Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / ICollection.cs / 1305376 / ICollection.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Interface: ICollection ** **[....] ** ** ** Purpose: Base interface for all collections. ** ** ===========================================================*/ namespace System.Collections { using System; using System.Diagnostics.Contracts; // Base interface for all collections, defining enumerators, size, and // synchronization methods. [ContractClass(typeof(ICollectionContract))] [System.Runtime.InteropServices.ComVisible(true)] public interface ICollection : IEnumerable { // Interfaces are not serialable // CopyTo copies a collection into an Array, starting at a particular // index into the array. // void CopyTo(Array array, int index); // Number of items in the collections. int Count { get; } // SyncRoot will return an Object to use for synchronization // (thread safety). You can use this object in your code to take a // lock on the collection, even if this collection is a wrapper around // another collection. The intent is to tunnel through to a real // implementation of a collection, and use one of the internal objects // found in that code. // // In the absense of a static Synchronized method on a collection, // the expected usage for SyncRoot would look like this: // // ICollection col = ... // lock (col.SyncRoot) { // // Some operation on the collection, which is now thread safe. // // This may include multiple operations. // } // // // The system-provided collections have a static method called // Synchronized which will create a thread-safe wrapper around the // collection. All access to the collection that you want to be // thread-safe should go through that wrapper collection. However, if // you need to do multiple calls on that collection (such as retrieving // two items, or checking the count then doing something), you should // NOT use our thread-safe wrapper since it only takes a lock for the // duration of a single method call. Instead, use Monitor.Enter/Exit // or your language's equivalent to the C# lock keyword as mentioned // above. // // For collections with no publically available underlying store, the // expected implementation is to simply return the this pointer. Note // that the this pointer may not be sufficient for collections that // wrap other collections; those should return the underlying // collection's SyncRoot property. Object SyncRoot { get; } // Is this collection synchronized (i.e., thread-safe)? If you want a // thread-safe collection, you can use SyncRoot as an object to // synchronize your collection with. If you're using one of the // collections in System.Collections, you could call the static // Synchronized method to get a thread-safe wrapper around the // underlying collection. bool IsSynchronized { get; } } [ContractClassFor(typeof(ICollection))] internal class ICollectionContract : ICollection { void ICollection.CopyTo(Array array, int index) { } int ICollection.Count { get { Contract.Ensures(Contract.Result() >= 0); return default(int); } } Object ICollection.SyncRoot { get { Contract.Ensures(Contract.Result
Link Menu
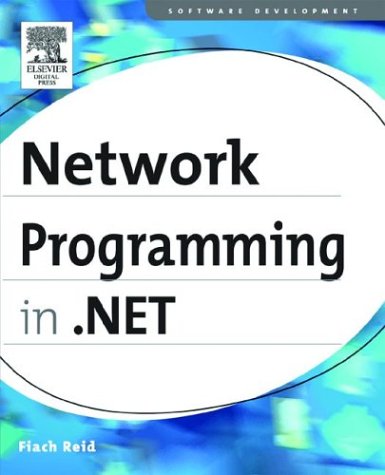
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyWorkItem.cs
- ColorDialog.cs
- WebPartVerbsEventArgs.cs
- Size3D.cs
- WebServiceParameterData.cs
- CaseStatementSlot.cs
- DataServiceRequest.cs
- DialogResultConverter.cs
- Property.cs
- ConfigXmlWhitespace.cs
- EntityDataSourceDataSelectionPanel.cs
- CaseStatementSlot.cs
- PenThreadPool.cs
- ValueChangedEventManager.cs
- Executor.cs
- BitmapEffect.cs
- DrawListViewSubItemEventArgs.cs
- TreeViewItemAutomationPeer.cs
- CachedPathData.cs
- DictionaryItemsCollection.cs
- ExeConfigurationFileMap.cs
- SimpleBitVector32.cs
- Span.cs
- _ContextAwareResult.cs
- LockedBorderGlyph.cs
- FormatConvertedBitmap.cs
- CommittableTransaction.cs
- RequestCachingSection.cs
- ImageSourceConverter.cs
- InternalControlCollection.cs
- IgnoreFileBuildProvider.cs
- ConditionalWeakTable.cs
- FrameSecurityDescriptor.cs
- ColumnClickEvent.cs
- ApplicationException.cs
- ConstraintManager.cs
- CustomLineCap.cs
- MatrixAnimationUsingPath.cs
- Image.cs
- IDQuery.cs
- DataList.cs
- ScalarOps.cs
- SqlUdtInfo.cs
- XmlAutoDetectWriter.cs
- AudioDeviceOut.cs
- LayoutTableCell.cs
- SqlCacheDependencySection.cs
- RemotingConfigParser.cs
- FtpWebResponse.cs
- WebPartZoneCollection.cs
- ExternalCalls.cs
- JoinSymbol.cs
- SplitContainer.cs
- VisualTreeHelper.cs
- ApplicationInfo.cs
- CompensableActivity.cs
- BrowserCapabilitiesCompiler.cs
- ComponentTray.cs
- EncoderNLS.cs
- Msec.cs
- LinqDataSourceValidationException.cs
- PageContent.cs
- GeometryCombineModeValidation.cs
- XmlSchemaAttribute.cs
- XmlSchemaSubstitutionGroup.cs
- PriorityChain.cs
- TaiwanLunisolarCalendar.cs
- SynchronizedInputHelper.cs
- UpDownEvent.cs
- UpdateDelegates.Generated.cs
- DefaultProxySection.cs
- XmlSchemaCompilationSettings.cs
- DSASignatureDeformatter.cs
- TypeElement.cs
- Trace.cs
- XmlSchemaRedefine.cs
- Constants.cs
- Brush.cs
- ContentWrapperAttribute.cs
- GridSplitterAutomationPeer.cs
- Annotation.cs
- Button.cs
- ParagraphVisual.cs
- Codec.cs
- ExtractorMetadata.cs
- HashRepartitionEnumerator.cs
- EntityDataSourceColumn.cs
- PageVisual.cs
- cookie.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- Encoder.cs
- XmlDataSourceView.cs
- ContentType.cs
- AppDomain.cs
- TransformPattern.cs
- AnnotationResourceCollection.cs
- LineServicesRun.cs
- XPathNodeIterator.cs
- PersonalizationAdministration.cs
- XmlEncodedRawTextWriter.cs