Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / schema / SchemaEntity.cs / 1 / SchemaEntity.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System; using System.Diagnostics; using System.Net; internal sealed class SchemaEntity { private XmlQualifiedName name; // Name of entity private String url; // Url for external entity (system id) private String pubid; // Pubid for external entity private String text; // Text for internal entity private XmlQualifiedName ndata = XmlQualifiedName.Empty; // NDATA identifier private int lineNumber; // line number private int linePosition; // character postion private bool isParameter; // parameter entity flag private bool isExternal; // external entity flag private bool isProcessed; // whether entity is being Processed. (infinite recurrsion check) private bool isDeclaredInExternal; // declared in external markup or not private string baseURI; private string declaredURI; internal SchemaEntity(XmlQualifiedName name, bool isParameter) { this.name = name; this.isParameter = isParameter; } internal static bool IsPredefinedEntity(String n) { return(n == "lt" || n == "gt" || n == "amp" || n == "apos" || n == "quot"); } internal XmlQualifiedName Name { get { return name;} } internal String Url { get { return url;} set { url = value; isExternal = true;} } internal String Pubid { get { return pubid;} set { pubid = value;} } internal bool IsProcessed { get { return isProcessed;} set { isProcessed = value;} } internal bool IsExternal { get { return isExternal;} set { isExternal = value;} } internal bool DeclaredInExternal { get { return isDeclaredInExternal;} set { isDeclaredInExternal = value;} } internal bool IsParEntity { get { return isParameter;} set { isParameter = value;} } internal XmlQualifiedName NData { get { return ndata;} set { ndata = value;} } internal String Text { get { return text;} set { text = value; isExternal = false;} } internal int Line { get { return lineNumber;} set { lineNumber = value;} } internal int Pos { get { return linePosition;} set { linePosition = value;} } internal String BaseURI { get { return (baseURI == null) ? String.Empty : baseURI; } set { baseURI = value; } } internal String DeclaredURI { get { return (declaredURI == null) ? String.Empty : declaredURI; } set { declaredURI = value; } } }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
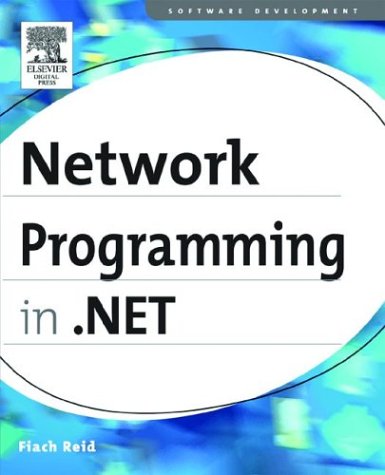
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlDigitalSignatureProcessor.cs
- Scheduling.cs
- BitmapEffectInputConnector.cs
- Menu.cs
- Triangle.cs
- AsymmetricSignatureDeformatter.cs
- Point4DConverter.cs
- Header.cs
- Win32Exception.cs
- BamlReader.cs
- FreeFormDesigner.cs
- LabelDesigner.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- PropertySourceInfo.cs
- UriTemplateLiteralQueryValue.cs
- AssemblySettingAttributes.cs
- ChameleonKey.cs
- CheckBoxList.cs
- TextRange.cs
- RewritingValidator.cs
- EmptyWorkItem.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- StorageInfo.cs
- BlobPersonalizationState.cs
- GPRECTF.cs
- ActivityCodeDomSerializer.cs
- ImageDrawing.cs
- WebPartConnectVerb.cs
- RightsManagementSuppressedStream.cs
- PtsContext.cs
- GrammarBuilderPhrase.cs
- Logging.cs
- ElementAtQueryOperator.cs
- DataTableMapping.cs
- NetworkInformationException.cs
- HtmlString.cs
- ConfigXmlComment.cs
- MultiView.cs
- CustomSignedXml.cs
- SqlConnectionString.cs
- JsonSerializer.cs
- DES.cs
- IisTraceListener.cs
- ProjectionPruner.cs
- IndependentAnimationStorage.cs
- SelectionService.cs
- ReflectEventDescriptor.cs
- ResourceType.cs
- NumberEdit.cs
- StateRuntime.cs
- XPathNodeList.cs
- KoreanCalendar.cs
- Divide.cs
- GlyphCollection.cs
- DoubleAnimationClockResource.cs
- TreeView.cs
- AttributeUsageAttribute.cs
- StringOutput.cs
- _SpnDictionary.cs
- DataGridColumnCollection.cs
- XmlArrayAttribute.cs
- NotifyInputEventArgs.cs
- ControlType.cs
- CodeNamespace.cs
- BaseCodePageEncoding.cs
- HwndProxyElementProvider.cs
- ClientCredentialsSecurityTokenManager.cs
- FontWeight.cs
- DesignerContextDescriptor.cs
- GridView.cs
- TablePatternIdentifiers.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- MultiSelectRootGridEntry.cs
- TabPanel.cs
- MetadataArtifactLoaderFile.cs
- Exceptions.cs
- EventListenerClientSide.cs
- ExpandSegment.cs
- TileModeValidation.cs
- DrawingGroup.cs
- GacUtil.cs
- Vector.cs
- StsCommunicationException.cs
- NamespaceEmitter.cs
- GreenMethods.cs
- ThreadAbortException.cs
- MemberCollection.cs
- ImageSourceConverter.cs
- TableHeaderCell.cs
- ObjectDataSourceStatusEventArgs.cs
- sortedlist.cs
- DataMisalignedException.cs
- NativeMethods.cs
- MappingMetadataHelper.cs
- TypeUtil.cs
- NonBatchDirectoryCompiler.cs
- NodeFunctions.cs
- ContentTextAutomationPeer.cs
- LineInfo.cs
- TrustManagerPromptUI.cs