Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / ObjectToken / VoiceObjectToken.cs / 1 / VoiceObjectToken.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Encapsulation for an Object Token of type voice // // History: // 7/1/2004 jeanfp //--------------------------------------------------------------------------- using Microsoft.Win32; using System; using System.Diagnostics; using RegistryEntry = System.Collections.Generic.KeyValuePair; namespace System.Speech.Internal.ObjectTokens { /// /// Summary description for VoiceObjectToken. /// #if VSCOMPILE [DebuggerDisplay ("{Name}")] #endif internal class VoiceObjectToken : ObjectToken { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors protected VoiceObjectToken (string keyId, RegistryKey hkey) : base (keyId, hkey) { } static internal VoiceObjectToken Create (string sCategoryId, string sTokenId) { string id; RegistryKey hkey = ObjectToken.CreateKey (sCategoryId, sTokenId, false, out id); if (hkey != null) { return new VoiceObjectToken (id, hkey); } return null; } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC public override bool Equals (object obj) { VoiceObjectToken refObj = obj as VoiceObjectToken; if (refObj == null) { return false; } return Id == refObj.Id; } /// TODOC public override int GetHashCode () { return Id.GetHashCode (); } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties ////// Returns the Age from a voice token /// ///internal string Age { get { string age; if (Attributes == null || !Attributes.TryGetString ("Age", out age)) { age = string.Empty; } return age; } } /// /// Returns the gender /// ///internal string Gender { get { string gender; if (Attributes == null || !Attributes.TryGetString ("Gender", out gender)) { gender = string.Empty; } return gender; } } #if SPEECHSERVER internal VoiceCategory VoiceCategory { set { _category = value; } get { return _category; } } #endif #endregion //******************************************************************* // // Protected Methods // //******************************************************************** #region Protected Methods protected override void Dispose (bool disposing) { base.Dispose (disposing); } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields #if SPEECHSERVER private VoiceCategory _category = VoiceCategory.Default; #endif #endregion } //******************************************************************* // // Private Types // //******************************************************************** #region Private Types #if SPEECHSERVER internal enum VoiceCategory { Default, ScanSoft } #endif #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Encapsulation for an Object Token of type voice // // History: // 7/1/2004 jeanfp //--------------------------------------------------------------------------- using Microsoft.Win32; using System; using System.Diagnostics; using RegistryEntry = System.Collections.Generic.KeyValuePair; namespace System.Speech.Internal.ObjectTokens { /// /// Summary description for VoiceObjectToken. /// #if VSCOMPILE [DebuggerDisplay ("{Name}")] #endif internal class VoiceObjectToken : ObjectToken { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors protected VoiceObjectToken (string keyId, RegistryKey hkey) : base (keyId, hkey) { } static internal VoiceObjectToken Create (string sCategoryId, string sTokenId) { string id; RegistryKey hkey = ObjectToken.CreateKey (sCategoryId, sTokenId, false, out id); if (hkey != null) { return new VoiceObjectToken (id, hkey); } return null; } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC public override bool Equals (object obj) { VoiceObjectToken refObj = obj as VoiceObjectToken; if (refObj == null) { return false; } return Id == refObj.Id; } /// TODOC public override int GetHashCode () { return Id.GetHashCode (); } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties ////// Returns the Age from a voice token /// ///internal string Age { get { string age; if (Attributes == null || !Attributes.TryGetString ("Age", out age)) { age = string.Empty; } return age; } } /// /// Returns the gender /// ///internal string Gender { get { string gender; if (Attributes == null || !Attributes.TryGetString ("Gender", out gender)) { gender = string.Empty; } return gender; } } #if SPEECHSERVER internal VoiceCategory VoiceCategory { set { _category = value; } get { return _category; } } #endif #endregion //******************************************************************* // // Protected Methods // //******************************************************************** #region Protected Methods protected override void Dispose (bool disposing) { base.Dispose (disposing); } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields #if SPEECHSERVER private VoiceCategory _category = VoiceCategory.Default; #endif #endregion } //******************************************************************* // // Private Types // //******************************************************************** #region Private Types #if SPEECHSERVER internal enum VoiceCategory { Default, ScanSoft } #endif #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
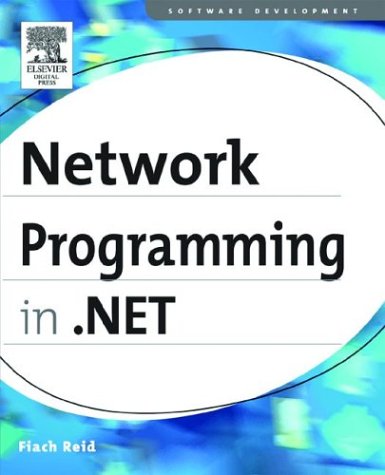
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityConnectionStringBuilder.cs
- WebPartEditorApplyVerb.cs
- TdsParser.cs
- MasterPage.cs
- CachedFontFace.cs
- ValidationSummary.cs
- ConditionalBranch.cs
- DetailsViewDeletedEventArgs.cs
- AnyAllSearchOperator.cs
- BinaryUtilClasses.cs
- FreezableCollection.cs
- EditingCommands.cs
- ScriptReferenceEventArgs.cs
- BezierSegment.cs
- SqlNodeAnnotations.cs
- TextContainerChangedEventArgs.cs
- XmlEventCache.cs
- WebPartDisplayMode.cs
- SecurityHeaderTokenResolver.cs
- Switch.cs
- MetabaseServerConfig.cs
- MessageFilterTable.cs
- StandardCommandToolStripMenuItem.cs
- SQLCharsStorage.cs
- RC2.cs
- StorageEntitySetMapping.cs
- ToolStripLocationCancelEventArgs.cs
- SpecularMaterial.cs
- ListItemsPage.cs
- XmlnsDictionary.cs
- UpdateEventArgs.cs
- XmlSchemaAnnotation.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- FullTextState.cs
- webeventbuffer.cs
- RelationshipEnd.cs
- GiveFeedbackEvent.cs
- SystemUdpStatistics.cs
- OpenTypeCommon.cs
- EmbossBitmapEffect.cs
- BitmapEncoder.cs
- TableProvider.cs
- XMLSyntaxException.cs
- ConfigurationSectionCollection.cs
- UnsafeNativeMethods.cs
- SqlDataAdapter.cs
- SessionPageStatePersister.cs
- PageRequestManager.cs
- ResizeGrip.cs
- AnimationStorage.cs
- FrameworkTextComposition.cs
- SpeechSeg.cs
- ContentValidator.cs
- ConfigurationErrorsException.cs
- JsonFormatReaderGenerator.cs
- XPathAxisIterator.cs
- PropertyChange.cs
- ISAPIApplicationHost.cs
- GenericQueueSurrogate.cs
- SQLDateTime.cs
- ColorIndependentAnimationStorage.cs
- Object.cs
- StateDesigner.Layouts.cs
- TextDecoration.cs
- FileChangesMonitor.cs
- FixedSOMPage.cs
- FontSource.cs
- AssemblyBuilder.cs
- NetTcpSecurity.cs
- ProcessInputEventArgs.cs
- MultiByteCodec.cs
- RuntimeWrappedException.cs
- RoleManagerModule.cs
- XamlPointCollectionSerializer.cs
- QueuePathEditor.cs
- HighlightComponent.cs
- SqlInfoMessageEvent.cs
- IPAddressCollection.cs
- TypeReference.cs
- XmlILIndex.cs
- SerializationException.cs
- GridViewColumnHeaderAutomationPeer.cs
- Page.cs
- TimeSpanConverter.cs
- SchemaLookupTable.cs
- VirtualPathUtility.cs
- Pkcs9Attribute.cs
- XmlMembersMapping.cs
- ApplyImportsAction.cs
- FlowLayoutPanel.cs
- ComboBox.cs
- EntityObject.cs
- ModelVisual3D.cs
- XmlUtilWriter.cs
- EntityCommandCompilationException.cs
- XmlElementCollection.cs
- BoolLiteral.cs
- _ScatterGatherBuffers.cs
- ThreadSafeList.cs
- TimeSpanMinutesConverter.cs