Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / ComponentModel / DesignerProperties.cs / 1 / DesignerProperties.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Provides attached properties used to communicate with a designer. // See spec at: http://team/sites/Cider/Cross%20Team/Design%20Mode%20Property.doc // // History: // 7/07/2006: [....], Created // //--------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Windows; using MS.Internal.KnownBoxes; ////// The DesignerProperties class provides attached properties that can be used to /// query the state of a control when it is running in a designer. Designer tools /// will set values for properties on objects that are running in the designer. /// public static class DesignerProperties { //----------------------------------------------------- // // Public Fields // //----------------------------------------------------- ////// Identifies the DesignerProperties.IsInDesignMode dependency property. /// This field is read only. /// public static readonly DependencyProperty IsInDesignModeProperty = DependencyProperty.RegisterAttached( "IsInDesignMode", typeof(bool), typeof(DesignerProperties), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.Inherits | FrameworkPropertyMetadataOptions.OverridesInheritanceBehavior)); //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Returns the attached property IsInDesignMode value for the given dependency object. /// /// This property will return true if the given element is running in the context of a /// designer. Component developers may use this property to perform different logic /// in the context of a designer than they would when running in an application. For /// example, expensive validation or connecting to an external resource like a server /// may not make sense while an application is being developed. /// /// Designers may change the value of this property to move a control from design /// mode to run mode and back. Components that make changes to their state based /// on the value of this property should override the virtual OnPropertyChanged method /// and update their state if the IsInDesignMode property value changes. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public static bool GetIsInDesignMode(DependencyObject element) { if (element == null) throw new ArgumentNullException("element"); return (bool)element.GetValue(IsInDesignModeProperty); } ////// Sets the value of the IsInDesignMode attached property for the given dependency object. /// public static void SetIsInDesignMode(DependencyObject element, bool value) { if (element == null) throw new ArgumentNullException("element"); element.SetValue(IsInDesignModeProperty, value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Provides attached properties used to communicate with a designer. // See spec at: http://team/sites/Cider/Cross%20Team/Design%20Mode%20Property.doc // // History: // 7/07/2006: [....], Created // //--------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Windows; using MS.Internal.KnownBoxes; ////// The DesignerProperties class provides attached properties that can be used to /// query the state of a control when it is running in a designer. Designer tools /// will set values for properties on objects that are running in the designer. /// public static class DesignerProperties { //----------------------------------------------------- // // Public Fields // //----------------------------------------------------- ////// Identifies the DesignerProperties.IsInDesignMode dependency property. /// This field is read only. /// public static readonly DependencyProperty IsInDesignModeProperty = DependencyProperty.RegisterAttached( "IsInDesignMode", typeof(bool), typeof(DesignerProperties), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.Inherits | FrameworkPropertyMetadataOptions.OverridesInheritanceBehavior)); //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Returns the attached property IsInDesignMode value for the given dependency object. /// /// This property will return true if the given element is running in the context of a /// designer. Component developers may use this property to perform different logic /// in the context of a designer than they would when running in an application. For /// example, expensive validation or connecting to an external resource like a server /// may not make sense while an application is being developed. /// /// Designers may change the value of this property to move a control from design /// mode to run mode and back. Components that make changes to their state based /// on the value of this property should override the virtual OnPropertyChanged method /// and update their state if the IsInDesignMode property value changes. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public static bool GetIsInDesignMode(DependencyObject element) { if (element == null) throw new ArgumentNullException("element"); return (bool)element.GetValue(IsInDesignModeProperty); } ////// Sets the value of the IsInDesignMode attached property for the given dependency object. /// public static void SetIsInDesignMode(DependencyObject element, bool value) { if (element == null) throw new ArgumentNullException("element"); element.SetValue(IsInDesignModeProperty, value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
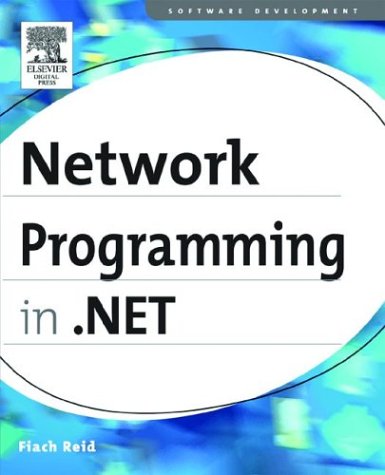
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FloaterParagraph.cs
- PartialCachingAttribute.cs
- DynamicPropertyHolder.cs
- FlowDocumentReader.cs
- TriState.cs
- ExtendedPropertyDescriptor.cs
- CompositeFontParser.cs
- TypefaceMetricsCache.cs
- DataGridColumnCollectionEditor.cs
- DataGridCommandEventArgs.cs
- RuntimeHandles.cs
- DesignerToolStripControlHost.cs
- TemplateDefinition.cs
- Matrix.cs
- QueueProcessor.cs
- RectIndependentAnimationStorage.cs
- AddInDeploymentState.cs
- StateInitializationDesigner.cs
- CoreSwitches.cs
- arabicshape.cs
- DataContractSerializerOperationGenerator.cs
- EdmComplexTypeAttribute.cs
- DescendantOverDescendantQuery.cs
- CultureInfo.cs
- _DomainName.cs
- SynchronizedChannelCollection.cs
- MemberAssignmentAnalysis.cs
- KnownIds.cs
- SessionIDManager.cs
- SimpleApplicationHost.cs
- HttpHandlerAction.cs
- TextServicesCompartment.cs
- XMLSyntaxException.cs
- EntityDataSourceChangedEventArgs.cs
- Scanner.cs
- GC.cs
- XmlSchemaGroup.cs
- IisTraceWebEventProvider.cs
- OpCellTreeNode.cs
- OrderedDictionaryStateHelper.cs
- ValueSerializer.cs
- XmlDomTextWriter.cs
- ControlPropertyNameConverter.cs
- WebException.cs
- EntityCollection.cs
- TextBoxBase.cs
- CodeExpressionCollection.cs
- ResourceExpressionBuilder.cs
- RegexParser.cs
- DynamicValueConverter.cs
- WindowsScroll.cs
- NavigationPropertyEmitter.cs
- Effect.cs
- ToolStripRenderer.cs
- PointHitTestResult.cs
- SafeBitVector32.cs
- XmlQualifiedName.cs
- NativeMethods.cs
- TypeListConverter.cs
- FixedDSBuilder.cs
- BitmapMetadata.cs
- SpecularMaterial.cs
- PageThemeParser.cs
- MouseEvent.cs
- DataGridViewTopRowAccessibleObject.cs
- WaitingCursor.cs
- KeyPressEvent.cs
- ApplicationGesture.cs
- InstanceData.cs
- WebPart.cs
- EditBehavior.cs
- ViewCellSlot.cs
- ReverseInheritProperty.cs
- CacheVirtualItemsEvent.cs
- RelatedCurrencyManager.cs
- ExternalFile.cs
- HistoryEventArgs.cs
- ServiceMetadataExtension.cs
- XmlNodeChangedEventArgs.cs
- TextStore.cs
- RuntimeConfigurationRecord.cs
- SQLBytesStorage.cs
- _AuthenticationState.cs
- EventHandlersStore.cs
- OutputCacheSection.cs
- ToolStripSplitStackLayout.cs
- XmlHierarchicalEnumerable.cs
- RegisteredArrayDeclaration.cs
- DecoderReplacementFallback.cs
- ToolboxComponentsCreatingEventArgs.cs
- RuntimeConfigurationRecord.cs
- handlecollector.cs
- BindingValueChangedEventArgs.cs
- invalidudtexception.cs
- AssemblyNameProxy.cs
- DataGridViewRowStateChangedEventArgs.cs
- HWStack.cs
- IssuedTokenClientBehaviorsElement.cs
- Assembly.cs
- KoreanLunisolarCalendar.cs