Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / SqlClient / SqlGen / JoinSymbol.cs / 2 / JoinSymbol.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// A Join symbol is a special kind of Symbol. /// It has to carry additional information /// internal sealed class JoinSymbol : Symbol { private List///
/// /// All the lists are set exactly once, and then used for lookups/enumerated. ///- ColumnList for the list of columns in the select clause if this /// symbol represents a sql select statement. This is set by
///. - ExtentList is the list of extents in the select clause.
///- FlattenedExtentList - if the Join has multiple extents flattened at the /// top level, we need this information to ensure that extent aliases are renamed /// correctly in
///- NameToExtent has all the extents in ExtentList as a dictionary. /// This is used by
///to flatten /// record accesses. - IsNestedJoin - is used to determine whether a JoinSymbol is an /// ordinary join symbol, or one that has a corresponding SqlSelectStatement.
///columnList; internal List ColumnList { get { if (null == columnList) { columnList = new List (); } return columnList; } set { columnList = value; } } private List extentList; internal List ExtentList { get { return extentList; } } private List flattenedExtentList; internal List FlattenedExtentList { get { if (null == flattenedExtentList) { flattenedExtentList = new List (); } return flattenedExtentList; } set { flattenedExtentList = value; } } private Dictionary nameToExtent; internal Dictionary NameToExtent { get { return nameToExtent; } } private bool isNestedJoin; internal bool IsNestedJoin { get { return isNestedJoin; } set { isNestedJoin = value; } } public JoinSymbol(string name, TypeUsage type, List extents) : base(name, type) { extentList = new List (extents.Count); nameToExtent = new Dictionary (extents.Count, StringComparer.OrdinalIgnoreCase); foreach (Symbol symbol in extents) { this.nameToExtent[symbol.Name] = symbol; this.ExtentList.Add(symbol); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// A Join symbol is a special kind of Symbol. /// It has to carry additional information /// internal sealed class JoinSymbol : Symbol { private List///
/// /// All the lists are set exactly once, and then used for lookups/enumerated. ///- ColumnList for the list of columns in the select clause if this /// symbol represents a sql select statement. This is set by
///. - ExtentList is the list of extents in the select clause.
///- FlattenedExtentList - if the Join has multiple extents flattened at the /// top level, we need this information to ensure that extent aliases are renamed /// correctly in
///- NameToExtent has all the extents in ExtentList as a dictionary. /// This is used by
///to flatten /// record accesses. - IsNestedJoin - is used to determine whether a JoinSymbol is an /// ordinary join symbol, or one that has a corresponding SqlSelectStatement.
///columnList; internal List ColumnList { get { if (null == columnList) { columnList = new List (); } return columnList; } set { columnList = value; } } private List extentList; internal List ExtentList { get { return extentList; } } private List flattenedExtentList; internal List FlattenedExtentList { get { if (null == flattenedExtentList) { flattenedExtentList = new List (); } return flattenedExtentList; } set { flattenedExtentList = value; } } private Dictionary nameToExtent; internal Dictionary NameToExtent { get { return nameToExtent; } } private bool isNestedJoin; internal bool IsNestedJoin { get { return isNestedJoin; } set { isNestedJoin = value; } } public JoinSymbol(string name, TypeUsage type, List extents) : base(name, type) { extentList = new List (extents.Count); nameToExtent = new Dictionary (extents.Count, StringComparer.OrdinalIgnoreCase); foreach (Symbol symbol in extents) { this.nameToExtent[symbol.Name] = symbol; this.ExtentList.Add(symbol); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
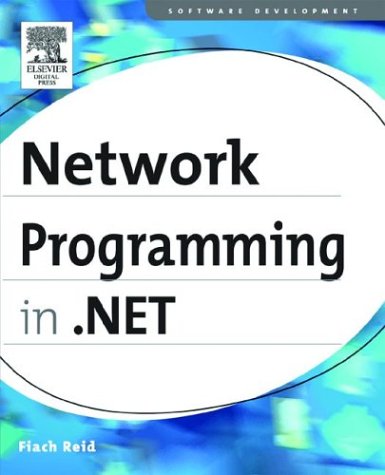
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StateDesigner.TransitionInfo.cs
- TreeNodeCollection.cs
- KeysConverter.cs
- XmlSerializationReader.cs
- IODescriptionAttribute.cs
- XmlSchemas.cs
- PropertyCondition.cs
- BCryptNative.cs
- LicenseProviderAttribute.cs
- RichTextBoxConstants.cs
- SqlDependencyListener.cs
- DecimalConstantAttribute.cs
- AssemblyBuilderData.cs
- Configuration.cs
- Simplifier.cs
- HttpProfileBase.cs
- ScaleTransform.cs
- AssemblyHash.cs
- DeviceContexts.cs
- ActiveXContainer.cs
- ControlTemplate.cs
- HiddenFieldPageStatePersister.cs
- ServiceRoute.cs
- DataGridRow.cs
- CryptographicAttribute.cs
- DataTemplateKey.cs
- EndOfStreamException.cs
- HtmlTitle.cs
- FastEncoderWindow.cs
- WindowClosedEventArgs.cs
- InternalConfigEventArgs.cs
- ProfileServiceManager.cs
- SynchronizedChannelCollection.cs
- CodeMethodInvokeExpression.cs
- MailFileEditor.cs
- HandlerBase.cs
- BufferBuilder.cs
- Module.cs
- dsa.cs
- RadioButtonRenderer.cs
- FileDialog.cs
- PagedDataSource.cs
- JsonReaderWriterFactory.cs
- OdbcConnectionHandle.cs
- _Rfc2616CacheValidators.cs
- Helpers.cs
- OpCodes.cs
- PageHandlerFactory.cs
- ActivitiesCollection.cs
- HuffModule.cs
- DataViewManagerListItemTypeDescriptor.cs
- InteropAutomationProvider.cs
- PropertyGridCommands.cs
- MetabaseReader.cs
- EventWaitHandleSecurity.cs
- ProbeDuplexAsyncResult.cs
- MulticastIPAddressInformationCollection.cs
- Relationship.cs
- AddIn.cs
- ItemsControlAutomationPeer.cs
- ContentElementAutomationPeer.cs
- XmlAttributeCollection.cs
- PrintDialog.cs
- x509utils.cs
- CallbackHandler.cs
- ThreadInterruptedException.cs
- ActivityDesigner.cs
- ADMembershipUser.cs
- SelectionItemProviderWrapper.cs
- GridViewColumnHeaderAutomationPeer.cs
- UniqueContractNameValidationBehavior.cs
- TreeViewBindingsEditorForm.cs
- AlternateView.cs
- BitmapEffectGroup.cs
- PeerEndPoint.cs
- ToolbarAUtomationPeer.cs
- DataBoundLiteralControl.cs
- AjaxFrameworkAssemblyAttribute.cs
- XsltOutput.cs
- ViewStateModeByIdAttribute.cs
- ConfigurationManager.cs
- SoapMessage.cs
- SymDocumentType.cs
- SettingsContext.cs
- RtfToXamlLexer.cs
- InvalidCastException.cs
- DataKey.cs
- MsmqBindingFilter.cs
- PageAdapter.cs
- TextPattern.cs
- LogEntryHeaderSerializer.cs
- DocumentPageTextView.cs
- BooleanExpr.cs
- EventsTab.cs
- ClientSettingsStore.cs
- Conditional.cs
- RegexFCD.cs
- FlagsAttribute.cs
- SchemaEntity.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs