Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / LambdaValue.cs / 1305376 / LambdaValue.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Expressions { using System; using System.Activities.ExpressionParser; using System.Activities.XamlIntegration; using System.Collections.Generic; using System.Linq.Expressions; using System.Runtime; using System.Windows.Markup; // consciously not XAML-friendly since Linq Expressions aren't create-set-use [Fx.Tag.XamlVisible(false)] public sealed class LambdaValue: CodeActivity , IExpressionContainer, IValueSerializableExpression { Func compiledLambdaValue; Expression > lambdaValue; Expression > rewrittenTree; public LambdaValue(Expression > lambdaValue) { Fx.Assert(lambdaValue != null, "valueExpression should not be null"); this.lambdaValue = lambdaValue; this.SkipArgumentResolution = true; } Expression IExpressionContainer.Expression { get { return this.lambdaValue; } } protected override void CacheMetadata(CodeActivityMetadata metadata) { // We need to rewrite the tree. Expression newTree; if (ExpressionUtilities.TryRewriteLambdaExpression(this.lambdaValue, out newTree, metadata)) { this.rewrittenTree = (Expression >)newTree; } else { this.rewrittenTree = this.lambdaValue; } } internal override bool TryGetValue(ActivityContext context, out TResult value) { if (this.compiledLambdaValue == null) { this.compiledLambdaValue = this.rewrittenTree.Compile(); } value = this.compiledLambdaValue(context); return true; } protected override TResult Execute(CodeActivityContext context) { return ExecuteWithTryGetValue(context); } public bool CanConvertToString(IValueSerializerContext context) { return true; } public string ConvertToString(IValueSerializerContext context) { // This workflow contains lambda expressions specified in code. // These expressions are not XAML serializable. // In order to make your workflow XAML-serializable, // use either VisualBasicValue/Reference or ExpressionServices.Convert // This will convert your lambda expressions into expression activities. throw FxTrace.Exception.AsError(new LambdaSerializationException()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
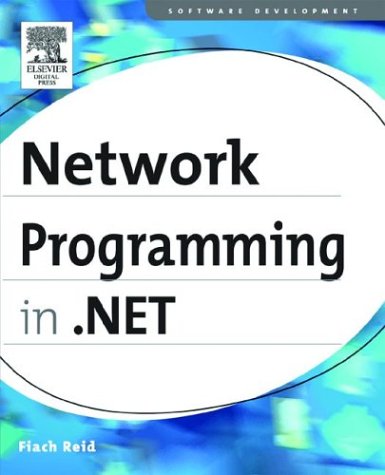
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridEntryCollection.cs
- IdentityNotMappedException.cs
- XhtmlBasicFormAdapter.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- _SslStream.cs
- PageCodeDomTreeGenerator.cs
- DriveInfo.cs
- MediaSystem.cs
- Wildcard.cs
- XmlNotation.cs
- HotSpotCollection.cs
- ModelItemDictionaryImpl.cs
- CounterSample.cs
- WebPart.cs
- WeakEventManager.cs
- DataList.cs
- MetadataItemEmitter.cs
- ExecutedRoutedEventArgs.cs
- VariableQuery.cs
- ComplexTypeEmitter.cs
- GenericPrincipal.cs
- XmlRawWriterWrapper.cs
- ActivityDefaults.cs
- FloaterBaseParagraph.cs
- WebPartEditVerb.cs
- XmlParserContext.cs
- ModelUIElement3D.cs
- SQLInt16Storage.cs
- PeerUnsafeNativeCryptMethods.cs
- TextBox.cs
- DialogResultConverter.cs
- UnsafeCollabNativeMethods.cs
- EnumBuilder.cs
- _ProxyChain.cs
- XmlSchemaSimpleContent.cs
- ProcessHost.cs
- EventsTab.cs
- documentsequencetextview.cs
- Italic.cs
- AutoScrollExpandMessageFilter.cs
- DataSourceConverter.cs
- NumberFormatInfo.cs
- LineMetrics.cs
- smtppermission.cs
- XmlSerializationGeneratedCode.cs
- WebGetAttribute.cs
- NumberFormatInfo.cs
- Bits.cs
- WorkflowOwnershipException.cs
- TagPrefixAttribute.cs
- IntermediatePolicyValidator.cs
- ZipFileInfo.cs
- DefaultPrintController.cs
- documentsequencetextcontainer.cs
- TimeoutException.cs
- MimeTextImporter.cs
- ExtendedTransformFactory.cs
- FixedDocument.cs
- Menu.cs
- AdRotator.cs
- ExeContext.cs
- AbstractSvcMapFileLoader.cs
- User.cs
- UserUseLicenseDictionaryLoader.cs
- HttpRawResponse.cs
- EngineSite.cs
- EnumerableRowCollectionExtensions.cs
- ObjectAssociationEndMapping.cs
- ConfigXmlDocument.cs
- DragAssistanceManager.cs
- HMACSHA384.cs
- XmlILAnnotation.cs
- EncodingInfo.cs
- SoapSchemaImporter.cs
- InputProcessorProfiles.cs
- TextTreeDeleteContentUndoUnit.cs
- DynamicPropertyHolder.cs
- ViewCellRelation.cs
- ReceiveActivityDesigner.cs
- MenuItem.cs
- DynamicPhysicalDiscoSearcher.cs
- ColumnResizeUndoUnit.cs
- HwndStylusInputProvider.cs
- StrongNameUtility.cs
- BuilderPropertyEntry.cs
- ObjectCloneHelper.cs
- RadioButton.cs
- DictionaryEntry.cs
- BinHexEncoder.cs
- WebConfigurationFileMap.cs
- ConnectionInterfaceCollection.cs
- ExceptionHandlerDesigner.cs
- WindowsClaimSet.cs
- Int32CAMarshaler.cs
- SystemNetworkInterface.cs
- ElementFactory.cs
- XamlSerializerUtil.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- PTConverter.cs
- ColorAnimation.cs