Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / DeleteCardRequest.cs / 1 / DeleteCardRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // Processes a delete card request. // // // Specify valid parent requests. // class DeleteCardRequest : UIAgentRequest { Uri m_cardId; // Specifies the card identifier. public DeleteCardRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } protected override void OnInitializeAsSystem() { base.OnInitializeAsSystem(); } // // Summary // Marshals input arguments for the request. The arguments are read from a stream in binary. // protected override void OnMarshalInArgs() { BinaryReader reader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); m_cardId = Utility.DeserializeUri( reader ); } // // Summary // Processes the request. // // The InfoCard, the claim collection associated with the card and the ledger entries // associated with the card are deleted from the store. // protected override void OnProcess() { StoreConnection connection = StoreConnection.GetConnection(); try { connection.BeginTransaction(); try { string name = null; // // Delete the infocard - we need the full row to get the name. // DataRow row = connection.GetSingleRow( QueryDetails.FullRow, new QueryParameter( SecondaryIndexDefinition.GlobalIdIndex, GlobalId.DeriveFrom( m_cardId.ToString() ) ) ); if( null != row ) { byte[ ] rawForm = row.GetDataField(); try { using ( MemoryStream stream = new MemoryStream( rawForm ) ) { InfoCard ic = new InfoCard( stream ); name = ic.Name; } } finally { connection.Delete( row ); Array.Clear( rawForm, 0, rawForm.Length ); } } IDT.TraceDebug( "Deleted infocard with id: #{0}", m_cardId ); // // Delete any other rows whose parent id is m_cardId // ListparamList = new List (); QueryParameter query = new QueryParameter( SecondaryIndexDefinition.ParentIdIndex, GlobalId.DeriveFrom( m_cardId.ToString() ) ); paramList.Add( query ); ICollection list = ( ICollection )connection.Query( QueryDetails.Identifiers, paramList.ToArray() ); if( null != list && list.Count > 0 ) { foreach( DataRow rowWithParentIdMatch in list ) { connection.Delete( rowWithParentIdMatch ); } } connection.CommitTransaction(); AuditLog.AuditCardDeletion(); } catch { connection.RollbackTransaction(); throw; } } finally { connection.Close(); } IDT.TraceDebug( "Deleted ledger entries with parent id: #{0}", m_cardId ); } // // Summary // Marshals output arguments for the request. The arguments are written to a stream in binary. // protected override void OnMarshalOutArgs() { // // This request has no output arguments. // } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
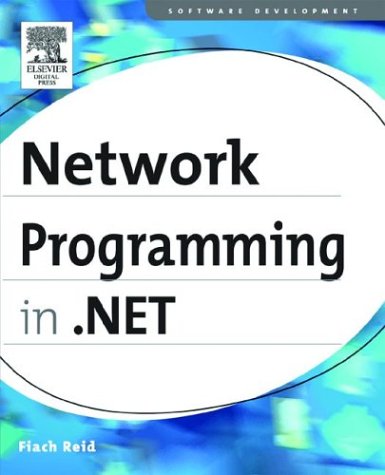
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReadOnlyCollection.cs
- SecurityElement.cs
- Suspend.cs
- AttributeParameterInfo.cs
- SecurityRuntime.cs
- WaitHandle.cs
- Viewport3DAutomationPeer.cs
- WebException.cs
- ErrorHandlingAcceptor.cs
- HttpHeaderCollection.cs
- CheckBoxFlatAdapter.cs
- OciHandle.cs
- XmlStreamStore.cs
- FlowDocumentReader.cs
- ControlDesigner.cs
- EncodingFallbackAwareXmlTextWriter.cs
- Span.cs
- TranslateTransform3D.cs
- PolyQuadraticBezierSegment.cs
- XslAstAnalyzer.cs
- ModuleConfigurationInfo.cs
- PaperSize.cs
- CollectionViewGroupInternal.cs
- GeneralTransformCollection.cs
- CodeEventReferenceExpression.cs
- sqlmetadatafactory.cs
- DesignerAttribute.cs
- ConfigXmlSignificantWhitespace.cs
- TextLineResult.cs
- codemethodreferenceexpression.cs
- SequentialWorkflowHeaderFooter.cs
- DbConnectionHelper.cs
- DiscoveryClientReferences.cs
- XmlStringTable.cs
- ManifestResourceInfo.cs
- VirtualizingStackPanel.cs
- ServicePoint.cs
- DesignerDataSourceView.cs
- HealthMonitoringSection.cs
- MultiPageTextView.cs
- DispatchOperationRuntime.cs
- TextServicesHost.cs
- SafeHandles.cs
- FrameSecurityDescriptor.cs
- AvTrace.cs
- PropertyMetadata.cs
- RoleManagerEventArgs.cs
- SqlNotificationEventArgs.cs
- FixedFlowMap.cs
- KeyValueInternalCollection.cs
- ColumnHeaderConverter.cs
- CustomErrorsSection.cs
- EventDescriptor.cs
- WindowsNonControl.cs
- EntityWrapper.cs
- ToolStripControlHost.cs
- DBParameter.cs
- EntityCommandDefinition.cs
- SettingsAttributes.cs
- TextModifierScope.cs
- GatewayIPAddressInformationCollection.cs
- SerialStream.cs
- XAMLParseException.cs
- SessionParameter.cs
- WindowsScrollBar.cs
- Substitution.cs
- ControlSerializer.cs
- WindowsStatic.cs
- SetState.cs
- EventEntry.cs
- RelationshipFixer.cs
- HtmlTextArea.cs
- util.cs
- AssociationType.cs
- X509Certificate2Collection.cs
- SingleKeyFrameCollection.cs
- FormViewDeletedEventArgs.cs
- BindingValueChangedEventArgs.cs
- ReliableDuplexSessionChannel.cs
- UnrecognizedPolicyAssertionElement.cs
- CheckoutException.cs
- FilterableAttribute.cs
- CompilerError.cs
- PersistenceContext.cs
- HtmlElementErrorEventArgs.cs
- RepeatButton.cs
- EncoderParameters.cs
- BaseParaClient.cs
- ExecutionContext.cs
- Serializer.cs
- DictionarySectionHandler.cs
- WebPartCancelEventArgs.cs
- OutputCacheModule.cs
- PerformanceCounter.cs
- SpellerError.cs
- BindingContext.cs
- RegexParser.cs
- TargetParameterCountException.cs
- AutomationProperty.cs
- SymbolType.cs