object className = null; int dummy = 0; int status = wbemObject.Get_("__CLASS", 0, ref className, ref dummy, ref dummy); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } if (className != System.DBNull.Value) newClass.path.internalClassName = (string)className; ObjectGetOptions options = mgObj.Options; if (null != options) newClass.options = ObjectGetOptions._Clone(options); // Finally we ensure that this object is marked as bound. // We do this as a last step since setting certain properties // (Options, Path and Scope) would mark it as unbound // // *** // * Changed isBound flag to wbemObject==null check. // * newClass.IsBound = true; // *** } return newClass; } internal static ManagementClass GetManagementClass( IWbemClassObjectFreeThreaded wbemObject, ManagementScope scope) { ManagementClass newClass = new ManagementClass(); newClass.path = new ManagementPath(ManagementPath.GetManagementPath(wbemObject)); if (null != scope) newClass.scope = ManagementScope._Clone(scope); newClass.wbemObject = wbemObject; return newClass; } //default constructor ////// Initializes a new instance /// of the ///class. /// /// ///Initializes a new instance of the ///class. This is the /// default constructor. /// public ManagementClass() : this ((ManagementScope)null, (ManagementPath)null, null) {} //parameterized constructors ///ManagementClass c = new ManagementClass(); ///
///Dim c As New ManagementClass() ///
////// /// AInitializes a new instance of the ///class initialized to the /// given path. specifying which WMI class to bind to. /// /// ///The ///parameter must specify a WMI class /// path. /// public ManagementClass(ManagementPath path) : this(null, path, null) {} ///ManagementClass c = new ManagementClass( /// new ManagementPath("Win32_LogicalDisk")); ///
///Dim c As New ManagementClass( _ /// New ManagementPath("Win32_LogicalDisk")) ///
////// /// The path to the WMI class. ///Initializes a new instance of the ///class initialized to the given path. /// public ManagementClass(string path) : this(null, new ManagementPath(path), null) {} ///ManagementClass c = new /// ManagementClass("Win32_LogicalDisk"); ///
///Dim c As New ManagementClass("Win32_LogicalDisk") ///
////// /// AInitializes a new instance of the ///class initialized to the /// given WMI class path using the specified options. representing the WMI class path. /// An representing the options to use when retrieving this class. /// /// public ManagementClass(ManagementPath path, ObjectGetOptions options) : this(null, path, options) {} ///ManagementPath p = new ManagementPath("Win32_Process"); /// //Options specify that amended qualifiers are to be retrieved along with the class /// ObjectGetOptions o = new ObjectGetOptions(null, true); /// ManagementClass c = new ManagementClass(p,o); ///
///Dim p As New ManagementPath("Win32_Process") /// ' Options specify that amended qualifiers are to be retrieved along with the class /// Dim o As New ObjectGetOptions(Null, True) /// Dim c As New ManagementClass(p,o) ///
////// /// The path to the WMI class. /// AnInitializes a new instance of the ///class initialized to the given WMI class path /// using the specified options. representing the options to use when retrieving the WMI class. /// /// public ManagementClass(string path, ObjectGetOptions options) : this(null, new ManagementPath(path), options) {} /////Options specify that amended qualifiers should be retrieved along with the class /// ObjectGetOptions o = new ObjectGetOptions(null, true); /// ManagementClass c = new ManagementClass("Win32_ComputerSystem",o); ///
///' Options specify that amended qualifiers should be retrieved along with the class /// Dim o As New ObjectGetOptions(Null, True) /// Dim c As New ManagementClass("Win32_ComputerSystem",o) ///
////// /// AInitializes a new instance of the ///class for the specified /// WMI class in the specified scope and with the specified options. that specifies the scope (server and namespace) where the WMI class resides. /// A that represents the path to the WMI class in the specified scope. /// An that specifies the options to use when retrieving the WMI class. /// /// ///The path can be specified as a full /// path (including server and namespace). However, if a scope is specified, it will /// override the first portion of the full path. ////// public ManagementClass(ManagementScope scope, ManagementPath path, ObjectGetOptions options) : base (scope, path, options) {} ///ManagementScope s = new ManagementScope("\\\\MyBox\\root\\cimv2"); /// ManagementPath p = new ManagementPath("Win32_Environment"); /// ObjectGetOptions o = new ObjectGetOptions(null, true); /// ManagementClass c = new ManagementClass(s, p, o); ///
///Dim s As New ManagementScope("\\MyBox\root\cimv2") /// Dim p As New ManagementPath("Win32_Environment") /// Dim o As New ObjectGetOptions(Null, True) /// Dim c As New ManagementClass(s, p, o) ///
////// /// The scope in which the WMI class resides. /// The path to the WMI class within the specified scope. /// AnInitializes a new instance of the ///class for the specified WMI class, in the /// specified scope, and with the specified options. that specifies the options to use when retrieving the WMI class. /// /// ///The path can be specified as a full /// path (including server and namespace). However, if a scope is specified, it will /// override the first portion of the full path. ////// public ManagementClass(string scope, string path, ObjectGetOptions options) : base (new ManagementScope(scope), new ManagementPath(path), options) {} protected ManagementClass(SerializationInfo info, StreamingContext context) : base(info, context) {} ///ManagementClass c = new ManagementClass("\\\\MyBox\\root\\cimv2", /// "Win32_Environment", /// new ObjectGetOptions(null, true)); ///
///Dim c As New ManagementClass("\\MyBox\root\cimv2", _ /// "Win32_Environment", _ /// new ObjectGetOptions(Null, True)) ///
////// ///Gets or sets the path of the WMI class to /// which the ////// object is bound. /// ///The path of the object's class. ////// ///When the property is set to a new value, /// the ////// object will be /// disconnected from any previously-bound WMI class. Reconnect to the new WMI class path. /// public override ManagementPath Path { get { return base.Path; } set { // This must be a class path or empty (don't allow instance paths) if ((null == value) || value.IsClass || value.IsEmpty) base.Path = value; else throw new ArgumentOutOfRangeException("value"); } } ///ManagementClass c = new ManagementClass(); /// c.Path = "Win32_Environment"; ///
///Dim c As New ManagementClass() /// c.Path = "Win32_Environment" ///
////// ///Gets or sets an array containing all WMI classes in the /// inheritance hierarchy from this class to the top. ////// A string collection containing the /// names of all WMI classes in the inheritance hierarchy of this class. /// ////// ///This property is read-only. ////// public StringCollection Derivation { get { StringCollection result = new StringCollection(); // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; int dummy1 = 0, dummy2 = 0; object val = null; int status = wbemObject.Get_("__DERIVATION", 0, ref val, ref dummy1, ref dummy2); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } if (null != val) result.AddRange((String [])val); return result; } } ///ManagementClass c = new ManagementClass("Win32_LogicalDisk"); /// foreach (string s in c.Derivation) /// Console.WriteLine("Further derived from : ", s); ///
///Dim c As New ManagementClass("Win32_LogicalDisk") /// Dim s As String /// For Each s In c.Derivation /// Console.WriteLine("Further derived from : " & s) /// Next s ///
////// ///Gets or sets a collection of ///objects that /// represent the methods defined in the WMI class. /// ///A ///representing the methods defined in the WMI class. /// public MethodDataCollection Methods { get { Initialize ( true ) ; if (methods == null) methods = new MethodDataCollection(this); return methods; } } // //Methods // //****************************************************** //GetInstances //***************************************************** ///ManagementClass c = new ManagementClass("Win32_Process"); /// foreach (Method m in c.Methods) /// Console.WriteLine("This class contains this method : ", m.Name); ///
///Dim c As New ManagementClass("Win32_Process") /// Dim m As Method /// For Each m in c.Methods /// Console.WriteLine("This class contains this method : " & m.Name) ///
////// Returns the collection of /// all instances of the class. /// ////// ///Returns the collection of all instances of the class. ////// ///A collection of the ///objects /// representing the instances of the class. /// public ManagementObjectCollection GetInstances() { return GetInstances((EnumerationOptions)null); } ///ManagementClass c = new ManagementClass("Win32_Process"); /// foreach (ManagementObject o in c.GetInstances()) /// Console.WriteLine("Next instance of Win32_Process : ", o.Path); ///
///Dim c As New ManagementClass("Win32_Process") /// Dim o As ManagementObject /// For Each o In c.GetInstances() /// Console.WriteLine("Next instance of Win32_Process : " & o.Path) /// Next o ///
////// /// The additional operation options. ///Returns the collection of all instances of the class using the specified options. ////// ///A collection of the ///objects /// representing the instances of the class, according to the specified options. /// public ManagementObjectCollection GetInstances(EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ); IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).CreateInstanceEnum_(ClassName, o.Flags, o.GetContext(), ref enumWbem ); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return new ManagementObjectCollection(Scope, o, enumWbem); } ///EnumerationOptions opt = new EnumerationOptions(); /// //Will enumerate instances of the given class and any subclasses. /// o.enumerateDeep = true; /// ManagementClass c = new ManagementClass("CIM_Service"); /// foreach (ManagementObject o in c.GetInstances(opt)) /// Console.WriteLine(o["Name"]); ///
///Dim opt As New EnumerationOptions() /// 'Will enumerate instances of the given class and any subclasses. /// o.enumerateDeep = True /// Dim c As New ManagementClass("CIM_Service") /// Dim o As ManagementObject /// For Each o In c.GetInstances(opt) /// Console.WriteLine(o["Name"]) /// Next o ///
////// /// The object to handle the asynchronous operation's progress. ///Returns the collection of all instances of the class, asynchronously. ////// public void GetInstances(ManagementOperationObserver watcher) { GetInstances(watcher, (EnumerationOptions)null); } ///ManagementClass c = new ManagementClass("Win32_Share"); /// MyHandler h = new MyHandler(); /// ManagementOperationObserver ob = new ManagementOperationObserver(); /// ob.ObjectReady += new ObjectReadyEventHandler (h.NewObject); /// ob.Completed += new CompletedEventHandler (h.Done); /// /// c.GetInstances(ob); /// /// while (!h.Completed) /// System.Threading.Thread.Sleep (1000); /// /// //Here you can use the object /// Console.WriteLine(o["SomeProperty"]); /// /// public class MyHandler /// { /// private bool completed = false; /// /// public void NewObject(object sender, ObjectReadyEventArgs e) { /// Console.WriteLine("New result arrived !", ((ManagementObject)(e.NewObject))["Name"]); /// } /// /// public void Done(object sender, CompletedEventArgs e) { /// Console.WriteLine("async Get completed !"); /// completed = true; /// } /// /// public bool Completed { /// get { /// return completed; /// } /// } /// } ///
///Dim c As New ManagementClass("Win32_Share") /// Dim h As New MyHandler() /// Dim ob As New ManagementOperationObserver() /// ob.ObjectReady += New ObjectReadyEventHandler(h.NewObject) /// ob.Completed += New CompletedEventHandler(h.Done) /// /// c.GetInstances(ob) /// /// While Not h.Completed /// System.Threading.Thread.Sleep(1000) /// End While /// /// 'Here you can use the object /// Console.WriteLine(o("SomeProperty")) /// /// Public Class MyHandler /// Private completed As Boolean = false /// /// Public Sub Done(sender As Object, e As EventArrivedEventArgs) /// Console.WriteLine("async Get completed !") /// completed = True /// End Sub /// /// Public ReadOnly Property Completed() As Boolean /// Get /// Return completed /// End Get /// End Property /// End Class ///
////// /// The object to handle the asynchronous operation's progress. /// The specified additional options for getting the instances. public void GetInstances(ManagementOperationObserver watcher, EnumerationOptions options) { if (null == watcher) throw new ArgumentNullException("watcher"); if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ) ; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink(Scope, o.Context); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).CreateInstanceEnumAsync_( ClassName, o.Flags, o.GetContext(), sink.Stub ); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } //***************************************************** //GetSubclasses //***************************************************** ///Returns the collection of all instances of the class, asynchronously, using /// the specified options. ////// Returns the collection of /// all derived classes for the class. /// ////// ///Returns the collection of all subclasses for the class. ////// public ManagementObjectCollection GetSubclasses() { return GetSubclasses((EnumerationOptions)null); } ///A collection of the ///objects that /// represent the subclasses of the WMI class. /// /// The specified additional options for retrieving subclasses of the class. ///Retrieves the subclasses of the class using the specified /// options. ////// ///A collection of the ///objects /// representing the subclasses of the WMI class, according to the specified /// options. /// public ManagementObjectCollection GetSubclasses(EnumerationOptions options) { if (null == Path) throw new InvalidOperationException(); Initialize ( false ) ; IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler( Scope.GetIWbemServices() ).CreateClassEnum_(ClassName, o.Flags, o.GetContext(), ref enumWbem); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return new ManagementObjectCollection(Scope, o, enumWbem); } ///EnumerationOptions opt = new EnumerationOptions(); /// /// //Causes return of deep subclasses as opposed to only immediate ones. /// opt.enumerateDeep = true; /// /// ManagementObjectCollection c = (new /// ManagementClass("Win32_Share")).GetSubclasses(opt); ///
///Dim opt As New EnumerationOptions() /// /// 'Causes return of deep subclasses as opposed to only immediate ones. /// opt.enumerateDeep = true /// /// Dim cls As New ManagementClass("Win32_Share") /// Dim c As ManagementObjectCollection /// /// c = cls.GetSubClasses(opt) ///
////// /// The object to handle the asynchronous operation's progress. public void GetSubclasses(ManagementOperationObserver watcher) { GetSubclasses(watcher, (EnumerationOptions)null); } ///Returns the collection of all classes derived from this class, asynchronously. ////// /// The object to handle the asynchronous operation's progress. /// The specified additional options to use in the derived class retrieval. public void GetSubclasses(ManagementOperationObserver watcher, EnumerationOptions options) { if (null == watcher) throw new ArgumentNullException("watcher"); if (null == Path) throw new InvalidOperationException(); Initialize ( false ) ; EnumerationOptions o = (null == options) ? new EnumerationOptions() : (EnumerationOptions)options.Clone(); //Need to make sure that we're not passing invalid flags to enumeration APIs. //The only flags in EnumerationOptions not valid for enumerations are EnsureLocatable & PrototypeOnly. o.EnsureLocatable = false; o.PrototypeOnly = false; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink(Scope, o.Context); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).CreateClassEnumAsync_(ClassName, o.Flags, o.GetContext(), sink.Stub); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } //****************************************************** //Derive //***************************************************** ///Retrieves all classes derived from this class, asynchronously, using the specified /// options. ////// /// The name of the new class to be derived. ///Derives a new class from this class. ////// ///A new ////// that represents a new WMI class derived from the original class. /// ///Note that the newly returned class has not been committed /// until the ///() method is explicitly called. /// public ManagementClass Derive(string newClassName) { ManagementClass newClass = null; if (null == newClassName) throw new ArgumentNullException("newClassName"); else { // Check the path is valid ManagementPath path = new ManagementPath(); try { path.ClassName = newClassName; } catch { throw new ArgumentOutOfRangeException("newClassName"); } if (!path.IsClass) throw new ArgumentOutOfRangeException("newClassName"); } if (PutButNotGot) { //Path = new ManagementPath(putPath); Get(); PutButNotGot = false; } // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; IWbemClassObjectFreeThreaded newWbemClass = null; int status = this.wbemObject.SpawnDerivedClass_(0, out newWbemClass); if (status >= 0) { object val = newClassName; status = newWbemClass.Put_("__CLASS", 0, ref val, 0); if (status >= 0) newClass = ManagementClass.GetManagementClass(newWbemClass, this); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return newClass; } //****************************************************** //CreateInstance //****************************************************** ///ManagementClass existingClass = new ManagementClass("CIM_Service"); /// ManagementClass newClass = existingClass.Derive("My_Service"); /// newClass.Put(); //to commit the new class to the WMI repository. ///
///Dim existingClass As New ManagementClass("CIM_Service") /// Dim newClass As ManagementClass /// /// newClass = existingClass.Derive("My_Service") /// newClass.Put() 'to commit the new class to the WMI repository. ///
////// ///Creates a new instance of the WMI class. ////// ///A ///that represents a new /// instance of the WMI class. /// ///Note that the new instance is not committed until the /// ///() method is called. Before committing it, the key properties must /// be specified. /// public ManagementObject CreateInstance() { ManagementObject newInstance = null; if (PutButNotGot) { //Path = new ManagementPath(putPath); Get(); PutButNotGot = false; } // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; IWbemClassObjectFreeThreaded newWbemInstance = null; int status = this.wbemObject.SpawnInstance_(0, out newWbemInstance); if (status >= 0) newInstance = ManagementObject.GetManagementObject(newWbemInstance, Scope); else { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return newInstance; } ///ManagementClass envClass = new ManagementClass("Win32_Environment"); /// ManagementObject newInstance = /// existingClass.CreateInstance("My_Service"); /// newInstance["Name"] = "Cori"; /// newInstance.Put(); //to commit the new instance. ///
///Dim envClass As New ManagementClass("Win32_Environment") /// Dim newInstance As ManagementObject /// /// newInstance = existingClass.CreateInstance("My_Service") /// newInstance("Name") = "Cori" /// newInstance.Put() 'to commit the new instance. ///
////// ///Returns a copy of the object. ////// ///The cloned /// object. ////// public override Object Clone() { // // Removed Initialize call since wbemObject is a property that will call Initialize ( true ) on // its getter. // // Initialize ( ) ; IWbemClassObjectFreeThreaded theClone = null; int status = wbemObject.Clone_(out theClone); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return ManagementClass.GetManagementClass(theClone, this); } //***************************************************** //GetRelatedClasses //****************************************************** ///Note that this does not create a copy of the /// WMI class; only an additional representation is created. ////// Retrieves classes related /// to the WMI class. /// ////// ///Retrieves classes related to the WMI class. ////// ///A collection of the ///or /// objects that represents WMI classes or instances related to /// the WMI class. /// ///The method queries the WMI schema for all /// possible associations that the WMI class may have with other classes, or in rare /// cases, to instances. ////// public ManagementObjectCollection GetRelatedClasses() { return GetRelatedClasses((string)null); } ///ManagementClass c = new ManagementClass("Win32_LogicalDisk"); /// /// foreach (ManagementClass r in c.GetRelatedClasses()) /// Console.WriteLine("Instances of {0} may have /// relationships to this class", r["__CLASS"]); ///
///Dim c As New ManagementClass("Win32_LogicalDisk") /// Dim r As ManagementClass /// /// For Each r In c.GetRelatedClasses() /// Console.WriteLine("Instances of {0} may have relationships _ /// to this class", r("__CLASS")) /// Next r ///
////// ///Retrieves classes related to the WMI class. ///The class from which resulting classes have to be derived. ////// A collection of classes related to /// this class. /// public ManagementObjectCollection GetRelatedClasses( string relatedClass) { return GetRelatedClasses(relatedClass, null, null, null, null, null, null); } ////// ///Retrieves classes related to the WMI class based on the specified /// options. ///The class from which resulting classes have to be derived. /// The relationship type which resulting classes must have with the source class. /// This qualifier must be present on the relationship. /// This qualifier must be present on the resulting classes. /// The resulting classes must have this role in the relationship. /// The source class must have this role in the relationship. /// The options for retrieving the resulting classes. ////// public ManagementObjectCollection GetRelatedClasses( string relatedClass, string relationshipClass, string relationshipQualifier, string relatedQualifier, string relatedRole, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ) ; IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null != options) ? (EnumerationOptions)options.Clone() : new EnumerationOptions(); //Ensure EnumerateDeep flag bit is turned off as it's invalid for queries o.EnumerateDeep = true; RelatedObjectQuery q = new RelatedObjectQuery(true, Path.Path, relatedClass, relationshipClass, relatedQualifier, relationshipQualifier, relatedRole, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).ExecQuery_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), ref enumWbem); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } //Create collection object return new ManagementObjectCollection(Scope, o, enumWbem); } ///A collection of classes related to /// this class. ////// /// The object to handle the asynchronous operation's progress. public void GetRelatedClasses( ManagementOperationObserver watcher) { GetRelatedClasses(watcher, (string)null); } ///Retrieves classes /// related to the WMI class, asynchronously. ////// /// The object to handle the asynchronous operation's progress. /// The name of the related class. public void GetRelatedClasses( ManagementOperationObserver watcher, string relatedClass) { GetRelatedClasses(watcher, relatedClass, null, null, null, null, null, null); } ///Retrieves classes related to the WMI class, asynchronously, given the related /// class name. ////// /// Handler for progress and results of the asynchronous operation. ///Retrieves classes related to the /// WMI class, asynchronously, using the specified options. ///The class from which resulting classes have to be derived. /// The relationship type which resulting classes must have with the source class. /// This qualifier must be present on the relationship. /// This qualifier must be present on the resulting classes. /// The resulting classes must have this role in the relationship. /// The source class must have this role in the relationship. /// The options for retrieving the resulting classes. public void GetRelatedClasses( ManagementOperationObserver watcher, string relatedClass, string relationshipClass, string relationshipQualifier, string relatedQualifier, string relatedRole, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( true ) ; if (null == watcher) throw new ArgumentNullException("watcher"); else { EnumerationOptions o = (null != options) ? (EnumerationOptions)options.Clone() : new EnumerationOptions(); //Ensure EnumerateDeep flag bit is turned off as it's invalid for queries o.EnumerateDeep = true; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink( Scope, o.Context); RelatedObjectQuery q = new RelatedObjectQuery(true, Path.Path, relatedClass, relationshipClass, relatedQualifier, relationshipQualifier, relatedRole, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).ExecQueryAsync_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), sink.Stub); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } } //***************************************************** //GetRelationshipClasses //***************************************************** ////// Retrieves relationship /// classes that relate the class to others. /// ////// ///Retrieves relationship classes that relate the class to others. ////// public ManagementObjectCollection GetRelationshipClasses() { return GetRelationshipClasses((string)null); } ///A collection of association classes /// that relate the class to any other class. ////// /// The endpoint class for all relationship classes returned. ///Retrieves relationship classes that relate the class to others, where the /// endpoint class is the specified class. ////// public ManagementObjectCollection GetRelationshipClasses( string relationshipClass) { return GetRelationshipClasses(relationshipClass, null, null, null); } ///A collection of association classes /// that relate the class to the specified class. ////// ///Retrieves relationship classes that relate this class to others, according to /// specified options. ///All resulting relationship classes must derive from this class. /// Resulting relationship classes must have this qualifier. /// The source class must have this role in the resulting relationship classes. /// Specifies options for retrieving the results. ////// public ManagementObjectCollection GetRelationshipClasses( string relationshipClass, string relationshipQualifier, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); Initialize ( false ) ; IEnumWbemClassObject enumWbem = null; EnumerationOptions o = (null != options) ? options : new EnumerationOptions(); //Ensure EnumerateDeep flag is turned off as it's invalid for queries o.EnumerateDeep = true; RelationshipQuery q = new RelationshipQuery(true, Path.Path, relationshipClass, relationshipQualifier, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; //Execute WMI query try { securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices() ).ExecQuery_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), ref enumWbem); } finally { if (securityHandler != null) securityHandler.Reset(); } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } //Create collection object return new ManagementObjectCollection(Scope, o, enumWbem); } ///A collection of association classes /// that relate this class to others, according to the specified options. ////// /// The object to handle the asynchronous operation's progress. public void GetRelationshipClasses( ManagementOperationObserver watcher) { GetRelationshipClasses(watcher, (string)null); } ///Retrieves relationship classes that relate the class to others, /// asynchronously. ////// /// The object to handle the asynchronous operation's progress. /// The WMI class to which all returned relationships should point. public void GetRelationshipClasses( ManagementOperationObserver watcher, string relationshipClass) { GetRelationshipClasses(watcher, relationshipClass, null, null, null); } ///Retrieves relationship classes that relate the class to the specified WMI class, /// asynchronously. ////// /// The handler for progress and results of the asynchronous operation. ///Retrieves relationship classes that relate the class according to the specified /// options, asynchronously. ///The class from which all resulting relationship classes must derive. /// The qualifier which the resulting relationship classes must have. /// The role which the source class must have in the resulting relationship classes. /// The options for retrieving the results. ////// public void GetRelationshipClasses( ManagementOperationObserver watcher, string relationshipClass, string relationshipQualifier, string thisRole, EnumerationOptions options) { if ((null == Path) || (null == Path.Path) || (0 == Path.Path.Length)) throw new InvalidOperationException(); if (null == watcher) throw new ArgumentNullException("watcher"); else { Initialize ( true ) ; EnumerationOptions o = (null != options) ? (EnumerationOptions)options.Clone() : new EnumerationOptions(); //Ensure EnumerateDeep flag is turned off as it's invalid for queries o.EnumerateDeep = true; // Ensure we switch off ReturnImmediately as this is invalid for async calls o.ReturnImmediately = false; // If someone has registered for progress, make sure we flag it if (watcher.HaveListenersForProgress) o.SendStatus = true; WmiEventSink sink = watcher.GetNewSink(Scope, o.Context); RelationshipQuery q = new RelationshipQuery(true, Path.Path, relationshipClass, relationshipQualifier, thisRole); SecurityHandler securityHandler = null; int status = (int)ManagementStatus.NoError; securityHandler = Scope.GetSecurityHandler(); status = scope.GetSecuredIWbemServicesHandler(Scope.GetIWbemServices()).ExecQueryAsync_( q.QueryLanguage, q.QueryString, o.Flags, o.GetContext(), sink.Stub); if (securityHandler != null) securityHandler.Reset(); if (status < 0) { watcher.RemoveSink(sink); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } } ///A collection of association classes /// relating this class to others, according to the given options. ////// ///Generates a strongly-typed class for a given WMI class. ////// ///Generates a strongly-typed class for a given WMI class. ///if the class for managing system properties must be included; otherwise, . /// if the generated class will manage system properties; otherwise, . /// /// ///A ///instance /// representing the declaration for the strongly-typed class. /// public CodeTypeDeclaration GetStronglyTypedClassCode(bool includeSystemClassInClassDef, bool systemPropertyClass) { // Ensure that the object is valid Get(); ManagementClassGenerator classGen = new ManagementClassGenerator(this); return classGen.GenerateCode(includeSystemClassInClassDef,systemPropertyClass); } ///using System; /// using System.Management; /// using System.CodeDom; /// using System.IO; /// using System.CodeDom.Compiler; /// using Microsoft.CSharp; /// /// void GenerateCSharpCode() /// { /// string strFilePath = "C:\\temp\\LogicalDisk.cs"; /// CodeTypeDeclaration ClsDom; /// /// ManagementClass cls1 = new ManagementClass(null,"Win32_LogicalDisk",null); /// ClsDom = cls1.GetStronglyTypedClassCode(false,false); /// /// ICodeGenerator cg = (new CSharpCodeProvider()).CreateGenerator (); /// CodeNamespace cn = new CodeNamespace("TestNamespace"); /// /// // Add any imports to the code /// cn.Imports.Add (new CodeNamespaceImport("System")); /// cn.Imports.Add (new CodeNamespaceImport("System.ComponentModel")); /// cn.Imports.Add (new CodeNamespaceImport("System.Management")); /// cn.Imports.Add(new CodeNamespaceImport("System.Collections")); /// /// // Add class to the namespace /// cn.Types.Add (ClsDom); /// /// //Now create the filestream (output file) /// TextWriter tw = new StreamWriter(new /// FileStream (strFilePath,FileMode.Create)); /// /// // And write it to the file /// cg.GenerateCodeFromNamespace (cn, tw, new CodeGeneratorOptions()); /// /// tw.Close(); /// } ///
////// /// The language of the code to be generated. /// The path of the file where the code is to be written. /// The .NET namespace into which the class should be generated. If this is empty, the namespace will be generated from the WMI namespace. ///Generates a strongly-typed class for a given WMI class. This function generates code for Visual Basic, /// C#, or JScript, depending on the input parameters. ////// ////// , if the method succeeded; /// otherwise, . /// public bool GetStronglyTypedClassCode(CodeLanguage lang, String filePath,String classNamespace) { // Ensure that the object is valid Get(); ManagementClassGenerator classGen = new ManagementClassGenerator(this); return classGen.GenerateCode(lang , filePath,classNamespace); } }//ManagementClass } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.using System; /// using System.Management; /// /// ManagementClass cls = new ManagementClass(null,"Win32_LogicalDisk",null,""); /// cls.GetStronglyTypedClassCode(CodeLanguage.CSharp,"C:\temp\Logicaldisk.cs",String.Empty); ///
///
Link Menu
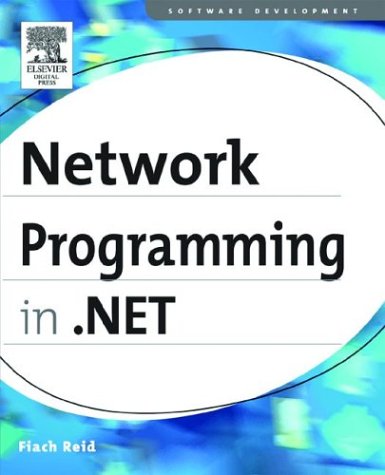
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TagMapCollection.cs
- ProgressiveCrcCalculatingStream.cs
- DataFormats.cs
- UInt16Storage.cs
- OperationResponse.cs
- IIS7WorkerRequest.cs
- SecuritySessionServerSettings.cs
- JsonDataContract.cs
- FacetValues.cs
- MethodCallTranslator.cs
- AssociationType.cs
- DateTimeFormat.cs
- VirtualPath.cs
- FileLogRecordStream.cs
- TextTabProperties.cs
- KeyValuePair.cs
- NotImplementedException.cs
- ListView.cs
- Calendar.cs
- EventManager.cs
- CompilationUtil.cs
- RemoteAsymmetricSignatureFormatter.cs
- EmbeddedMailObjectsCollection.cs
- BindingBase.cs
- EntityStoreSchemaFilterEntry.cs
- SecurityTokenAuthenticator.cs
- StateChangeEvent.cs
- PageSettings.cs
- MetabaseSettings.cs
- FlowDocumentFormatter.cs
- BamlResourceDeserializer.cs
- DataServiceRequest.cs
- SQLInt64Storage.cs
- PhysicalAddress.cs
- ToolStripContentPanel.cs
- PageOutputColor.cs
- AnnotationComponentManager.cs
- ModuleBuilderData.cs
- ChtmlTextWriter.cs
- SqlMethods.cs
- CommonXSendMessage.cs
- CriticalHandle.cs
- LookupNode.cs
- DataTable.cs
- ArgumentNullException.cs
- ByteConverter.cs
- WindowsIdentity.cs
- FrameworkElementFactory.cs
- DigestComparer.cs
- BamlLocalizationDictionary.cs
- Console.cs
- smtppermission.cs
- ListItemCollection.cs
- EmptyEnumerator.cs
- PreProcessor.cs
- XmlSchema.cs
- IndicFontClient.cs
- WebDisplayNameAttribute.cs
- KeyValueSerializer.cs
- objectquery_tresulttype.cs
- Int64Animation.cs
- DbMetaDataColumnNames.cs
- UserPersonalizationStateInfo.cs
- WebPartEditorApplyVerb.cs
- RegexRunnerFactory.cs
- SubMenuStyleCollection.cs
- BooleanExpr.cs
- RowToFieldTransformer.cs
- Table.cs
- SecurityCriticalDataForSet.cs
- BitmapPalette.cs
- LinqDataSourceContextEventArgs.cs
- SqlCacheDependency.cs
- PresentationSource.cs
- BitFlagsGenerator.cs
- ObjectDataSourceEventArgs.cs
- NonPrimarySelectionGlyph.cs
- AttachedAnnotationChangedEventArgs.cs
- Console.cs
- Math.cs
- mda.cs
- OdbcConnectionOpen.cs
- SecurityHelper.cs
- TabItem.cs
- EnglishPluralizationService.cs
- TextBoxBase.cs
- WebWorkflowRole.cs
- httpstaticobjectscollection.cs
- HtmlEmptyTagControlBuilder.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- UnmanagedMemoryStreamWrapper.cs
- unsafeIndexingFilterStream.cs
- StreamInfo.cs
- DrawingCollection.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- DesignRelation.cs
- Privilege.cs
- InvalidOperationException.cs
- PartManifestEntry.cs
- TypeBuilder.cs