Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / System / Collections / ObjectModel / ReadOnlyObservableCollection.cs / 1 / ReadOnlyObservableCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Read-only wrapper around an ObservableCollection. // // See spec at [....]/connecteddata/Specs/Collection%20Interfaces.mht // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; namespace System.Collections.ObjectModel { ////// Read-only wrapper around an ObservableCollection. /// public class ReadOnlyObservableCollection: ReadOnlyCollection , INotifyCollectionChanged, INotifyPropertyChanged { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- /// /// Initializes a new instance of ReadOnlyObservableCollection that /// wraps the given ObservableCollection. /// public ReadOnlyObservableCollection(ObservableCollectionlist) : base(list) { ((INotifyCollectionChanged)Items).CollectionChanged += new NotifyCollectionChangedEventHandler(HandleCollectionChanged); ((INotifyPropertyChanged)Items).PropertyChanged += new PropertyChangedEventHandler(HandlePropertyChanged); } #endregion Constructors #region Interfaces //------------------------------------------------------ // // Interfaces // //----------------------------------------------------- #region INotifyCollectionChanged /// /// CollectionChanged event (per event NotifyCollectionChangedEventHandler INotifyCollectionChanged.CollectionChanged { add { CollectionChanged += value; } remove { CollectionChanged -= value; } } ///). /// /// Occurs when the collection changes, either by adding or removing an item. /// ////// see protected virtual event NotifyCollectionChangedEventHandler CollectionChanged; ////// /// raise CollectionChanged event to any listeners /// protected virtual void OnCollectionChanged(NotifyCollectionChangedEventArgs args) { if (CollectionChanged != null) { CollectionChanged(this, args); } } #endregion INotifyCollectionChanged #region INotifyPropertyChanged ////// PropertyChanged event (per event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add { PropertyChanged += value; } remove { PropertyChanged -= value; } } ///). /// /// Occurs when a property changes. /// ////// see protected virtual event PropertyChangedEventHandler PropertyChanged; ////// /// raise PropertyChanged event to any listeners /// protected virtual void OnPropertyChanged(PropertyChangedEventArgs args) { if (PropertyChanged != null) { PropertyChanged(this, args); } } #endregion INotifyPropertyChanged #endregion Interfaces #region Private Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ // forward CollectionChanged events from the base list to our listeners void HandleCollectionChanged(object sender, NotifyCollectionChangedEventArgs e) { OnCollectionChanged(e); } // forward PropertyChanged events from the base list to our listeners void HandlePropertyChanged(object sender, PropertyChangedEventArgs e) { OnPropertyChanged(e); } #endregion Private Methods #region Private Fields //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
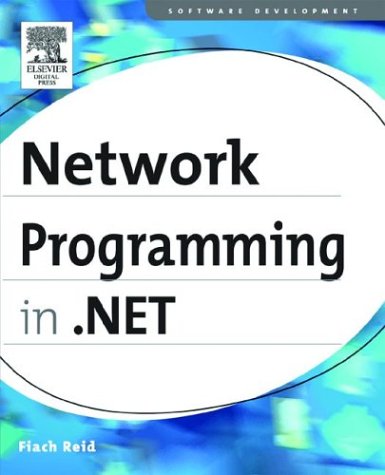
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DayRenderEvent.cs
- Label.cs
- SignedInfo.cs
- DesignerWidgets.cs
- DeflateStream.cs
- GroupBox.cs
- HighlightComponent.cs
- CngProvider.cs
- OleDbEnumerator.cs
- FixedSOMSemanticBox.cs
- UrlMapping.cs
- WebRequestModulesSection.cs
- DiscardableAttribute.cs
- Oid.cs
- HtmlProps.cs
- EventToken.cs
- ScriptResourceInfo.cs
- Point3DKeyFrameCollection.cs
- PropertyConverter.cs
- RuleConditionDialog.Designer.cs
- APCustomTypeDescriptor.cs
- SoapProtocolImporter.cs
- OracleException.cs
- AspCompat.cs
- TraceSource.cs
- BamlRecordReader.cs
- RTLAwareMessageBox.cs
- EntityDataSourceContainerNameItem.cs
- ClientUrlResolverWrapper.cs
- DataObjectCopyingEventArgs.cs
- UpdateTracker.cs
- ConversionContext.cs
- ExpressionParser.cs
- HttpServerVarsCollection.cs
- MetadataPropertyvalue.cs
- WindowsSecurityTokenAuthenticator.cs
- SvcMapFileLoader.cs
- xmlsaver.cs
- DirectoryRootQuery.cs
- StatusBarPanel.cs
- MembershipSection.cs
- ToolStripDropDownButton.cs
- SizeAnimationUsingKeyFrames.cs
- Composition.cs
- ModuleElement.cs
- MouseOverProperty.cs
- FileRegion.cs
- autovalidator.cs
- ListViewPagedDataSource.cs
- DbConvert.cs
- StructuredTypeEmitter.cs
- _ProxyChain.cs
- ColorDialog.cs
- TransformDescriptor.cs
- XPathDocumentNavigator.cs
- WebPartPersonalization.cs
- OdbcEnvironment.cs
- AsyncOperation.cs
- ColorAnimationBase.cs
- TextServicesHost.cs
- RenderContext.cs
- mda.cs
- ColorTranslator.cs
- DataGridViewCellStyleConverter.cs
- BulletedListEventArgs.cs
- SettingsSection.cs
- WindowsTokenRoleProvider.cs
- CodeAccessPermission.cs
- PassportAuthenticationEventArgs.cs
- NodeFunctions.cs
- ControlEvent.cs
- PageCodeDomTreeGenerator.cs
- TypedReference.cs
- StylusTip.cs
- SiteMapNodeCollection.cs
- ConstructorNeedsTagAttribute.cs
- TdsParserSessionPool.cs
- _ListenerAsyncResult.cs
- ObjectTag.cs
- ServiceManager.cs
- ComponentResourceKey.cs
- RegularExpressionValidator.cs
- OleDbInfoMessageEvent.cs
- ListSourceHelper.cs
- CompositeCollection.cs
- CommentEmitter.cs
- StateWorkerRequest.cs
- EntityDescriptor.cs
- WebBrowserNavigatingEventHandler.cs
- VarRemapper.cs
- ComboBoxAutomationPeer.cs
- RuntimeConfig.cs
- ActivationServices.cs
- DataGridViewCellCancelEventArgs.cs
- SvcMapFile.cs
- AutoScrollExpandMessageFilter.cs
- DataGridViewIntLinkedList.cs
- OleDbEnumerator.cs
- KeyBinding.cs
- FileDialog.cs