Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Command / KeyBinding.cs / 1 / KeyBinding.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyBinding class is used by the developer to create Keyboard Input Bindings // // See spec at : http://avalon/coreui/Specs/Commanding(new).mht // //* KeyBinding class serves the purpose of Input Bindings for Keyboard Device. // // History: // 06/01/2003 : chandras - Created // 05/01/2004 : chandra - changed to accommodate new design // ( http://avalon/coreui/Specs/Commanding(new).mht ) //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.ComponentModel; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// KeyBinding - Implements InputBinding (generic InputGesture-Command map) /// KeyBinding acts like a map for KeyGesture and Commands. /// Most of the logic is in InputBinding and KeyGesture, this only /// facilitates user to add Key/Modifiers directly without going in /// KeyGesture path. Also it provides the KeyGestureTypeConverter /// on the Gesture property to have KeyGesture, like Ctrl+X, Alt+V /// defined in Markup as Gesture="Ctrl+X" working /// public class KeyBinding : InputBinding { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructor ////// Constructor /// public KeyBinding() : base() { } ////// Constructor /// /// Command associated /// KeyGesture associated public KeyBinding(ICommand command, KeyGesture gesture) : base(command, gesture) { } ////// Constructor /// /// /// modifiers /// key public KeyBinding(ICommand command, Key key, ModifierKeys modifiers) : base(command, new KeyGesture(key, modifiers)) { } #endregion Constructor //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// KeyGesture Override, to ensure type-safety and provide a /// TypeConverter for KeyGesture /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public override InputGesture Gesture { get { return base.Gesture as KeyGesture; } set { if (value is KeyGesture) { base.Gesture = value; } else { throw new ArgumentException(SR.Get(SRID.InputBinding_ExpectedInputGesture, typeof(KeyGesture))); } } } ////// Modifier /// public ModifierKeys Modifiers { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Modifiers; } return ModifierKeys.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture(Key.None, (ModifierKeys)value, /*validateGesture = */ false); } else { Gesture = new KeyGesture(((KeyGesture)Gesture).Key, value, /*validateGesture = */ false); } } } } ////// Key /// public Key Key { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Key; } return Key.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture((Key)value, ModifierKeys.None, /*validateGesture = */ false); } else { Gesture = new KeyGesture(value, ((KeyGesture)Gesture).Modifiers, /*validateGesture = */ false); } } } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyBinding class is used by the developer to create Keyboard Input Bindings // // See spec at : http://avalon/coreui/Specs/Commanding(new).mht // //* KeyBinding class serves the purpose of Input Bindings for Keyboard Device. // // History: // 06/01/2003 : chandras - Created // 05/01/2004 : chandra - changed to accommodate new design // ( http://avalon/coreui/Specs/Commanding(new).mht ) //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.ComponentModel; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// KeyBinding - Implements InputBinding (generic InputGesture-Command map) /// KeyBinding acts like a map for KeyGesture and Commands. /// Most of the logic is in InputBinding and KeyGesture, this only /// facilitates user to add Key/Modifiers directly without going in /// KeyGesture path. Also it provides the KeyGestureTypeConverter /// on the Gesture property to have KeyGesture, like Ctrl+X, Alt+V /// defined in Markup as Gesture="Ctrl+X" working /// public class KeyBinding : InputBinding { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructor ////// Constructor /// public KeyBinding() : base() { } ////// Constructor /// /// Command associated /// KeyGesture associated public KeyBinding(ICommand command, KeyGesture gesture) : base(command, gesture) { } ////// Constructor /// /// /// modifiers /// key public KeyBinding(ICommand command, Key key, ModifierKeys modifiers) : base(command, new KeyGesture(key, modifiers)) { } #endregion Constructor //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// KeyGesture Override, to ensure type-safety and provide a /// TypeConverter for KeyGesture /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public override InputGesture Gesture { get { return base.Gesture as KeyGesture; } set { if (value is KeyGesture) { base.Gesture = value; } else { throw new ArgumentException(SR.Get(SRID.InputBinding_ExpectedInputGesture, typeof(KeyGesture))); } } } ////// Modifier /// public ModifierKeys Modifiers { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Modifiers; } return ModifierKeys.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture(Key.None, (ModifierKeys)value, /*validateGesture = */ false); } else { Gesture = new KeyGesture(((KeyGesture)Gesture).Key, value, /*validateGesture = */ false); } } } } ////// Key /// public Key Key { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Key; } return Key.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture((Key)value, ModifierKeys.None, /*validateGesture = */ false); } else { Gesture = new KeyGesture(value, ((KeyGesture)Gesture).Modifiers, /*validateGesture = */ false); } } } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
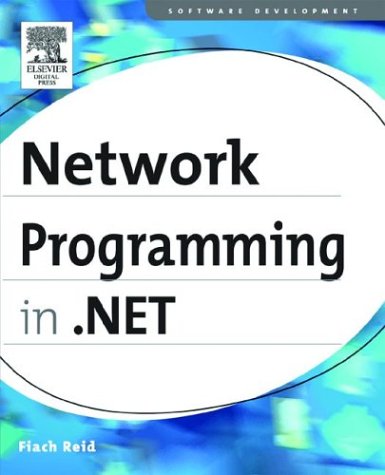
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializationEventsCache.cs
- ObjectDataSourceSelectingEventArgs.cs
- SqlLiftWhereClauses.cs
- FixedDocument.cs
- SafeReversePInvokeHandle.cs
- DropDownList.cs
- Regex.cs
- ContainerVisual.cs
- FlowDocumentPaginator.cs
- HostDesigntimeLicenseContext.cs
- RunClient.cs
- DesignerDeviceConfig.cs
- PassportAuthentication.cs
- PropertyGridView.cs
- PermissionSet.cs
- WebSysDescriptionAttribute.cs
- validationstate.cs
- shaperfactory.cs
- SolidColorBrush.cs
- WindowsListViewSubItem.cs
- CodeNamespaceImport.cs
- Compress.cs
- FirewallWrapper.cs
- DataRowComparer.cs
- Ipv6Element.cs
- HtmlEmptyTagControlBuilder.cs
- FileNameEditor.cs
- LineGeometry.cs
- DataIdProcessor.cs
- PlatformCulture.cs
- ArraySet.cs
- SqlBuilder.cs
- AppDomainProtocolHandler.cs
- FragmentQuery.cs
- ExtensibleClassFactory.cs
- WindowsUpDown.cs
- EventProviderClassic.cs
- WebEventCodes.cs
- CommonDialog.cs
- X509CertificateStore.cs
- TextBounds.cs
- ValueTable.cs
- TextParaLineResult.cs
- InputLanguageManager.cs
- _ListenerResponseStream.cs
- ContextMenu.cs
- CmsUtils.cs
- XmlSchemaElement.cs
- UICuesEvent.cs
- TextSimpleMarkerProperties.cs
- DbConnectionPoolGroupProviderInfo.cs
- CoreSwitches.cs
- GraphicsContainer.cs
- DataGridViewCellValidatingEventArgs.cs
- MonthCalendar.cs
- DesignerImageAdapter.cs
- OleDbFactory.cs
- FileNotFoundException.cs
- FunctionImportElement.cs
- mda.cs
- Quaternion.cs
- CheckBox.cs
- NativeMethods.cs
- TraversalRequest.cs
- DispatcherOperation.cs
- OneToOneMappingSerializer.cs
- ProcessModuleCollection.cs
- InvalidCastException.cs
- SequenceFullException.cs
- BuildTopDownAttribute.cs
- XmlMapping.cs
- SafeEventLogWriteHandle.cs
- AstNode.cs
- BaseConfigurationRecord.cs
- ServiceThrottlingBehavior.cs
- PreviewPageInfo.cs
- XhtmlBasicTextViewAdapter.cs
- TargetParameterCountException.cs
- ClientSettings.cs
- ThreadStartException.cs
- MetafileHeader.cs
- PropertyMap.cs
- DashStyles.cs
- Type.cs
- LoginCancelEventArgs.cs
- EntityContainer.cs
- NameValuePermission.cs
- XmlStringTable.cs
- TemplateInstanceAttribute.cs
- EntityDataSourceDataSelection.cs
- _SpnDictionary.cs
- ContainerUIElement3D.cs
- CodeCompileUnit.cs
- Win32.cs
- InvokeHandlers.cs
- TextModifier.cs
- DomNameTable.cs
- ServiceProviders.cs
- OperationCanceledException.cs
- MaskDescriptor.cs