Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / FontCache / CachedFontFace.cs / 1 / CachedFontFace.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The CachedFontFace class // // History: // 03/04/2004 : [....] - Cache layout and interface changes for font enumeration. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Media; using MS.Win32; using MS.Utility; using MS.Internal; using MS.Internal.FontFace; namespace MS.Internal.FontCache { ////// This structure exists because we need a common wrapper for enumeration, but we can't use original cache structures: /// 1. C# doesn't allow IEnumerable/IEnumerator on pointer. /// 2. The cache structures don't inherit from base class. /// internal struct CachedFontFace { private FamilyCollection _familyCollection; ////// Critical: This is a pointer variable and hence not safe to expose. /// [SecurityCritical] private unsafe FamilyCollection.CachedFace * _face; ////// Critical: Determines value of CheckedPointer.Size, which is used for bounds checking. /// [SecurityCritical] private unsafe int _sizeInBytes; ////// Critical: This accesses a pointer and is unsafe; the sizeInBytes is critical because it /// is used for bounds checking (via CheckedPointer) /// [SecurityCritical] public unsafe CachedFontFace(FamilyCollection familyCollection, FamilyCollection.CachedFace* face, int sizeInBytes) { _familyCollection = familyCollection; _face = face; _sizeInBytes = sizeInBytes; } ////// Critical: This accesses a pointer and is unsafe /// TreatAsSafe: This information is ok to return /// public bool IsNull { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _face == null; } } } ////// Critical: This contructs a null object /// TreatAsSafe: This is ok to execute /// public static CachedFontFace Null { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return new CachedFontFace(null, null, 0); } } } ////// Critical: This accesses unsafe code and returns a pointer /// public unsafe FamilyCollection.CachedPhysicalFace* CachedPhysicalFace { [SecurityCritical] get { return (FamilyCollection.CachedPhysicalFace *)_face; } } ////// Critical: This accesses unsafe code and returns a pointer /// public unsafe FamilyCollection.CachedCompositeFace* CompositeFace { [SecurityCritical] get { return (FamilyCollection.CachedCompositeFace *)_face; } } ////// Critical: Accesses critical fields and constructs a CheckedPointer which is a critical operation. /// TreatAsSafe: The fields used to construct the CheckedPointer are marked critical and CheckedPointer /// itself is safe to expose. /// public CheckedPointer CheckedPointer { [SecurityCritical, SecurityTreatAsSafe] get { unsafe { return new CheckedPointer(_face, _sizeInBytes); } } } ////// Critical: This accesses a pointer and is unsafe /// TreatAsSafe: This information is ok to return /// public FontStyle Style { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _face->style; } } } ////// Critical: This accesses a pointer and is unsafe /// TreatAsSafe: This information is ok to return /// public FontWeight Weight { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _face->weight; } } } ////// Critical: This accesses a pointer and is unsafe /// TreatAsSafe: This information is ok to return /// public FontStretch Stretch { [SecurityCritical,SecurityTreatAsSafe] get { unsafe { return _face->stretch; } } } ////// Matching style /// public MatchingStyle MatchingStyle { get { return new MatchingStyle(Style, Weight, Stretch); } } ////// Critical - as this accesses unsafe pointers and returns GlyphTypeface created from internal constructor /// which exposes windows font information. /// Safe - as this doesn't allow you to create a GlyphTypeface object for a specific /// font and thus won't allow you to figure what fonts might be installed on /// the machine. /// [SecurityCritical, SecurityTreatAsSafe] public GlyphTypeface CreateGlyphTypeface() { unsafe { return new GlyphTypeface( _familyCollection.GetFontUri(CachedPhysicalFace), CachedPhysicalFace->styleSimulations, /* fromPublic = */ false ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
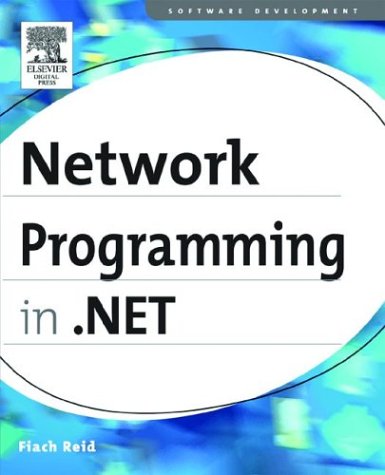
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadInterruptedException.cs
- DataContractSerializerSection.cs
- DesignerProperties.cs
- XmlSchemaGroupRef.cs
- MinimizableAttributeTypeConverter.cs
- Soap.cs
- CalculatedColumn.cs
- Transform.cs
- DummyDataSource.cs
- NumberFormatInfo.cs
- DateTimeUtil.cs
- HttpConfigurationSystem.cs
- RangeBaseAutomationPeer.cs
- DictionaryEntry.cs
- TagElement.cs
- ListParagraph.cs
- ViewCellSlot.cs
- StringConverter.cs
- XmlArrayItemAttributes.cs
- AbstractExpressions.cs
- TemplateKey.cs
- SetIterators.cs
- UnsupportedPolicyOptionsException.cs
- WebContext.cs
- Image.cs
- ColumnReorderedEventArgs.cs
- QilGenerator.cs
- PrtTicket_Public_Simple.cs
- GorillaCodec.cs
- Border.cs
- SafeSystemMetrics.cs
- ContainerUIElement3D.cs
- OrderedHashRepartitionEnumerator.cs
- Model3DGroup.cs
- EventWaitHandleSecurity.cs
- WSTransactionSection.cs
- GetWinFXPath.cs
- PromptBuilder.cs
- RelAssertionDirectKeyIdentifierClause.cs
- LongValidator.cs
- BindingNavigator.cs
- ToolStripPanelRow.cs
- BitmapEncoder.cs
- PointHitTestResult.cs
- SystemNetHelpers.cs
- Lock.cs
- CharacterMetricsDictionary.cs
- TableRowGroup.cs
- InvariantComparer.cs
- PointF.cs
- AttributeCollection.cs
- WasHttpModulesInstallComponent.cs
- RepeatButton.cs
- PointKeyFrameCollection.cs
- PenThreadPool.cs
- ActivationService.cs
- CompositeDispatchFormatter.cs
- DependencyProperty.cs
- AppDomain.cs
- DataGridViewTopRowAccessibleObject.cs
- DocumentReferenceCollection.cs
- FileLoadException.cs
- InputChannelAcceptor.cs
- DesignerEditorPartChrome.cs
- GridPattern.cs
- SystemIPAddressInformation.cs
- _Events.cs
- ResolvePPIDRequest.cs
- SourceFileBuildProvider.cs
- hebrewshape.cs
- AdRotator.cs
- XmlWriter.cs
- WorkflowMessageEventHandler.cs
- DeclarativeExpressionConditionDeclaration.cs
- SerialPinChanges.cs
- RectValueSerializer.cs
- BamlRecordWriter.cs
- ReadOnlyCollection.cs
- FunctionMappingTranslator.cs
- GenericPrincipal.cs
- InvokeProviderWrapper.cs
- XsdBuildProvider.cs
- StrongTypingException.cs
- BooleanConverter.cs
- Separator.cs
- CompilerState.cs
- DefaultProxySection.cs
- ToolStripContentPanelRenderEventArgs.cs
- EdmToObjectNamespaceMap.cs
- WsatTransactionInfo.cs
- SafeCertificateStore.cs
- ImmutablePropertyDescriptorGridEntry.cs
- odbcmetadatacollectionnames.cs
- DataGridRelationshipRow.cs
- LambdaReference.cs
- HttpCookieCollection.cs
- ConnectionManagementSection.cs
- GraphicsPathIterator.cs
- ZipIOCentralDirectoryBlock.cs
- XamlStyleSerializer.cs