Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / DataSourceConverter.cs / 1 / DataSourceConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.CodeDom; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Diagnostics; using System.Runtime.InteropServices; using System.Globalization; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class DataSourceConverter : TypeConverter { ////// Provides design-time support for a component's data source property. /// ////// /// public DataSourceConverter() { } ////// Initializes a new instance of ///. /// /// /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return false; } ////// Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. /// ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { return String.Empty; } else if (value.GetType() == typeof(string)) { return (string)value; } throw GetConvertFromException(value); } ////// Converts the given object to the converter's native type. /// ////// /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { object[] names = null; if (context != null) { ArrayList list = new ArrayList(); IContainer cont = context.Container; if (cont != null) { ComponentCollection objs = cont.Components; foreach (IComponent comp in (IEnumerable)objs) { if (IsValidDataSource(comp) && !Marshal.IsComObject(comp)) { PropertyDescriptor modifierProp = TypeDescriptor.GetProperties(comp)["Modifiers"]; if (modifierProp != null) { MemberAttributes modifiers = (MemberAttributes)modifierProp.GetValue(comp); if ((modifiers & MemberAttributes.AccessMask) == MemberAttributes.Private) { // must be declared as public or protected continue; } } ISite site = comp.Site; if (site != null) { string name = site.Name; if (name != null) { list.Add(name); } } } } } names = list.ToArray(); Array.Sort(names, Comparer.Default); } return new StandardValuesCollection(names); } ////// Gets the standard data sources accessible to the control. /// ////// /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// /// /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } protected virtual bool IsValidDataSource(IComponent component) { return (component is IEnumerable) || (component is IListSource); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. /// ///
Link Menu
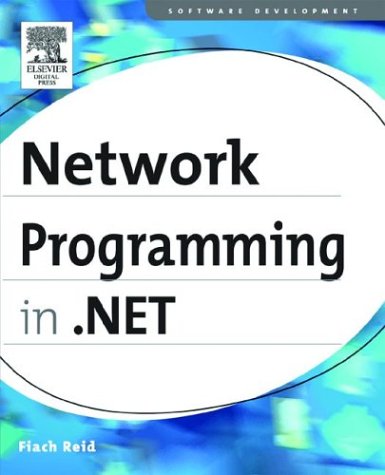
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AdCreatedEventArgs.cs
- DataGridColumn.cs
- RectangleF.cs
- Application.cs
- WebPartEditorCancelVerb.cs
- SiteMapNodeCollection.cs
- EdmItemCollection.cs
- InternalCache.cs
- DetailsViewRow.cs
- NonSerializedAttribute.cs
- AspNetSynchronizationContext.cs
- SoapFormatter.cs
- SqlDependency.cs
- AssemblyCache.cs
- XmlFormatExtensionPointAttribute.cs
- TextParentUndoUnit.cs
- DeflateInput.cs
- WorkflowQueuingService.cs
- wgx_sdk_version.cs
- ScaleTransform3D.cs
- WsdlWriter.cs
- AsyncResult.cs
- TextStore.cs
- XMLSchema.cs
- TextTreePropertyUndoUnit.cs
- DecimalConstantAttribute.cs
- LabelEditEvent.cs
- UnionCodeGroup.cs
- CacheRequest.cs
- UnauthorizedWebPart.cs
- InvokeSchedule.cs
- WebServiceReceiveDesigner.cs
- ExpressionPrefixAttribute.cs
- UniqueSet.cs
- PopupRoot.cs
- Bits.cs
- DynamicMethod.cs
- Simplifier.cs
- FontWeight.cs
- NoClickablePointException.cs
- DataViewManager.cs
- MailFileEditor.cs
- MetadataItem.cs
- FontStretch.cs
- UserNamePasswordValidationMode.cs
- FontUnit.cs
- StorageBasedPackageProperties.cs
- NullableDoubleMinMaxAggregationOperator.cs
- ListManagerBindingsCollection.cs
- PrincipalPermission.cs
- TextTrailingCharacterEllipsis.cs
- BitmapImage.cs
- XmlSchemaComplexContentRestriction.cs
- OleAutBinder.cs
- SafeNativeMemoryHandle.cs
- DbDataReader.cs
- Currency.cs
- TextOptionsInternal.cs
- FormatException.cs
- DesignTimeTemplateParser.cs
- FormClosedEvent.cs
- TimeSpan.cs
- StreamGeometryContext.cs
- Wildcard.cs
- OutputCacheSection.cs
- ObjectStateEntry.cs
- DefaultValueTypeConverter.cs
- SoapServerMethod.cs
- ResXResourceReader.cs
- GetPageCompletedEventArgs.cs
- BuildProviderAppliesToAttribute.cs
- AdornerLayer.cs
- EntityContainerEmitter.cs
- XmlDataSource.cs
- XmlBoundElement.cs
- RequestUriProcessor.cs
- CatalogPartCollection.cs
- PropertyDescriptorGridEntry.cs
- DeclarativeCatalogPart.cs
- MatrixStack.cs
- ClientSideProviderDescription.cs
- TextServicesCompartment.cs
- EnumerableWrapperWeakToStrong.cs
- RoutingExtensionElement.cs
- CircleHotSpot.cs
- ACL.cs
- ObjectDataProvider.cs
- OneWayChannelFactory.cs
- AttributeCallbackBuilder.cs
- SmiGettersStream.cs
- PointValueSerializer.cs
- DateTimeOffsetAdapter.cs
- MetafileHeaderEmf.cs
- GestureRecognitionResult.cs
- CodeConstructor.cs
- OleDbDataAdapter.cs
- DataGridViewColumnEventArgs.cs
- InfoCardBinaryReader.cs
- ResponseBodyWriter.cs
- WindowsTitleBar.cs