Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / EncoderParameters.cs / 1 / EncoderParameters.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing.Internal; using Marshal = System.Runtime.InteropServices.Marshal; using System.Drawing; //[StructLayout(LayoutKind.Sequential)] ////// /// public sealed class EncoderParameters : IDisposable { EncoderParameter[] param; ///[To be supplied.] ////// /// public EncoderParameters(int count) { param = new EncoderParameter[count]; } ///[To be supplied.] ////// /// public EncoderParameters() { param = new EncoderParameter[1]; } ///[To be supplied.] ////// /// public EncoderParameter[] Param { // get { return param; } set { param = value; } } ///[To be supplied.] ////// Copy the EncoderParameters data into a chunk of memory to be consumed by native GDI+ code. /// /// We need to marshal the EncoderParameters info from/to native GDI+ ourselve since the definition of the managed/unmanaged classes /// are different and the native class is a bit weird. The native EncoderParameters class is defined in GDI+ as follows: /// /// class EncoderParameters { /// UINT Count; // Number of parameters in this structure /// EncoderParameter Parameter[1]; // Parameter values /// }; /// /// We don't have the 'Count' field since the managed array contains it. In order for this structure to work with more than one /// EncoderParameter we need to preallocate memory for the extra n-1 elements, something like this: /// /// EncoderParameters* pEncoderParameters = (EncoderParameters*) malloc(sizeof(EncoderParameters) + (n-1) * sizeof(EncoderParameter)); /// /// Also, in 64-bit platforms, 'Count' is aligned in 8 bytes (4 extra padding bytes) so we use IntPtr instead of Int32 to account for /// that (See VSW#451333). /// internal IntPtr ConvertToMemory() { int size = Marshal.SizeOf(typeof(EncoderParameter)); IntPtr memory = Marshal.AllocHGlobal(param.Length * size + Marshal.SizeOf(typeof(IntPtr))); if (memory == IntPtr.Zero){ throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); } Marshal.WriteIntPtr(memory, (IntPtr) param.Length); long arrayOffset = (long) memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i/// Copy the native GDI+ EncoderParameters data from a chunk of memory into a managed EncoderParameters object. /// See ConvertToMemory for more info. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] internal static EncoderParameters ConvertFromMemory(IntPtr memory) { if (memory == IntPtr.Zero) { throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); } int count = Marshal.ReadIntPtr(memory).ToInt32(); EncoderParameters p = new EncoderParameters(count); int size = Marshal.SizeOf(typeof(EncoderParameter)); long arrayOffset = (long)memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i public void Dispose() { foreach (EncoderParameter p in param) { if( p != null ){ p.Dispose(); } } param = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing.Internal; using Marshal = System.Runtime.InteropServices.Marshal; using System.Drawing; //[StructLayout(LayoutKind.Sequential)] ////// /// public sealed class EncoderParameters : IDisposable { EncoderParameter[] param; ///[To be supplied.] ////// /// public EncoderParameters(int count) { param = new EncoderParameter[count]; } ///[To be supplied.] ////// /// public EncoderParameters() { param = new EncoderParameter[1]; } ///[To be supplied.] ////// /// public EncoderParameter[] Param { // get { return param; } set { param = value; } } ///[To be supplied.] ////// Copy the EncoderParameters data into a chunk of memory to be consumed by native GDI+ code. /// /// We need to marshal the EncoderParameters info from/to native GDI+ ourselve since the definition of the managed/unmanaged classes /// are different and the native class is a bit weird. The native EncoderParameters class is defined in GDI+ as follows: /// /// class EncoderParameters { /// UINT Count; // Number of parameters in this structure /// EncoderParameter Parameter[1]; // Parameter values /// }; /// /// We don't have the 'Count' field since the managed array contains it. In order for this structure to work with more than one /// EncoderParameter we need to preallocate memory for the extra n-1 elements, something like this: /// /// EncoderParameters* pEncoderParameters = (EncoderParameters*) malloc(sizeof(EncoderParameters) + (n-1) * sizeof(EncoderParameter)); /// /// Also, in 64-bit platforms, 'Count' is aligned in 8 bytes (4 extra padding bytes) so we use IntPtr instead of Int32 to account for /// that (See VSW#451333). /// internal IntPtr ConvertToMemory() { int size = Marshal.SizeOf(typeof(EncoderParameter)); IntPtr memory = Marshal.AllocHGlobal(param.Length * size + Marshal.SizeOf(typeof(IntPtr))); if (memory == IntPtr.Zero){ throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); } Marshal.WriteIntPtr(memory, (IntPtr) param.Length); long arrayOffset = (long) memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i/// Copy the native GDI+ EncoderParameters data from a chunk of memory into a managed EncoderParameters object. /// See ConvertToMemory for more info. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] internal static EncoderParameters ConvertFromMemory(IntPtr memory) { if (memory == IntPtr.Zero) { throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); } int count = Marshal.ReadIntPtr(memory).ToInt32(); EncoderParameters p = new EncoderParameters(count); int size = Marshal.SizeOf(typeof(EncoderParameter)); long arrayOffset = (long)memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i public void Dispose() { foreach (EncoderParameter p in param) { if( p != null ){ p.Dispose(); } } param = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
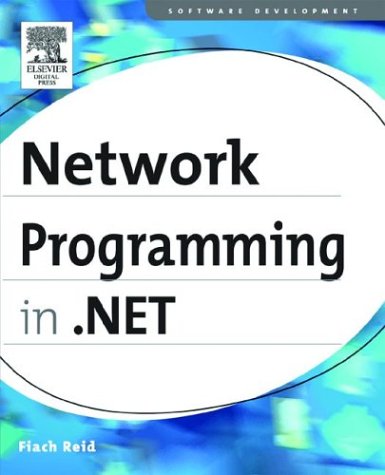
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttributeProviderAttribute.cs
- TreeWalker.cs
- XslAstAnalyzer.cs
- FormViewDesigner.cs
- ResourceManagerWrapper.cs
- _SafeNetHandles.cs
- IgnoreFlushAndCloseStream.cs
- ConnectionStringsExpressionBuilder.cs
- Content.cs
- CallbackValidatorAttribute.cs
- XmlHierarchyData.cs
- LabelExpression.cs
- MappedMetaModel.cs
- TypeToken.cs
- ConnectionPoolManager.cs
- HostingEnvironmentException.cs
- XmlExtensionFunction.cs
- RegularExpressionValidator.cs
- ClipboardData.cs
- TableRow.cs
- SqlMethodTransformer.cs
- Quaternion.cs
- SynchronizedInputProviderWrapper.cs
- ViewSimplifier.cs
- Triplet.cs
- MasterPageCodeDomTreeGenerator.cs
- GlyphRun.cs
- GlobalItem.cs
- SpecularMaterial.cs
- CodeTypeReference.cs
- TextTreeRootNode.cs
- PasswordTextNavigator.cs
- ListViewContainer.cs
- WebHttpEndpointElement.cs
- Vars.cs
- _NestedSingleAsyncResult.cs
- CheckBoxList.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- Propagator.JoinPropagator.cs
- DSASignatureDeformatter.cs
- SkipQueryOptionExpression.cs
- NumericUpDownAcceleration.cs
- ManagedIStream.cs
- SingleAnimationBase.cs
- DetailsViewInsertedEventArgs.cs
- ObjectQueryProvider.cs
- SettingsPropertyIsReadOnlyException.cs
- ItemContainerGenerator.cs
- LocalFileSettingsProvider.cs
- MinMaxParagraphWidth.cs
- ConstructorArgumentAttribute.cs
- ColorTransform.cs
- EditorZoneDesigner.cs
- ConversionHelper.cs
- DataGridItemCollection.cs
- ChtmlCalendarAdapter.cs
- PageRequestManager.cs
- QilSortKey.cs
- ContextStack.cs
- ContentFilePart.cs
- DoWorkEventArgs.cs
- NameValueConfigurationElement.cs
- Mappings.cs
- InternalBufferOverflowException.cs
- DefaultSection.cs
- ComboBox.cs
- DriveNotFoundException.cs
- DocumentXmlWriter.cs
- XmlDataDocument.cs
- WinInet.cs
- StreamAsIStream.cs
- CookielessData.cs
- SoapHeaders.cs
- TreeNodeConverter.cs
- DesignerAttribute.cs
- SectionInput.cs
- ObjectDataSourceDisposingEventArgs.cs
- EntityContainer.cs
- SortedList.cs
- SafeHandles.cs
- ExpressionBinding.cs
- SafeNativeMethodsOther.cs
- ThaiBuddhistCalendar.cs
- DESCryptoServiceProvider.cs
- OneOfElement.cs
- ToolboxItemFilterAttribute.cs
- InvalidEnumArgumentException.cs
- Material.cs
- WebPartConnectionsCancelVerb.cs
- Subtree.cs
- DocumentCollection.cs
- _CommandStream.cs
- UIElementCollection.cs
- _ContextAwareResult.cs
- HtmlControl.cs
- ProcessHostMapPath.cs
- Int32Converter.cs
- GenericEnumConverter.cs
- ConfigXmlElement.cs
- EUCJPEncoding.cs