Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Ink / ElementsClipboardData.cs / 1305600 / ElementsClipboardData.cs
//---------------------------------------------------------------------------- // // File: ElementsClipboardData.cs // // Description: // A base class which can convert the clipboard data from/to FrameworkElement array // // Features: // // History: // 11/17/2004 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Controls; namespace MS.Internal.Ink { internal abstract class ElementsClipboardData : ClipboardData { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors // The default constructor internal ElementsClipboardData() { } // The constructor which takes a FrameworkElement array. internal ElementsClipboardData(UIElement[] elements) { if ( elements != null ) { ElementList = new List(elements); } } #endregion Constructors //-------------------------------------------------------------------------------- // // Internal Properties // //------------------------------------------------------------------------------- #region Internal Properties // Gets the element array. internal List Elements { get { if ( ElementList != null ) { return _elementList; } else { return new List (); } } } #endregion Internal Properties //-------------------------------------------------------------------------------- // // Protected Properties // //-------------------------------------------------------------------------------- #region Protected Properties // Sets/Gets the internal array list protected List ElementList { get { return _elementList; } set { _elementList = value; } } #endregion Protected Properties //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private List _elementList; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: ElementsClipboardData.cs // // Description: // A base class which can convert the clipboard data from/to FrameworkElement array // // Features: // // History: // 11/17/2004 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Controls; namespace MS.Internal.Ink { internal abstract class ElementsClipboardData : ClipboardData { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors // The default constructor internal ElementsClipboardData() { } // The constructor which takes a FrameworkElement array. internal ElementsClipboardData(UIElement[] elements) { if ( elements != null ) { ElementList = new List (elements); } } #endregion Constructors //-------------------------------------------------------------------------------- // // Internal Properties // //------------------------------------------------------------------------------- #region Internal Properties // Gets the element array. internal List Elements { get { if ( ElementList != null ) { return _elementList; } else { return new List (); } } } #endregion Internal Properties //-------------------------------------------------------------------------------- // // Protected Properties // //-------------------------------------------------------------------------------- #region Protected Properties // Sets/Gets the internal array list protected List ElementList { get { return _elementList; } set { _elementList = value; } } #endregion Protected Properties //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private List _elementList; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
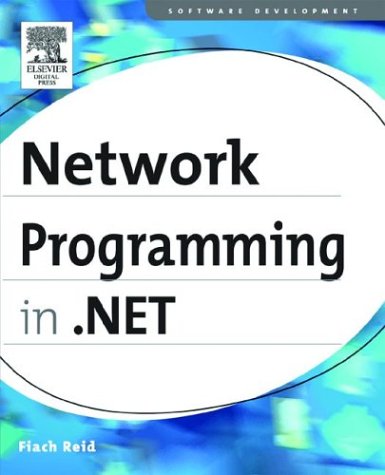
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Stacktrace.cs
- MemoryMappedViewAccessor.cs
- EntityContainer.cs
- MapPathBasedVirtualPathProvider.cs
- XmlQualifiedName.cs
- XPathSelectionIterator.cs
- TypeConstant.cs
- SafeBitVector32.cs
- ContentFileHelper.cs
- RoleService.cs
- TaskFormBase.cs
- TCEAdapterGenerator.cs
- NativeMethods.cs
- HandledMouseEvent.cs
- Globals.cs
- TraceFilter.cs
- WsdlImporterElement.cs
- MetabaseSettingsIis7.cs
- SQLMoney.cs
- CompilerResults.cs
- ObfuscationAttribute.cs
- TypeListConverter.cs
- FillRuleValidation.cs
- HtmlTableRowCollection.cs
- BinaryFormatterSinks.cs
- DataBoundControlDesigner.cs
- TogglePatternIdentifiers.cs
- WaitHandle.cs
- StaticFileHandler.cs
- SortFieldComparer.cs
- RewritingSimplifier.cs
- TextChangedEventArgs.cs
- DerivedKeySecurityToken.cs
- GroupByQueryOperator.cs
- TextBlockAutomationPeer.cs
- BinaryNode.cs
- CacheVirtualItemsEvent.cs
- CodeExpressionRuleDeclaration.cs
- TraceFilter.cs
- Serializer.cs
- ControlHelper.cs
- CryptoHelper.cs
- EmptyQuery.cs
- ExecutorLocksHeldException.cs
- TextServicesProperty.cs
- baseaxisquery.cs
- GPPOINTF.cs
- EventLogReader.cs
- AmbiguousMatchException.cs
- DbModificationClause.cs
- TextTreeText.cs
- TransformPatternIdentifiers.cs
- PropertyItemInternal.cs
- SqlNode.cs
- SqlDataSourceCache.cs
- WeakReferenceList.cs
- ObjectQueryProvider.cs
- SqlRewriteScalarSubqueries.cs
- PairComparer.cs
- RangeValidator.cs
- MDIWindowDialog.cs
- CaseStatement.cs
- CriticalHandle.cs
- XmlNode.cs
- sapiproxy.cs
- TemplateInstanceAttribute.cs
- SafeNativeMethodsMilCoreApi.cs
- ServiceHostFactory.cs
- EventDescriptorCollection.cs
- SqlNodeAnnotation.cs
- WebBrowserNavigatingEventHandler.cs
- MulticastOption.cs
- SessionParameter.cs
- ControllableStoryboardAction.cs
- CheckBox.cs
- TransformGroup.cs
- DocumentPaginator.cs
- OutputCacheSection.cs
- SafeHandles.cs
- WindowsStatusBar.cs
- LineSegment.cs
- XmlSchemaNotation.cs
- StatusBar.cs
- safelink.cs
- Lasso.cs
- ListSortDescription.cs
- SimpleWorkerRequest.cs
- ListDictionaryInternal.cs
- InternalMappingException.cs
- NativeMethods.cs
- BufferModeSettings.cs
- AuthorizationSection.cs
- WebRequest.cs
- DataColumnChangeEvent.cs
- EditorPart.cs
- BackStopAuthenticationModule.cs
- DataListItemCollection.cs
- FileDialog_Vista_Interop.cs
- DynamicDiscoSearcher.cs
- ArglessEventHandlerProxy.cs