Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / StreamGeometryContext.cs / 1 / StreamGeometryContext.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This class is used by the StreamGeometry class to generate an inlined, // flattened geometry stream. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.Security; using System.Security.Permissions; #if !PBTCOMPILER using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Effects; using System.Windows.Media.Imaging; using System.Windows.Media.Media3D; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Internal.PresentationCore; namespace System.Windows.Media #elif PBTCOMPILER using MS.Internal.Markup; namespace MS.Internal.Markup #endif { ////// StreamGeometryContext /// #if ! PBTCOMPILER public abstract class StreamGeometryContext : DispatcherObject, IDisposable #else internal abstract class StreamGeometryContext : IDisposable #endif { #region Constructors ////// This constructor exists to prevent external derivation /// internal StreamGeometryContext() { } #endregion Constructors #region IDisposable void IDisposable.Dispose() { #if ! PBTCOMPILER VerifyAccess(); #endif DisposeCore(); } #endregion IDisposable #region Public Methods ////// Closes the StreamContext and flushes the content. /// Afterwards the StreamContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// public virtual void Close() { DisposeCore(); } ////// BeginFigure - Start a new figure. /// public abstract void BeginFigure(Point startPoint, bool isFilled, bool isClosed); ////// LineTo - append a LineTo to the current figure. /// public abstract void LineTo(Point point, bool isStroked, bool isSmoothJoin); ////// QuadraticBezierTo - append a QuadraticBezierTo to the current figure. /// public abstract void QuadraticBezierTo(Point point1, Point point2, bool isStroked, bool isSmoothJoin); ////// BezierTo - apply a BezierTo to the current figure. /// public abstract void BezierTo(Point point1, Point point2, Point point3, bool isStroked, bool isSmoothJoin); ////// PolyLineTo - append a PolyLineTo to the current figure. /// public abstract void PolyLineTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// PolyQuadraticBezierTo - append a PolyQuadraticBezierTo to the current figure. /// public abstract void PolyQuadraticBezierTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// PolyBezierTo - append a PolyBezierTo to the current figure. /// public abstract void PolyBezierTo(IListpoints, bool isStroked, bool isSmoothJoin); /// /// ArcTo - append an ArcTo to the current figure. /// // Special case this one. Bringing in sweep direction requires code-gen changes. // #if !PBTCOMPILER public abstract void ArcTo(Point point, Size size, double rotationAngle, bool isLargeArc, SweepDirection sweepDirection, bool isStroked, bool isSmoothJoin); #else public abstract void ArcTo(Point point, Size size, double rotationAngle, bool isLargeArc, bool sweepDirection, bool isStroked, bool isSmoothJoin); #endif #endregion Public Methods ////// This is the same as the Close call: /// Closes the Context and flushes the content. /// Afterwards the Context can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// internal virtual void DisposeCore() {} ////// SetClosedState - Sets the current closed state of the figure. /// internal abstract void SetClosedState(bool closed); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
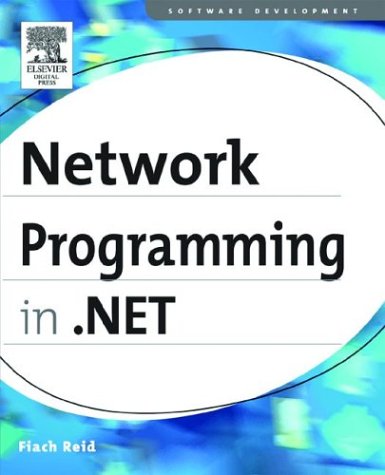
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CallTemplateAction.cs
- DataListItem.cs
- Image.cs
- Bitmap.cs
- PerformanceCounterPermissionEntry.cs
- Brushes.cs
- DSGeneratorProblem.cs
- AutomationIdentifier.cs
- PersistenceProviderBehavior.cs
- SafeViewOfFileHandle.cs
- HtmlFormWrapper.cs
- DbConnectionPoolOptions.cs
- TokenBasedSet.cs
- DSACryptoServiceProvider.cs
- ProtocolsConfigurationEntry.cs
- BrowserTree.cs
- JulianCalendar.cs
- SafeRegistryHandle.cs
- VerbConverter.cs
- DesignerSerializationOptionsAttribute.cs
- XmlMtomWriter.cs
- FloaterBaseParaClient.cs
- Accessible.cs
- ColumnMapTranslator.cs
- StorageAssociationTypeMapping.cs
- DataRecordInternal.cs
- EnumUnknown.cs
- PageContentAsyncResult.cs
- CorrelationManager.cs
- TableRow.cs
- ExternalDataExchangeService.cs
- WebEvents.cs
- JoinElimination.cs
- BooleanAnimationBase.cs
- PrintDialog.cs
- PropertyGroupDescription.cs
- LinqDataView.cs
- Popup.cs
- ListMarkerLine.cs
- TryExpression.cs
- TogglePattern.cs
- OdbcErrorCollection.cs
- ICollection.cs
- AmbientProperties.cs
- PowerStatus.cs
- FrameworkReadOnlyPropertyMetadata.cs
- CuspData.cs
- _HelperAsyncResults.cs
- DebugView.cs
- TargetException.cs
- OdbcRowUpdatingEvent.cs
- DetailsViewInsertedEventArgs.cs
- ListBase.cs
- SimplePropertyEntry.cs
- PathFigureCollection.cs
- SamlEvidence.cs
- Point3DAnimationUsingKeyFrames.cs
- EntityContainer.cs
- HashAlgorithm.cs
- glyphs.cs
- BitmapEffectInputConnector.cs
- ReadOnlyDictionary.cs
- PageCatalogPart.cs
- TaskDesigner.cs
- TraceListener.cs
- StorageMappingItemLoader.cs
- SystemWebExtensionsSectionGroup.cs
- XmlElementCollection.cs
- XmlEncoding.cs
- ContainerFilterService.cs
- XmlAttributeCollection.cs
- WindowsMenu.cs
- TextEditorContextMenu.cs
- webproxy.cs
- RegexReplacement.cs
- SqlDependencyUtils.cs
- TemplateBindingExtension.cs
- Double.cs
- ThicknessAnimationBase.cs
- DbProviderServices.cs
- CounterSampleCalculator.cs
- KeyValueInternalCollection.cs
- TextElement.cs
- SettingsSection.cs
- CodeTypeMemberCollection.cs
- NumberFormatter.cs
- LateBoundBitmapDecoder.cs
- StoreContentChangedEventArgs.cs
- DataGrid.cs
- StreamUpdate.cs
- TimersDescriptionAttribute.cs
- Frame.cs
- BrowserInteropHelper.cs
- PageThemeParser.cs
- VectorAnimation.cs
- PropertyCollection.cs
- DataGridViewCellCollection.cs
- GridViewHeaderRowPresenter.cs
- SubstitutionDesigner.cs
- ObjectStateFormatter.cs