Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / Microsoft / Win32 / CommonDialog.cs / 1 / CommonDialog.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // CommonDialog is a base class representing common dialogs. // At this time, we intend it only to be used as a parent class // for the FileDialog class, although it could be used to implement // other commdlg.dll dialogs in the future. It is not a // general-purpose dialog class - it's specific to Win32 common // dialogs. // // // History: // t-benja 7/7/2005 Created // //--------------------------------------------------------------------------- namespace Microsoft.Win32 { using System; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Security; using System.Security.Permissions; using System.Threading; using System.Windows; using System.Windows.Interop; using MS.Internal.PresentationFramework; using MS.Win32; ////// An abstract base class for displaying common dialogs. /// ////// InheritanceDemand for UIPermission (UIPermissionWindow.AllWindows) /// ////// We Don't want arbitrary Partially trusted code deriving from CommonDialog. /// InheritanceDemand for UIPermission (UIPermissionWindow.AllWindows) /// [UIPermission(SecurityAction.InheritanceDemand, Window = UIPermissionWindow.AllWindows)] public abstract class CommonDialog { //--------------------------------------------------- // // Constructors // //--------------------------------------------------- //#region Constructors //#endregion Constructors //---------------------------------------------------- // // Public Methods // //--------------------------------------------------- #region Public Methods ////// When overridden in a derived class, resets the properties /// of a common dialog to their default values. /// ////// Critical: Changes Dialog options /// PublicOk: This method is abstract, and there is an InheritanceDemand for /// UIPermission (UIPermissionWindow.AllWindows) to derive from CommonDialog. /// [SecurityCritical] public abstract void Reset(); ////// This is the public method that will be called to actually show /// a common dialog. Since CommonDialog is abstract, this function /// performs initialization tasks for all common dialogs and then /// calls RunDialog. /// ////// Callers must have UIPermission(UIPermissionWindow.AllWindows) to call this API. /// ////// Critical: Calls RunDialog, and accesses parking window hwnd from Application. /// PublicOk: Demands Permission appropriate to the dialog (defaults to UIPermissionWindow.AllWindows) /// [SecurityCritical] public virtual NullableShowDialog() { CheckPermissionsToShowDialog(); // Don't allow file dialogs to be shown if not in interactive mode // (for example, if we're running as a service) if (!Environment.UserInteractive) { throw new InvalidOperationException(SR.Get(SRID.CantShowModalOnNonInteractive)); } // Call GetActiveWindow to retrieve the window handle to the active window // attached to the calling thread's message queue. We'll set the owner of // the common dialog to this handle. IntPtr hwndOwner = UnsafeNativeMethods.GetActiveWindow(); if (hwndOwner == IntPtr.Zero) { // No active window, so we'll use the parking window as the owner, // if its available. if (Application.Current != null) { hwndOwner = Application.Current.ParkingHwnd; } } HwndWrapper tempParentHwnd = null; try { // No active window and application wasn't available or didn't have // a ParkingHwnd, we create a hidden parent window for the dialog to // prevent breaking UIAutomation. if (hwndOwner == IntPtr.Zero) { tempParentHwnd = new HwndWrapper(0, 0, 0, 0, 0, 0, 0, "", IntPtr.Zero, null); hwndOwner = tempParentHwnd.Handle; } // Store the handle of the owner window inside our class so we can use it // to center the dialog later. _hwndOwnerWindow = hwndOwner; // Call RunDialog(hwndOwner) return RunDialog(hwndOwner); } finally { if (tempParentHwnd != null) tempParentHwnd.Dispose(); } } /// /// Runs a common dialog box, with the owner as the given Window /// ////// Callers must have UIPermission(UIPermissionWindow.AllWindows) to call this API. /// ////// Critical: Calls RunDialog and accesses owner handle and parking window hwnd from Application. /// PublicOk: Demands UIPermission (UIPermissionWindow.AllWindows) /// [SecurityCritical] public NullableShowDialog(Window owner) { CheckPermissionsToShowDialog(); // If a valid window wasn't passed into this function, we'll // call ShowDialog() to use the active window instead of // throwing an exception if (owner == null) { return ShowDialog(); } // Don't allow file dialogs to be shown if not in interactive mode // (for example, if we're running as a service) if (!Environment.UserInteractive) { throw new InvalidOperationException(SR.Get(SRID.CantShowModalOnNonInteractive)); } // Get the handle of the owner window using WindowInteropHelper. IntPtr hwndOwner = (new WindowInteropHelper(owner)).CriticalHandle; // Just in case, check if the window's handle is zero. if (hwndOwner == IntPtr.Zero) { // CODE throw new InvalidOperationException(); } // Store the handle of the owner window inside our class so we can use it // to center the dialog later. _hwndOwnerWindow = hwndOwner; return RunDialog(hwndOwner); } #endregion Public Methods //---------------------------------------------------- // // Public Properties // //---------------------------------------------------- #region Public Properties /// /// Provides the ability to attach an arbitrary object to the dialog. /// public object Tag { get { return _userData; } set { _userData = value; } } #endregion Public Properties //--------------------------------------------------- // // Public Events // //---------------------------------------------------- //#region Public Events //#endregion Public Events //--------------------------------------------------- // // Protected Methods // //--------------------------------------------------- #region Protected Methods ////// Defines the common dialog box hook procedure that is overridden to /// add specific functionality to a common dialog box. /// ////// Critical: Calls UnsafeNativeMethods.SetFocus() and UnsafeNativeMethods.PostMessage() /// [SecurityCritical] protected virtual IntPtr HookProc(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam) { // WM_INITDIALOG // The WM_INITDIALOG message is sent to the dialog box procedure immediately // before a dialog box is displayed. Dialog box procedures typically use // this message to initialize controls and carry out any other initialization // tasks that affect the appearance of the dialog box. // // We handle WM_INITDIALOG to move the dialog to the center of the screen // and to store the default control for later use. if (msg == NativeMethods.WM_INITDIALOG) { // call MoveToScreenCenter to reposition the dialog based on the location // of the owner window. MoveToScreenCenter(new HandleRef(this,hwnd), new HandleRef(this,OwnerWindowHandle)); // when the message is WM_INITDIALOG, wparam contains a handle to the // control that should receive the default keyboard focus. We store it // for use if we get a WM_SETFOCUS later. this._hwndDefaultControl = wParam; // Under some circumstances, the dialog does not initially focus on // any control. We fix that by explicitly setting focus ourselves. See // Apps Srv/URT 39435. http://bugcheck/default.asp?URL=/Bugs/URT/39435.asp UnsafeNativeMethods.SetFocus(new HandleRef(this, wParam)); } // WM_SETFOCUS // The WM_SETFOCUS message is sent to a window after it has // gained the keyboard focus. // If we got this message, it means focus has been set to the dialog // itself, so we want to refocus on the default control; we accomplish // this by posting a message back to ourselves (CDM_SETDEFAULTFOCUS) that // we'll handle later to actually reset the focus. else if (msg == NativeMethods.WM_SETFOCUS) { UnsafeNativeMethods.PostMessage(new HandleRef(this, hwnd), CDM_SETDEFAULTFOCUS, IntPtr.Zero, IntPtr.Zero); } // CDM_SETDEFAULTFOCUS // The CDM_SETDEFAULTFOCUS message (which we define ourselves as a constant // in this class) is posted back to ourselves above in WM_SETFOCUS. When // we receive it, we set the focus to the default item in the dialog. else if (msg == CDM_SETDEFAULTFOCUS) { // If the dialog box gets focus, bounce it to the default control. // so we post a message back to ourselves to wait for the focus change then push it to the default // control. See Windows Forms ASURT 84016. http://bugcheck/default.asp?URL=/Bugs/URT/84016.asp UnsafeNativeMethods.SetFocus(new HandleRef(this, _hwndDefaultControl)); } return IntPtr.Zero; } ////// When overridden in a derived class, displays a particular type of common dialog box. /// protected abstract bool RunDialog(IntPtr hwndOwner); ////// Demands permissions appropriate to the dialog to be shown. /// protected virtual void CheckPermissionsToShowDialog() { SecurityHelper.DemandUIWindowPermission(); } #endregion Protected Methods //--------------------------------------------------- // // Internal Methods // //---------------------------------------------------- #region Internal Methods ////// Centers the given window on the screen. This method is used by HookProc /// to center the dialog on the screen before it is shown. We can't mark it /// private because we need to call it from our derived classes like /// FileDialog. /// ////// Critical: Calls UnsafeNativeMethods.SetWindowPos() /// [SecurityCritical] internal static void MoveToScreenCenter(HandleRef hWnd, HandleRef ownerHwnd) { // Create an IntPtr to store a handle to the monitor. IntPtr hMonitor = IntPtr.Zero; // Get the monitor to use based on the location of the parent window if (ownerHwnd.Handle != IntPtr.Zero) { // we have a owner hwnd; center on the screen on // which our owner hwnd is. // We use MONITOR_DEFAULTTONEAREST to get the monitor // nearest to the window if the window doesn't intersect // any display monitor. hMonitor = SafeNativeMethods.MonitorFromWindow( ownerHwnd, // window to find monitor location for NativeMethods.MONITOR_DEFAULTTONEAREST); // get the monitor nearest to the window // Only move the window if we got a valid monitor... otherwise let Windows // position the dialog. if (hMonitor != IntPtr.Zero) { // Now, create another RECT and fill it with the bounds of the parent window. NativeMethods.RECT dialogRect = new NativeMethods.RECT(); SafeNativeMethods.GetWindowRect(hWnd, ref dialogRect); Size dialogSize = new Size((dialogRect.right - dialogRect.left), /*width*/ (dialogRect.bottom - dialogRect.top)); /*height*/ // create variables that will receive the new position of the dialog double x = 0; double y = 0; // Call into a static function in System.Windows.Window to calculate // the actual new position Window.CalculateCenterScreenPosition( new HandleRef(hWnd.Wrapper, hMonitor), dialogSize, ref x, ref y); // Call SetWindowPos to actually move the window. UnsafeNativeMethods.SetWindowPos(hWnd, // handle to the window to move NativeMethods.NullHandleRef, // window to precede this one in zorder (int)Math.Round(x), (int)Math.Round(y), // new X and Y positions 0, 0, // new width and height, if applicable // Flags: // SWP_NOSIZE: Retains current size // SWP_NOZORDER: retains current zorder // SWP_NOACTIVATE: does not activate the window NativeMethods.SWP_NOSIZE | NativeMethods.SWP_NOZORDER | NativeMethods.SWP_NOACTIVATE); } } } #endregion Internal Methods //--------------------------------------------------- // // Internal Properties // //---------------------------------------------------- #region Internal Properties ////// The handle to the parent window we store internally /// needs to be accessible to derived classes so they can /// pass it back to our static MoveWindowToScreenCenter /// function, so we expose it as a read-only internal /// property. /// ////// Critical: Returns hWnd of the Application window /// internal IntPtr OwnerWindowHandle { [SecurityCritical] get { return _hwndOwnerWindow; } } #endregion Internal Properties //---------------------------------------------------- // // Internal Events // //--------------------------------------------------- //#region Internal Events //#endregion Internal Events //---------------------------------------------------- // // Private Methods // //--------------------------------------------------- //#region Private Methods //#endregion Private Methods //--------------------------------------------------- // // Protected Properties // //--------------------------------------------------- //#region Protected Properties //#endregion Protected Properties //---------------------------------------------------- // // Private Fields // //--------------------------------------------------- #region Private Fields // This is a custom message used in the CommonDialog hook procedure private const int CDM_SETDEFAULTFOCUS = NativeMethods.WM_USER + 0x51; // When the dialog is first displayed, we get a WM_INITDIALOG message, // and the handle to the default control is passed into HookProc. // We save that handle so we can set focus to the same default control // later if necessary. ////// Critical: hWnds are critical data. /// [SecurityCritical] private IntPtr _hwndDefaultControl; // Private variable used to store data for the Tag property private object _userData; ////// The owner hwnd passed into the dialog is stored as a private /// member so that the dialog can be properly centered onscreen. /// It is exposed through the OwnerWindowHandle property. /// ////// Critical: hWnds are critical data. /// [SecurityCritical] private IntPtr _hwndOwnerWindow; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // CommonDialog is a base class representing common dialogs. // At this time, we intend it only to be used as a parent class // for the FileDialog class, although it could be used to implement // other commdlg.dll dialogs in the future. It is not a // general-purpose dialog class - it's specific to Win32 common // dialogs. // // // History: // t-benja 7/7/2005 Created // //--------------------------------------------------------------------------- namespace Microsoft.Win32 { using System; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Security; using System.Security.Permissions; using System.Threading; using System.Windows; using System.Windows.Interop; using MS.Internal.PresentationFramework; using MS.Win32; ////// An abstract base class for displaying common dialogs. /// ////// InheritanceDemand for UIPermission (UIPermissionWindow.AllWindows) /// ////// We Don't want arbitrary Partially trusted code deriving from CommonDialog. /// InheritanceDemand for UIPermission (UIPermissionWindow.AllWindows) /// [UIPermission(SecurityAction.InheritanceDemand, Window = UIPermissionWindow.AllWindows)] public abstract class CommonDialog { //--------------------------------------------------- // // Constructors // //--------------------------------------------------- //#region Constructors //#endregion Constructors //---------------------------------------------------- // // Public Methods // //--------------------------------------------------- #region Public Methods ////// When overridden in a derived class, resets the properties /// of a common dialog to their default values. /// ////// Critical: Changes Dialog options /// PublicOk: This method is abstract, and there is an InheritanceDemand for /// UIPermission (UIPermissionWindow.AllWindows) to derive from CommonDialog. /// [SecurityCritical] public abstract void Reset(); ////// This is the public method that will be called to actually show /// a common dialog. Since CommonDialog is abstract, this function /// performs initialization tasks for all common dialogs and then /// calls RunDialog. /// ////// Callers must have UIPermission(UIPermissionWindow.AllWindows) to call this API. /// ////// Critical: Calls RunDialog, and accesses parking window hwnd from Application. /// PublicOk: Demands Permission appropriate to the dialog (defaults to UIPermissionWindow.AllWindows) /// [SecurityCritical] public virtual NullableShowDialog() { CheckPermissionsToShowDialog(); // Don't allow file dialogs to be shown if not in interactive mode // (for example, if we're running as a service) if (!Environment.UserInteractive) { throw new InvalidOperationException(SR.Get(SRID.CantShowModalOnNonInteractive)); } // Call GetActiveWindow to retrieve the window handle to the active window // attached to the calling thread's message queue. We'll set the owner of // the common dialog to this handle. IntPtr hwndOwner = UnsafeNativeMethods.GetActiveWindow(); if (hwndOwner == IntPtr.Zero) { // No active window, so we'll use the parking window as the owner, // if its available. if (Application.Current != null) { hwndOwner = Application.Current.ParkingHwnd; } } HwndWrapper tempParentHwnd = null; try { // No active window and application wasn't available or didn't have // a ParkingHwnd, we create a hidden parent window for the dialog to // prevent breaking UIAutomation. if (hwndOwner == IntPtr.Zero) { tempParentHwnd = new HwndWrapper(0, 0, 0, 0, 0, 0, 0, "", IntPtr.Zero, null); hwndOwner = tempParentHwnd.Handle; } // Store the handle of the owner window inside our class so we can use it // to center the dialog later. _hwndOwnerWindow = hwndOwner; // Call RunDialog(hwndOwner) return RunDialog(hwndOwner); } finally { if (tempParentHwnd != null) tempParentHwnd.Dispose(); } } /// /// Runs a common dialog box, with the owner as the given Window /// ////// Callers must have UIPermission(UIPermissionWindow.AllWindows) to call this API. /// ////// Critical: Calls RunDialog and accesses owner handle and parking window hwnd from Application. /// PublicOk: Demands UIPermission (UIPermissionWindow.AllWindows) /// [SecurityCritical] public NullableShowDialog(Window owner) { CheckPermissionsToShowDialog(); // If a valid window wasn't passed into this function, we'll // call ShowDialog() to use the active window instead of // throwing an exception if (owner == null) { return ShowDialog(); } // Don't allow file dialogs to be shown if not in interactive mode // (for example, if we're running as a service) if (!Environment.UserInteractive) { throw new InvalidOperationException(SR.Get(SRID.CantShowModalOnNonInteractive)); } // Get the handle of the owner window using WindowInteropHelper. IntPtr hwndOwner = (new WindowInteropHelper(owner)).CriticalHandle; // Just in case, check if the window's handle is zero. if (hwndOwner == IntPtr.Zero) { // CODE throw new InvalidOperationException(); } // Store the handle of the owner window inside our class so we can use it // to center the dialog later. _hwndOwnerWindow = hwndOwner; return RunDialog(hwndOwner); } #endregion Public Methods //---------------------------------------------------- // // Public Properties // //---------------------------------------------------- #region Public Properties /// /// Provides the ability to attach an arbitrary object to the dialog. /// public object Tag { get { return _userData; } set { _userData = value; } } #endregion Public Properties //--------------------------------------------------- // // Public Events // //---------------------------------------------------- //#region Public Events //#endregion Public Events //--------------------------------------------------- // // Protected Methods // //--------------------------------------------------- #region Protected Methods ////// Defines the common dialog box hook procedure that is overridden to /// add specific functionality to a common dialog box. /// ////// Critical: Calls UnsafeNativeMethods.SetFocus() and UnsafeNativeMethods.PostMessage() /// [SecurityCritical] protected virtual IntPtr HookProc(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam) { // WM_INITDIALOG // The WM_INITDIALOG message is sent to the dialog box procedure immediately // before a dialog box is displayed. Dialog box procedures typically use // this message to initialize controls and carry out any other initialization // tasks that affect the appearance of the dialog box. // // We handle WM_INITDIALOG to move the dialog to the center of the screen // and to store the default control for later use. if (msg == NativeMethods.WM_INITDIALOG) { // call MoveToScreenCenter to reposition the dialog based on the location // of the owner window. MoveToScreenCenter(new HandleRef(this,hwnd), new HandleRef(this,OwnerWindowHandle)); // when the message is WM_INITDIALOG, wparam contains a handle to the // control that should receive the default keyboard focus. We store it // for use if we get a WM_SETFOCUS later. this._hwndDefaultControl = wParam; // Under some circumstances, the dialog does not initially focus on // any control. We fix that by explicitly setting focus ourselves. See // Apps Srv/URT 39435. http://bugcheck/default.asp?URL=/Bugs/URT/39435.asp UnsafeNativeMethods.SetFocus(new HandleRef(this, wParam)); } // WM_SETFOCUS // The WM_SETFOCUS message is sent to a window after it has // gained the keyboard focus. // If we got this message, it means focus has been set to the dialog // itself, so we want to refocus on the default control; we accomplish // this by posting a message back to ourselves (CDM_SETDEFAULTFOCUS) that // we'll handle later to actually reset the focus. else if (msg == NativeMethods.WM_SETFOCUS) { UnsafeNativeMethods.PostMessage(new HandleRef(this, hwnd), CDM_SETDEFAULTFOCUS, IntPtr.Zero, IntPtr.Zero); } // CDM_SETDEFAULTFOCUS // The CDM_SETDEFAULTFOCUS message (which we define ourselves as a constant // in this class) is posted back to ourselves above in WM_SETFOCUS. When // we receive it, we set the focus to the default item in the dialog. else if (msg == CDM_SETDEFAULTFOCUS) { // If the dialog box gets focus, bounce it to the default control. // so we post a message back to ourselves to wait for the focus change then push it to the default // control. See Windows Forms ASURT 84016. http://bugcheck/default.asp?URL=/Bugs/URT/84016.asp UnsafeNativeMethods.SetFocus(new HandleRef(this, _hwndDefaultControl)); } return IntPtr.Zero; } ////// When overridden in a derived class, displays a particular type of common dialog box. /// protected abstract bool RunDialog(IntPtr hwndOwner); ////// Demands permissions appropriate to the dialog to be shown. /// protected virtual void CheckPermissionsToShowDialog() { SecurityHelper.DemandUIWindowPermission(); } #endregion Protected Methods //--------------------------------------------------- // // Internal Methods // //---------------------------------------------------- #region Internal Methods ////// Centers the given window on the screen. This method is used by HookProc /// to center the dialog on the screen before it is shown. We can't mark it /// private because we need to call it from our derived classes like /// FileDialog. /// ////// Critical: Calls UnsafeNativeMethods.SetWindowPos() /// [SecurityCritical] internal static void MoveToScreenCenter(HandleRef hWnd, HandleRef ownerHwnd) { // Create an IntPtr to store a handle to the monitor. IntPtr hMonitor = IntPtr.Zero; // Get the monitor to use based on the location of the parent window if (ownerHwnd.Handle != IntPtr.Zero) { // we have a owner hwnd; center on the screen on // which our owner hwnd is. // We use MONITOR_DEFAULTTONEAREST to get the monitor // nearest to the window if the window doesn't intersect // any display monitor. hMonitor = SafeNativeMethods.MonitorFromWindow( ownerHwnd, // window to find monitor location for NativeMethods.MONITOR_DEFAULTTONEAREST); // get the monitor nearest to the window // Only move the window if we got a valid monitor... otherwise let Windows // position the dialog. if (hMonitor != IntPtr.Zero) { // Now, create another RECT and fill it with the bounds of the parent window. NativeMethods.RECT dialogRect = new NativeMethods.RECT(); SafeNativeMethods.GetWindowRect(hWnd, ref dialogRect); Size dialogSize = new Size((dialogRect.right - dialogRect.left), /*width*/ (dialogRect.bottom - dialogRect.top)); /*height*/ // create variables that will receive the new position of the dialog double x = 0; double y = 0; // Call into a static function in System.Windows.Window to calculate // the actual new position Window.CalculateCenterScreenPosition( new HandleRef(hWnd.Wrapper, hMonitor), dialogSize, ref x, ref y); // Call SetWindowPos to actually move the window. UnsafeNativeMethods.SetWindowPos(hWnd, // handle to the window to move NativeMethods.NullHandleRef, // window to precede this one in zorder (int)Math.Round(x), (int)Math.Round(y), // new X and Y positions 0, 0, // new width and height, if applicable // Flags: // SWP_NOSIZE: Retains current size // SWP_NOZORDER: retains current zorder // SWP_NOACTIVATE: does not activate the window NativeMethods.SWP_NOSIZE | NativeMethods.SWP_NOZORDER | NativeMethods.SWP_NOACTIVATE); } } } #endregion Internal Methods //--------------------------------------------------- // // Internal Properties // //---------------------------------------------------- #region Internal Properties ////// The handle to the parent window we store internally /// needs to be accessible to derived classes so they can /// pass it back to our static MoveWindowToScreenCenter /// function, so we expose it as a read-only internal /// property. /// ////// Critical: Returns hWnd of the Application window /// internal IntPtr OwnerWindowHandle { [SecurityCritical] get { return _hwndOwnerWindow; } } #endregion Internal Properties //---------------------------------------------------- // // Internal Events // //--------------------------------------------------- //#region Internal Events //#endregion Internal Events //---------------------------------------------------- // // Private Methods // //--------------------------------------------------- //#region Private Methods //#endregion Private Methods //--------------------------------------------------- // // Protected Properties // //--------------------------------------------------- //#region Protected Properties //#endregion Protected Properties //---------------------------------------------------- // // Private Fields // //--------------------------------------------------- #region Private Fields // This is a custom message used in the CommonDialog hook procedure private const int CDM_SETDEFAULTFOCUS = NativeMethods.WM_USER + 0x51; // When the dialog is first displayed, we get a WM_INITDIALOG message, // and the handle to the default control is passed into HookProc. // We save that handle so we can set focus to the same default control // later if necessary. ////// Critical: hWnds are critical data. /// [SecurityCritical] private IntPtr _hwndDefaultControl; // Private variable used to store data for the Tag property private object _userData; ////// The owner hwnd passed into the dialog is stored as a private /// member so that the dialog can be properly centered onscreen. /// It is exposed through the OwnerWindowHandle property. /// ////// Critical: hWnds are critical data. /// [SecurityCritical] private IntPtr _hwndOwnerWindow; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
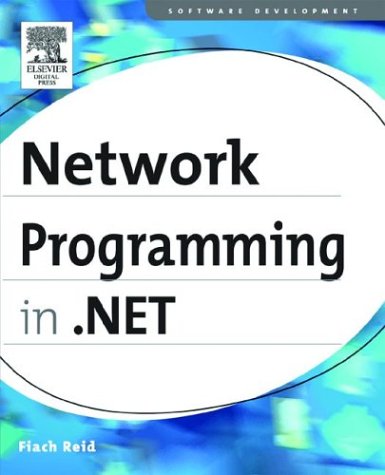
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SvcMapFileSerializer.cs
- DocumentReference.cs
- CryptoProvider.cs
- COM2PropertyDescriptor.cs
- DelegatingHeader.cs
- Button.cs
- SqlErrorCollection.cs
- Html32TextWriter.cs
- SiteIdentityPermission.cs
- ShaderEffect.cs
- columnmapkeybuilder.cs
- RepeaterCommandEventArgs.cs
- RenderDataDrawingContext.cs
- ObjectDataSource.cs
- ToggleProviderWrapper.cs
- XmlToDatasetMap.cs
- ResolveNameEventArgs.cs
- WorkBatch.cs
- XmlSchema.cs
- AutoGeneratedField.cs
- TagPrefixInfo.cs
- Message.cs
- RecordsAffectedEventArgs.cs
- Panel.cs
- DataBindingHandlerAttribute.cs
- TreeViewEvent.cs
- Rect.cs
- PagesSection.cs
- TransportContext.cs
- Int32Converter.cs
- ProxyManager.cs
- ThemeableAttribute.cs
- SrgsDocument.cs
- ListViewGroupConverter.cs
- XmlDataImplementation.cs
- UnknownBitmapEncoder.cs
- CompatibleComparer.cs
- XComponentModel.cs
- TextDecorationUnitValidation.cs
- DataBoundControlHelper.cs
- Compiler.cs
- PopOutPanel.cs
- WebBrowser.cs
- ContentElementCollection.cs
- ObjectManager.cs
- StringFreezingAttribute.cs
- DataGridViewRowCancelEventArgs.cs
- TextBlockAutomationPeer.cs
- ProxyFragment.cs
- StorageEntityContainerMapping.cs
- HttpResponseInternalWrapper.cs
- SpeechRecognizer.cs
- XamlDesignerSerializationManager.cs
- ObjRef.cs
- X509RawDataKeyIdentifierClause.cs
- RowUpdatedEventArgs.cs
- ListViewDesigner.cs
- SerialErrors.cs
- HttpCapabilitiesEvaluator.cs
- _SslStream.cs
- DbBuffer.cs
- HtmlLink.cs
- HuffmanTree.cs
- FontUnit.cs
- HtmlTable.cs
- Utils.cs
- StylusPointPropertyUnit.cs
- ChtmlPageAdapter.cs
- StatusStrip.cs
- SimpleMailWebEventProvider.cs
- CqlGenerator.cs
- DbDataReader.cs
- XsdValidatingReader.cs
- CodeArgumentReferenceExpression.cs
- SaveRecipientRequest.cs
- HtmlTableCell.cs
- Control.cs
- ClaimTypes.cs
- HttpResponse.cs
- EncodingInfo.cs
- DeleteCardRequest.cs
- Oid.cs
- SHA384.cs
- OrthographicCamera.cs
- ApplicationException.cs
- SelectionRangeConverter.cs
- PrivilegeNotHeldException.cs
- DocumentPage.cs
- Clipboard.cs
- CheckBoxAutomationPeer.cs
- PeerNearMe.cs
- EventLogPermissionEntry.cs
- SizeLimitedCache.cs
- WindowsFormsDesignerOptionService.cs
- InternalsVisibleToAttribute.cs
- sqlpipe.cs
- EntityType.cs
- CompilationRelaxations.cs
- DetailsViewModeEventArgs.cs
- PointCollection.cs