Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / Column.cs / 1305376 / Column.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web; using System.Web.UI; ////// Creates a column and is the base class for all [ TypeConverterAttribute(typeof(ExpandableObjectConverter)) ] public abstract class DataGridColumn : IStateManager { private DataGrid owner; private TableItemStyle itemStyle; private TableItemStyle headerStyle; private TableItemStyle footerStyle; private StateBag statebag; private bool marked; ///column types. /// /// protected DataGridColumn() { statebag = new StateBag(); } ///Initializes a new instance of the System.Web.UI.WebControls.Column class. ////// protected bool DesignMode { get { if (owner != null) { return owner.DesignMode; } return false; } } ///[To be supplied.] ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataGridColumn_FooterStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public virtual TableItemStyle FooterStyle { get { if (footerStyle == null) { footerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)footerStyle).TrackViewState(); } return footerStyle; } } ///Gets the style properties for the footer item. ////// internal TableItemStyle FooterStyleInternal { get { return footerStyle; } } ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataGridColumn_FooterText) ] public virtual string FooterText { get { object o = ViewState["FooterText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["FooterText"] = value; OnColumnChanged(); } } ///Gets or sets the text displayed in the footer of the /// System.Web.UI.WebControls.Column. ////// [ WebCategory("Appearance"), DefaultValue(""), UrlProperty(), WebSysDescription(SR.DataGridColumn_HeaderImageUrl) ] public virtual string HeaderImageUrl { get { object o = ViewState["HeaderImageUrl"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["HeaderImageUrl"] = value; OnColumnChanged(); } } ///Gets or sets the URL reference to an image to display /// instead of text on the header of this System.Web.UI.WebControls.Column /// . ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataGridColumn_HeaderStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public virtual TableItemStyle HeaderStyle { get { if (headerStyle == null) { headerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)headerStyle).TrackViewState(); } return headerStyle; } } ///Gets the style properties for the header of the System.Web.UI.WebControls.Column. This property is read-only. ////// internal TableItemStyle HeaderStyleInternal { get { return headerStyle; } } ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataGridColumn_HeaderText) ] public virtual string HeaderText { get { object o = ViewState["HeaderText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["HeaderText"] = value; OnColumnChanged(); } } ///Gets or sets the text displayed in the header of the /// System.Web.UI.WebControls.Column. ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataGridColumn_ItemStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public virtual TableItemStyle ItemStyle { get { if (itemStyle == null) { itemStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)itemStyle).TrackViewState(); } return itemStyle; } } ///Gets the style properties of an item within the System.Web.UI.WebControls.Column. This property is read-only. ////// internal TableItemStyle ItemStyleInternal { get { return itemStyle; } } ////// protected DataGrid Owner { get { return owner; } } ///Gets the System.Web.UI.WebControls.DataGrid that the System.Web.UI.WebControls.Column is a part of. This property is read-only. ////// [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.DataGridColumn_SortExpression) ] public virtual string SortExpression { get { object o = ViewState["SortExpression"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["SortExpression"] = value; OnColumnChanged(); } } ///Gets or sets the expression used when this column is used to sort the data source> by. ////// protected StateBag ViewState { get { return statebag; } } ///Gets the statebag for the System.Web.UI.WebControls.Column. This property is read-only. ////// [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataGridColumn_Visible) ] public bool Visible { get { object o = ViewState["Visible"]; if (o != null) return(bool)o; return true; } set { ViewState["Visible"] = value; OnColumnChanged(); } } ///Gets or sets a value to indicate whether the System.Web.UI.WebControls.Column is visible. ////// public virtual void Initialize() { } ////// public virtual void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { switch (itemType) { case ListItemType.Header: { WebControl headerControl = null; bool sortableHeader = true; string sortExpression = null; if ((owner != null) && (owner.AllowSorting == false)) { sortableHeader = false; } if (sortableHeader) { sortExpression = SortExpression; if (sortExpression.Length == 0) sortableHeader = false; } string headerImageUrl = HeaderImageUrl; if (headerImageUrl.Length != 0) { if (sortableHeader) { ImageButton sortButton = new ImageButton(); sortButton.ImageUrl = HeaderImageUrl; sortButton.CommandName = DataGrid.SortCommandName; sortButton.CommandArgument = sortExpression; sortButton.CausesValidation = false; headerControl = sortButton; } else { Image headerImage = new Image(); headerImage.ImageUrl = headerImageUrl; headerControl = headerImage; } } else { string headerText = HeaderText; if (sortableHeader) { LinkButton sortButton = new DataGridLinkButton(); sortButton.Text = headerText; sortButton.CommandName = DataGrid.SortCommandName; sortButton.CommandArgument = sortExpression; sortButton.CausesValidation = false; headerControl = sortButton; } else { if (headerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. headerText = " "; } cell.Text = headerText; } } if (headerControl != null) { cell.Controls.Add(headerControl); } } break; case ListItemType.Footer: { string footerText = FooterText; if (footerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. footerText = " "; } cell.Text = footerText; } break; } } ///Initializes a cell in the System.Web.UI.WebControls.Column. ////// protected bool IsTrackingViewState { get { return marked; } } ///Determines if the System.Web.UI.WebControls.Column is marked to save its state. ////// protected virtual void LoadViewState(object savedState) { if (savedState != null) { object[] myState = (object[])savedState; if (myState[0] != null) ((IStateManager)ViewState).LoadViewState(myState[0]); if (myState[1] != null) ((IStateManager)ItemStyle).LoadViewState(myState[1]); if (myState[2] != null) ((IStateManager)HeaderStyle).LoadViewState(myState[2]); if (myState[3] != null) ((IStateManager)FooterStyle).LoadViewState(myState[3]); } } ///Loads the state of the System.Web.UI.WebControls.Column. ////// protected virtual void TrackViewState() { marked = true; ((IStateManager)ViewState).TrackViewState(); if (itemStyle != null) ((IStateManager)itemStyle).TrackViewState(); if (headerStyle != null) ((IStateManager)headerStyle).TrackViewState(); if (footerStyle != null) ((IStateManager)footerStyle).TrackViewState(); } ///Marks the starting point to begin tracking and saving changes to the /// control as part of the control viewstate. ////// protected virtual void OnColumnChanged() { if (owner != null) { owner.OnColumnsChanged(); } } ///Raises the ColumnChanged event for a System.Web.UI.WebControls.Column. ////// protected virtual object SaveViewState() { object propState = ((IStateManager)ViewState).SaveViewState(); object itemStyleState = (itemStyle != null) ? ((IStateManager)itemStyle).SaveViewState() : null; object headerStyleState = (headerStyle != null) ? ((IStateManager)headerStyle).SaveViewState() : null; object footerStyleState = (footerStyle != null) ? ((IStateManager)footerStyle).SaveViewState() : null; if ((propState != null) || (itemStyleState != null) || (headerStyleState != null) || (footerStyleState != null)) { return new object[4] { propState, itemStyleState, headerStyleState, footerStyleState }; } return null; } ///Saves the current state of the System.Web.UI.WebControls.Column. ////// internal void SetOwner(DataGrid owner) { this.owner = owner; } ////// public override string ToString() { return String.Empty; } ///Converts the System.Web.UI.WebControls.Column to string. ////// /// Return true if tracking state changes. /// bool IStateManager.IsTrackingViewState { get { return IsTrackingViewState; } } ////// /// Load previously saved state. /// void IStateManager.LoadViewState(object state) { LoadViewState(state); } ////// /// Start tracking state changes. /// void IStateManager.TrackViewState() { TrackViewState(); } ////// /// Return object containing state changes. /// object IStateManager.SaveViewState() { return SaveViewState(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web; using System.Web.UI; ////// Creates a column and is the base class for all [ TypeConverterAttribute(typeof(ExpandableObjectConverter)) ] public abstract class DataGridColumn : IStateManager { private DataGrid owner; private TableItemStyle itemStyle; private TableItemStyle headerStyle; private TableItemStyle footerStyle; private StateBag statebag; private bool marked; ///column types. /// /// protected DataGridColumn() { statebag = new StateBag(); } ///Initializes a new instance of the System.Web.UI.WebControls.Column class. ////// protected bool DesignMode { get { if (owner != null) { return owner.DesignMode; } return false; } } ///[To be supplied.] ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataGridColumn_FooterStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public virtual TableItemStyle FooterStyle { get { if (footerStyle == null) { footerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)footerStyle).TrackViewState(); } return footerStyle; } } ///Gets the style properties for the footer item. ////// internal TableItemStyle FooterStyleInternal { get { return footerStyle; } } ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataGridColumn_FooterText) ] public virtual string FooterText { get { object o = ViewState["FooterText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["FooterText"] = value; OnColumnChanged(); } } ///Gets or sets the text displayed in the footer of the /// System.Web.UI.WebControls.Column. ////// [ WebCategory("Appearance"), DefaultValue(""), UrlProperty(), WebSysDescription(SR.DataGridColumn_HeaderImageUrl) ] public virtual string HeaderImageUrl { get { object o = ViewState["HeaderImageUrl"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["HeaderImageUrl"] = value; OnColumnChanged(); } } ///Gets or sets the URL reference to an image to display /// instead of text on the header of this System.Web.UI.WebControls.Column /// . ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataGridColumn_HeaderStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public virtual TableItemStyle HeaderStyle { get { if (headerStyle == null) { headerStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)headerStyle).TrackViewState(); } return headerStyle; } } ///Gets the style properties for the header of the System.Web.UI.WebControls.Column. This property is read-only. ////// internal TableItemStyle HeaderStyleInternal { get { return headerStyle; } } ////// [ WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.DataGridColumn_HeaderText) ] public virtual string HeaderText { get { object o = ViewState["HeaderText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["HeaderText"] = value; OnColumnChanged(); } } ///Gets or sets the text displayed in the header of the /// System.Web.UI.WebControls.Column. ////// [ WebCategory("Styles"), DefaultValue(null), WebSysDescription(SR.DataGridColumn_ItemStyle), DesignerSerializationVisibility(DesignerSerializationVisibility.Content), PersistenceMode(PersistenceMode.InnerProperty) ] public virtual TableItemStyle ItemStyle { get { if (itemStyle == null) { itemStyle = new TableItemStyle(); if (IsTrackingViewState) ((IStateManager)itemStyle).TrackViewState(); } return itemStyle; } } ///Gets the style properties of an item within the System.Web.UI.WebControls.Column. This property is read-only. ////// internal TableItemStyle ItemStyleInternal { get { return itemStyle; } } ////// protected DataGrid Owner { get { return owner; } } ///Gets the System.Web.UI.WebControls.DataGrid that the System.Web.UI.WebControls.Column is a part of. This property is read-only. ////// [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.DataGridColumn_SortExpression) ] public virtual string SortExpression { get { object o = ViewState["SortExpression"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["SortExpression"] = value; OnColumnChanged(); } } ///Gets or sets the expression used when this column is used to sort the data source> by. ////// protected StateBag ViewState { get { return statebag; } } ///Gets the statebag for the System.Web.UI.WebControls.Column. This property is read-only. ////// [ WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.DataGridColumn_Visible) ] public bool Visible { get { object o = ViewState["Visible"]; if (o != null) return(bool)o; return true; } set { ViewState["Visible"] = value; OnColumnChanged(); } } ///Gets or sets a value to indicate whether the System.Web.UI.WebControls.Column is visible. ////// public virtual void Initialize() { } ////// public virtual void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { switch (itemType) { case ListItemType.Header: { WebControl headerControl = null; bool sortableHeader = true; string sortExpression = null; if ((owner != null) && (owner.AllowSorting == false)) { sortableHeader = false; } if (sortableHeader) { sortExpression = SortExpression; if (sortExpression.Length == 0) sortableHeader = false; } string headerImageUrl = HeaderImageUrl; if (headerImageUrl.Length != 0) { if (sortableHeader) { ImageButton sortButton = new ImageButton(); sortButton.ImageUrl = HeaderImageUrl; sortButton.CommandName = DataGrid.SortCommandName; sortButton.CommandArgument = sortExpression; sortButton.CausesValidation = false; headerControl = sortButton; } else { Image headerImage = new Image(); headerImage.ImageUrl = headerImageUrl; headerControl = headerImage; } } else { string headerText = HeaderText; if (sortableHeader) { LinkButton sortButton = new DataGridLinkButton(); sortButton.Text = headerText; sortButton.CommandName = DataGrid.SortCommandName; sortButton.CommandArgument = sortExpression; sortButton.CausesValidation = false; headerControl = sortButton; } else { if (headerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. headerText = " "; } cell.Text = headerText; } } if (headerControl != null) { cell.Controls.Add(headerControl); } } break; case ListItemType.Footer: { string footerText = FooterText; if (footerText.Length == 0) { // the browser does not render table borders for cells with nothing // in their content, so we add a non-breaking space. footerText = " "; } cell.Text = footerText; } break; } } ///Initializes a cell in the System.Web.UI.WebControls.Column. ////// protected bool IsTrackingViewState { get { return marked; } } ///Determines if the System.Web.UI.WebControls.Column is marked to save its state. ////// protected virtual void LoadViewState(object savedState) { if (savedState != null) { object[] myState = (object[])savedState; if (myState[0] != null) ((IStateManager)ViewState).LoadViewState(myState[0]); if (myState[1] != null) ((IStateManager)ItemStyle).LoadViewState(myState[1]); if (myState[2] != null) ((IStateManager)HeaderStyle).LoadViewState(myState[2]); if (myState[3] != null) ((IStateManager)FooterStyle).LoadViewState(myState[3]); } } ///Loads the state of the System.Web.UI.WebControls.Column. ////// protected virtual void TrackViewState() { marked = true; ((IStateManager)ViewState).TrackViewState(); if (itemStyle != null) ((IStateManager)itemStyle).TrackViewState(); if (headerStyle != null) ((IStateManager)headerStyle).TrackViewState(); if (footerStyle != null) ((IStateManager)footerStyle).TrackViewState(); } ///Marks the starting point to begin tracking and saving changes to the /// control as part of the control viewstate. ////// protected virtual void OnColumnChanged() { if (owner != null) { owner.OnColumnsChanged(); } } ///Raises the ColumnChanged event for a System.Web.UI.WebControls.Column. ////// protected virtual object SaveViewState() { object propState = ((IStateManager)ViewState).SaveViewState(); object itemStyleState = (itemStyle != null) ? ((IStateManager)itemStyle).SaveViewState() : null; object headerStyleState = (headerStyle != null) ? ((IStateManager)headerStyle).SaveViewState() : null; object footerStyleState = (footerStyle != null) ? ((IStateManager)footerStyle).SaveViewState() : null; if ((propState != null) || (itemStyleState != null) || (headerStyleState != null) || (footerStyleState != null)) { return new object[4] { propState, itemStyleState, headerStyleState, footerStyleState }; } return null; } ///Saves the current state of the System.Web.UI.WebControls.Column. ////// internal void SetOwner(DataGrid owner) { this.owner = owner; } ////// public override string ToString() { return String.Empty; } ///Converts the System.Web.UI.WebControls.Column to string. ////// /// Return true if tracking state changes. /// bool IStateManager.IsTrackingViewState { get { return IsTrackingViewState; } } ////// /// Load previously saved state. /// void IStateManager.LoadViewState(object state) { LoadViewState(state); } ////// /// Start tracking state changes. /// void IStateManager.TrackViewState() { TrackViewState(); } ////// /// Return object containing state changes. /// object IStateManager.SaveViewState() { return SaveViewState(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
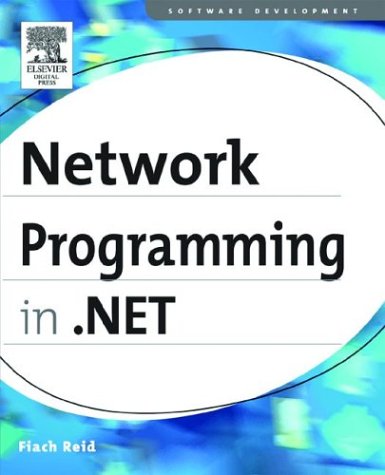
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthorizationSection.cs
- PeerDefaultCustomResolverClient.cs
- CodeSubDirectory.cs
- SessionIDManager.cs
- VoiceObjectToken.cs
- ProxyGenerationError.cs
- PointF.cs
- NotImplementedException.cs
- MemberDescriptor.cs
- WebHttpEndpoint.cs
- rsa.cs
- Exceptions.cs
- BamlResourceSerializer.cs
- MenuScrollingVisibilityConverter.cs
- PassportPrincipal.cs
- Header.cs
- LineBreakRecord.cs
- ProtocolElement.cs
- XmlAnyAttributeAttribute.cs
- _CookieModule.cs
- TextEditorTyping.cs
- DbgUtil.cs
- SerializationTrace.cs
- EntitySqlQueryState.cs
- XmlSchemaAnnotation.cs
- ClientBuildManagerCallback.cs
- DataStreams.cs
- Keywords.cs
- EntityCommand.cs
- EdmComplexTypeAttribute.cs
- ApplicationSecurityManager.cs
- TextProperties.cs
- Positioning.cs
- CompilerScope.Storage.cs
- Buffer.cs
- GACMembershipCondition.cs
- Timeline.cs
- TemplateAction.cs
- ThumbAutomationPeer.cs
- DoubleKeyFrameCollection.cs
- DataServiceQuery.cs
- ObjectToIdCache.cs
- ShaderRenderModeValidation.cs
- EpmContentSerializer.cs
- BuilderElements.cs
- Parameter.cs
- BooleanAnimationUsingKeyFrames.cs
- XmlDeclaration.cs
- ItemMap.cs
- FileNotFoundException.cs
- SortDescription.cs
- HttpFileCollection.cs
- SpellerInterop.cs
- TypeHelpers.cs
- ImageDesigner.cs
- X509CertificateChain.cs
- DockAndAnchorLayout.cs
- BitmapInitialize.cs
- XmlException.cs
- InternalSafeNativeMethods.cs
- CatalogPartChrome.cs
- OdbcInfoMessageEvent.cs
- TypeLibConverter.cs
- XmlSerializerNamespaces.cs
- SqlConnection.cs
- SignatureHelper.cs
- ParameterBuilder.cs
- Translator.cs
- Update.cs
- Asn1IntegerConverter.cs
- DataTableCollection.cs
- AttributeCollection.cs
- Random.cs
- ComplexBindingPropertiesAttribute.cs
- CssClassPropertyAttribute.cs
- DataGridBoolColumn.cs
- DrawingGroup.cs
- DataGridViewButtonColumn.cs
- Int16.cs
- Compress.cs
- log.cs
- Graph.cs
- EqualityComparer.cs
- DataGridCellsPanel.cs
- ISAPIApplicationHost.cs
- EntityClientCacheKey.cs
- ReflectionPermission.cs
- BasicHttpMessageSecurityElement.cs
- ToolStripEditorManager.cs
- LayoutTable.cs
- ButtonPopupAdapter.cs
- UpdateTranslator.cs
- NominalTypeEliminator.cs
- SystemIPGlobalStatistics.cs
- ErrorFormatterPage.cs
- HtmlInputButton.cs
- TextRangeProviderWrapper.cs
- SqlVersion.cs
- QueryableDataSourceHelper.cs
- PolicyLevel.cs